easypoi依赖导出word依赖
<dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-base</artifactId> <version>3.0.3</version> </dependency> <dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-web</artifactId> <version>3.0.3</version> </dependency> <dependency> <groupId>cn.afterturn</groupId> <artifactId>easypoi-annotation</artifactId> <version>3.0.3</version> </dependency>
easyexcel依赖导出excel依赖
<dependency> <groupId>com.alibaba</groupId> <artifactId>easyexcel</artifactId> <version>2.2.8</version> </dependency>
word转pdf依赖
<dependency> <groupId>com.documents4j</groupId> <artifactId>documents4j-local</artifactId> <version>1.0.3</version> </dependency> <dependency> <groupId>com.documents4j</groupId> <artifactId>documents4j-transformer-msoffice-word</artifactId> <version>1.0.3</version> </dependency>
导出word无法使用添加依赖
<dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>4.1.2</version> </dependency> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>4.1.2</version> </dependency> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml-schemas</artifactId> <version>4.1.2</version> </dependency>
word工具类
/**
* 导出word
* <p>第一步生成替换后的word文件,只支持docx</p>
* <p>第二步下载生成的文件</p>
* <p>第三步删除生成的临时文件</p>
* 模版变量中变量格式:{{foo}}
* @param templatePath word模板地址
* @param temDir 生成临时文件存放地址
* @param fileName 文件名
* @param params 替换的参数
* @param request HttpServletRequest
* @param response HttpServletResponse
*/
public static void exportWord(String templatePath, String temDir, String fileName, Map<String, Object> params, HttpServletRequest request, HttpServletResponse response) {
Assert.notNull(templatePath,"模板路径不能为空");
Assert.notNull(temDir,"临时文件路径不能为空");
Assert.notNull(fileName,"导出文件名不能为空");
Assert.isTrue(fileName.endsWith(".docx"),"word导出请使用docx格式");
if (!temDir.endsWith("/")){
temDir = temDir + File.separator;
}
File dir = new File(temDir);
if (!dir.exists()) {
dir.mkdirs();
}
try {
String userAgent = request.getHeader("user-agent").toLowerCase();
if (userAgent.contains("msie") || userAgent.contains("like gecko")) {
fileName = fileName;
// fileName = URLEncoder.encode(fileName, "UTF-8");
} else {
fileName = new String(fileName.getBytes("utf-8"), "ISO-8859-1");
}
XWPFDocument doc = WordExportUtil.exportWord07(templatePath, params);
String tmpPath = temDir + fileName;
FileOutputStream fos = new FileOutputStream(tmpPath);
doc.write(fos);
// 设置强制下载不打开
response.setContentType("application/force-download");
// 设置文件名
response.addHeader("Content-Disposition", "attachment;fileName=" + fileName);
OutputStream out = response.getOutputStream();
doc.write(out);
out.close();
} catch (Exception e) {
e.printStackTrace();
} finally {
// delAllFile(temDir);//这一步看具体需求,要不要删
deleteTempFile(temDir, filename);
}
}
/**
* 删除临时生成的文件
*/
public static void deleteTempFile(String filePath, String fileName) {
File file = new File(filePath + fileName);
File f = new File(filePath);
file.delete();
f.delete();
}
导出excel工具类
/**
* 导出Excel
*
* @param headList Excel表头列表
* @param dataList Excel内容列表
* @param sheetName Excel工作表名称
* @param response 响应对象
* @throws IOException
*/
public static void exportExcel(List<String> headList, List<LinkedHashMap<String, Object>> dataList, String sheetName, HttpServletResponse response)
throws IOException {
ServletOutputStream servletOutputStream = null;
try {
servletOutputStream = response.getOutputStream();
response.setContentType("multipart/form-data");
