目录
- Introduction
- I.PSP Table
- II.Problem-solving ideas
- IV. Code Description
- Here is the code that completes the functionality, regarding the division by 0 and remainder by 0 operations:
- Here is the code to redesign the WeChat applet interface(Write it in a global json file):
- Here is the code for the data storage section(The history and result arrays are used to store the history and results of calculations.):
- Here is a demonstration of the operating system storing data:
- The following code reads data from the database:
- V. Video Demonstration
- VI.Summary and Reflection
Introduction
In this blog post, I will detail the implementation process of the front-end and back-end interactive WeChat applet calculator and show the final product.
This calculator I created can implement addition, subtraction, multiplication, division, backspace and ans operations (get history). Before implementing the back-end database, I found a problem with my calculator, that is, I didn’t take into account the special case of 0, which can’t divide by 0 and balance to 0. I fixed this problem with this update.
The Link of Class | https://bbs.csdn.net/forums/ssynkqtd-04 |
---|---|
The Link of Requirement of This Assignment | Second assignment-- Back-end separation calculator programming-CSDN社区 |
The Aim of This Assignment | Improve the function of calculator and realize front-end interaction |
MU STU ID and FZU STU ID | 21124841_832101219 |
Link here to the project code:https://github.com/cxlyk/Bruce.git
I.PSP Table
Personal Software Process Stages | Estimated Time(minutes) | Actual Time(minutes) |
---|---|---|
Planning | 40 | 50 |
• Estimate | 40 | 50 |
Development | 365 | 500 |
• Analysis | 25 | 30 |
• Design Spec | 20 | 40 |
• Design Review | 10 | 20 |
• Coding Standard | 10 | 10 |
• Design | 60 | 80 |
• Coding | 140 | 140 |
• Code Review | 60 | 75 |
• Test | 40 | 50 |
Reporting | 120 | 150 |
• Test Repor | 60 | 80 |
• Size Measurement | 10 | 10 |
• Postmortem & Process Improvement Plan | 30 | 60 |
Sum | 525 | 700 |
II.Problem-solving ideas
Front-end design concepts: along the lines of WeChat applets:
-
wxml structure: Create a wxml file to define the applet page. Add input boxes, buttons and other elements to allow users to enter mathematical expressions and perform calculations.
-
wxss Styles: Use wxss to beautify the page and make it look more beautiful and easy to use. You can add styles like background, font, colour and layout. I used gradient colour to make the background look better.
-
Js Interaction: Use the .js file of WeChat app to write the front-end logic and implement the function:
- Listen to button click events to capture user input.
- Handle expression evaluation when user clicks on the equal sign
button. - Display the result of the calculation on the web page.
4.User Experience: Ensure the interface is user friendly and easy to use. Add error handling features, (which was not available last time) such as divide by zero and remainder to zero errors to enhance logic and improve user experience.
Backend Design Ideas:
I wasted a lot of time before finding the right approach. My first thought was to rent a server piggybacking on the database for connectivity, and then I found out that Huawei Cloud has a free cloud database rental (one month). After applying for it, I realised that I knew nothing about the database and API content, and had no idea where to start. Then I found out that there are private databases that I can rent, which are much easier to use compared to Huawei Cloud, and although the memory is small, it’s perfectly adequate.
1.Backend: Use WeChat apps to write the backend code, using post to ensure data security, so as to interact with the database and feed back to the front-end later.
2.API design: design two apis, respectively for storage and reading.
3.Database Connection: Use MySQL to connect to the database and perform the necessary database operations to store the calculation history.
4.Results Return: Send the stored expressions and their corresponding computations back to the front-end, usually in JSON format. Make sure to handle any potential errors or exceptions and provide friendly error messages.
III.Design and Implementation process
After a week of studying and looking up information, I found a relatively workable way to combine a WeChat applet with a backend database. However, since I had studied microcontrollers before and had not been exposed to front-end and back-end stuff, I was a bit overwhelmed by using it. But fortunately, with my efforts, I was able to achieve the function of storing and reading calculator data.
Firstly I found a website developed by an individual that offers a rental service for MySql databases and corresponding APIs. By using their database, I don’t need to build my own database on a server, which is the best way I could find.
Links to the sites I use:https://zbmysql.huaxio.cn/index.php
Then there is the design of the WeChat applet part, for the new update of the division by 0 and the remainder of 0 part will be shown in the code section.
