目录
P1217 [USACO1.5] 回文质数 Prime Palindromes
P4956 [COCI2017-2018#6] Davor编辑
P5718 【深基4.例2】找最小值
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int min=Integer.MAX_VALUE;
for(int i=0;i<n;i++){
min=Math.min(min,sc.nextInt());
}
System.out.println(min);
sc.close();
}
}
P5719 【深基4.例3】分类平均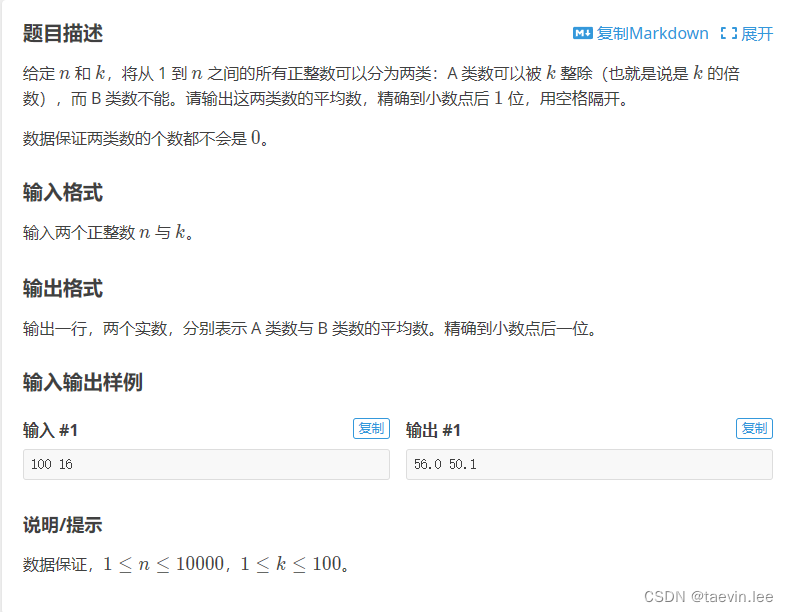
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int k=sc.nextInt();
double sumA=0;
double sumB=0;
for(int i=k;i<=n;i+=k) {
sumA+=i;
}
sumB=n*(1+n)/2-sumA;
System.out.printf("%.1f %.1f",sumA/(n/k),sumB/(n-n/k));
}
}
P5720 【深基4.例4】一尺之棰
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int day=1;
while(n>1) {
n/=2;
day++;
}
System.out.println(day);
}
}
P5721 【深基4.例6】数字直角三角形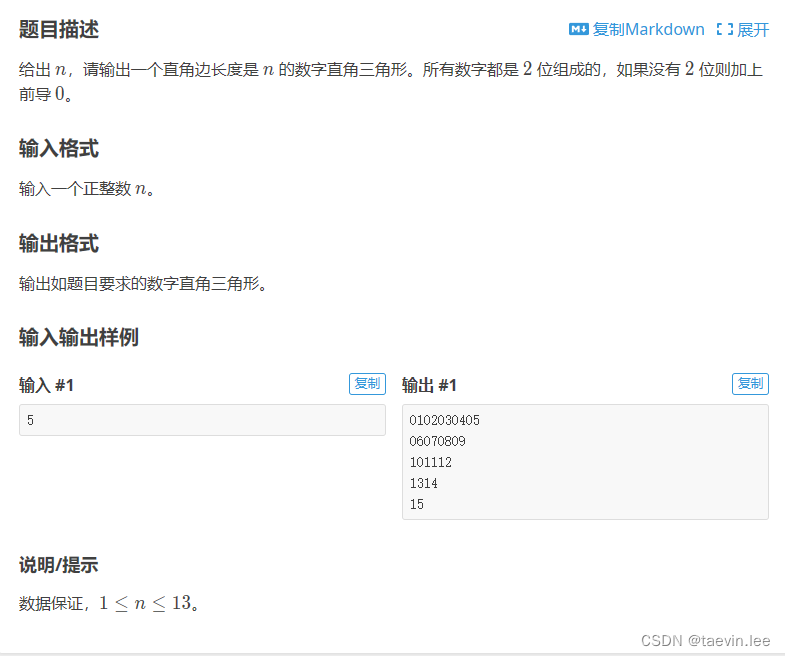
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int count=1;
for (int i = 0; i < n; i++) {
for (int j = n-i; j>0; j--) {