response.setCharacterEncoding("utf-8");
String fileName = new String((sheetName + new SimpleDateFormat("yyyy-MM-dd").format(new Date())).getBytes(), StandardCharsets.UTF_8);
response.setHeader("Content-Disposition", "attachment;filename=" + fileName + ".xlsx");
ExcelWriter writer = new ExcelWriter(servletOutputStream, ExcelTypeEnum.XLSX, true);
Sheet sheet = new Sheet(1, 0);
sheet.setSheetName(sheetName);
Table table = new Table(1);
List<List<String>> head = new ArrayList<>();
if (!CollectionUtils.isEmpty(headList)) {
headList.forEach(headName -> head.add(Arrays.asList(headName)));
}
table.setHead(head);
List<List<String>> data = new ArrayList<>();
if (!CollectionUtils.isEmpty(dataList)) {
dataList.forEach(map -> {
List<String> list = new ArrayList<>();
for (Entry<String, Object> entry : map.entrySet()) {
Object value = entry.getValue();
list.add(value == null ? null : value.toString());
}
data.add(list);
});
}
writer.write0(data, sheet, table);
writer.finish();
servletOutputStream.flush();
} catch (IOException e) {
throw new ServiceException(e.toString());
} finally {
if (servletOutputStream != null) {
servletOutputStream.close();
}
}
}
word转pdf工具类
/**
* word转pdf
* @param staticsUrl 静态资源地址
* @param name 文档名称
*/
public static void docxToPdf(String staticsUrl,String name){
File inputWord = new File(staticsUrl + "/" + name +".docx");//word文档位置
File outputFile = new File(staticsUrl+ "/" + name +".pdf");//转成pdf后存放位置
try {
InputStream docxInputStream = new FileInputStream(inputWord);
OutputStream outputStream = new FileOutputStream(outputFile);
IConverter converter = LocalConverter.builder().build();
converter.convert(docxInputStream).as(DocumentType.DOCX).to(outputStream).as(DocumentType.PDF).execute();
outputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
导出word,加转pdf然后展示案例
@PostMapping(value = "/export")
public void exportXLS(@RequestBody ExcelVO excelVO ,HttpServletRequest request, HttpServletResponse response){
String[] headList = excelVO.getHeadList();//表格标题
List<String[]> dataSource = excelVO.getDataList();//选择的数据
String sheetName = excelVO.getSheetName();//工单编号
List<LinkedHashMap<String,Object>> dataList = new ArrayList<>();
dataSource.forEach(item -> {
LinkedHashMap<String,Object> map = new LinkedHashMap<>();
for (int i = 0; i < item.length; i++) {
map.put(headList[i],item[i]);
}
dataList.add(map);
});
List<Map<String, Object>> collect = dataList.stream().map(item -> {
Map<String, Object> map = new HashMap<String, Object>();
map.put("number", item.get("工单编号"));
map.put("code", item.get("零件编码"));
map.put("name", item.get("零件名称"));
map.put("quantity", item.get("计划数"));
map.put("time", item.get("计划工时"));
map.put("staff", item.get("生产员工"));
map.put("seat", item.get("生产机位"));
return map;
}).collect(Collectors.toList());
Map<String, Object> map = new HashMap<String, Object>();
map.put("taxlist", collect);//循环数据
map.put("totalpreyear", "2660");//单条数据
map.put("totalthisyear", "3400");//单条数据
String str = UUID.randomUUID().toString();
FileUtils.exportWord("static/word/派遣模板.docx",staticsUrl,str+".docx",map,request,response);
FileUtils.docxToPdf(staticsUrl,str);
String URL = "api/mes"+ askTop + "/"+str+".pdf";
ps02Service.batchProductCode(sheetName,URL);//将地址存入数据库,前端跳转用
}
模板位置
前端按钮事件
const $table = this.$refs.mainTableTwo
const records = $table.getCheckboxRecords()
if (records.length == 0) {
this.$message({
message: '请选择至少一条记录',
type: 'warning'
})
