该小说网站运用Springboot,redis和Mybatis技术,前台为JSP技术编写!该网站完成了基本的需求,但也存在许多的不足😐😐,比如后台数据库设计的不是太合理等,感兴趣的可以分享源码给你们,在此基础上修该完善!前台页面借鉴了一些博主的JSP页面,然后自己改了该的,但没记住是哪位大神,至此表示感谢!😘评论区私信免费分享源码
小说前台页面展示
登录页面
一个简单的登录页面😓,这里用了js和cookie缓存,实现了记住密码和自动登录功能!
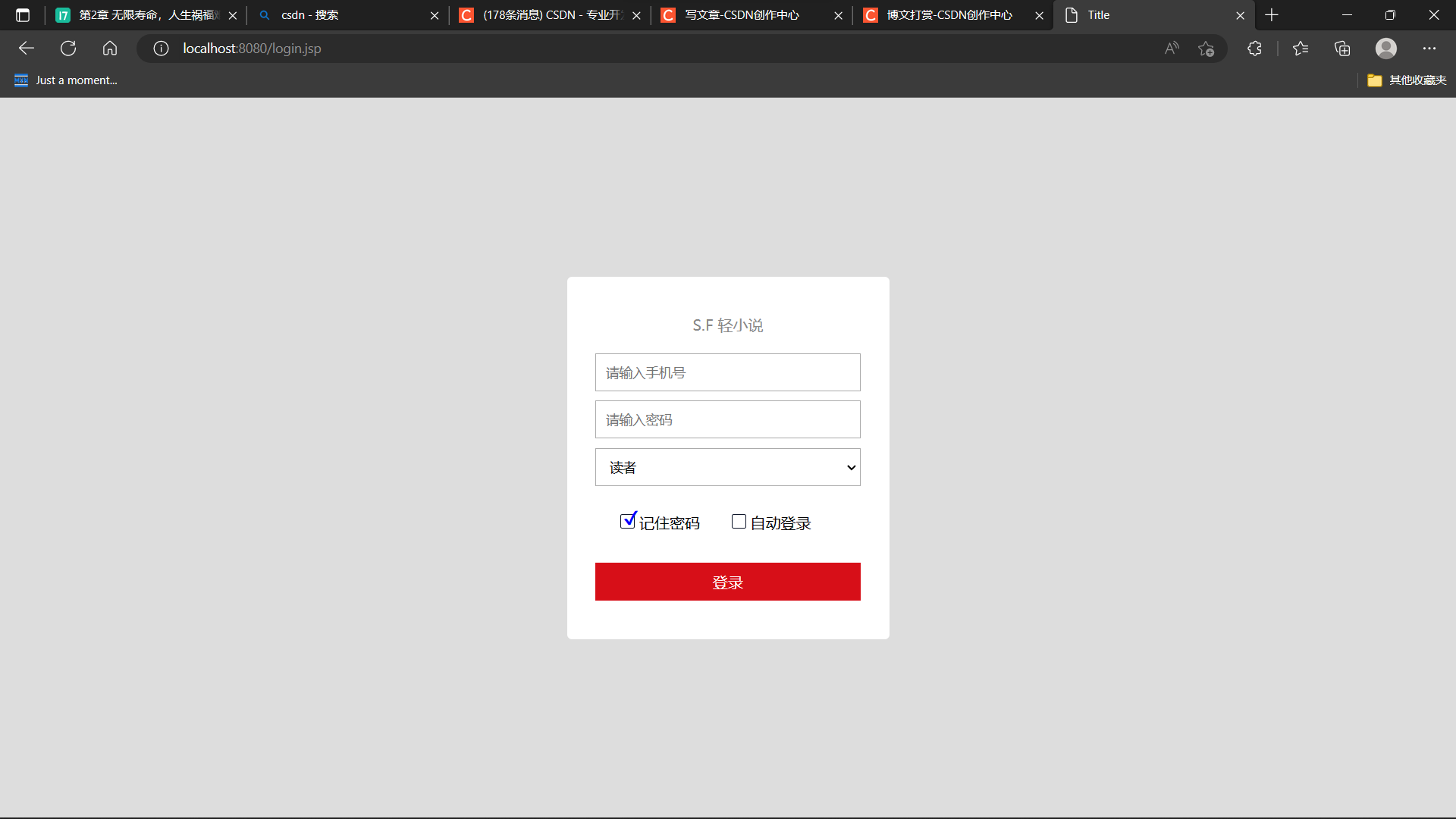
下面都是一些前端页面展示💯
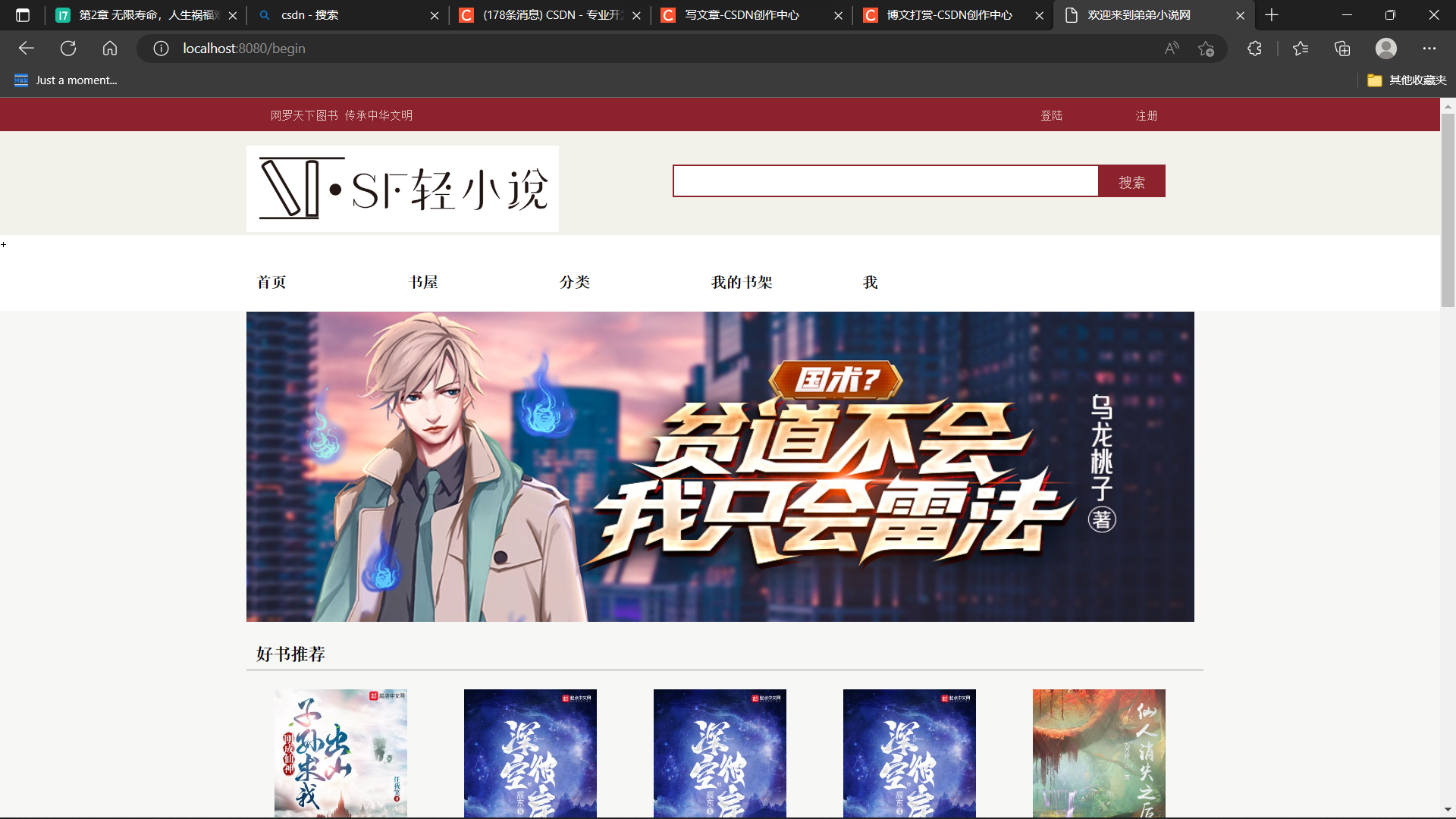
logo是从网上粘贴
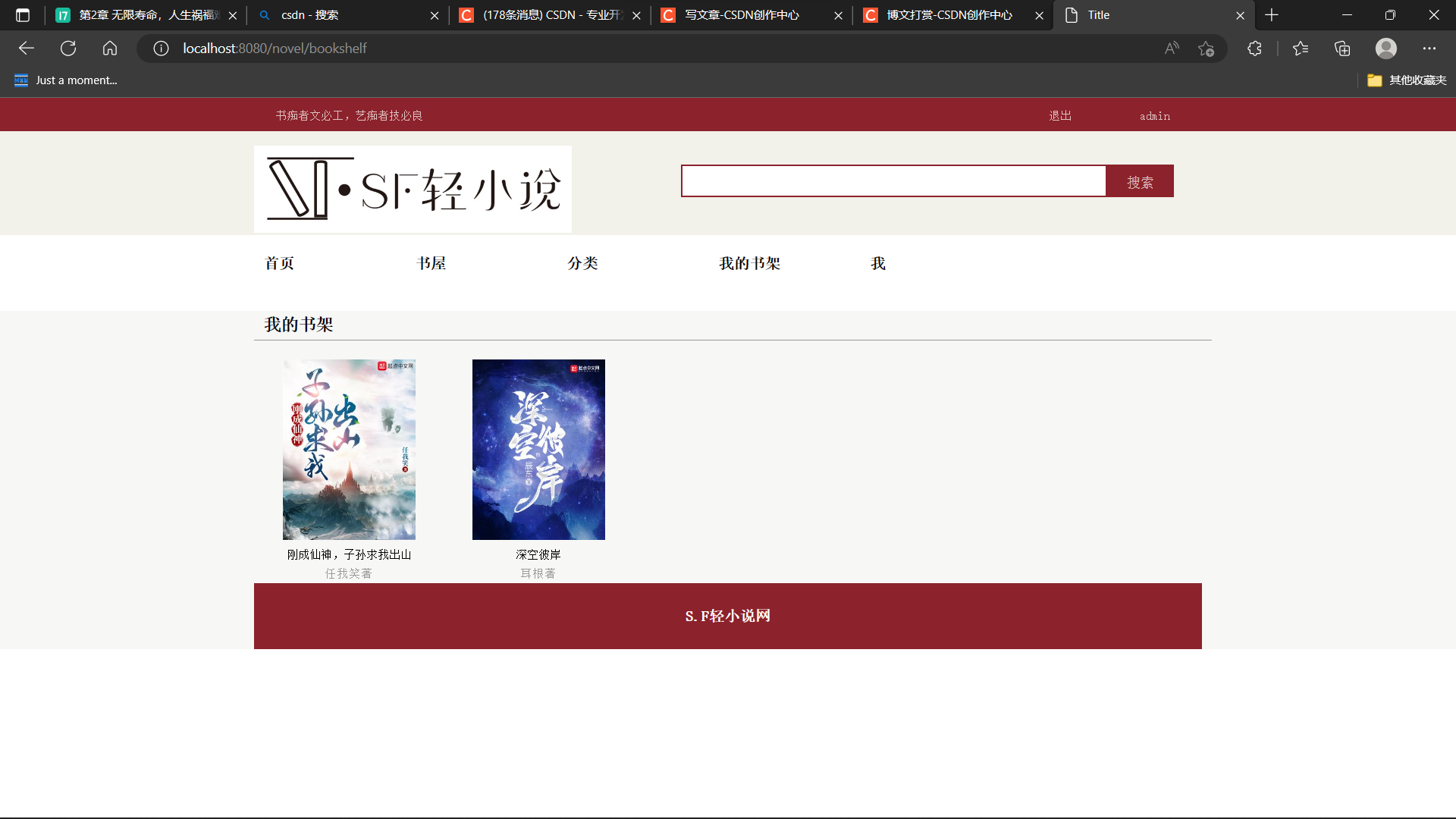
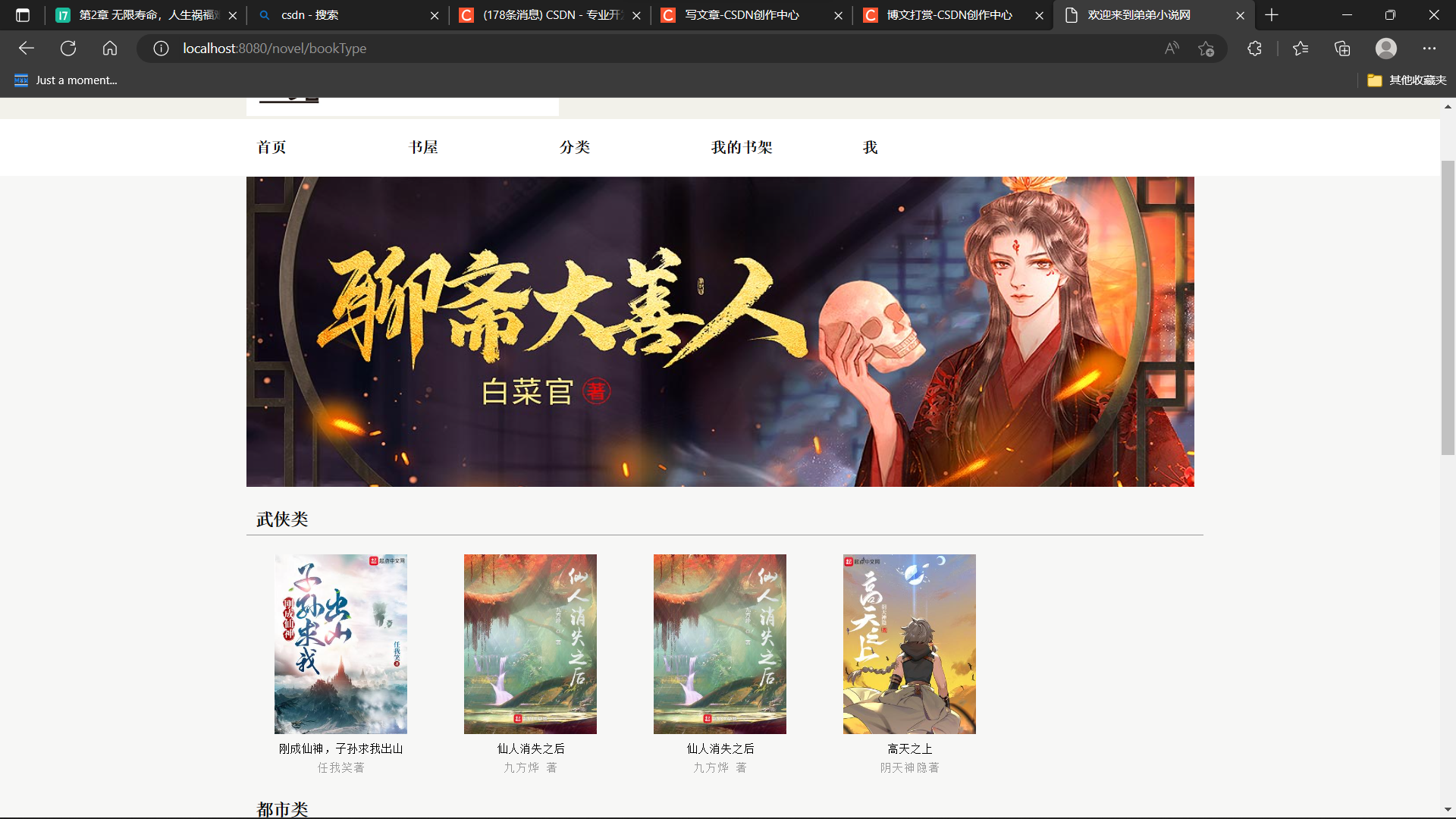
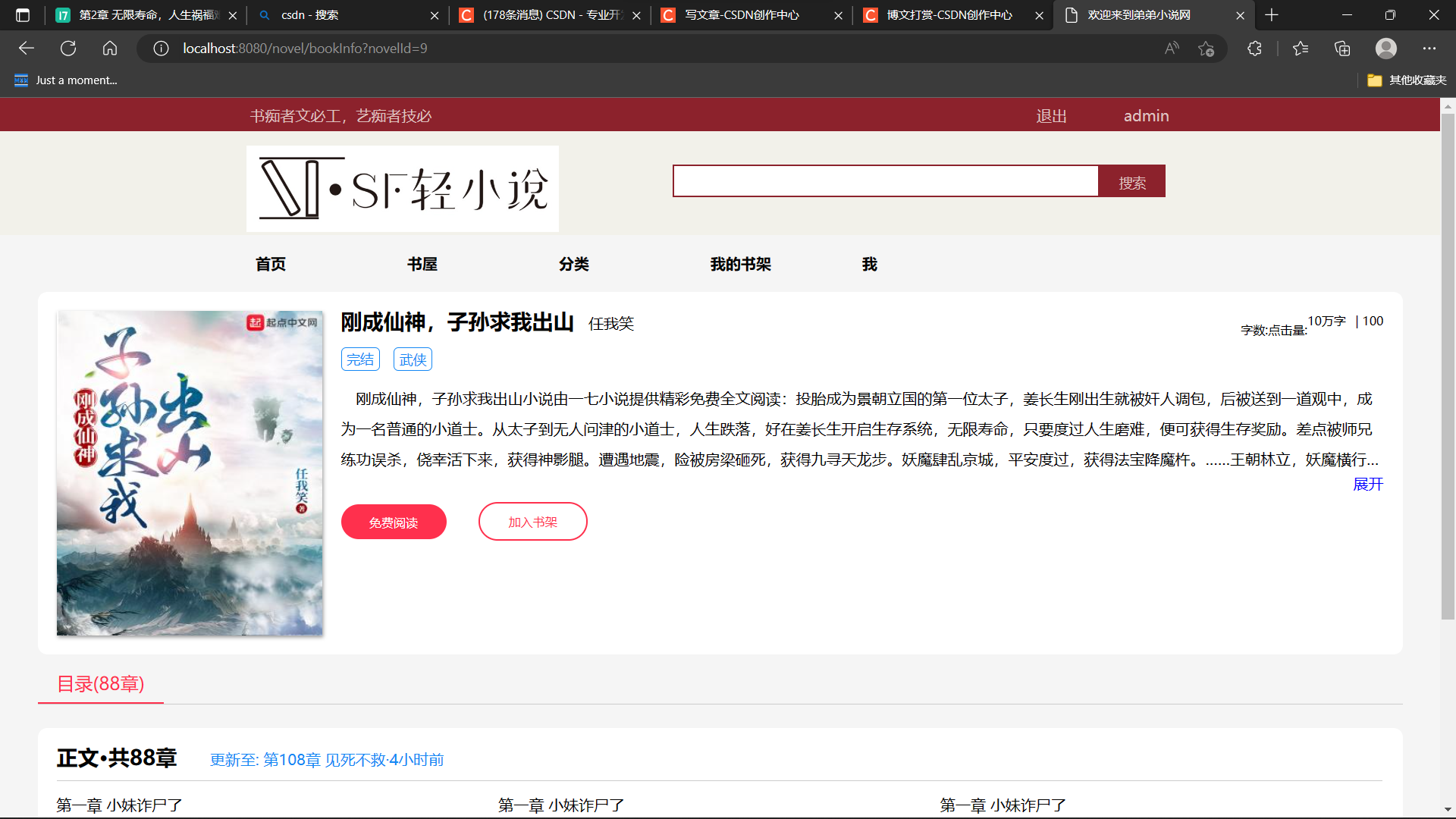
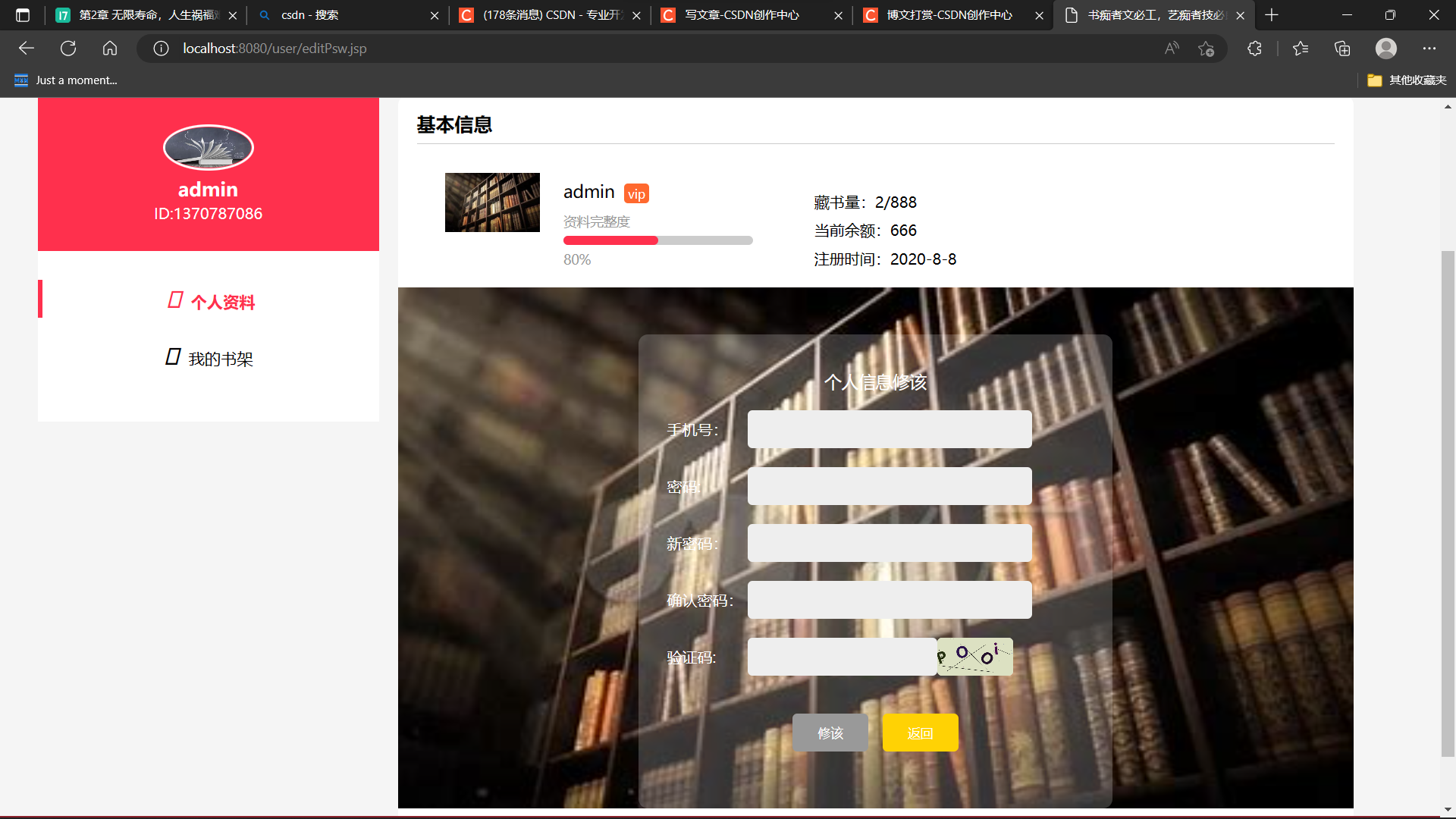
小说后台页面展示
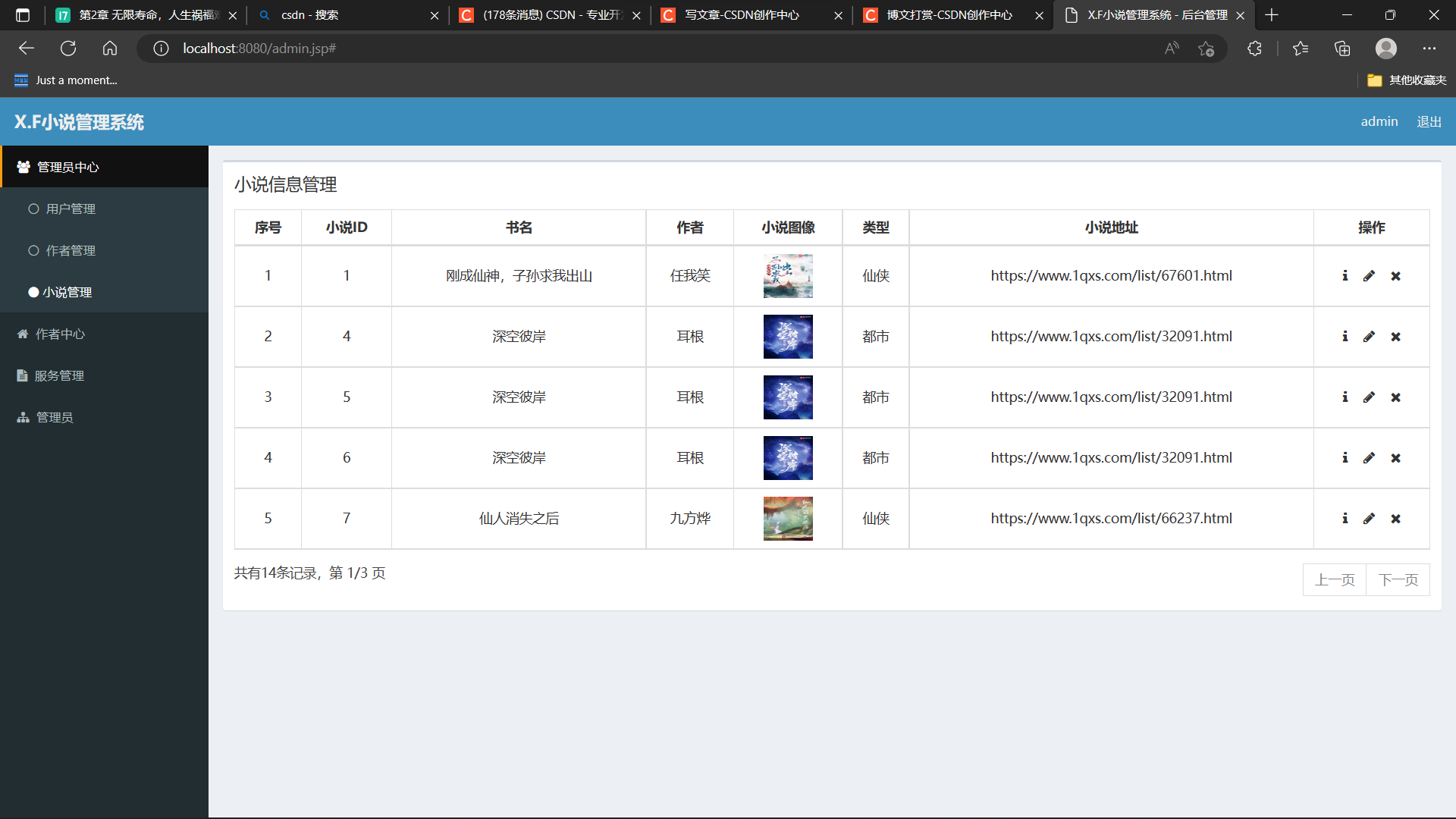
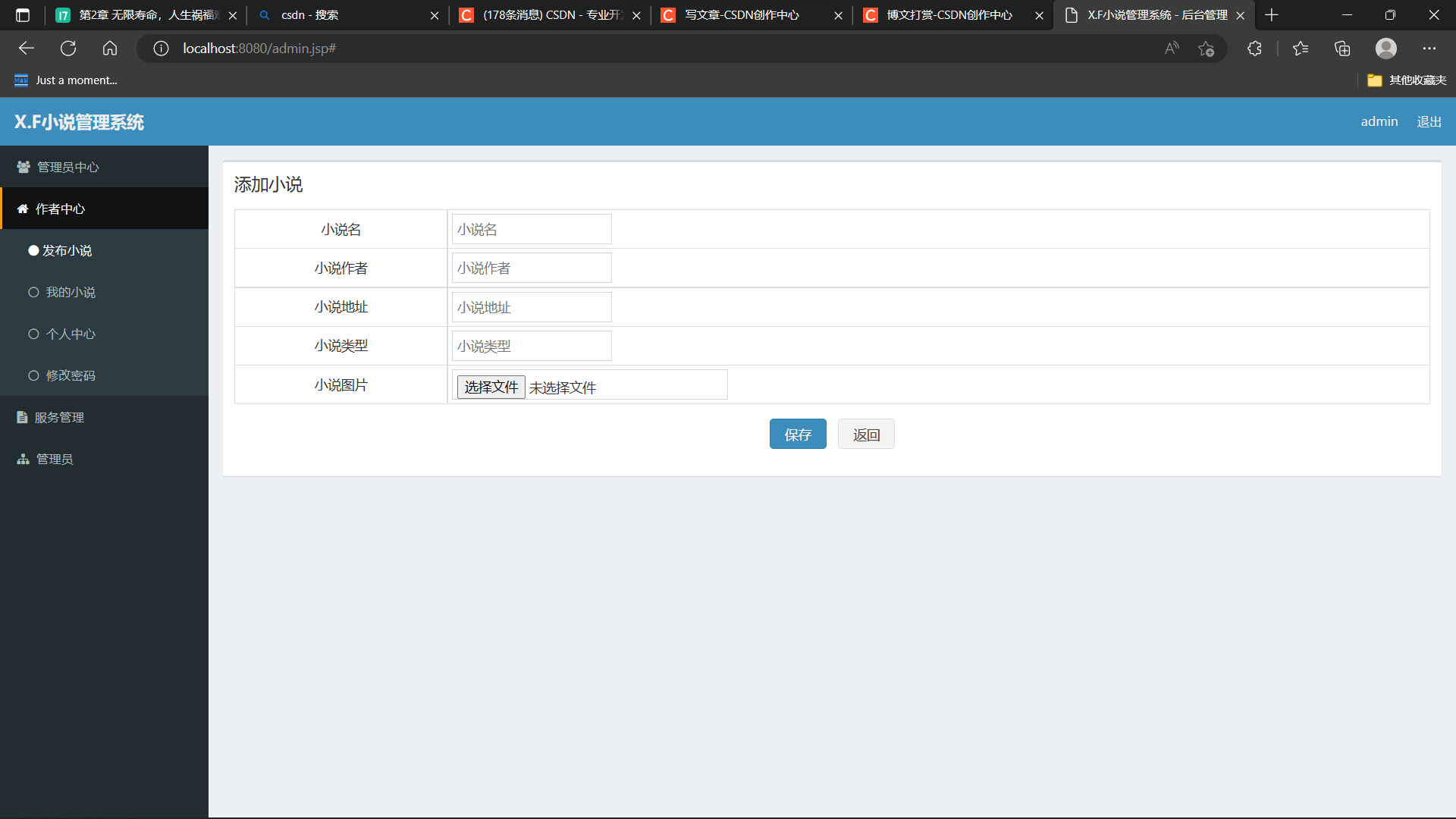
别的就不展示了,第一次搞,本人还是学生,没事瞎搞的😂,部分源码见下方:
源码展示
1所需要的依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.novel</groupId>
<artifactId>novel</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<name>novel</name>
<description>novel</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.8</version>
</dependency>
<dependency>
