在这里插入代码片
```#include "stdio.h"
#include "malloc.h"
#define max 20
//创建二叉树结构体
typedef struct node{
char data;
struct node *lchild,*rchild;
}Tree,*btree;
//创建栈
typedef struct{
Tree elem[max];
int top;
}stack;
void init(stack *s){
s->top=0;
}
void push(stack *s,Tree e){
if(s->top==20){
printf("栈满\n");
return;
}else{
s->elem[s->top++]=e;
}
}
void pop(stack *s,Tree *e){
if(s->top==0){
printf("栈空\n");
return;
}else{
s->top--;
*e=s->elem[s->top];
}
}
int empty(stack *s){
if(s->top==0){
return 1;
}else{
return 0;
}
}
//创建二叉树
void creat(btree *t){
char a;
scanf("%c",&a);
if(' '==a){
*t=NULL;
}else{
*t=(btree)malloc(sizeof(btree));
(*t)->data=a;
creat(&((*t)->lchild));
creat(&((*t)->rchild));
}
}
//中序非递归遍历输出
void inorder(btree t){
if(!t){
return;
}
stack s;
init(&s);
Tree e;
btree p=t;
while(p||!empty(&s)){
if(p){
push(&s,*p);
p=p->lchild;
}else{
pop(&s,&e);
printf("%c\n",e.data);
p=e.rchild;
}
}
}
int main(){
btree t=NULL;
printf("输入:\n");
creat(&t);
printf("中序遍历输出:\n");
inorder(t);
}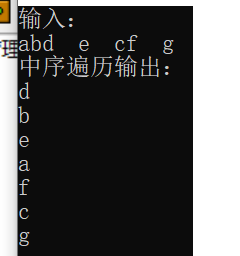
2021-11-14
最新推荐文章于 2025-04-24 19:44:51 发布