System.out.printf("%02d", count++);
}
System.out.println();
}
sc.close();
}
}
P1009 [NOIP1998 普及组] 阶乘之和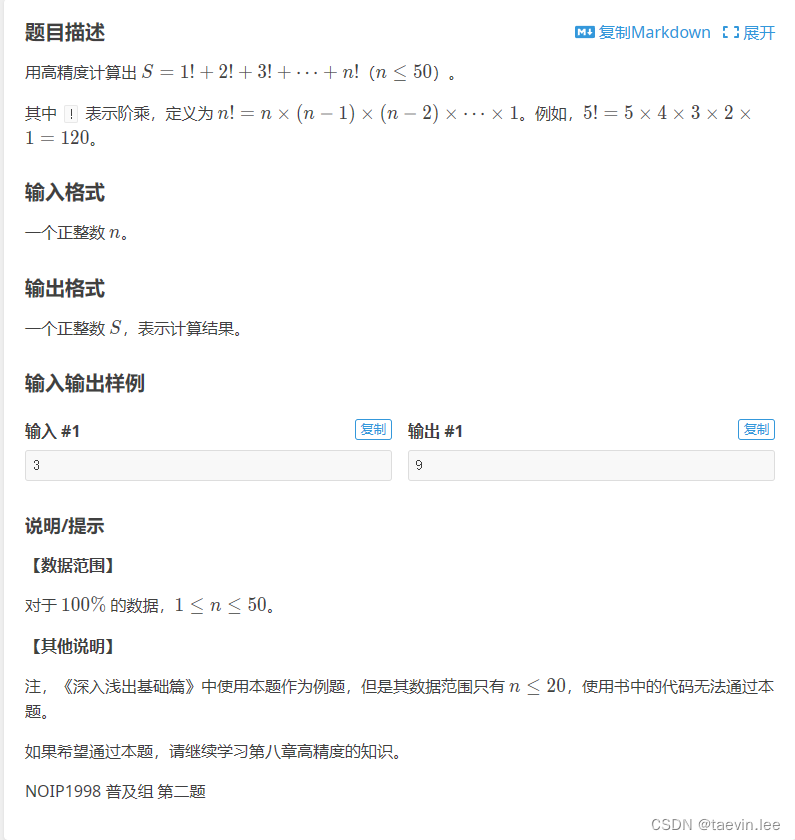
import java.math.BigInteger;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n = scanner.nextInt();
BigInteger sum = BigInteger.ZERO;
for (int i = 1; i <= n; i++) {
BigInteger factorial = BigInteger.ONE;
for (int j = 1; j <= i; j++) {
factorial = factorial.multiply(BigInteger.valueOf(j));
}
sum = sum.add(factorial);
}
System.out.println(sum);
}
}
P1980 [NOIP2013 普及组] 计数问题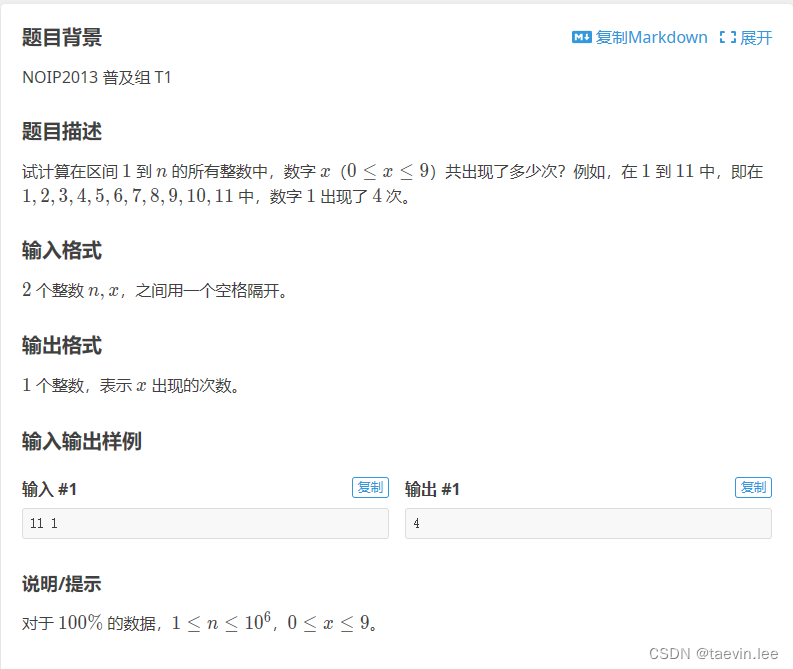
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int x=sc.nextInt();
int count=0;
for(int i=1;i<=n;i++) {
String[] str=(i+"").split("");
for(int j=0;j<str.length;j++) {
if(str[j].equals(String.valueOf(x))) {
count++;
}
}
}
System.out.println(count);
}
}
P1035 [NOIP2002 普及组] 级数求和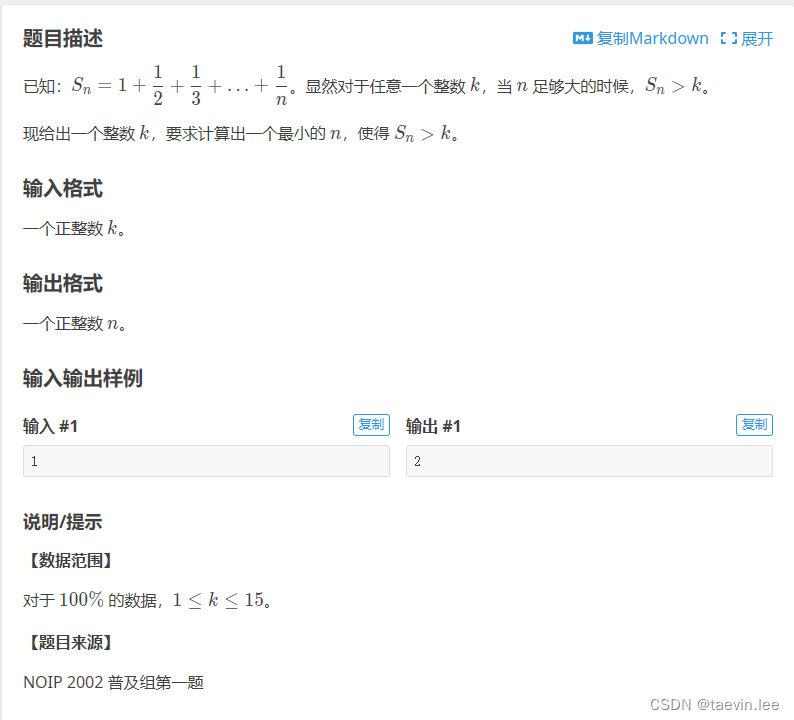
