1. 什么是 SPA
- 之前关于 VueRouter 的文章里写过,这里就不过多叙述了 跳转目标文章
2. 通过 hash 实现
2.1 实现思路
- 改变 url 的 Hash 值,也就是 # 后面的路径
- 通过 hashchange 来监测变化,做出对应的操作
2.2 代码实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hash</title>
<style>
footer {
position: fixed;
left: 0;
bottom: 0;
display: flex;
justify-content: space-between;
width: 100%;
}
footer .box {
display: flex;
justify-content: center;
width: 100px;
height: 60px;
text-align: center;
border: 1px solid #ccc;
box-sizing: border-box;
}
footer .box button {
background-color: white;
border: none;
outline: none;
}
</style>
</head>
<body>
<div class="contaienr">
<div class="content">我是内容区域哈哈哈</div>
<footer>
<div class="box">
<button onclick="to('home')">首页</button>
</div>
<div class="box">
<button onclick="to('mall')">商城</button>
</div>
<div class="box">
<button onclick="to('shopping')">购物车</button>
</div>
<div class="box">
<button onclick="to('user')">我的</button>
</div>
</footer>
</div>
<script>
class MyHash {
constructor(routes) {
this.routes = routes;
this.watchHash();
}
to(path) {
location.hash = path;
}
watchHash() {
window.addEventListener("hashchange", (e) => {
let { newURL } = e;
let path = newURL.split("#")[1];
let { component } = this.routes.find((item) => item.path == path);
content.innerHTML = component();
});
}
}
let content = document.querySelector(".content");
let myHash = new MyHash([
{
path: "home",
component: () => `<h1>我是首页</h1>`,
},
{
path: "mall",
component: () => `<h1>我是商城页</h1>`,
},
{
path: "shopping",
component: () => `<h1>我是购物车页</h1>`,
},
{
path: "user",
component: () => `<h1>我是用户页</h1>`,
},
]);
function to(path) {
myHash.to(path);
}
</script>
</body>
</html>
3. 通过 history 实现
3.1 实现思路
- 在 window 上有一个 history 对象,使用该对象的 pushState、replaceState方法时,对浏览器的历史记录进行操作,页面并不会进行刷新
- 而使用
history.go
,history.back(工具栏的后退)
,history.forward(工具栏的前进)
时会触发 window 上的 popstate 方法,页面也不会进行刷新
3.2 代码实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>history</title>
<style>
footer {
position: fixed;
left: 0;
bottom: 0;
display: flex;
justify-content: space-between;
width: 100%;
}
footer .box {
display: flex;
justify-content: center;
width: 100px;
height: 60px;
text-align: center;
border: 1px solid #ccc;
box-sizing: border-box;
}
footer .box button {
background-color: white;
border: none;
outline: none;
}
</style>
</head>
<body>
<div class="contaienr">
<div class="content">我是内容区域哈哈哈</div>
<footer>
<div class="box"><button onclick="to('home')">首页</button></div>
<div class="box"><button onclick="to('mall')">商城</button></div>
<div class="box"><button onclick="to('shopping')">购物车</button></div>
<div class="box">
<button onclick="to('user','replaceState')">我的</button>
</div>
</footer>
</div>
<script>
class MyHistory {
constructor(routes) {
this.routes = routes;
this.watchHistory();
}
rewriteApi(path, type) {
history[type]({ path }, "", "/" + path);
this.matchCurRoute(path);
}
watchHistory() {
window.addEventListener("popstate", (e) => {
this.matchCurRoute(e.state.path);
});
}
matchCurRoute(path) {
let { component } = this.routes.find((item) => item.path == path);
content.innerHTML = component();
}
}
let content = document.querySelector(".content");
let myHistory = new MyHistory([
{
path: "home",
component: () => `<h1>我是首页</h1>`,
},
{
path: "mall",
component: () => `<h1>我是商城页</h1>`,
},
{
path: "shopping",
component: () => `<h1>我是购物车页</h1>`,
},
{
path: "user",
component: () => `<h1>我是用户页</h1>`,
},
]);
function to(path, type = "pushState") {
myHistory.rewriteApi(path, type);
}
</script>
</body>
</html>
4. 效果图
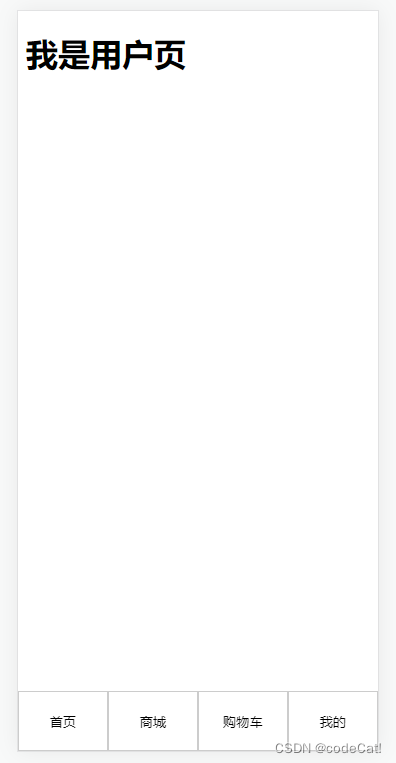