using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
AddressBook person = new AddressBook(textBox1.Text, textBox2.Text, textBox3.Text);
textBox4.Text=person.GetMessage();
}
}
}
class AddressBook
{
private string name;
private string phone;
private string email;
public AddressBook(string name,string phone,string email)
{
this.name=name;
this.phone=phone;
this.email=email;
}
public string Name
{
get{return name;}
}
public string Phone
{
get
{
if(phone==null)return"no value";
else return phone;
}
set
{
phone=value;
}
}
public string GetMessage()
{
return string.Format("name:{0}\r\nphone:{1}\r\nEmail:{2}", Name, Phone, email);
}
}
![]()
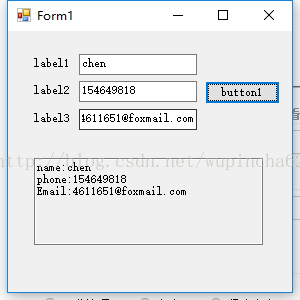
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace C4_Time
{
public partial class Form1 : Form
{
Time now;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
now = new Time();
textBox1.Text = now.ShowHour().ToString();
textBox2.Text = now.ShowMinute().ToString();
textBox3.Text = now.ShowSecond().ToString();
}
private void button1_Click(object sender, EventArgs e)
{
now.AddSecond();
textBox1.Text = now.ShowHour().ToString();
textBox2.Text = now.ShowMinute().ToString();
textBox3.Text = now.ShowSecond().ToString();
}
}
}
class Time
{
private int hour, minute, second;
public Time()
{
hour = System.DateTime.Now.Hour;
minute = System.DateTime.Now.Minute;
second = System.DateTime.Now.Second;
}
public Time(int h, int m, int s)
{
hour = h; minute = m; second = s;
}
public int ShowHour()
{
return hour;
}
public int ShowMinute()
{
return minute;
}
public int ShowSecond()
{
return second;
}
public void AddSecond()
{
second++;
if (second >= 60)
{
second = second % 60;
minute++;
}
if (minute >= 60)
{
minute = minute % 60;
hour++;
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace C5_Score
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
int a;
double ch, ma;
a = Convert.ToInt32(textBox2.Text);
ch = Convert.ToDouble(textBox3.Text);
ma = Convert.ToDouble(textBox4.Text);
Pupil person = new Pupil(textBox1.Text, a, ch, ma);
textBox6.Text += person.getMessage();
}
private void button2_Click(object sender, EventArgs e)
{
int a;
double ch, ma,eng;
a = Convert.ToInt32(textBox2.Text);
ch = Convert.ToDouble(textBox3.Text);
ma = Convert.ToDouble(textBox4.Text);
eng = Convert.ToDouble(textBox5.Text);
middle person = new middle(textBox1.Text, a, ch, ma, eng);
textBox6.Text += person.getMessage();
}
}
}
public abstract class Student
{
protected string name;
protected int age;
public static int number;
public Student(string name, int age)
{
this.name = name;
this.age = age;
number++;
}
public string Name { get { return name; } }
public abstract double Average();
public string getMessage()
{
return string.Format("总人数:{0},姓名:{1},平均成绩:{2}\r\n", number, name, Average());
}
}
public class Pupil : Student
{
protected double chinese;
protected double math;
public Pupil(string name, int age, double chinese, double math)
: base(name, age)
{
this.chinese = chinese;
this.math = math;
}
public override double Average()
{
return (chinese + math) / 2;
}
}
public class middle : Pupil
{
protected double english;
public middle(string name, int age, double chinese, double math, double english)
: base(name, age, chinese, math)
{
this.english = english;
}
public override double Average()
{
return (chinese + math + english) / 3;
}
}