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int k=sc.nextInt();
double Sn=0;
int i=1;
while(true) {
Sn=Sn+(double)1/i;
if(Sn>k) {
break;
}
i++;
}
System.out.println(i);
}
}
P2669 [NOIP2015 普及组] 金币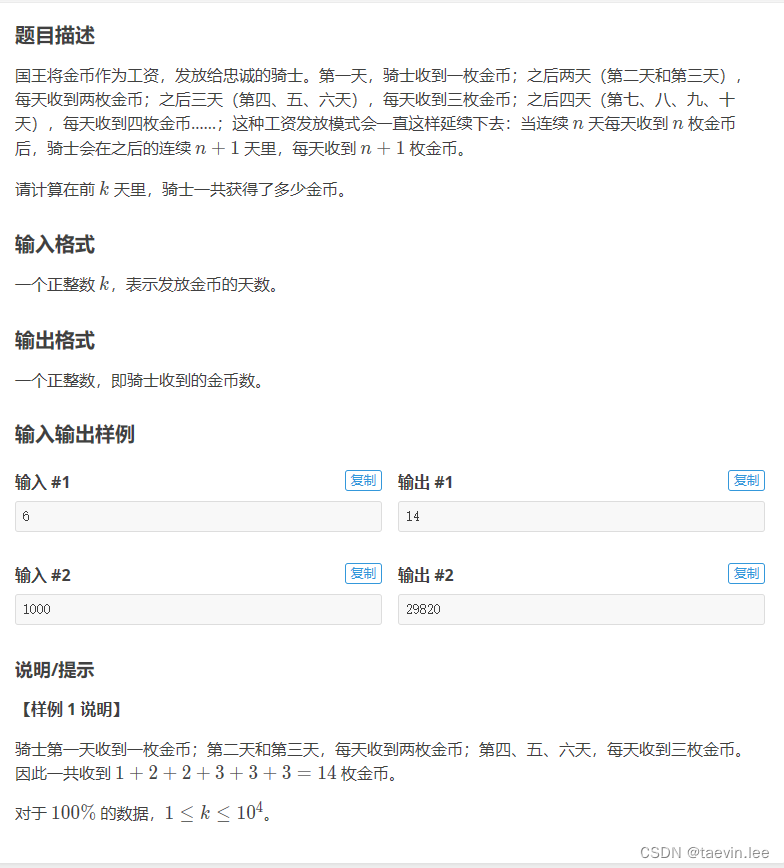
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int k=sc.nextInt();
int sum=0;
int day=1,j=1;
for(int i=1;i<=k;i++) {
sum+=day;
if(j==day) {
day++;
j=0;
}
j++;
}
System.out.println(sum);
}
}
P5722 【深基4.例11】数列求和
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int sum=n*(n+1)/2;
System.out.println(sum);
}
}
P5723 【深基4.例13】质数口袋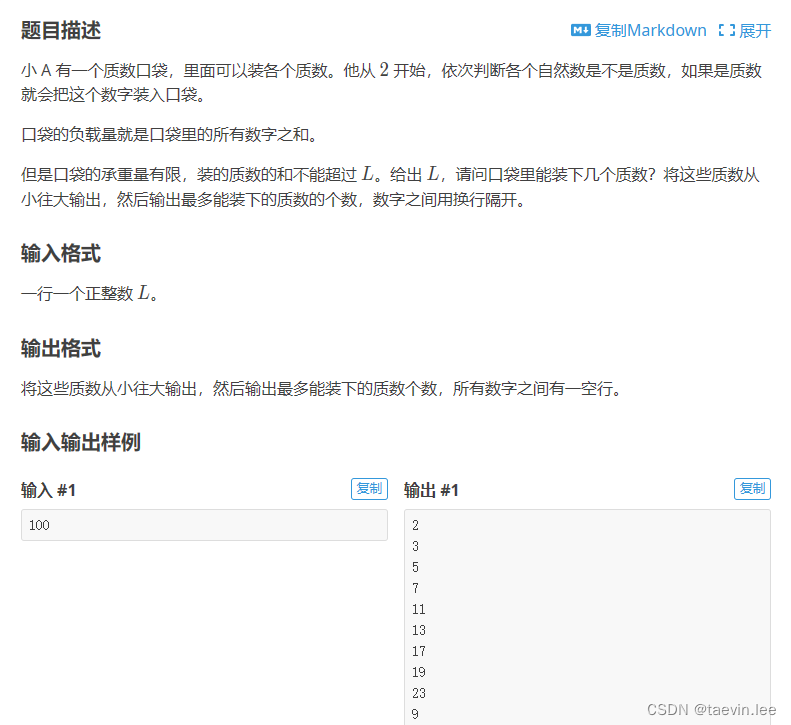
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int L=sc.nextInt();
int prime=2,sum=0;
int count=0;
while(true) {
if(isPrime(prime)){
if(sum+prime>L) {
break;
}
sum+=prime;
System.out.println(prime);
count++;
}
prime++;
}
System.out.println(count);
