WEBGIS开发
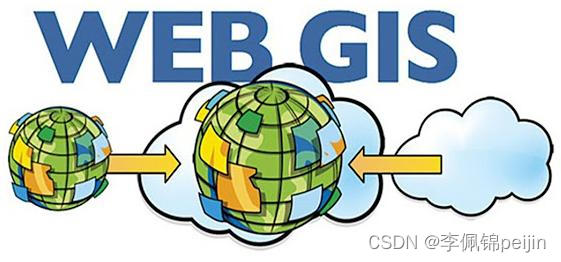
01、input时间
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input type="text" name="" id="">
<script>
const input = document.querySelector('input')
// 获取焦点
input.onfocus = function(){
input.style.borderBlockColor = 'blue'
}
// 失去焦点
input.onblur = function(){
input.style.borderBlockColor = 'red'
}
</script>
</body>
</html>
02、鼠标移入移出效果
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#app{
width: 100px;
height: 100px;
background-color: blueviolet;
}
</style>
</head>
<body>
<div id="app">app</div>
<script>
const app = document.querySelector('#app')
app.onmouseover = function(){
app.style.backgroundColor = 'red'
}
app.onmouseout =function(){
app.style.backgroundColor = 'blue'
}
</script>
</body>
</html>
03、键盘事件key
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<input type="text" name="" id="">
<button onclick="getValue()">确定</button>
</div>
<script>
const input = document.querySelector('input')
const app = document.querySelector('#app')
input.onkeydown = function (event) {
if (event.keyCode == 13) {
if (!input.value) {
alert('请填写信息')
} else {
const p = document.createElement('p')
p.innerHTML = '<span>'+ input.value+'</span><button onclick="toDel(this)">删除</button>'
app.append(p)
input.value = ''
}
}
}
function toDel(btn){
// 获取button的父元素
const parentElement = btn.parentElement
// 删除父元素
parentElement.remove()
}
</script>
</body>
</html>
04、间隔行效果
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
<div>5</div>
<div>6</div>
<div>7</div>
<div>8</div>
<script>
const divs = document.querySelectorAll('div')
divs.forEach((item,index) => {
item.style.background = index%2 ? 'blue':'red'
});
</script>
</body>
</html>
05、window对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// window是内置对象 不需要创建
console.log(window)
// docment也是属于window的一部分
console.log(document = window.document)
// alert也是window的一部分
window.alert('111')
// window可以省略
</script>
</body>
</html>
06、window相关事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div style="height: 2000px;"></div>
<script>
// 网页窗口大小改变触发
window.onresize = function(){
console.log(1111)
}
// 页面滚动时出发
window.onscroll = function(){
console.log('滚动')
}
</script>
</body>
</html>
07、location
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button onclick="btnReload()">刷新</button>
<script>
console.log(location)
consolo.log(window.location == location)
function btnReload(){
// 页面刷新
// location.reload()
// 页面跳转
location.href = 'hppts://www.baidu.com'
}
</script>
</body>
</html>
08、定时器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div></div>
<script>
// 定时器也是属于window属性
// 间隔一定时间触发 并且只触发一次
// setTimeout(()=>{
// console.log('hello')
// },1000) // 第二个参数为时间
const div = document.querySelector('div')
// 间隔一段时间重复触发
setInterval(() => {
let d = new Date()
div.innerHTML = d
}, 1000);
</script>
</body>
</html>
09、清除定时器
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<button onclick="toClear()">删除</button>
<script>
// 用一个变量接收定时器返回的值
const time1 = setTimeout(() => {
console.log(1);
}, 1000);
const timer2 = setInterval(() => {
console.log(2)
}, 1000);
function toClear() {
// 清除定时器
clearTimeout(time1);
clearInterval(timer2)
}
</script>
</body>
</html>
10、倒计时跳转
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>请在5秒内点击此处,否则自动跳转</h1>
<h2>5</h2>
<script>
// const time = setInterval(() => {
// const h1 = document.querySelector('h1')
// let str = h1.textContent
// let time = str[2]
// time -= 1
// if (time > 0) {
// h1.textContent = str.substr(0, 2) + time + str.substr(3)
// } else {
// clearInterval(timer)
// location.href = "https://www.baidu.com"
// }
// }, 1000);
const h2 = document.querySelector('h2')
let timer
function go(){
timer = setTimeout(() => {
if(h2.innerHTML>0){
h2.innerHTML--
go()
}else{
console.log(1)
clearTimeout(timer)
}
}, 1000);
}
go()
</script>
</body>
</html>
11、短信验证码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 25vw;
margin: 0 auto;
}
</style>
</head>
<body>
<div>
<input type="text" name="" id="">
<button>发送验证短信</button>
</div>
<script>
