一.在servlet程序上通过浏览器访问servlet程序的基础
1.新建项目
2、将项目添加为web项目
3. 选择web
创建好之后使用IDEA编写Servlet程序
1.在创建的web项目中找到 web 文件夹的 WEB-INF 中创建目录起名字叫 lib ,然后放入需要的jar包
2.
在
src
的包中创建一个
Java
类,让该类实现
Servlet
接口,实现它的方法,那么该类就是一个
Servlet
类。
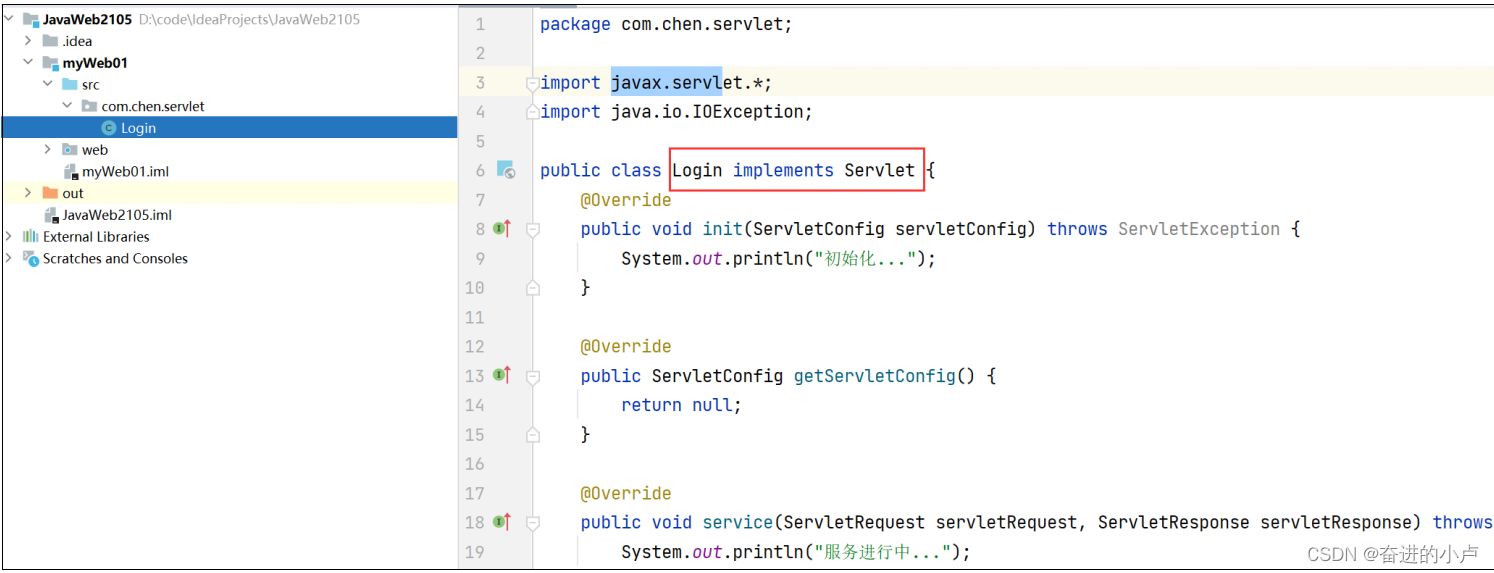
3.
在
web.xml
中配置
servlet
的映射关系。
告诉系统当浏览器请求
login
这个地址的时候,要执行
login
这个
servlet
,
login
这个
servlet
就是
com.chen.servlet
包里面的
Login
这个类
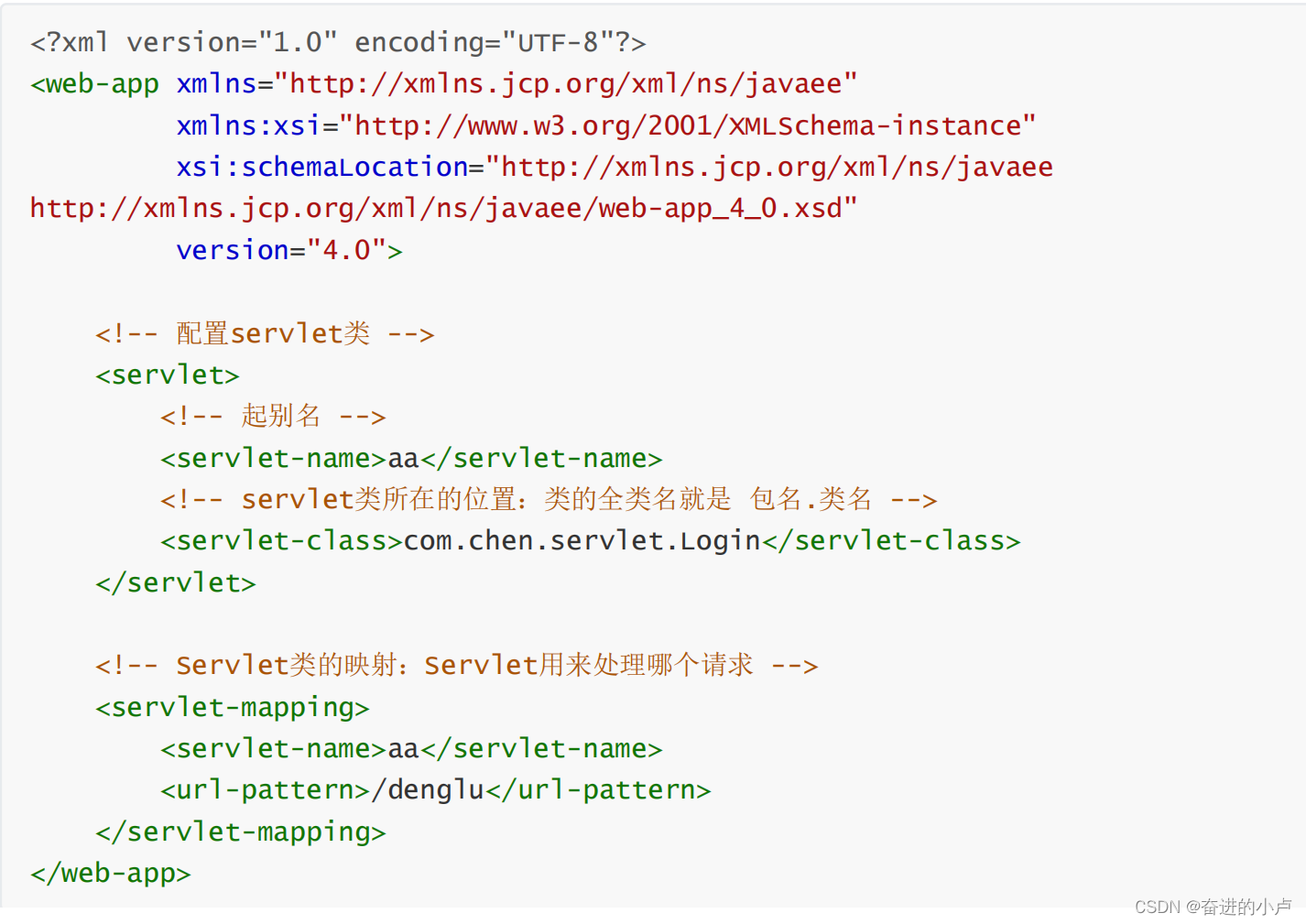
4.在浏览器上输入login的请求,测试servlet程序是否执行
二.实现使用HttpServlet完成一个假登录;
在上面的基础上,将方法
改为extends HttpServlet
如图:
1. 原本的类为登录,再创建一个注册类
2.在web.xml里配置两个servlet类
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!-- 配置servlet类 -->
<servlet>
<!-- 起别名 -->
<servlet-name>login</servlet-name>
<!-- servlet类所在的位置:类的全类名就是 包名.类名 -->
<servlet-class>com.lu.servlet.Login</servlet-class>
</servlet>
<!-- Servlet类的映射:Servlet用来处理哪个请求 -->
<servlet-mapping>
<servlet-name>login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
<!-- 配置servlet类 -->
<servlet>
<!-- 起别名 -->
<servlet-name>zhuce</servlet-name>
<!-- servlet类所在的位置:类的全类名就是 包名.类名 -->
<servlet-class>com.lu.servlet.Zhuce</servlet-class>
</servlet>
<!-- Servlet类的映射:Servlet用来处理哪个请求 -->
<servlet-mapping>
<servlet-name>zhuce</servlet-name>
<url-pattern>/zhuce</url-pattern>
</servlet-mapping>
</web-app>
3.在index.jsp中写自己想要的html页面效果
%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>第一个Web项目</title>
</head>
<body>
<h2>欢迎来到Java Web世界!</h2>
<a href="login.jsp">登录</a>
<a href="zhuce.jsp">注册</a>
</body>
</html>
4.分别 写好跳转之后的登录与注册页面(创建两个jsp,必须与index.jsp是同一级)
登录:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>登录</title>
</head>
<body>
<h2>注册</h2>
<form action="login" method="post">
账号:<input type="text" name="user" value=""/><br/>
密码:<input type="password" name="pwd" value=""/><br/>
<input type="submit" value="登录">
</form>
</body>
</html>
注册:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>注册</title>
</head>
<body>
<h2>注册</h2>
<form action="zhuce" method="post">