}
public static boolean isPrime(int n) {
if(n==2) {
return true;
}else {
for(int i=2;i<n;i++) {
if(n%i==0) {
return false;
}
}
return true;
}
}
}
P1217 [USACO1.5] 回文质数 Prime Palindromes
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a = sc.nextInt();
int b = sc.nextInt();
sc.close();
StringBuilder sb = new StringBuilder();
if (a % 2 == 0) {
a += 1;
}
for (int i = a; i <= b; i += 2) {
if (isPalindrome(i) == i && isPrime(i)) {
System.out.println(i);
}
}
}
public static int isPalindrome(int num) {
int t=num;
int ans=0;
while(t!=0) {
ans=ans*10+t%10;
t/=10;
}
return ans;
}
public static boolean isPrime(int num)
{
for (int i = 2; i <= Math.sqrt(num); i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
}
P1423 小玉在游泳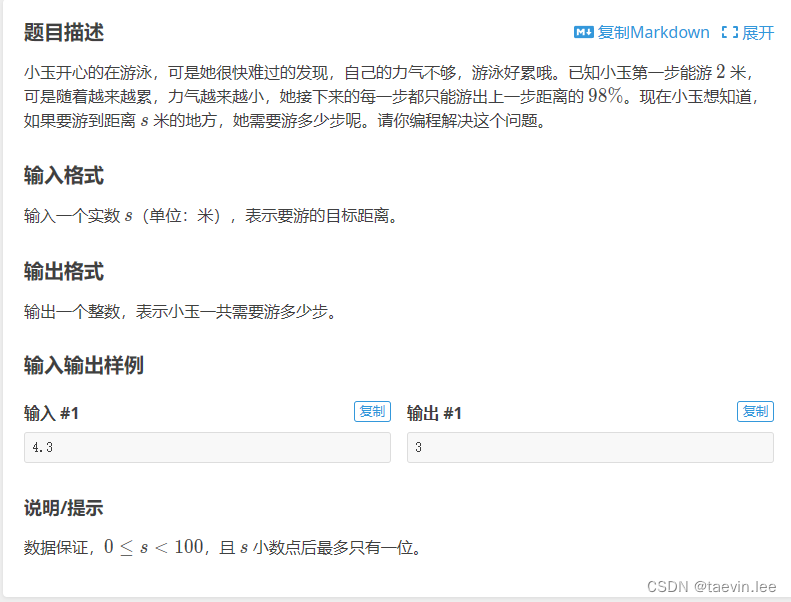
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double s=sc.nextDouble();
double sum=0,i=2;