} else {
// 获得主表的表头
const headList = []
const fieldList = []
const headColumn = $table.getTableColumn().collectColumn
for (let i = 0; i < headColumn.length; i++) {
if (headColumn[i].title !== undefined) {
headList.push(headColumn[i].title)
fieldList.push(headColumn[i].field)
}
}
// 获得表格内填充的数据
let data = []
const dataList = []
for (let i = 0; i < records.length; i++) {
for (let j = 0; j < fieldList.length; j++) {
const fieldName = fieldList[j]
data.push(records[i][fieldName])
}
dataList.push(data)
data = []
}
const sheetName = dataList[0][0]
exportXLS(dataList, headList, sheetName).then(response => {
selectDetailed(this.queryWork).then(response => {
this.subList = response.data.list
window.open(this.subList[0].url)
})
})
}
},
跳转成功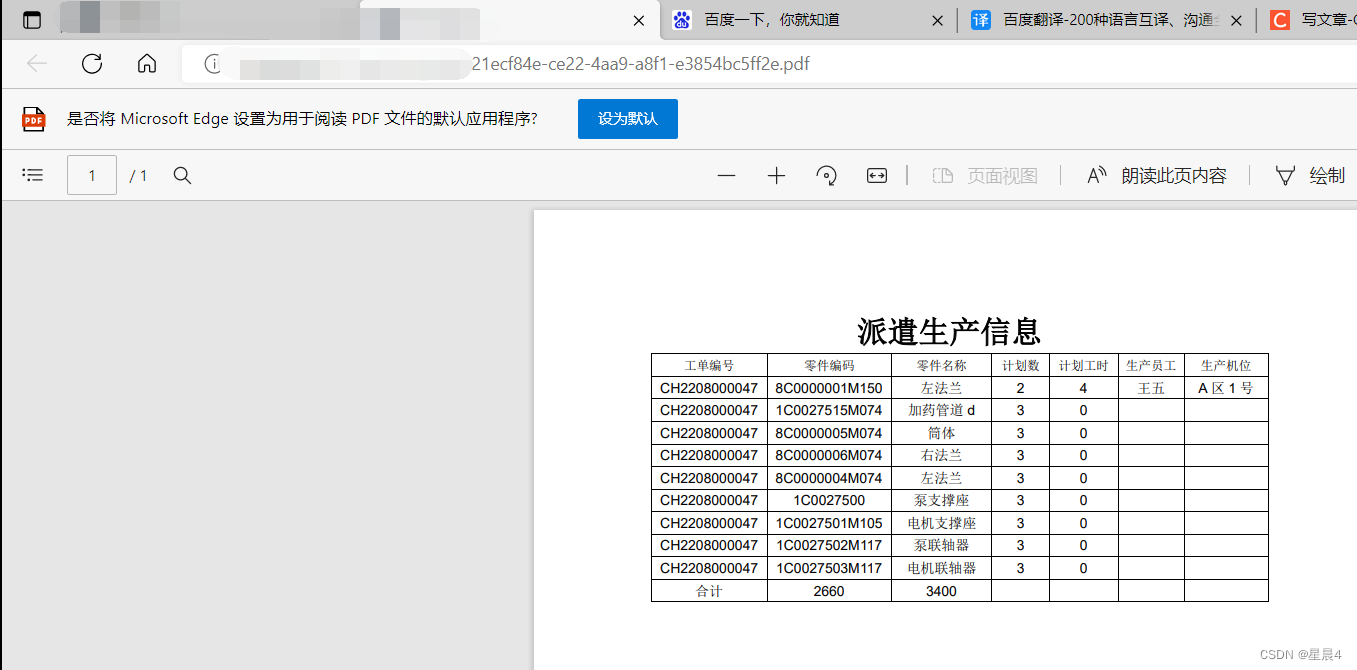
模板样式
导出excel案例
@PostMapping(value = "/export")
public void exportXLS(@RequestBody ExcelVO excelVO , HttpServletResponse response){
// 获取表格数据
String[] headList = excelVO.getHeadList();
List<String[]> dataSource = excelVO.getDataList();
String sheetName = excelVO.getSheetName();
List<LinkedHashMap<String,Object>> dataList = new ArrayList<>();
dataSource.forEach(item -> {
LinkedHashMap<String,Object> map = new LinkedHashMap<>();
for (int i = 0; i < item.length; i++) {
map.put(headList[i],item[i]);
}
dataList.add(map);
});
try {
ExcelUtils.exportExcel(Arrays.asList(headList),dataList,sheetName,response);
} catch (IOException e) {
e.printStackTrace();
}
}
VO
public class ExcelVO { private String[] headList; private List<String[]> dataList; private String sheetName; public String[] getHeadList() { return headList; } public void setHeadList(String[] headList) { this.headList = headList; } public List<String[]> getDataList() { return dataList; } public void setDataList(List<String[]> dataList) { this.dataList = dataList; } public String getSheetName() { return sheetName; } public void setSheetName(String sheetName) { this.sheetName = sheetName; } @Override public String toString() { return "ExcelVO{" + "headList=" + Arrays.toString(headList) + ", dataList=" + dataList + ", sheetName='" + sheetName + '\'' + '}'; } }
前端事件
// 导出从表数据
handleSubExport() {
// 获得从表的表头
const headList = []
const fieldList = []
const $table = this.$refs.subTable
const headColumn = $table.getTableColumn().collectColumn
for (let i = 0; i < headColumn.length; i++) {
if (headColumn[i].title !== undefined) {
headList.push(headColumn[i].title)
fieldList.push(headColumn[i].field)
}
}
// 获得表格内填充的数据
let data = []
const dataList = []
const records = $table.getCheckboxRecords()
for (let i = 0; i < records.length; i++) {
for (let j = 0; j < fieldList.length; j++) {
const fieldName = fieldList[j]
data.push(records[i][fieldName])
}
dataList.push(data)
data = []
}
console.log(dataList)
const sheetName = '测试'
exportXLS(dataList, headList, sheetName).then(response => {
const blob = new Blob([response], { type: 'multipart/form-data' })
const a = document.createElement('a')
// 创建URL
const blobUrl = window.URL.createObjectURL(blob)
const nowDate = new Date()
const date = {
year: nowDate.getFullYear(),
month: nowDate.getMonth() + 1,
date: nowDate.getDate()
}
if (parseInt(date.date) < 10) {
date.date = '0' + date.date
}
a.download = '测试' + '.xlsx'
a.href = blobUrl
document.body.appendChild(a)
// 下载文件
a.click()
// 释放内存
URL.revokeObjectURL(blobUrl)
document.body.removeChild(a)
})
},
需要注意的是这里没用使用模板
标题和数据内容都是通过element ui 获取然后传到后端