账号:<input type="text" name="user" value=""/><br/>
密码:<input type="password" name="pwd" value=""/><br/>
<input type="submit" value="注册">
</form>
</body>
5.完成在Login中的功能(前端请求(request),后端处理后,最后给前端做响应(respon))
实现使用HttpServlet完成一个假登录
package com.lu.servlet;
import javax.servlet.*;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class Login extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("Login-get...");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("Login-post...");
//前端请求(request),后端处理后,最后给前端做响应(respon)
//这三个都是获取请求的地址相关
String url=request.getRequestURI();
String contextPath=request.getContextPath();
String servletPath= request.getServletPath();
System.out.println(url);//包含项目名和资源路径
System.out.println(contextPath);//项目名称
System.out.println(servletPath);//请求的资源路径
System.out.println("================");
//1.从请求中获取用户提取的参数(数据)
request.setCharacterEncoding("utf-8");//设置请求的编码格式为中文
String user=request.getParameter("user");
String pwd=request.getParameter("pwd");
System.out.println(user);
System.out.println(pwd);
//3.判断成功要干什么?判断失败要干什么?---作响应
response.setCharacterEncoding("utf-8");
response.setContentType("text/html;charset=UTF-8");
if (user.equals("张三")&& pwd.equals("666")){
//登录成功
response.getWriter().write("登录成功!");
}else {
//登录失败
response.getWriter().write("登录失败");
}
}
}
6.与Login同理,写功能和链接
package com.lu.servlet;
import javax.servlet.*;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
public class Zhuce extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("zhuce-get...");
}
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("zhuce-post");
//前端请求(request),后端处理后,最后给前端做响应(respon)
//这三个都是获取请求的地址相关
String url=request.getRequestURI();
String contextPath=request.getContextPath();
String servletPath= request.getServletPath();
System.out.println(url);//包含项目名和资源路径
System.out.println(contextPath);//项目名称
System.out.println(servletPath);//请求的资源路径
}
}
共通:
最后的效果:
1.
2.会进行账户密码判断
正确:登录成功 不正确:登录失败
并且路径你能查看到