const btn = document.querySelector('button')
// btn.onclick = function () {
// btn.disabled = true
// let time = 5
// const timer = setInterval(() => {
// console.log(time)
// if (time > 0) {
// time--
// } else {
// clearInterval(timer)
// btn.disabled = false
// }
// }, 1000);
// }
let num = 5
btn.onclick = function () {
let num = 5
btn.disabled = true
btn.innerHTML = 5
function go() {
timer = setTimeout(() => {
if (num > 0) {
num--
btn.innerHTML = num
go()
} else {
clearTimeout(timer)
btn.disabled = false
}
}, 1000);
}
}
</script>
</body>
</html>
12、轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
img {
width: 80vw;
height: 60vh;
margin: 0 auto;
}
</style>
</head>
<body>
<img src="./images/1.jpg" alt="">
<script>
let arr = ["./images/1.jpg", "./images/2.jpg", "./images/3.jpg"]
const img = document.querySelector("img")
let num = 0
setInterval(() => {
num++
if(num>arr.length-1){
num=0
}
img.src = arr[num]
}, 2000);
</script>
</body>
</html>
13、jquery入门
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery.min.js"></script>
</head>
<body>
<div>你好</div>
<script>
// const div = document.querySelector('div') //之间
// $是jquery别名 用起来方便 书写更简洁
// console.log($('div'))
$('div').hide() // 选中的是div元素 传入的还是css选择器
// hide是jquery隐藏的元素的方法
</script>
</body>
</html>
14、jquery事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery.min.js"></script>
</head>
<body>
<button>按钮</button>
<script>
$('button').click(() => {
console.log('我被点击了')
})
</script>
</body>
</html>
15、jquery遍历
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery.min.js"></script>
</head>
<body>
<div>2</div>
<div>2</div>
<div>2</div>
<div>2</div>
<div>2</div>
<div>2</div>
<div>2</div>
<div>2</div>
<script>
const arr = $('div')
console.log(arr)
for (let index = 0; index < arr.length; index++) {
console.log(arr[index].innerHTML)
}
arr.each((index,item)=>{
console.log(index,item)
})
</script>
</body>
</html>
16、jquery操作
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery.min.js"></script>
</head>
<body>
<div id="d1"><span>11111111111111</span></div>
<script>
const d = $('#d1')
console.log(d)
// 获取div标签中的内容
console.log(d.html())
d.html('333333333333')
// 原生js操作html元素 本质操作元素的属性
// jquery操作html 是调用封装好的方法
</script>
</body>
</html>
17、jquery操作属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="./jquery.min.js"></script>
</head>
<body>
<img src="../day2/img/on.gif" alt="">
<script>
$('img').click(() => {
// attr 第一个参数告诉jquery修改那个属性 第二个参数为修改的值
$('img').attr('src').includes('on')?
$('img').attr('src', '../day2/img/off.gif'):$('img').attr('src', '../day2/img/on.gif')
// if ($('img').attr('src').includes('on')) {
// $('img').attr('src', '../day2/img/off.gif')
// } else {
// $('img').attr('src', '../day2/img/on.gif')
// }
})
</script>
</body>
</html>
18、jquery操作样式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="jquery.min.js"></script>
<style>
div{
width: 100px;
height: 100px;
background-color: rebeccapurple;
}
</style>
</head>
<body>
<div></div>
<script>
$('div').click(()=>{
// 第一个参数css属性,第二个参数要更改的属性值
$('div').css('background-color','blue')
})
</script>
</body>
</html>
19、jquery操作节点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="jquery.min.js"></script>
</head>
<body>
<script>
const hr = $('<hr>') //只是创建了 数据在内存中
const body = $('body') //获取父元素对象
body.append(hr) //将生成的hr添加到body
body.append('<div>;aljf;ljas;klf<div/>') //通过字符串添加标签
hr.remove() //元素的删除
</script>
</body>
</html>
20、数据请求
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="jquery.min.js"></script>
</head>
<body>
<script>
// $.ajax({
// type:'GET',
// url:'./data.json',
// success:function(data){
// console.log(data)
// data.user.forEach((item) => {
// console.log(item)
// // ``模板字符串 可以换行
// $('body').append(
// `<div>
// 姓名:${item.name}
// 年龄:${item.age}
// id:${item.id}
// </div>`)
// });
// }
// })
fetch('./data.json').then(res => res.json()).then(data => {
data.user.forEach((item) => {
console.log(item)
// ``模板字符串 可以换行
$('body').append(
`<div>
姓名:${item.name}
年龄:${item.age}
id:${item.id}
</div>`)
})
})
</script>
</body>
</html>