步骤分析:
- 获取相关元素
1.1 开始按钮
1.2 分数框
1.3 所有的地鼠洞 - 给开始按钮定义函数startGame
2.1 隐藏开始按钮
2.2 清除分数为0: 允许多次玩
2.3 开启定时器: 间隔时间自定义
2.3.1 获取随机整数: 0-地鼠洞长度之间
2.3.2 给随机数对应的地鼠洞添加up类(显示地鼠)
2.3.3 给当前地鼠洞增加一个延时器: 到时自动清除up类(隐藏地鼠) - 给所有的地鼠(mole)增加点击事件
3.1 获取所有的地鼠
3.2 给所有的地鼠绑定点击事件
3.3 干掉地鼠对应的洞的类up
3.4 增加分数的数量
3.5 清理地鼠所在洞本身的延时器
最终的效果:
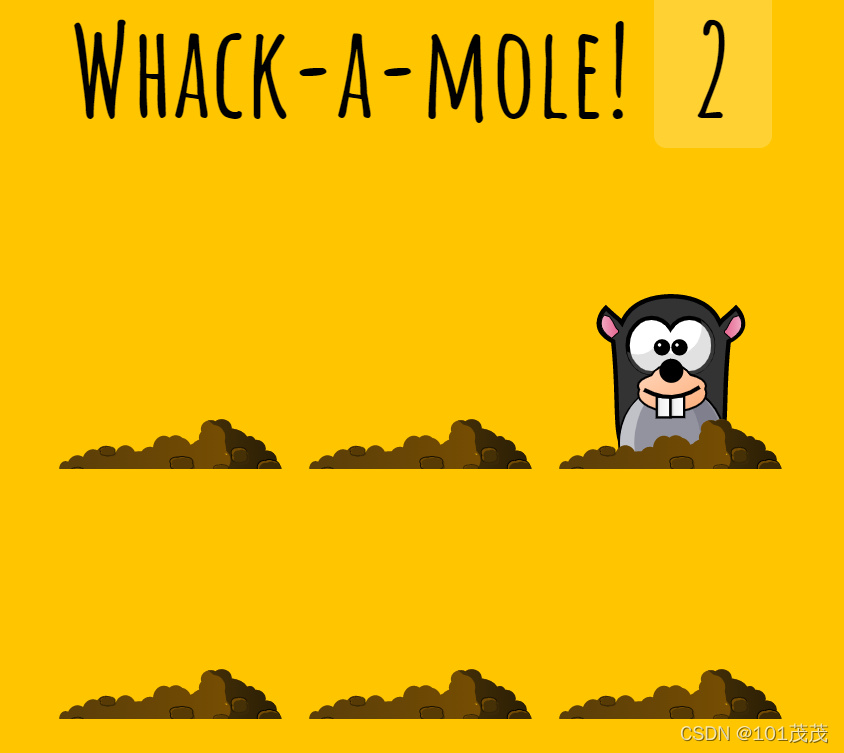
html部分
<h1>Whack-a-mole! <span class="score">0</span></h1>
<p><button class="start">Start</button></p>
<div class="game">
<div class="hole hole1">
<div class="mole"></div>
</div>
<div class="hole hole2">
<div class="mole"></div>
</div>
<div class="hole hole3">
<div class="mole"></div>
</div>
<div class="hole hole4">
<div class="mole"></div>
</div>
<div class="hole hole5">
<div class="mole"></div>
</div>
<div class="hole hole6">
<div class="mole"></div>
</div>
</div>
<h2 class="game-status"></h2>
//引入js文件
<script src="./index.js"></script>
css部分
html {
box-sizing: border-box;
font-size: 10px;
background: #ffc600;
}
*,
*:before,
*:after {
box-sizing: inherit;
}
body {
padding: 0;
margin: 0;
font-family: 'Amatic SC', cursive;
}
h1,
h2 {
text-align: center;
font-size: 10rem;
line-height: 1;
margin-bottom: 0;
}
h2 {
font-size: 8rem;
}
.score {
background: rgba(255, 255, 255, 0.2);
padding: 0 3rem;
line-height: 1;
border-radius: 1rem;
}
p {
height: 5rem;
text-align: center;
}
button {
margin: 0 auto;
width: 10rem;
font-size: 3rem;
font-family: 'Amatic SC', cursive;
-webkit-appearance: none;
appearance: none;
font-weight: bold;
background: rgba(255, 255, 255, 0.2);
border: 1px solid black;
border-radius: 1rem;
}
.game {
width: 600px;
height: 400px;
display: flex;
flex-wrap: wrap;
margin: 0 auto;
}
.hole {
flex: 1 0 33.33%;
overflow: hidden;
position: relative;
}
.hole:after {
display: block;
background: url(dirt.svg) bottom center no-repeat;
background-size: contain;
content: '';
width: 100%;
height: 70px;
position: absolute;
z-index: 2;
bottom: -30px;
}
.mole {
background: url('mole.svg') bottom center no-repeat;
background-size: 60%;
position: absolute;
top: 70%;
width: 100%;
height: 100%;
transition: all 0.4s;
}
.hole.up .mole {
top: 0;
}
js部分
window.addEventListener('load', () => {
let start = document.querySelector('.start')
let mole = document.querySelectorAll('.mole')
let score = document.querySelector('.score')
let timerId
let index
let num = 0
start.addEventListener('click', () => {
num = 0
score.innerHTML = num
start.style.display = 'none'
timerId = setInterval(() => {
index = parseInt(Math.random() * mole.length)
mole[index].style.top = '0%'
time = setTimeout(() => {
mole[index].style.top = '70%'
}, 500)
}, 1000)
setInterval(() => {
start.style.display = 'block'
clearInterval(timerId)
}, 10000)
})
mole.forEach((value, index) => {
value.addEventListener('click', () => {
mole[index].style.top = '70%'
num++
score.innerHTML = num
})
});