A. Basic Naming Conventions
1. Class names always begin with upper-case and continue with camel-case. Example: AecDbWall, AecUiDlgOptions, etc.
2. Member functions should begin with lower-case and continue with camel-case. Example: startPoint(), getOsanpPoints(), etc.
3. Member variables should begin with “m_” and continue with camel-case. Example: m_startPt, m_radius.
4. Local variables should begin with lower-case and continue with camel-case. Example: startPt, xDist, etc.
5. Global variables are discouraged. Try to use a static member var of a class instead. In the rare cases we do have a global variable, which usually exists only at file scope. In this case, make it follow class name conventions and clearly mark it as a global variable with comments.
6. #defines should be in all caps, with “_” to separate words. Example: AEC_ASSERT(), ACRX_DECLARE(), etc.
7. Enum values should begin with “k”, or in the special case of error codes, “e”. Example:
enum OffsetType {
kFromStartOfCurve =0,
kFromEndOfCurve,
kFromMidpointOfCurve
};
If the enum values are written to the DWG file through the filing mechanism, explicitly declare all enum values and leave a comment stating that they cannot be changed arbitrarily. Example:
// written to DWG (extend only, do not change value)
enum DoorType {
kDoorTypeCustom = 0,
kDoorTypeSingle = 1,
kDoorTypeDouble = 2,
kDoorTypeSingleDhung = 3,
kDoorTypeDoubleDhung = 4,
kDoorTypeDoubleOpposing = 5,
};
8. Hungarian notation is as follow. // I copied it from website and it may be not consistent with existing code of ADT, so please feel free to update it if you find some inconsistency.
Prefix
|
Type
|
Example
|
b
|
boolean
|
bool bStillGoing
|
c
|
character
|
char cLetterGrade;
|
str
|
C++ String
|
string strFirstName;
|
s
|
short integer
|
short sChairs;
|
i
|
integer
|
int iCars;
|
l
|
long integer
|
long lStars;
|
f
|
floating point
|
float fPercent;
|
d
|
double-precision floating point
|
double dMiles;
|
ld
|
long double-precision floating point
|
long double ldLightYears;
|
sz
|
Old-Style Null Terminated String
|
char szName[NAME_LEN];
|
The following table contains letters that go before the above prefixes.
Prefix
|
Type
|
Example
|
u
|
unsigned
|
unsigned short usiStudents;
|
s
|
static
|
static char scChoice;
|
rg
|
array (stands for range)
|
float rgfTemp[MAX_TEMP];
|
m_
|
member variable of a struct or class
|
char m_cLetterGrade;
|
p
|
pointers
|
int *piGrade;
|
For pointers, we can also use Ptr as postfix, for example, m_facePtr.
B. Class Design
1. Prefix Naming Scheme
There are a set of established naming prefixes to consider when creating new classes:
Prefix
|
Classes
|
Aec
|
The prefix for all classes that are part of ADT
|
AecDb
|
Database resident object
|
AecImp
|
Implementation object (owned by an AecDb object)
|
AecGe
|
Geometry related classes
|
AecUi
|
All User Interface classes
|
AecUiPr
|
Command line prompt classes
|
AecUiPs
|
PropertySheet
|
AecUiPp
|
PropertyPage
|
AecUiDlg
|
Dialogs
|
AecWsht
|
Work sheets
|
AecUiCmd
|
Command classes
|
2. Try to keep class header files in the simple public/protected/private order instead of having multiple sections of each protection level. Example:
class MyClass :
public BaseClass
{
public:
// Constructors/Destructor
MyClass();
virtual ~MyClass();
// Accessor functions
AcGePoint3d startPoint()
const;
Acad::ErrorStatus setStartPoint(
const AcGePoint3d&);
// Other public interface
protected:
// protected interface
private:
// private interface
};
3. Accessor functions should be in the following pattern:
AcGePoint3d startPoint()
const;
Acad::ErrorStatus setStartPoint(
const AcGePoint3d&);
Only use the “get” prefix if it is not a simple data member that is being returned from the underlying class, like when a property is calculated or retrieved from somewhere else and has a chance of failing. Example:
Acad::ErrorStatus getArea(
double& area);
C. Comments
1. Put in reason comments for difficult code that explains why you chose to implement something in a certain way. This will be very useful especially if you do significant research to determine how something should be implemented.