<groupId>org.projectlombok </groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!--解析jsp-->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
</dependency>
<!--redis-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/com.alibaba/fastjson -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>2.0.20</version>
</dependency>
<!--分页查询 -->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.4.6</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.7.6</version>
</plugin>
</plugins>
</build>
</project>
2.部分前台控制层代码
package com.novel.controller;
import com.novel.entity.Novel;
import com.novel.entity.page;
import com.novel.service.novelService;
import org.apache.ibatis.annotations.Param;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.data.domain.Pageable;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.List;
@Controller
@RequestMapping("/novel")
public class novel {
@Autowired
private novelService novelService;
@GetMapping("/add")
public void add(@Param("novelId") String novelId, HttpServletRequest request,HttpServletResponse response) throws IOException {
int Id=Integer.parseInt(novelId);
HttpSession session=request.getSession();
int userId=(Integer)session.getAttribute("Id");
int n=novelService.addBookshelf(Id,userId);
response.setContentType("text/html; charset=UTF-8");
PrintWriter writer=response.getWriter();
if(n!=0){
writer.println("<script>");
writer.println("alert('添加成功!');");
writer.println("history.back();");
writer.println("</script>");
}else {
writer.println("<script>");
writer.println("alert('添加失败,重新尝试或联系管理员!');");
writer.println("history.back();");
writer.println("</script>");
}
}
@GetMapping("/bookshelf")
public String bookshelf(HttpServletRequest request, Model model){
HttpSession session=request.getSession();
int Id=(Integer) session.getAttribute("Id");
List<Novel> bookshelf=novelService.queryBookshelf(Id);
model.addAttribute("bookshelf",bookshelf);
return "forward:/novel/bookshelf.jsp";
}
@GetMapping("/bookInfo")
public String bookInfo(HttpServletRequest request,@Param("novelId")String novelId,Model model){
