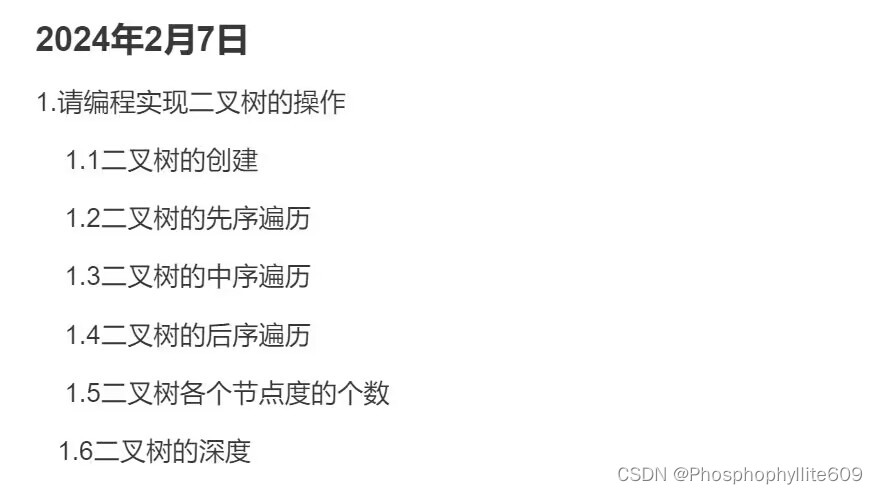
源代码:
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
typedef char datatype;
typedef struct Node
{
datatype data;
struct Node *lchild;
struct Node *rchild;
}*Btree;
Btree creat_node()
{
Btree s=(Btree)malloc(sizeof(struct Node));
if(s==NULL)
return NULL;
s->data='\0';
s->lchild=NULL;
s->rchild=NULL;
return s;
}
Btree creat_tree()
{
datatype element;
printf("please enter element:");
scanf(" %c",&element);
if(element=='#')
return NULL;
Btree tree =creat_node();
tree->data=element;
tree->lchild=creat_tree();
tree->rchild=creat_tree();
return tree;
}
void first_tree(Btree tree)
{
if(tree==NULL)
return ;
printf("%-4c",tree->data);
first_tree(tree->lchild);
first_tree(tree->rchild);
}
void mid_tree(Btree tree)
{
if(tree==NULL)
return ;
mid_tree(tree->lchild);
printf("%-4c",tree->data);
mid_tree(tree->rchild);
}
void last_tree(Btree tree)
{
if(tree==NULL)
return ;
mid_tree(tree->lchild);
mid_tree(tree->rchild);
printf("%-4c",tree->data);
}
void Count(Btree tree ,int *n0,int *n1 ,int *n2)
{
if(tree==NULL)
return;
if(!tree->lchild && !tree->rchild)
++*n0;
else if(tree->lchild && tree->rchild)
++*n2;
else
++*n1;
Count(tree->lchild,n0,n1,n2);
Count(tree->rchild,n0,n1,n2);
}
int high(Btree tree)
{
if(tree==NULL)
return 0;
int left=1+high(tree->lchild);
int right=1+high(tree->rchild);
return left>right?left:right;
}
int main(int argc,char *argv[])
{
Btree tree =creat_tree();
first_tree(tree);
puts("");
mid_tree(tree);
puts("");
last_tree(tree);
puts("");
int n0,n1,n2;
n0=n1=n2=0;
Count(tree,&n0,&n1,&n2);
printf("n0=%d,n1=%d,n2=%d\n",n0,n1,n2);
int high_tree=high(tree);
printf("high=%d\n",high_tree);
return 0;
}
效果图:
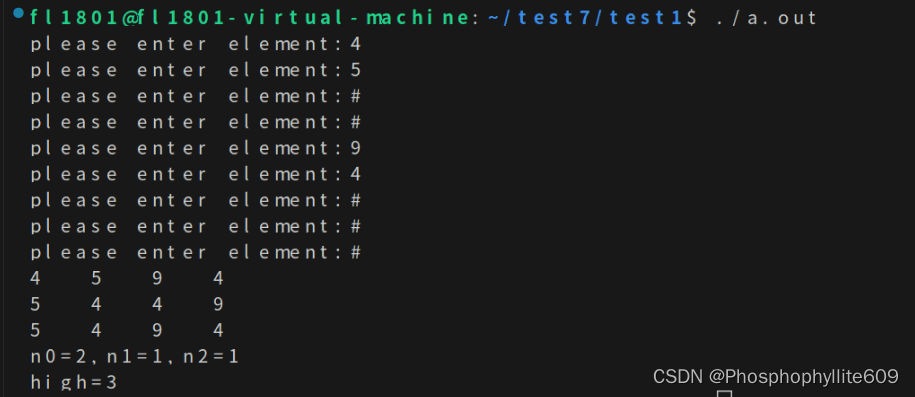