2. If you are leaving some portions of code for later then put comments in the format “// TBD VERSION_NAME YOURNAME: the comments”. This will help us to go back and find out what we have left unfinished. The following is one example.
case
VT_BSTR:
{
if
((_bstr_t)newVal == variesBSTR())
{
// TBD KIASMA CLAUDE: deal with varies properly
hr = S_OK;
}
break
;
}
3. Out-of-date comments should be removed, especially TBDs in previous versions.
4. Commented code will be retained only if it may be recovered later or be helpful for later development.
5. A comment should be a well formed sentence.
6. Be sure to add overview block for new classes, add header for new files, add george block for definitions of new functions. M:/Kiasma/Devtools/VC7Macros/ADTGeneral.vsmacros can be loaded and run the macros.
D. Performance
1. In performance sensitive functions avoid following
for (
int i = 0; i < m_aMyArray.length(); ++i ){
Instead do this
int nTotalItems = m_aMyArray.length();
for (
int i = 0; i < nTotalItems; ++i ){
2. In performance sensitive functions, if possible declare the member variables outside the functions.
for (
int i = 0; i < nTotalItems; ++i )
{
MyClass a = b;
// Other code
}
Instead do this
MyClass a;
for (
int i = 0; i < nTotalItems; ++i )
{
a = b;
// Other code
}
E. Miscellaneous
1. Editor Setting:
Tab size must be set to 4 spaces. This ensures that files are formatted with the same spacing when used by multiple developers. All other text editor settings are based on user preference because they don’t affect the appearance of the file for other users.
Tab size must be set to 4 spaces. This ensures that files are formatted with the same spacing when used by multiple developers. All other text editor settings are based on user preference because they don’t affect the appearance of the file for other users.
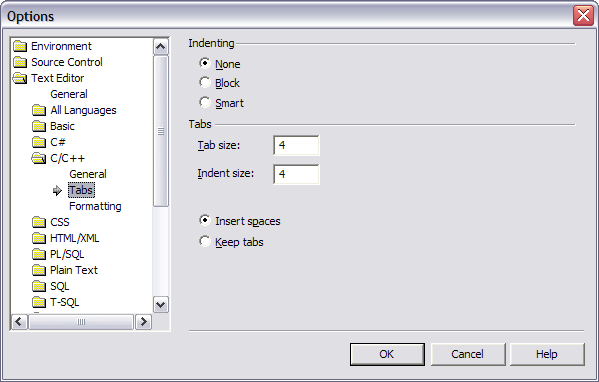
2. Use AcDbObjectPointer wherever possible to open an object that way you don’t have to worry about closing it.
3. Use AEC_ASSERT(!“Comment”) macro for putting asserts. Don’t use assert or ASSERT.
4. If needed use AEC_DELETE, which sets the pointer to NULL after deleting it.
5. Use one of the following coding styles for braces for better code readability. In one file, you should keep consistent with one of these styles
if ()
{
}
else
{
}
Or
if (){
}
else {
}
Following is not a good readable code.
if (){
}
else {
}
6. If you are allocating memory in a function where there are multiple return conditions and if this data needs to be freed up use std::auto_ptr. Following is one example
std::auto_ptr<AecGeRing> pRing( new AecGeRing );
7. Check pointer before use it.
8. If extensive calculations aren’t needed, AcArray template class is more voted than stl classes to be used for debugging.
9. Use Aec::fuzzyEqualXXX help functions for float variables’ compare.
10. If a string needs to be extracted and if you are not moving it to a .rc file please move it under macro _T() so that localization people (who translate the software) can catch it.
11. For strings like *NONE*, *VARIES*, etc use AcadString::none, AcadString::varies1.
12. AecRmCString str(_DNT("")); - No need for this, just write like this AecRmCString str.
F. References
1. UI Guidelines
If you are working with UI then refer this document will help you about the UI controls, layout guidelines etc.
//shasrvms3/documents/Dev/ BSD Desktop UI Design Elements.doc
If you are working with UI then refer this document will help you about the UI controls, layout guidelines etc.
//shasrvms3/documents/Dev/ BSD Desktop UI Design Elements.doc
2. Database side implementation
If you are adding any new members which gets filed out / in, please refer following document
M:/Kiasma/Docs/Internal/General/AEC Database Side Implementation Brain Dump.doc
If you are adding any new members which gets filed out / in, please refer following document
M:/Kiasma/Docs/Internal/General/AEC Database Side Implementation Brain Dump.doc