1. 二叉树排序算法
- 构建二叉查找树,对任意输入(字符、整型、浮点型)进行排序;
- BinaryTreeNode 类为树的节点结构,包括节点值,树的左右指针;BinaryTree 类对树节点进行排序的实现方法,包括插入节点insertNode 方法,中序遍历 midOrder 等方法;
- 使用 C++ 实现二叉树的构建,主要运用到 C++ 的知识点有: 容器vector, 智能指针shared_ptr, 模板类template<class T>;
2. C++ 代码实现
- BinaryTreeNode.h
#pragma once
#include <memory>
using std::shared_ptr;
template <class T>
class BinaryTree;
template <class T>
class BinaryTreeNode
{
friend class BinaryTree<T>;
public:
BinaryTreeNode() = default;
BinaryTreeNode(const T &tempValue):value(tempValue),leftChild(nullptr),rightChild(nullptr) {}
private:
T value;
shared_ptr<BinaryTreeNode<T>> leftChild;
shared_ptr<BinaryTreeNode<T>> rightChild;
};
- BinaryTree.h
#pragma once
#include <iostream>
#include <vector>
#include <memory>
#include "BinaryTreeNode.h"
using std::cout;
using std::endl;
using std::vector;
using std::shared_ptr;
using std::make_shared;
template <class T>
class BinaryTree
{
public:
BinaryTree():root(nullptr) {}
void insertNode(const T &nodeValue);
void recursion(shared_ptr<BinaryTreeNode<T>> &root, shared_ptr<BinaryTreeNode<T>> &temp);
void sort();
void midOrder(shared_ptr<BinaryTreeNode<T>> &nodeRoot);
inline void printNode(shared_ptr<BinaryTreeNode<T>> &nodeRoot) const;
vector<T> show;
private:
shared_ptr<BinaryTreeNode<T>> root;
};
template <class T>
void BinaryTree<T>::insertNode(const T &nodeValue)
{
shared_ptr<BinaryTreeNode<T>> temp = make_shared<BinaryTreeNode<T>>(); // The () is needed if want to use make_shared;
temp -> value = nodeValue;
temp -> leftChild = nullptr;
temp -> rightChild = nullptr;
recursion(root, temp);
}
template <class T>
void BinaryTree<T>::recursion(shared_ptr<BinaryTreeNode<T>> &root, shared_ptr<BinaryTreeNode<T>> &temp)
{
if(!root)
{
root = temp;
}
else
{
if(root->value > temp->value)
{
recursion(root->leftChild, temp);
}
else if(root->value == temp->value)
{
;
}
else
{
recursion(root->rightChild, temp);
}
}
}
template <class T>
void BinaryTree<T>::sort()
{
midOrder(root);
}
template <class T>
void BinaryTree<T>::midOrder(shared_ptr<BinaryTreeNode<T>> &nodeRoot)
{
if(nodeRoot)
{
midOrder(nodeRoot->leftChild);
printNode(nodeRoot);
show.push_back(nodeRoot->value);
midOrder(nodeRoot->rightChild);
}
}
template <class T>
inline void BinaryTree<T>::printNode(shared_ptr<BinaryTreeNode<T>> &nodeRoot) const
{
cout << nodeRoot->value << " ";
}
- BinaryTreeNode.cpp
