WEBGIS开发
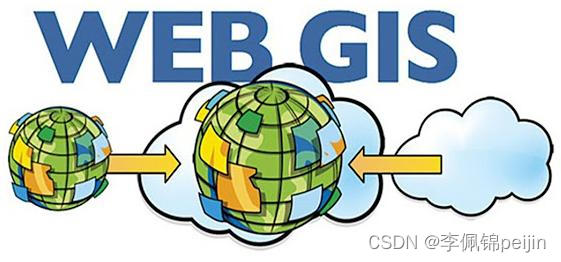
01、面向对象_类的定义
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 构造函数来定义,构造函数手写为大写字母
// 定义person类(没有任何属性以及方法)
function Person() {
}
// 通过类来创建一个实例对象
let p = new Person() // new 创建了一个Person实例
console.log(p)
console.log(typeof p) // object
</script>
</body>
</html>
02、面向对象_属性的定义
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function Person(name,age){
this.name = name
this.age = age
}
let p = new Person('john',23)
console.log(p.name,p.age
)
</script>
</body>
</html>
03、面向对象_行为的定义
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
function Person(name, age) {
// 定义属性
this.name = name
this.age = age
// 定义类的方法
this.eat = function () {
console.log('吃饭')
}
}
let p = new Person('张三', 40)
console.log(p.age)
p.eat()
p.name = '张三三'
console.log(p)
</script>
</body>
</html>
04、面向对象_字面量的定义
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 创建一个空对象
let p ={}
console.log(p)
console.log(typeof p)
// 对象中属性名都是字符串
let p1 = {
name:'张三',
age:23,
111:333,
eat:function(){
console.log('吃饭')
}
}
p1.eat()
// 添加属性
p1.sex = '男'
console.log(p1)
console.log(p1.sex)
console.log(p1['sex'])
console.log(p1['111'])
</script>
</body>
</html>
05、js中的内置Math对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// Math对象用于数学任务
// 属性
console.log(Math)
console.log(Math.PI) // 圆周率
console.log(Math.min(2,4,100,70,1)) // 返回一个最小的数
console.log(Math.max(2,4,100,70,1)) // 返回一个最大的数
console.log(Math.ceil(1.1)) // 对数字进行上舍入
console.log(Math.floor(1.99)) // 对数字进行下舍入
console.log(Math.round(1.5)) // 四舍五入
console.log(Math.random()) // 返回0-1之间的随机数
</script>
</body>
</html>
06、js中的内置Date对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 创建一个当前时间
let d1 = new Date()
console.log(d1) // Thu Jan 18 2024 10:04:33 GMT+0800 (中国标准时间)
// 指定时间
let d2 = new Date('2024-12-1')
console.log(d2)
// getFullYear() 从Date对象中 四位数字 返回年份
console.log(d1.getFullYear())
console.log(d1.getMonth(+1)) // 月
console.log(d1.getDate()) // 日
console.log(d1.getHours()) // 小时
console.log(d1.getMinutes())// 分钟
console.log(d1.getSeconds())// 秒钟
// 1970年1月1日 至今 毫秒数
console.log(d1.getTime()) // 时间戳
console.log(d2.getMonth())
</script>
</body>
</html>
07、Array对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 定义一个空数组
let arr = []
console.log(arr)
// 定义一个有元素的数组
let arr1 = [80,90,100]
console.log(arr1)
// 数组取值
console.log(arr1[1])
// 数组的长度
console.log(arr1.length)
// 遍历数组
for (let i = 0; i < arr1.length; i++) {
console.log(arr1[i])
}
// 数组循环
arr1.forEach((item,index) => {
console.log('索引:'+index,'值:'+item)
});
// 给数组的末尾添加一个元素
arr1.push(110)
arr1.push(120)
console.log(arr1)
// 删除数组的末尾元素
// arr.pop()
// console.log(arr)
// splice 从数组中添加或者删除元素(指定位置添加或删除)
// 第一个参数:必选 规定在哪里开始 删除/添加
// 第二个参数:可选 规定应该删除多少个元素。如果不写,从第一个参数位置开始删除到最后
// 第三个参数:可选 需要添加的新元素
// arr1.splice(2,0,95);
// 数组反转
// arr1.reverse()
// console.log(arr1)
// 返回某个值在数组中首次出现的位置
console.log(arr1.indexOf(100)) // 如果没有的话 返回-1
// join() 根据分隔符转成字符串
console.log(arr1.join('+')) // 默认用,分割
let arr2 = arr1.filter((item)=>{
return item>100
})
console.log(arr2)
let a = arr1.find((item)=>{
return item>110
})
console.log(a)
let oldArr = [100,80,80,2,1,3,1,5]
let newArr = []
oldArr.forEach(item => {
if(newArr.indexOf(item)==-1){
newArr.push(item)
}
});
console.log(newArr)
</script>
</body>
</html>
08、String对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
let str = 'asdfghjkla'
// length字符串的长度
console.log(str.length)
// chatAt() 返回指定位置的字符
console.log(str.charAt(3))
// indexOf 返回某个字符首次出现的位置
console.log(str.indexOf('1'))
// includes判断字符串中是否有这个字符 返回一个布尔值
console.log(str.includes('a'))
// substr 从索引位置开始切多少个字符 得到的是切出来的字符串
console.log(str.substr(3,5))
// 替换字符 不影响旧字符串
let newStr = str.replace('a','z')
console.log(newStr)
// split 根据字符将字符串分割成数组
console.log(str.split('')) // 传空字符串'' 会把每个字符当单个元素处理
</script>
</body>
</html>
09、Es6新特性 const关键字
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// const 声明的基本数据类型,值不能变(常量)