int count=1;
while(true) {
sum+=i;
if(sum>s) {
break;
}
i*=0.98;
count++;
}
System.out.println(count);
}
}
P1307 [NOIP2011 普及组] 数字反转
import java.util.Scanner;
import javax.swing.plaf.multi.MultiInternalFrameUI;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String str=sc.nextLine().trim();
char first=str.charAt(0);
boolean isNegative=first=='-';
StringBuilder sb=new StringBuilder(str);
if(isNegative) {
sb.deleteCharAt(0);
sb.reverse();
sb.insert(0, '-');
}else {
sb.reverse();
}
int result=Integer.parseInt(sb.toString());
System.out.println(result);
}
}
P1720 月落乌啼算钱(斐波那契数列)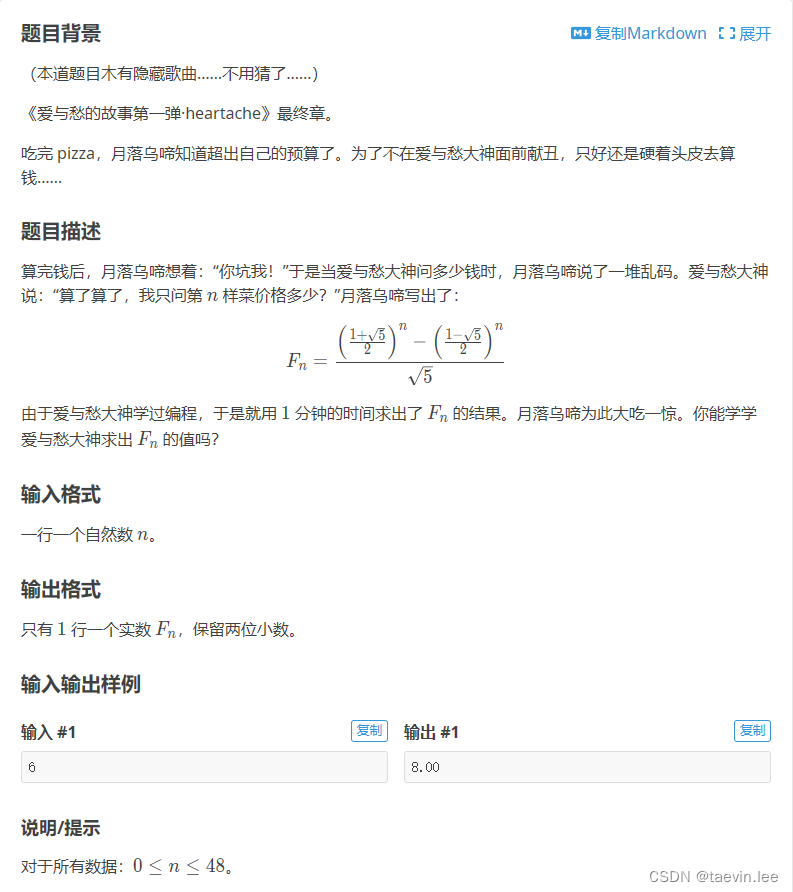
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
double a=(1+Math.sqrt(5))/2;
double b=(1-Math.sqrt(5))/2;
double result=(Math.pow(a,n)-Math.pow(b, n))/Math.sqrt(5);
System.out.printf("%.2f",result);
}
}
P5724 【深基4.习5】求极差 / 最大跨度值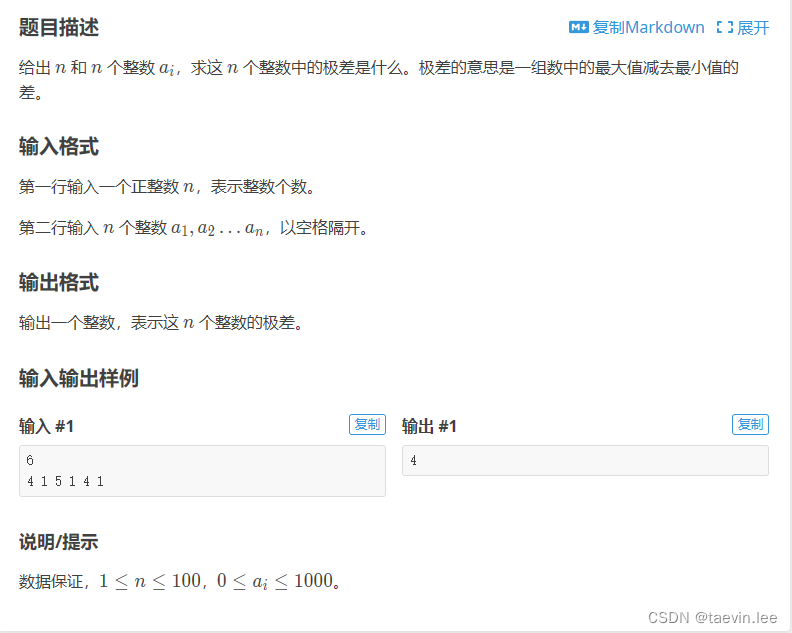