int Id=Integer.parseInt( novelId);
Novel novel=novelService.queryById(Id);
HttpSession session=request.getSession();
int userId=(Integer) session.getAttribute("Id");
if(novelService.queryBook(userId,Id)!=0){
model.addAttribute("judge",1);
}else {
model.addAttribute("judge",0);
}
model.addAttribute("bookInfo",novel);
return "forward:/novel/bookInfo.jsp";
}
@GetMapping("/delete")
public String delete(@Param("userId") String userId,@Param("novelId")String novelId){
System.out.println(userId+"::"+novelId);
int n= novelService.delete(Integer.parseInt(userId),Integer.parseInt(novelId));
if(n!=0){
return "redirect:/novel/bookshelf";
}
return null;
}
@GetMapping("/bookall")
public String bookall(Model model,Integer currentPage){
int size=10;
if(currentPage==null){
currentPage=1;
}
Pageable pageable= PageRequest.of(currentPage-1,10);
page<Novel> page=novelService.findPage(pageable);
page.setCurrent_page(currentPage);
model.addAttribute("pageInfo",page);
return "forward:/novel/bookall.jsp";
}
@GetMapping("/search")
public String search(@Param("novelName") String novelName,Model model){
Novel novel=new Novel();
if (novelName.equals("")){
return "forward:/novel/sou.jsp";
}else {
novel.setNovelName(novelName);
List<Novel> list=novelService.search(novel);
model.addAttribute("list",list);
return "forward:/novel/sou.jsp";
}
}
@GetMapping("/bookType")
public String bookType(Model model){
List<Novel> list=novelService.search(new Novel());
model.addAttribute("list",list);
return "forward:/novel/bookType.jsp";
}
}
3.部分后台控制层源码
import org.springframework.web.bind.annotation.RequestMapping;
import javax.annotation.Resource;
@Controller
@RequestMapping("/admin")
public class admin {
@Resource
private adminService adminService;
@GetMapping("/{type}/{pageNum}")
public String pageInfo(@PathVariable("type") String type, @PathVariable("pageNum") Integer pageNum,Model model){
if(pageNum==null){
pageNum=1;
}
System.out.println(type);