const age = 10
// age = 11
console.log(age)
// const 声明引用数据类型(数组,对象,方法)
// 可以在原来的数据上面进行修改,但是原本指向的内存地址不能变,即不能赋值一个新的数组
const arr = [1,2,3]
arr[2] = 100
console.log(arr)
// arr=[]
// 浏览器会对const声明做一些优化,const定义变量性能会变好
// 如果变量需要多次修改 就使用let定义
// var 不要出现(最原始的定义方法),可重复定义
// var a = 100
// var a = 200
// 引用数据类型布尔类型都是true,因为引用类型占用了内存地址
console.log(!!{})
</script>
</body>
</html>
10、Dom
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
app
</div>
<div class="one">one</div>
<div class="one">one</div>
<script>
// Dom document object model 文档对象模型
// Dom 是关于增删改查HTML元素的标准
// 查
// 通过id
const app = document.getElementById('app')
console.log(app)
// 通过类名获取到一个集合(跟数组相似)
const ones = document.getElementsByClassName('one')
console.log(ones[1])
// 通过标签名返回元素
const divs = document.getElementsByTagName('div')
console.log(divs)
// 返回文档中匹配的CSS选择器所有元素节点
const oness = document.querySelectorAll('.one')
console.log(oness)
</script>
</body>
</html>
11、增加一个节点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<p>1</p>
</div>
<script>
const app =document.getElementById('app')
// 1、创建一个元素
const p = document.createElement('p')
console.log(p)
// 2、给标签设置内容
p.innerHTML = '2'
console.log(p)
// 3、加上去
app.append(p) // 加在父元素的最后面
// p.innerHTML = '我被修改了' // 可以修改内容
const h1 = document.createElement('h1')
h1.innerText = '<button>点击<button>' //不能放标签内容,只能放文本
h1.innerHTML = '0' // 可以加标签内容
app.prepend(h1)
</script>
</body>
</html>
12、事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#app{
width: 100px;
height: 100px;
background-color: red;
}
</style>
</head>
<body>
<div id="app"></div>
<button>点击</button>
<h1>0</h1>
<script>
const btn = document.querySelector('button')
function btnClick(){
alert('我被那个了')
}
btn.onclick = btnClick
console.log(btn)
const app = document.querySelector('#app')
console.log(app)
app.onclick = function(){
app.style.backgroundColor = 'blue'
}
const h1 = document.querySelector('h1')
console.log(h1)
h1.onclick = function(){
h1.innerHTML++
}
</script>
</body>
</html>
13、修改节点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<p>hello</p>
</div>
<script>
const app = document.querySelector('#app')
// 创建新的 代替旧的
const p = document.querySelector('p')
const h1 = document.createElement('h1')
h1.innerHTML = 'change'
app.replaceChild(h1,p)
</script>
</body>
</html>
14、删除节点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<div class="one">one</div>
<div class="two">two</div>
<button>删除</button>
</div>
<script>
const btn = document.querySelector('button')
const app = document.querySelector('#app')
const one = document.querySelector('.one')
const two = document.querySelector('.two')
btn.onclick = function(){
// app.removeChild(one) //根据父元素删除节点
two.remove() //直接删除节点
}
</script>
</body>
</html>
15、灯泡切换
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<img src="./img/off.gif" alt="">
<script>
const img = document.querySelector('img')
// let count = 0
// img.onclick = function () {
// if (count % 2 == 1) {
// img.src = './img/on.gif'
// } else {
// img.src = './img/off.gif'
// }
// console.log(count)
// count += 1
// }
</script>
</body>
</html>
16、内联事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button onclick="go()">点击</button>
<script>
function go(){
alert('一刀999')
}
</script>
</body>
</html>
17、选中问题
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 100px;
height: 100px;
background-color: red;
margin: 20px auto;
color: white;
text-align: center;
font-size: 30px;
cursor: pointer;
line-height: 100px;
}
</style>
</head>
<body>
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
<div>5</div>
<div>6</div>
<div>7</div>
<script>
const divs = document.querySelectorAll('div')
let lastPick
divs.forEach(item => {
item.onclick = function () {
if (lastPick) {
lastPick.style.backgroundColor = 'red'
}
item.style.backgroundColor = 'yellow'
lastPick = item
}
});
</script>
</body>
</html>