#pragma mark - UITableViewDelegate
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
if(editingStyle == UITableViewCellEditingStyleDelete)
{
[self.dealsData removeObjectAtIndex:indexPath.row];
[self.tableView reloadData];
}else if (editingStyle == UITableViewCellEditingStyleInsert)
{
NSLog(@"点击%zd行的编辑模式下添加按钮",indexPath.row);
}
}
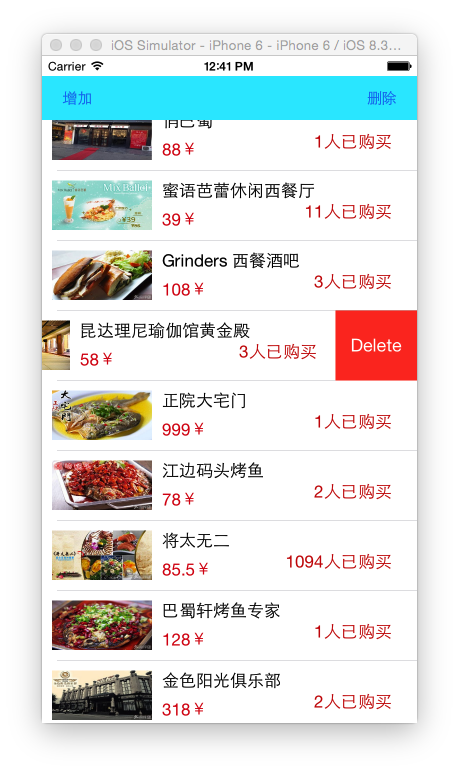
/**
* 左划cell自定义多个功能选项
* 8.0后增加的功能
* @param tableView 所在的tableView
* @param indexPath 左划的行数
*
* @return UITableViewRowAction对象的数组,每一个是对应自定义按钮,比如删除,标记,屏蔽...
*/
- (NSArray *)tableView:(UITableView *)tableView editActionsForRowAtIndexPath:(NSIndexPath *)indexPath
{
UITableViewRowAction *rowaction = [UITableViewRowAction rowActionWithStyle:UITableViewRowActionStyleDefault title:@"标记" handler:^(UITableViewRowAction *action, NSIndexPath *indexPath) {
}];
rowaction.backgroundColor = [UIColor blueColor];
UITableViewRowAction *rowaction1 = [UITableViewRowAction rowActionWithStyle:UITableViewRowActionStyleDestructive title:@"删除" handler:^(UITableViewRowAction *action, NSIndexPath *indexPath) {
}];
UITableViewRowAction *rowaction2 = [UITableViewRowAction rowActionWithStyle:UITableViewRowActionStyleNormal title:@"关心" handler:^(UITableViewRowAction *action, NSIndexPath *indexPath) {
}];
return @[rowaction, rowaction1, rowaction2];
}
/**
+ (instancetype)rowActionWithStyle:(UITableViewRowActionStyle)style title:(NSString *)title handler:(void (^)(UITableViewRowAction *action, NSIndexPath *indexPath))handler;
@property (nonatomic, readonly) UITableViewRowActionStyle style;
@property (nonatomic, copy) NSString *title;
@property (nonatomic, copy) UIColor *backgroundColor; // default background color is dependent on style
@property (nonatomic, copy) UIVisualEffect* backgroundEffect;
*/
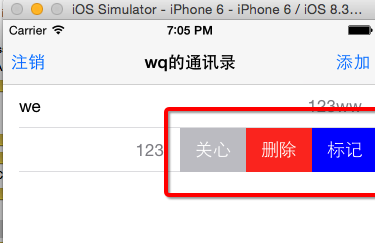
- 编辑模式
- 当进入编辑模式tableView每个cell左侧会显示编辑样式:
删除或者增加 - 可以通过editingStyleForRowAtIndexPath返回每行的编辑样式,不识闲默认为删除样式
- 点击删除会先到左划出现的删除按钮
- 下一步会执行commitEditingStyle方法,对选中行进行操作
[self.tableView setEditing:!self.tableView.isEditing animated:YES];
- (UITableViewCellEditingStyle)tableView:(UITableView *)tableView editingStyleForRowAtIndexPath:(NSIndexPath *)indexPath
{
return indexPath.row % 2 == 0 ? UITableViewCellEditingStyleInsert: UITableViewCellEditingStyleDelete;
}
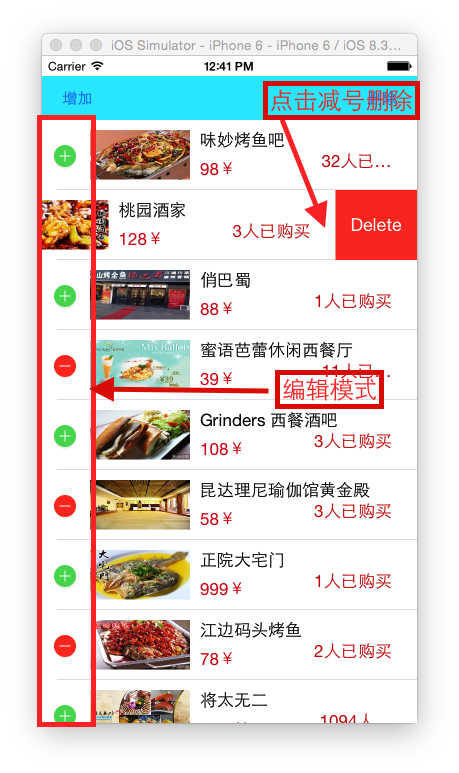