思路
- 遍历生成且设置按钮
- 当手指点击或者滑动的时候拿到当前触摸点,遍历每一个按钮,将当前触摸点转换为按钮上点,判断时候在按钮上
- 如果在按钮上,设置当前按钮为选中状态,添加到选中按钮数组中
- 在drawInRect:中画线,遍历每一个按钮,让第一个按钮的中心点为线段的起点
- 松开手指时,清空选中按钮数组中的值,重绘
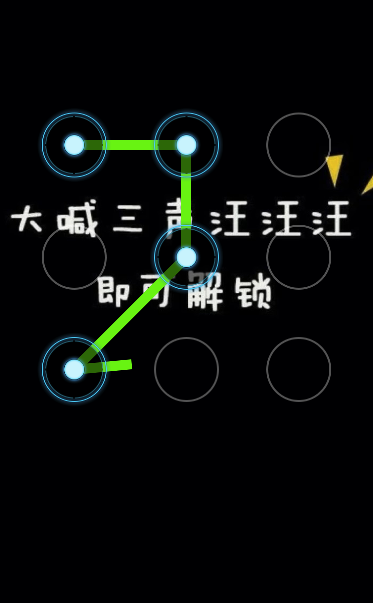
@interface LockView ()
@property (nonatomic, assign) CGPoint curP;
@property (nonatomic, strong) NSMutableArray *selectedBtns;
@end
@implementation LockView
- (NSMutableArray *)selectedBtns
{
if (_selectedBtns == nil) {
_selectedBtns = [NSMutableArray array];
}
return _selectedBtns;
}
- (void)drawRect:(CGRect)rect
{
if (self.selectedBtns.count == 0) return;
UIBezierPath *path = [UIBezierPath bezierPath];
int i = 0;
for (UIButton *selBtn in self.selectedBtns) {
if (i == 0) {
[path moveToPoint:selBtn.center];
}else{
[path addLineToPoint:selBtn.center];
}
i++;
}
[path addLineToPoint:_curP];
[[UIColor greenColor] set];
path.lineWidth = 10;
path.lineJoinStyle = kCGLineJoinRound;
[path stroke];
}
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event
{
[self selectBtnWithTouches:touches withEvent:event];
}
- (void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event
{
[self selectBtnWithTouches:touches withEvent:event];
[self setNeedsDisplay];
}
- (void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event
{
NSMutableString *strM = [NSMutableString string];
for (UIButton *selBtn in self.selectedBtns) {
selBtn.selected = NO;
[strM appendFormat:@"%ld",selBtn.tag];
}
NSLog(@"%@",strM);
[self.selectedBtns removeAllObjects];
[self setNeedsDisplay];
}
- (void)selectBtnWithTouches:(NSSet *)touches withEvent:(UIEvent *)event
{
UITouch *touch = [touches anyObject];
CGPoint curP = [touch locationInView:self];
_curP = curP;
for (UIButton *btn in self.subviews) {
CGPoint btnP = [self convertPoint:curP toView:btn];
if ([btn pointInside:btnP withEvent:event] && btn.selected == NO) {
btn.selected = YES;
[self.selectedBtns addObject:btn];
}
}
}
- (void)awakeFromNib
{
for (int i = 0; i < 9; i++) {
UIButton *btn = [UIButton buttonWithType:UIButtonTypeCustom];
btn.tag = i;
[btn setImage:[UIImage imageNamed:@"gesture_node_normal"] forState:UIControlStateNormal];
[btn setImage:[UIImage imageNamed:@"gesture_node_highlighted"] forState:UIControlStateSelected];
btn.userInteractionEnabled = NO;
[self addSubview:btn];
}
}
- (void)layoutSubviews
{
[super layoutSubviews];
int col = 0;
int row = 0;
int cols = 3;
CGFloat x = 0;
CGFloat y = 0;
CGFloat wh = 74;
CGFloat margin = (self.bounds.size.width - cols * wh) / (cols + 1);
for (int i = 0; i < 9; i++) {
UIButton *btn = self.subviews[i];
col = i % cols;
row = i / cols;
x = margin + (margin + wh) * col;
y = (margin + wh) * row;
btn.frame = CGRectMake(x, y, wh, wh);
}
}
@end