题目:手动实现My_vector,要实现的函数: 构造函数 析构函数 拷贝构造 拷贝赋值 at() empty() full() front() back() size() clear() expand() 二倍扩容函数 push_back() pop_back()
代码:
#include <iostream>
using namespace std;
template<typename T>
class my_vector
{
private:
T *data;
T *first;
T *last;
T *end;
public:
my_vector()
{
data=new T;
first=data;
last=data;
end=data;
}
my_vector(int num,T d)
{
if(num==0)
{
data=new T;
first=data;
last=data;
end=data;
}
else
{
int n=1;
while (num>n)
{
n=n*2;
}
data=new T[n+1];
first=data;
last=data+num;
end=data+n;
for(int i=0;i<num;i++)
{
data[i]=d;
}
}
}
my_vector(T *a,T *b)
{
int num=b-a;
if(num==0)
{
data=new T;
first=data;
last=data;
end=data;
}
else
{
int n=1;
while (num>n)
{
n=n*2;
}
data=new T[n+1];
first=data;
last=data+num;
end=data+n;
for(int i=0;i<num;i++)
{
data[i]=a[i];
}
}
}
~my_vector()
{
delete [] data;
data=nullptr;
first=nullptr;
last=nullptr;
end=nullptr;
}
my_vector(const my_vector &other)
{
int n=other.end-other.first;
int num=other.last-other.first;
data=new T[n+1];
first=data;
last=data+num;
end=data+n;
for(int i=0;i<num;i++)
{
data[i]=other.data[i];
}
}
my_vector &operator=(const my_vector &other)
{
int n=other.end-other.first;
int num=other.last-other.first;
delete [] data;
data=new T[n+1];
first=data;
last=data+num;
end=data+n;
for(int i=0;i<num;i++)
{
data[i]=other.data[i];
}
return *this;
}
void clear()
{
delete [] data;
data=nullptr;
first=nullptr;
last=nullptr;
end=nullptr;
}
bool empty()
{
return first==last?true:false;
}
bool full()
{
return last==end?true:false;
}
int capacity()
{
return end-first;
}
int size()
{
return last-first;
}
void show()
{
for(auto i=first;i!=last;i++)
{
cout<<*i<<" ";
}
cout<<endl;
}
T &at(int num)
{
if(num>=0&&num<=(this->last-this->first))
{
return this->data[num];
}
else
{
throw -1;
}
}
T &front()
{
return *(this->first);
}
T &back()
{
return *((this->last)-1);
}
void expand()
{
if(this->first==this->last&&this->first==this->end)
{
delete [] data;
data=new T[2];
first=data;
last=data;
end=data+1;
}
else
{
int n=end-first;
int num=last-first;
n=n*2;
T *temp=new T[n+1];
first=temp;
last=temp+num;
end=temp+n;
for(int i=0;i<num;i++)
{
temp[i]=data[i];
}
delete [] data;
data=temp;
}
}
void push_back(T a)
{
if(full())
{
expand();
}
*last=a;
last++;
}
void pop_back()
{
if(empty())
{
cout<<"空"<<endl;
}
else
{
last--;
}
}
};
int main()
{
my_vector<int> a1;
a1.show();
cout<<a1.size()<<endl;
cout<<a1.capacity()<<endl;
my_vector<int> a2(9,'a');
a2.show();
cout<<a2.size()<<endl;
cout<<a2.capacity()<<endl;
int arr[] = {3,5,2,4,6,8,7,9};
my_vector<int> a3(arr+1, arr+5);
a3.show();
cout<<a3.size()<<endl;
cout<<a3.capacity()<<endl;
my_vector<int> a4(a3);
a4.show();
a4=a2;
a4.show();
try {
cout<<a3.at(-2)<<endl;
} catch (int e) {
if(e==-1)
{
cout<<"下标错误"<<endl;
}
}
try {
cout<<a3.at(2)<<endl;
} catch (int e) {
if(e==-1)
{
cout<<"下标错误"<<endl;
}
}
a3.front()=1;
a3.back()=0;
a3.show();
for(int i=0;i<20;i++)
{
a1.push_back(i+1);
cout<<a1.size()<<" "<<a1.capacity()<<endl;
}
a1.show();
a1.pop_back();
a1.show();
return 0;
}
运行结果:
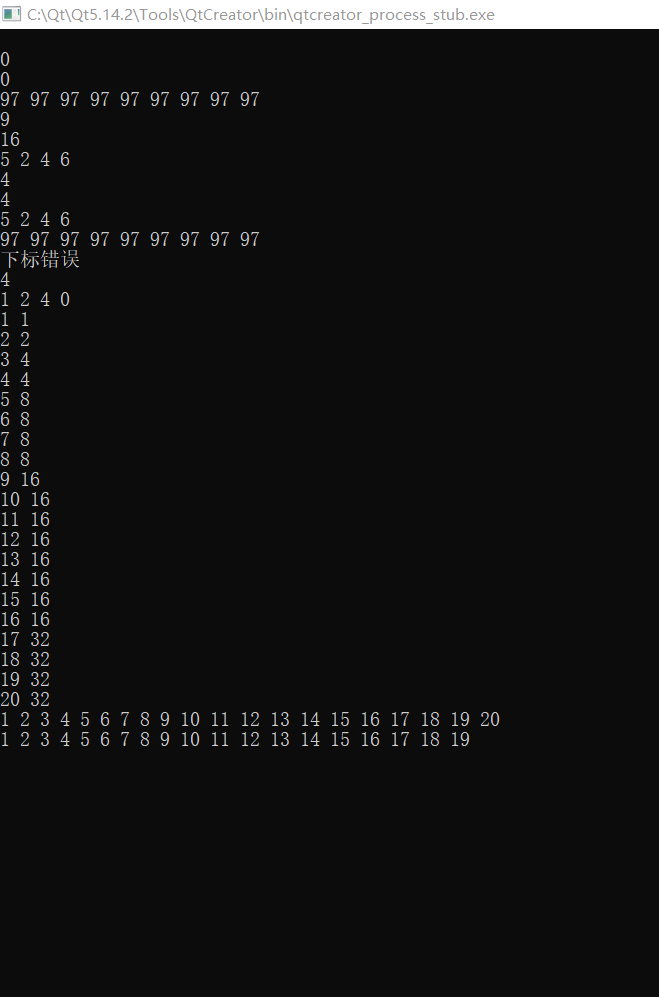