model.addAttribute("pageInfo",adminService.pageInfo(pageNum, type));
return "forward:/admin/"+type+".jsp";
}
@GetMapping("/delete/{type}/{Id}/{pageNum}")
public String delete(@PathVariable("type") String type,@PathVariable("Id") String Id,@PathVariable("pageNum") String pageNum){
adminService.delete(type,Id);
return "redirect:/admin/"+type+"?pageNum="+pageNum;
}
@GetMapping("/edit/{type}/{Id}/{pageNum}")
public String edit(@PathVariable("type") String type,@PathVariable("Id") String Id,@PathVariable("pageNum") String pageNum,Model model){
if(type.equals("author")){
Author author=(Author) adminService.edit(type,Id);
model.addAttribute("object",author);
model.addAttribute("pageNum",pageNum);
model.addAttribute("entity","Author");
}else if(type.equals("user")){
User user=(User) adminService.edit(type,Id);
model.addAttribute("entity","User");
model.addAttribute("object",user);
model.addAttribute("pageNum",pageNum);
}
return "forward:/admin/edit.jsp";
}
@PostMapping("/update/{type}/{pageNum}")
public String Update(User user,Author author,@PathVariable("type") String type,@PathVariable("pageNum") String pageNum){
if(type.equals("user")){
adminService.update(user);
}else {
adminService.update(author);
}
return "redirect:/admin/"+type+"/"+pageNum;
}
}
4.Redis工具类部分源码,主要是序列化的实现
public <K, V> void add(K key, V value) {
try {
if (value != null) {
redisTemplate
.opsForValue()
.set(DEFAULT_KEY_PREFIX + key, JSON.toJSONString(value));
}
} catch (Exception e) {
throw new RuntimeException("数据缓存至redis失败");
}
}
/**
* 数据缓存至redis并设置过期时间
*
* @param key
* @param value
* @return
*/
public <K, V> void add(K key, V value, long timeout, TimeUnit unit) {
try {
if (value != null) {
redisTemplate
.opsForValue()
.set(DEFAULT_KEY_PREFIX + key, JSON.toJSONString(value), timeout, unit);
}
} catch (Exception e) {
throw new RuntimeException("数据缓存至redis失败");
}
}
/**
* 写入 hash-set,已经是key-value的键值,不能再写入为hash-set
*
* @param key must not be {@literal null}.