#include <iostream>
#include "../include/BinaryTreeNode.h"
#include "../include/BinaryTree.h"
using std::string;
int main()
{
BinaryTree<int> tree1;
tree1.insertNode(6);
tree1.insertNode(8);
tree1.insertNode(4);
tree1.insertNode(9);
tree1.insertNode(7);
tree1.insertNode(2);
tree1.sort();
BinaryTree<string> tree2;
tree2.insertNode("x");
tree2.insertNode("a");
tree2.insertNode("hx");
tree2.insertNode("un");
tree2.sort();
return 0;
}
3. UT测试
通过上述代码即可实现二叉树查找树的构建,下面对实现的代码进行UT test单元测试,单元测试的好处在于更早的发现功能点或者接口的bug,而不是等到代码都开发完了再去找bug,那样的成本太高了;
测试类 BinaryTree,将文件命名为BinaryTreeTest.cpp,代码如下:
- BinaryTreeTest.cpp
#include <iostream>
#include <vector>
#include "../include/BinaryTreeNode.h"
#include "../include/BinaryTree.h"
#include <gtest/gtest.h>
using std::vector;
using std::string;
namespace
{
TEST(BinaryTreeTest, NodeIntOneInput)
{
BinaryTree<int> tree;
tree.insertNode(6);
tree.sort();
vector<int> expect{6};
EXPECT_EQ(expect, tree.show);
}
TEST(BinaryTreeTest, NodeIntMultInput)
{
BinaryTree<int> tree;
tree.insertNode(6);
tree.insertNode(8);
tree.insertNode(4);
tree.insertNode(3);
tree.insertNode(10);
tree.sort();
vector<int> expect{3, 4, 6, 8, 10};
EXPECT_EQ(expect, tree.show);
}
TEST(BinaryTreeTest, NodeIntMultSameInput)
{
BinaryTree<int> tree;
tree.insertNode(6);
tree.insertNode(8);
tree.insertNode(6);
tree.insertNode(4);
tree.insertNode(3);
tree.sort();
vector<int> expect{3, 4, 6, 8};
EXPECT_EQ(expect, tree.show);
}
TEST(BinaryTreeTest, NodeStringOneInput)
{
BinaryTree<string> tree;
tree.insertNode("ia");
tree.sort();
vector<string> expect{"ia"};
EXPECT_EQ(expect, tree.show);
}
TEST(BinaryTreeTest, NodeStringMultInput)
{
BinaryTree<string> tree;
tree.insertNode("ia");
tree.insertNode("x");
tree.insertNode("ha");
tree.insertNode("u");
tree.insertNode("uia");
tree.sort();
vector<string> expect{"ha", "u", "uia", "x", "ia"};
EXPECT_EQ(expect, tree.show);
}
TEST(BinaryTreeTest, NodeStringMultSameInput)
{
BinaryTree<string> tree;
tree.insertNode("ia");
tree.insertNode("x");
tree.insertNode("ha");
tree.insertNode("u");
tree.insertNode("ha");
tree.sort();
vector<string> expect{"ha", "u", "x", "ia"};
EXPECT_EQ(expect, tree.show);
}
}
4. 实现结果
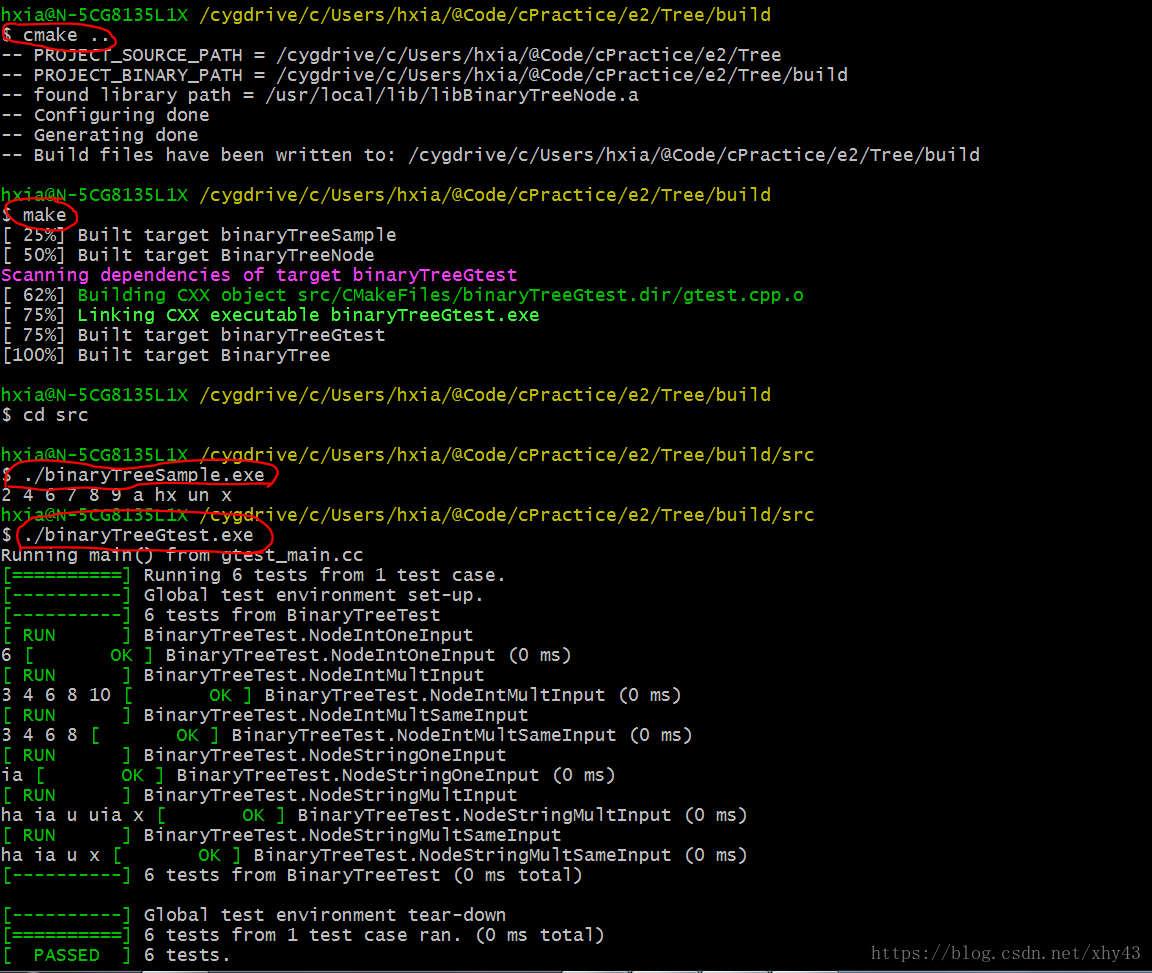
推荐博文:
二叉树的基本功能实现方法(C++) : https://www.cnblogs.com/SarahZhang0104/p/5827532.html
数据结构中排序算法 - 二叉树排序 : https://blog.csdn.net/chenxieyy/article/details/48181203