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
int[] arr=new int[n];
for(int i=0;i<n;i++) {
arr[i]=sc.nextInt();
}
int max=Integer.MIN_VALUE;
int min=Integer.MAX_VALUE;
for(int i=0;i<n;i++) {
if(arr[i]>max) {
max=arr[i];
}
if(arr[i]<min) {
min=arr[i];
}
}
System.out.println(max-min);
}
}
P1420 最长连号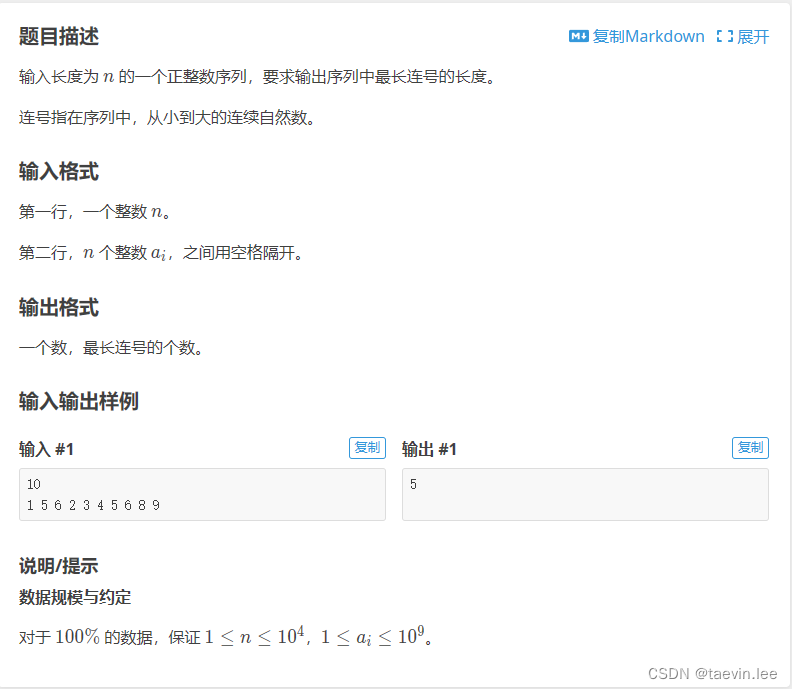
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
sc.nextLine();
String str=sc.nextLine();
String[] arr=str.split(" ");
int maxCount=0;
int count=0;
for(int i=1;i<n;i++) {
if(Integer.valueOf(arr[i])-Integer.valueOf(arr[i-1])==1) {
count++;
}else {
if(count>maxCount) {
maxCount=count;
}
count=0;
}
}
if(n>1&&count>0) {
if(count>maxCount) {
maxCount=count;
}
}
System.out.println(maxCount+1);
}
}
P1075 [NOIP2012 普及组] 质因数分解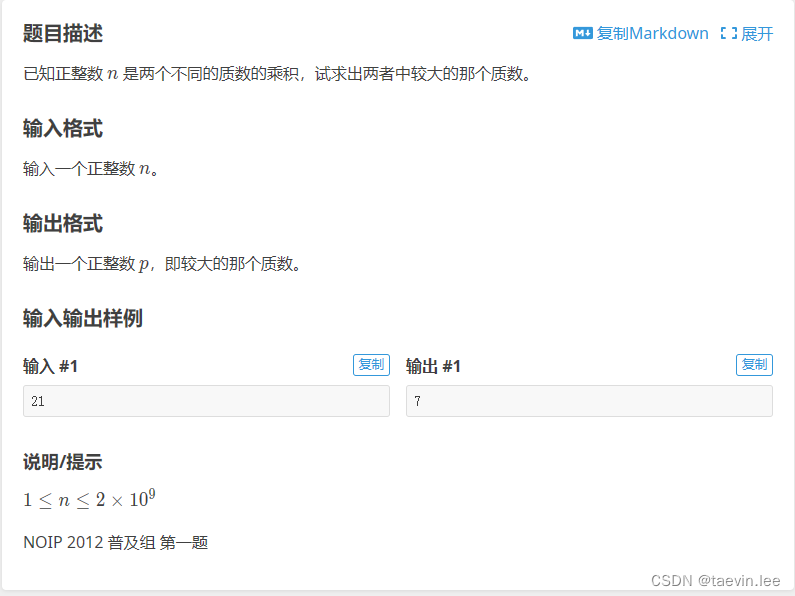