* @param subKey must not be {@literal null}.
* @param value 写入的值
*/
public <K, SK, V> void addHashCache(K key, SK subKey, V value) {
redisTemplate.opsForHash().put(DEFAULT_KEY_PREFIX + key, subKey, value);
}
/**
* 写入 hash-set,并设置过期时间
*
* @param key must not be {@literal null}.
* @param subKey must not be {@literal null}.
* @param value 写入的值
*/
public <K, SK, V> void addHashCache(K key, SK subKey, V value, long timeout, TimeUnit unit) {
redisTemplate.opsForHash().put(DEFAULT_KEY_PREFIX + key, subKey, value);
redisTemplate.expire(DEFAULT_KEY_PREFIX + key, timeout, unit);
}
5.前端部分源码展示:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%--
Created by IntelliJ IDEA.
User: 86177
Date: 2022/12/13
Time: 15:25
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
<link rel="stylesheet" href="/css/bookall.css">
</head>
<body>
<div class="top">
<div class="to">
<p>书痴者文必工,艺痴者技必良</p>
<ul>
<c:if test="${not empty sessionScope}">
<li> <a href="/user/userInfo.jsp">${sessionScope.username}</a></li>
<li> <a href="/user/logout">退出</a></li>
</c:if>
<c:if test="${empty sessionScope}">
<li><a href="/register.jsp">注册</a></li>
<li><a href="/login.jsp">登陆</a></li></ul>
</c:if>
</div><div class="clearit"></div>
</div>
<div class="lg">
<div class="to">
<div class="logo"><img src="/img/logo.jpg"/></div>
<div class="sou"><form action="/novel/search" method="get" id="mainForm"><input name="novelName" type="text" placeholder=""/><p><a href="javascript:void(0)" onclick="document.getElementById('mainForm').submit();return false;">搜索</a></p></form></div>
</div><div class="clearit"></div>
</div>
<div class="dao"><ul>
<li><a href="/index" class="active">首页</a></li>
<li><a href="/novel/bookall">书屋</a></li>
<li><a href="/novel/bookType">分类</a></li>
<li><a href="/novel/bookshelf">我的书架</a></li>
<li><a href="/user/userInfo">我</a></li></ul>
</div>
<div class="nav">
<div class="da">
<%-- <div class="ban"><img src="#" width="1000" height="327" /></div>--%>
<div class="hao"><h2>书屋子</h2>
<ul>
<c:forEach items="${requestScope.pageInfo.novel}" var="Novel">
<li><a href="/novel/bookInfo?novelId=${Novel.novelId}" ><img src="${Novel.novelImage}" width="140" height="190" /><br />${Novel.novelName}<br /><samp> ${Novel.novelAuthor}著</samp><br /></a></li>
</c:forEach>
</ul>
</div>
<div class="pager-info">
<div>共有${requestScope.pageInfo.total_count} 条记录,第 ${requestScope.pageInfo.current_page}/${requestScope.pageInfo.total_page} 页</div>
<div>
<ul class="pagination">
<li class="paginate_button previous disabled">
<a href="${pageContext.request.contextPath}/novel/bookall?currentPage=${requestScope.pageInfo.current_page-1}">上一页</a>
</li>
<li class="paginate_button next disabled">
<a href="${pageContext.request.contextPath}/novel/bookall?currentPage=${requestScope.pageInfo.current_page+1}">下一页</a>
</li>
</ul>
</div>
</div>
<div class="foot">S.F轻小说网</div>
</div>
</div>
</body>
</html>
项目结构
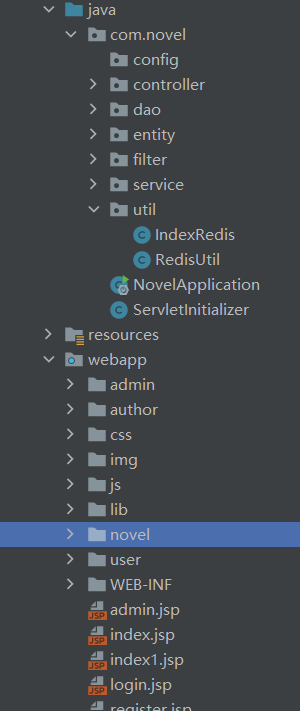
分享结束,感兴趣的可以关注打赏一波,私信分享源码!第一次发布,看着好像不咋地,抱歉抱歉!
😰😰😰😰😰😰😰