// C#程序结构
using System; //导入命名空间
namespace HelloCSharpApplication // 声明命名空间
{
class HelloCSharp // 声明类
{
static void Main(string[] args) // 声明方法 Main方法是入口点
{
Console.WriteLine("HelloCSharp"); // 控制台打印
}
}
}
// C#基本语法
using System;
namespace RectangleApplication
{
class Rectangle
{
// 成员变量
double length;
double width;
// 成员赋值
public void Acceptdetails()
{
length = 4.5;
width = 3.5;
}
public double GetArea()
{
return length * width;
}
public void Display()
{
Console.WriteLine("Area :{0}", GetArea());
}
}
class ExecuteRectangle
{
static void Main(string[] args)
{
// 类的实例化 类里调用其他类
Rectangle r = new Rectangle();
// 调用类的方法
r.Acceptdetails();
r.Display();
Console.ReadLine();
}
}
}
// C#数据类型 bool byte char decimal double float int long sbyte short uint ulong ushort
// sizepof(type)产生以字节为单位的存储尺寸
// 字符串类型的转义方法
string str = @"C:\Windows";
string str = "C:\\Windows";
// 类型转换方法
// 装箱 值类型转换为对象类型
int val = 8;
object obj = val;
// 拆箱 对象类型转换为值对象
int val = 8;
object obj = val;
int nval = (int) obj;
// C# 类型转换
using System;
namespace TypeConversionApplication
{
class ExplicitConversion
{
static void Main(string[] args)
{
double d = 777.777
int i
// double -> int
i = (int) d;
Console.WriteLine(i);
Console.RedaKey();
}
}
}
// string -> int
using System;
namespace StudyCSharpTest
{
class StudyCSharp
{
static void Main(string[] args)
{
string losctr = 123.ToString();
int i = Convert.ToInt16(losctr);
int ii = int.Parse(losctr);
Console.WriteLine(i);
Console.WriteLine(ii);
}
}
}
// int.TryParse(string s,out int i)
// 该方式也是将数字内容的字符串转换为int类型,但是该方式比int.Parse(string s) 好一些,它不会出现异常,最后一个参数result是输出值,如果转换成功则输出相应的值,转换失败则输出0。
using System;
namespace StudyCSharpTest
{
class StudyCSharp
{
static void Main(string[] args)
{
string s1="abcd";
string s2="1234";
int a,b;
bool bo1=int.TryParse(s1,out a);
Console.WriteLine(s1+" "+bo1+" "+a);
bool bo2=int.TryParse(s2,out b);
Console.WriteLine(s2+" "+bo2+" "+b);
}
}
}
// C#变量
// <data_type> <variable_list>
int i, j, k;
char c, ch;
float f;
double d;
// 变量初始化
<data_type> <variable_list> = value;
int i = 100, f = 5;
char x = 'x';
using System;
namespace VariableDefinition
{
class Program
{
static void Main(string[] args)
{
short a;
int b;
double c;
// 初始化变量
a = 10;
b = 15;
c = a + b;
Console.WriteLine("c = {0}", c);
}
}
}
// C# 中所有可用的算术运算符
using System;
namespace OperatorApp1
{
class Program
{
static void Main(string[] args)
{
int a = 21;
int b = 10;
int c;
c = a + b;
c = a - b;
c = a * b;
c = a / b;
c = a % b;
c = ++a;
c = --a;
c = a;
c += a;
c -= a;
c *= a;
c /= a;
c %= a;
c <<= 2; //二进制左移
c >>= 2; //二进制右移
c &= 2;
c ^= 2;
c |= 2;
}
}
}
c = a++: 先将 a 赋值给 c,再对 a 进行自增运算。
c = ++a: 先将 a 进行自增运算,再将 a 赋值给 c 。
c = a--: 先将 a 赋值给 c,再对 a 进行自减运算。
c = --a: 先将 a 进行自减运算,再将 a 赋值给 c 。
using System;
namespace OperatorApp1
{
class Program
{
static void Main(string[] args)
{
int a = 1;
int b;
// a++ 先赋值再进行自增运算
b = a++; //b = 1, a = 2
// ++a 先自增运算再进行赋值
b = ++a; //b = 2, a = 2
// a-- 先赋值再进行自减运算
b = a--; //b = 1, a = 0
// --a 先自减运算再进行赋值
b = --a; //b = 0, a = 0
}
}
}
// C#判断
//Exp1 ? Exp2 : Exp3; Exp1为真 取Exp2 否则去 Exp3
// if语句
using System;
namespace StudyCSharpTest
{
class StudyCSharp
{
static void Main(string[] args)
{
int a = 6;
if (a < 5)
{
Console.WriteLine("{0}",a+5);
}
else if (a < 10 & a >=5 )
{
Console.WriteLine("{0}",a+5);
}
else
{
Console.WriteLine(a);
}
}
}
}
// switch 语句
using System;
namespace StudyCSharpTest
{
class StudyCSharp
{
static void Main(string[] args)
{
int a = 5;
int b = 10;
switch(a)
{
case 5:
switch(b)
C#学习笔记(一):C#基础语法千行(含实例)
最新推荐文章于 2024-07-09 12:13:30 发布
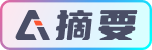