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner=new Scanner(System.in);
int n=scanner.nextInt();
int max=0;
for(int i=2;i<Math.sqrt(n);i++) {
if(n%i==0) {
max=i;
}
}
System.out.printf("%d",n/max);
}
}
P5725 【深基4.习8】求三角形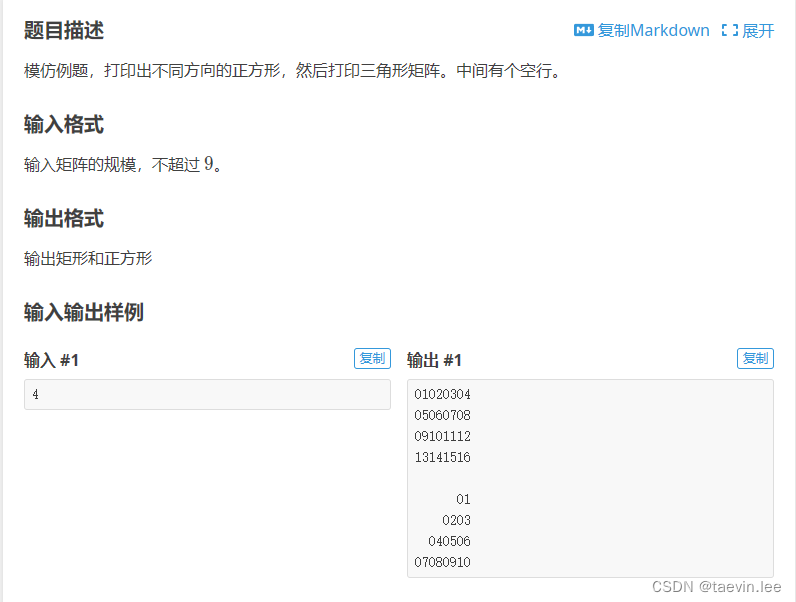
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int size = scanner.nextInt();
scanner.nextLine();
printRectangle(size);
System.out.println();
printTriangle(size);
}
private static void printRectangle(int size) {
int input=1;
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
System.out.printf("%02d",input);
input++;
}
System.out.println();
}
}
private static void printTriangle(int n) {
int input=1;
for (int i = 0; i < n; i++) {
for (int k = n - i - 1; k > 0; k--) {
System.out.print(" ");
}
for (int j = 0; j <=i; j++) {
System.out.printf("%02d",input);
input++;
}
System.out.println();
}
}
}
P5726 【深基4.习9】打分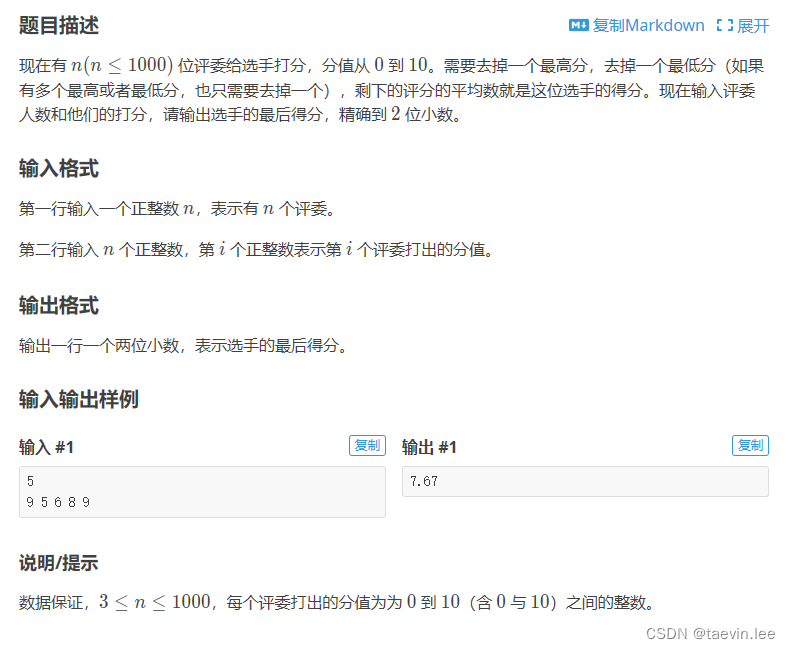