I used tabbar operation to divide the applet page into two parts. On the left is the calculator page, switch to the right is the history query. This is the overall design. Regarding the data storage and reading part, I use the wx:require method, and use the post method to input the password of the database to ensure the security of data transmission. The specific code and explanation will be put in the code section.Flowchart of front - and back-end interactions
IV. Code Description
Here is the code that completes the functionality, regarding the division by 0 and remainder by 0 operations:
case '÷':
if (right == 0) { //如果数字不合规-除以0
wx.showToast({
title: "Can't divided by 0 !",
icon: 'none'
})
ans = left
} else {
let _ans = acc.div(left, right)
let x = String(_ans).indexOf('.') + 1;
let y = String(_ans).length - x;
if (y > 10) {
ans = _ans.toFixed(10);
} else {
ans = _ans
}
}
break;
case '%':
if (right == 0) { //如果数字不合规-余0
wx.showToast({
title: "Can't take a remainder of 0 !",
icon: 'none'
})
ans = left
} else {
ans = left % right
}
break;
default:
ans = left
break;
Here is the code to redesign the WeChat applet interface(Write it in a global json file):
"tabBar": {
"list": [{
"pagePath": "pages/index/index",
"text": "calculator",
"iconPath": "/pages/history/images/图标.jpg",
"selectedIconPath": "/pages/history/images/图标.jpg"
},{
"pagePath": "pages/history/history",
"text": "ans_history",
"iconPath": "/pages/history/images/3_static.png",
"selectedIconPath": "/pages/history/images/3_active.png"
}
]
},
Here is the code for the data storage section(The history and result arrays are used to store the history and results of calculations.):
numBtn(e) {
// other logic
if (!isNaN(num) || num === '.') { //.
this.addToHistory(expression.join(' '));
}
addToHistory(expression) {
let historyItem = {
expression: expression,
};
this.data.history.push(historyItem);
this.setData({
history: this.data.history
});
}
history arrays:
The history array is used to store the history of the user’s calculations. Each time the user completes a calculation, the addToHistory function is called to add the current expression to the history array.
The addToHistory function takes an expression argument, wraps it into an object, and adds the object to the history array.
this.data.history.push(historyItem) adds the new calculated history item to the history array.
this.setData({ history: this.data.history }) Updates the applet’s data, ensuring that the calculation history on the page is updated.
finish(e) {
// ...(计算逻辑)
// 将计算结果加入 result 数组
this.data.result.push(expression[0]);
// 更新数据
this.setData({
'counter.expression': expression,
'counter.ansType': ansType,
equalButtonCount: this.data.equalButtonCount + 1,
result: this.data.result
});
// 发送请求将历史记录存入数据库(在这之前确保 this.data.history 和 this.data.result 已经准备好了)
wx.request({
url: "https://zbmysql.huaxio.cn/api/15260107375uMTIC.php?",
data: {
sql: 'INSERT INTO new_calcultor VALUES( null, "' + this.data.history[this.data.equalButtonCount].expression + '","=","' + this.data.result[this.data.equalButtonCount] + '");',
api_pawsord: "wdmpOtiaZhFkKv6"
},
method: 'post',
header: {
"Content-Type": "application/x-www-form-urlencoded"
},
dataType: 'json',
responseType: 'text',
success: (res) => {
console.log(res.data);
if (res.data.affected = 1) {
console.log('操作成功!');
}
},
fail: () => {},
complete: () => {},
});
}
The result array:
The result array is used to store the results of each calculation. In the finish function, when you finish a calculation, you add the result to the result array and save it in setData.
In the finish function, when the calculation is complete, the current result of the calculation, expression[0], is added to the result array.
The result array is updated in setData.
When the request is sent, get the current number of button clicks from this.data.equalButtonCount, and get the corresponding expression and result from the history and result arrays.
Here is a demonstration of the operating system storing data:
storing data
The following code reads data from the database:
.wxml:
<button bindtap="sec_bt">Click for historical data</button>
<view class="view1">
<view>{{data1a}}</view> <!-- 用于显示从数据库获取的数据 -->
<view>{{data1b}}</view>
<view>{{data1c}}</view>
</view>
<view class="view1">
<view>{{data2a}}</view>
<view>{{data2b}}</view>
<view>{{data2c}}</view>
</view>
.js:
sec_bt() {
wx.request({
url: "https://zbmysql.huaxio.cn/api/15260107375uMTIC.php?", // 请求的API地址
data: {
sql: 'select * from new_calcultor where id="1";', // SQL语句
api_pawsord: "wdmpOtiaZhFkKv6" // API密码
},
method: 'post',
header: {
"Content-Type": "application/x-www-form-urlencoded"
},
dataType: 'json',
responseType: 'text',
success: (res) => {
console.log(res.data) // 得到返回的数据
this.setData({
data1a: res.data.result[0].expression,
data1b: res.data.result[0].equal,
data1c: res.data.result[0].result,
});
},
fail: () => {},
complete: () => {}
});
wx.request({
url: "https://zbmysql.huaxio.cn/api/15260107375uMTIC.php?", // 请求的API地址
data: {
sql: 'select * from new_calcultor where id="2";', // SQL语句
api_pawsord: "wdmpOtiaZhFkKv6" // API密码
},
method: 'post',
header: {
"Content-Type": "application/x-www-form-urlencoded"
},
dataType: 'json',
responseType: 'text',
success: (res) => {
console.log(res.data) // 得到返回的数据
this.setData({
data2a: res.data.result[0].expression,
data2b: res.data.result[0].equal,
data2c: res.data.result[0].result,
});
},
fail: () => {},
complete: () => {}
});
This is only part of it, because to display ten entries, another eight requests are needed, and only the id and data binding parts need to be changed. The data in the database consists of three parts, the first is the expression, the second is the equals sign and the last is the result.
