.h
#ifndef _BINARYSEARCHTREE_H
#define _BINARYSEARCHTREE_H
struct tree_node;
typedef int element_type;
typedef struct tree_node* search_tree;
struct tree_node{
element_type element;
search_tree left;
search_tree right;
};
search_tree make_empty(search_tree root);
search_tree find(search_tree root,element_type e);
search_tree find_min(search_tree root);
search_tree find_max(search_tree root);
search_tree insert(search_tree root,element_type e);
search_tree delete(search_tree root,element_type e);
element_type retrieve(search_tree root);
#endif
.c
#include "BinarySearchTree.h"
#include <stdio.h>
#include <stdlib.h>
#include <memory.h>
static search_tree delete_min(search_tree root);
static void fatal_error(const char* msg);
search_tree make_empty(search_tree root){
if(root != NULL){
make_empty(root->left);
make_empty(root->right);
free(root);
}
return NULL;
}
search_tree find(search_tree root,element_type e){
if(root == NULL){
return NULL;
}
if(e < root->element){
return find(root->left,e);
}else if(e > root->element){
return find(root->right,e);
}else{
return root;
}
}
search_tree find_min(search_tree root){
if(root == NULL){
return NULL;
}else if(root->left == NULL){
return root;
}else{
return find_min(root->left);
}
}
search_tree find_max(search_tree root){
if(root == NULL){
return NULL;
}
while(root->right!=NULL){
root = root->right;
}
return root;
}
search_tree insert(search_tree root,element_type e){
if(root == NULL){
root = malloc(sizeof(struct tree_node));
if(root == NULL){
fatal_error("malloc failed");
}
root->element = e;
root->left = root->right = NULL;
}else {
search_tree father;
search_tree node = root;
while(node != NULL){
father = node;
if(e < node->element){
node = node->left;
}else if(e > node->element){
node = node->right;
}else{
return root;
}
}
node = malloc(sizeof(struct tree_node));
if(node == NULL){
fatal_error("malloc failed");
}
node->element = e;
node->left = node->right = NULL;
if(e < father->element){
father->left = node;
}else{
father->right = node;
}
}
return root;
}
#if 0
search_tree insert(search_tree root,element_type e){
if(root == NULL){
root = malloc(sizeof(struct tree_node));
if(root == NULL){
fatal_error("malloc failed");
}
root->element = e;
root->left = root->right = NULL;
}else if(e < root->element){
root->left = insert(root->left,e);
}else if(e > root->element){
root->right = insert(root->right,e);
}
return root;
}
#endif
search_tree delete(search_tree root,element_type e){
if(root == NULL){
return NULL;
}else if(e < root->element){
root->left = delete(root->left,e);
}else if(e > root->element){
root->right = delete(root->right,e);
}else if(root->left && root->right){
search_tree temp = find_min(root->right);
root->element = temp->element;
root->right = delete(root->right,e);
}else{
search_tree temp = root;
if(root->left == NULL){
root = root->right;
}else{
root = root->left;
}
free(temp);
}
return root;
}
search_tree delete_min(search_tree root){
if(root == NULL){
return NULL;
}else if(root->left == NULL){
search_tree right = root->right;
free(root);
return right;
}else{
root->left = delete_min(root->left);
return root;
}
}
#if 0
static delete_min(search_tree root){
if(root == NULL){
return NULL;
}else if(root->left == NULL){
search_tree right = root->right;
free(root);
return right;
}else{
search_tree father = root;
search_tree node = root;
while(node->left != NULL){
father = node;
node = node->left;
}
father->left = node->right;
free(node);
return father;
}
}
#endif
element_type retrieve(search_tree root){
if(root) return root->element;
}
void fatal_error(const char* msg){
fprintf(stderr,"%s\n",msg);
exit(1);
}
test
#include "BinarySearchTree.h"
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
void print_tree(search_tree tree,int depth){
if(tree != NULL){
for(int i = 0;i<depth;++i){
printf(" ");
}
printf("%d\n",retrieve(tree));
print_tree(tree->left,depth+1);
print_tree(tree->right,depth+1);
}
}
int main(int argc,char** argv){
search_tree tree=NULL;
tree = insert(tree,7);
assert(tree != NULL);
insert(tree,3);
insert(tree,9);
insert(tree,4);
insert(tree,2);
insert(tree,5);
insert(tree,8);
print_tree(tree,0);
printf("min=%d\n",retrieve(find_min(tree)));
printf("max=%d\n",retrieve(find_max(tree)));
printf("find 4 =%d\n",retrieve(find(tree,4)));
tree = delete(tree,4);
print_tree(tree,0);
tree = delete(tree,8);
print_tree(tree,0);
tree = make_empty(tree);
print_tree(tree,0);
return 0;
}
out
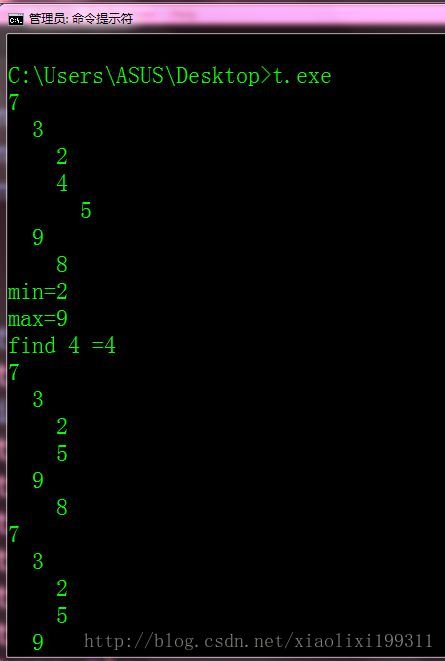