Description
'Oh no, they've done it again', cries the chief designer at the Waferland chip factory. Once more the routing designers have screwed up completely, making the signals on the chip connecting the ports of two functional blocks cross each other all over the place. At this late stage of the process, it is too expensive to redo the routing. Instead, the engineers have to bridge the signals, using the third dimension, so that no two signals cross. However, bridging is a complicated operation, and thus it is desirable to bridge as few signals as possible. The call for a computer program that finds the maximum number of signals which may be connected on the silicon surface without crossing each other, is imminent. Bearing in mind that there may be thousands of signal ports at the boundary of a functional block, the problem asks quite a lot of the programmer. Are you up to the task?
A typical situation is schematically depicted in figure 1. The ports of the two functional blocks are numbered from 1 to p, from top to bottom. The signal mapping is described by a permutation of the numbers 1 to p in the form of a list of p unique numbers in the range 1 to p, in which the i:th number specifies which port on the right side should be connected to the i:th port on the left side.Two signals cross if and only if the straight lines connecting the two ports of each pair do.
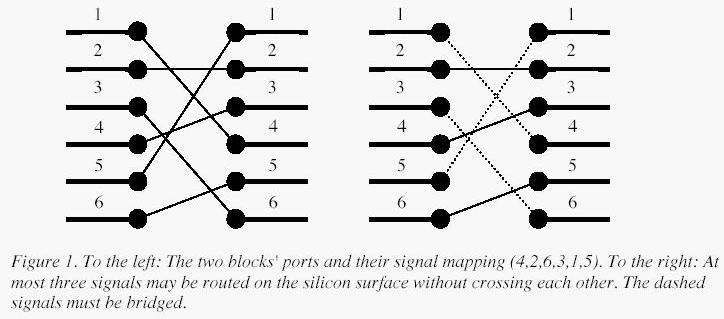
A typical situation is schematically depicted in figure 1. The ports of the two functional blocks are numbered from 1 to p, from top to bottom. The signal mapping is described by a permutation of the numbers 1 to p in the form of a list of p unique numbers in the range 1 to p, in which the i:th number specifies which port on the right side should be connected to the i:th port on the left side.Two signals cross if and only if the straight lines connecting the two ports of each pair do.
Input
On the first line of the input, there is a single positive integer n, telling the number of test scenarios to follow. Each test scenario begins with a line containing a single positive integer p < 40000, the number of ports on the two functional blocks. Then follow p lines, describing the signal mapping:On the i:th line is the port number of the block on the right side which should be connected to the i:th port of the block on the left side.
Output
For each test scenario, output one line containing the maximum number of signals which may be routed on the silicon surface without crossing each other.
Sample Input
4
6
4
2
6
3
1
5
10
2
3
4
5
6
7
8
9
10
1
8
8
7
6
5
4
3
2
1
9
5
8
9
2
3
1
7
4
6
Sample Output
3
9
1
4
样例:
#include <stdio.h>
int f[40010];
int longest(int a,int l,int r) //定义二分查找函数
{
int m;
while(l<=r)
{
m=(l+r)/2;
if(f[m]==a) //如果出现当前值和查找的值相同那么自然就更新当前的f[]值,也可理解为不用更新
{
l=m;
return l;
}
else if(f[m]>a)
r=m-1;
else
l=m+1;
}
return l; //每次返回的都是在f[]中最大的j能够使得f[j]<a[i]的j+1.
}
int main()
{
int N,n,a,i,t,k,j;
scanf("%d",&N);
while(N--)
{
t=0;
scanf("%d",&n);
for(i=0;i<n;i++)
{
scanf("%d",&a); //每次输入一个数就处理一个数
k=longest(a,1,t);
if(k<=t) //如果k<=t说明是情况3.
f[k]=a;
else //否则为情况1,表示最大长度可以更新
{t++;f[t]=a;}
}
printf("%d\n",t);
}
return 0;
}
题目大概意思:
先输入一个数N表示有N组测试数据
每组数据先输入一个数n表示有n个
接下来的n排分别表示左边1~n与右边的连接序号.
输出最多有多少不交叉的连接.
这道题实质就是数列中最长不下降子序列问题. 具体:(2)最长不下降子序列问题____动态规划