this.setData({
data1a: res.data.result[0].expression,
data1b: res.data.result[0].equal,
data1c: res.data.result[0].result,
});
V. Video Demonstration
This video is a demonstration of the prohibition of division by 0 and remainder 0:
new function
This video is calculate ten results, pressing is equivalent to automatically storing the data in the database (new_caculator):
Calculator Calculator
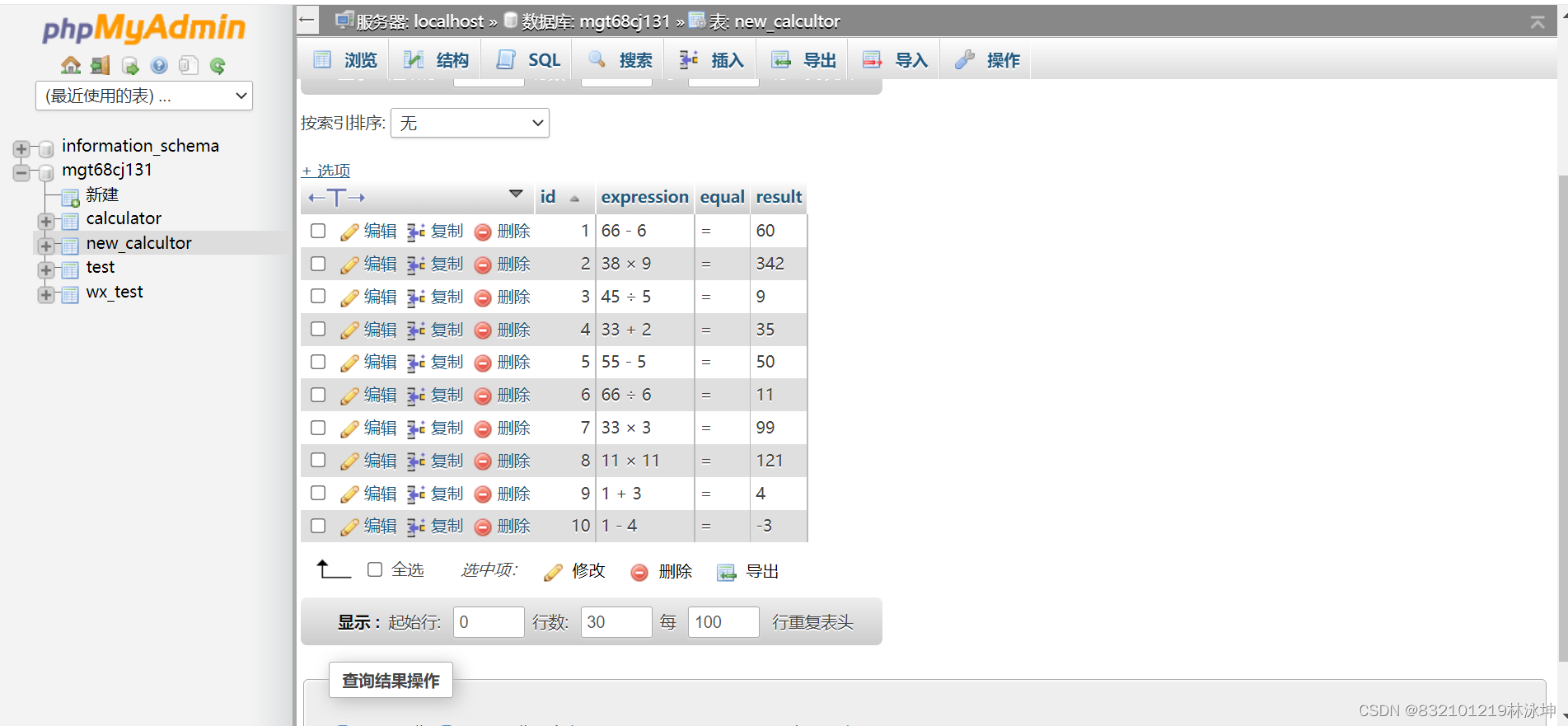
The database after calculation
This video is a demonstration of a history query:
history query
An online experiential version of the applet
VI.Summary and Reflection
This assignment has given me a good understanding of how WeChat applets are connected to the back-end database. However, I personally feel that there must be a better way to be able to achieve the functionality, and there are still a lot of inadequacies in my project. I will need to continue to study it when I have time afterwards