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int n=scanner.nextInt();
int[] scores=new int[n];
for(int i=0;i<n;i++) {
scores[i]=scanner.nextInt();
}
Arrays.sort(scores);
int sum=0;
for(int i=1;i<n-1;i++) {
sum+=scores[i];
}
System.out.printf("%.2f",(double)sum/(n-2));
}
}
P4956 [COCI2017-2018#6] Davor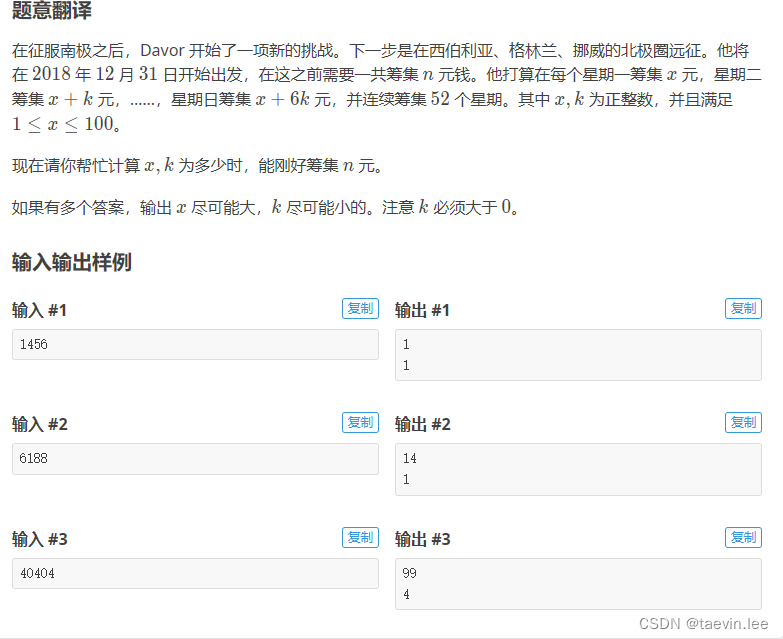
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for(int k=1;;k++) {
for(int x=100;x>=1;x--) {
if((7*x+21*k)*52==n) {
System.out.println(x);
System.out.println(k);
return;
}
}
}
}
}
P1089 [NOIP2004 提高组] 津津的储蓄计划
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int mom=0;
int me=0;
for(int i=1;i<=12;i++) {
int budget=sc.nextInt();
me+=300;
if(budget>me) {
System.out.println(-i);
return;
}else {
me-=budget;
if(me>=100) {
mom+=me/100*100;
me-=me/100*100;
}
}
}
System.out.println((int)(mom*1.2+me));
}
}