Time Limit: 8000MS | Memory Limit: 65536K | |
Total Submissions: 6908 | Accepted: 1998 | |
Case Time Limit: 2000MS | Special Judge |
Description
John is a Chief Executive Officer at a privately owned medium size company. The owner of the company has decided to make his son Scott a manager in the company. John fears that the owner will ultimately give CEO position to Scott if he does well on his new manager position, so he decided to make Scott’s life as hard as possible by carefully selecting the team he is going to manage in the company.
John knows which pairs of his people work poorly in the same team. John introduced a hardness factor of a team — it is a number of pairs of people from this team who work poorly in the same team divided by the total number of people in the team. The larger is the hardness factor, the harder is this team to manage. John wants to find a group of people in the company that are hardest to manage and make it Scott’s team. Please, help him.
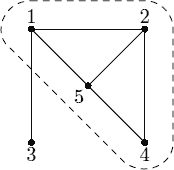
In the example on the picture the hardest team consists of people 1, 2, 4, and 5. Among 4 of them 5 pairs work poorly in the same team, thus hardness factor is equal to 5⁄4. If we add person number 3 to the team then hardness factor decreases to 6⁄5.
Input
The first line of the input file contains two integer numbers n and m (1 ≤ n ≤ 100, 0 ≤ m ≤ 1000). Here n is a total number of people in the company (people are numbered from 1 to n), and m is the number of pairs of people who work poorly in the same team. Next m lines describe those pairs with two integer numbers ai and bi (1 ≤ ai, bi ≤ n, ai ≠ bi) on a line. The order of people in a pair is arbitrary and no pair is listed twice.
Output
Write to the output file an integer number k (1 ≤ k ≤ n) — the number of people in the hardest team, followed by k lines listing people from this team in ascending order. If there are multiple teams with the same hardness factor then write any one.
Sample Input
sample input #1 5 6 1 5 5 4 4 2 2 5 1 2 3 1 sample input #2 4 0
Sample Output
sample output #1 4 1 2 4 5 sample output #2 1 1
Hint
Note, that in the last example any team has hardness factor of zero, and any non-empty list of people is a valid answer.
题意:给出一个无向图,n个点,m条边,选一个子图,使得该子图 边数:点数最大。
思路:最大密度子图。
设原点s,汇点t。
原点s和1~n点连接,容量为m。
对于原图中的无向边a,b,连接a->b,容量1,连接b->a,容量1。
二分枚举一个double数字,左边界L = 0,右边界R = m。
跳出条件是while(R – L >= (1 / n / n))
对于每一个mid = (L + R) * 0.5。
1~n每个点和t连接,容量为m + 2 * mid – d[i]。
求最大流得到一个double数字ans。
如果 (m * n – ans) * 0.5大于0, L = mid
否则R = mid。
最后跳出的L就是最大密度。
拿这个L再重新建图,求最大流。
然后从s出发dfs,走残留容量大于0的边,所有能到达的点就是答案。
AC代码:
#include <iostream>
#include <cmath>
#include <cstdlib>
#include <cstring>
#include <cstdio>
#include <queue>
#include <ctime>
#include <vector>
#include <algorithm>
#define ll long long
#define L(rt) (rt<<1)
#define R(rt) (rt<<1|1)
using namespace std;
const double INF = 1e9;
const int maxn = 105;
const double eps = 1e-7;
struct Edge{
int u, v;
double cap, flow;
int next;
}et[maxn * maxn];
int cnt[maxn], dis[maxn], pre[maxn], cur[maxn], eh[maxn], ans[maxn];
double low[maxn];
int n, m, s, t, num, tot;
int a[1005], b[1005], d[maxn];
bool vis[maxn];
void init(){
memset(eh, -1, sizeof(eh));
num = 0;
}
void add(int u, int v, double cap, double flow){
Edge e = {u, v, cap, flow, eh[u]};
et[num] = e;
eh[u] = num++;
}
void addedge(int u, int v, double cap){
add(u, v, cap, 0);
add(v, u, 0, 0);
}
double isap(int s, int t, int nv){
int u, v, now;
double flow = 0;
memset(low, 0, sizeof(low));
memset(cnt, 0, sizeof(cnt));
memset(dis, 0, sizeof(dis));
for(u = 0; u <= nv; u++) cur[u] = eh[u];
low[s] = INF, cnt[0] = nv, u = s;
while(dis[s] < nv)
{
for(now = cur[u]; now != -1; now = et[now].next)
if(et[now].cap - et[now].flow > eps && dis[u] == dis[v = et[now].v] + 1) break;
if(now != -1)
{
cur[u] = pre[v] = now;
low[v] = min(low[u], et[now].cap - et[now].flow);
u = v;
if(u == t)
{
for(; u != s; u = et[pre[u]].u)
{
et[pre[u]].flow += low[t];
et[pre[u]^1].flow -= low[t];
}
flow += low[t];
low[s] = INF;
}
}
else
{
if(--cnt[dis[u]] == 0) break;
dis[u] = nv, cur[u] = eh[u];
for(now = eh[u]; now != -1; now = et[now].next)
if(et[now].cap - et[now].flow > eps&& dis[u] > dis[et[now].v] + 1)
dis[u] = dis[et[now].v] + 1;
cnt[dis[u]]++;
if(u != s) u = et[pre[u]].u;
}
}
return flow;
}
void build(double cc){
init();
for(int i = 1; i <= n; i++)
{
addedge(s, i, m);
addedge(i, t, m + 2 * cc - d[i]);
}
for(int i = 0; i < m; i++)
{
addedge(a[i], b[i], 1);
addedge(b[i], a[i], 1);
}
}
void dfs(int u){
vis[u] = 1;
if(u >= 1 && u <= n) ans[tot++] = u;
for(int i = eh[u]; i != -1; i = et[i].next)
if(et[i].cap - et[i].flow > eps)
{
int v = et[i].v;
if(!vis[v]) dfs(v);
}
}
int main()
{
while(~scanf("%d%d", &n, &m))
{
memset(d, 0, sizeof(d));
s = 0, t = n + 1;
for(int i = 0; i < m; i++)
{
scanf("%d%d", &a[i], &b[i]);
d[a[i]]++;
d[b[i]]++;
}
double low = 0, high = m, mmin = 1.0 / n / n;
while(high - low >= mmin)
{
double mid = (low + high) * 0.5;
build(mid);
if((m * n - isap(s, t, t + 1)) * 0.5 > 0) low = mid;
else high = mid;
}
build(low);
isap(s, t, t + 1);
tot = 0;
memset(vis, 0, sizeof(vis));
dfs(s);
if(tot == 0) ans[tot++] = 1;
sort(ans, ans + tot);
printf("%d\n", tot);
for(int i = 0; i < tot; i++) printf("%d\n", ans[i]);
}
return 0;
}