一,利用内容提供者读取数据库联系人:
1,联系人数据在contact2数据库中的存储方式:
(图contact)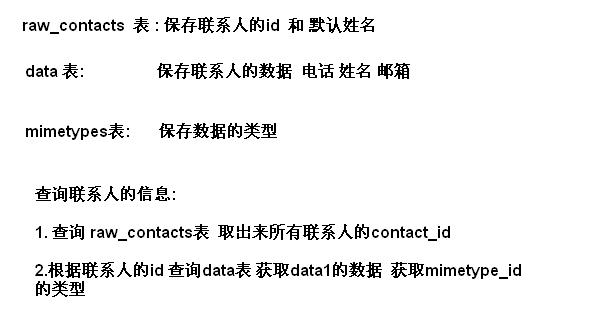
2,实现获取联系人数据的代码
package cn.guoqing.readContacts.service;
import java.util.ArrayList;
import java.util.List;
import cn.guoqing.readContacts.domain.Contact;
import android.content.ContentResolver;
import android.content.Context;
import android.database.Cursor;
import android.net.Uri;
/**
* 从系统的数据库中获取用户的记录信息
* @author YGQ
*
*/
public class ReadContactsService {
public static List<Contact> getContactInfos(Context context){
//1.获取内容解析者
ContentResolver resolver = context.getContentResolver();
//2,获取raw_contacts表的URI --->暗号
Uri uri_contact = Uri.parse("content://com.android.contacts/data");
Uri uri=Uri.parse("content://com.android.contacts/raw_contacts");
//3,查询raw_contacts表获取联系人的id
Cursor cursor = resolver.query(uri, new String[]{"contact_id"}, null, null, null);
// System.out.println(cursor.getCount());
//遍历结果集中的用户id
//用于存储联系人的集合
ArrayList<Contact> list=new ArrayList<Contact>();
while(cursor.moveToNext()){
String contact_id = cursor.getString(0);
//利用联系人的id查询data表,获取联系人的详细记录
Contact contact = new Contact();;
Cursor cursor2 = resolver.query(uri_contact, new String[]{"mimetype","data1"}, "raw_contact_id=?", new String[]{contact_id}, null);
// System.out.println(cursor2.getCount());
while(cursor2.moveToNext()){
String mimetype = cursor2.getString(0);
String data1 = cursor2.getString(1);
//判断mimetypq的类型,看是否为姓名,电话,email
if("vnd.android.cursor.item/phone_v2".equals(mimetype)){
contact.setPhone(data1);
}
if("vnd.android.cursor.item/email_v2".equals(mimetype)){
contact.setEmail(data1);
}
if("vnd.android.cursor.item/name".equals(mimetype)){
contact.setName(data1);
}
}
//将一个用户的记录填充到contact对象中
list.add(contact);
}
//返回所用的用户记录信息
return list;
}
}
二,从互联网上查看图片
package cn.guoqing.picnet;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends Activity {
protected static final int SUCCESS = 1;
protected static final int ERROR = 2;
protected static final int LOAD = 3;
ImageView ivimage=null;
EditText eturl=null;
@SuppressLint("HandlerLeak")
@SuppressWarnings("all")
private Handler handler =new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case SUCCESS:
Bitmap bitmap=(Bitmap) msg.obj;
ivimage.setImageBitmap(bitmap);
break;
case ERROR:
Toast.makeText(getApplicationContext(), "链接服务器获取资源错误", 0).show();
break;
case LOAD:
Toast.makeText(getApplicationContext(), "下载图片失败!", 0).show();
break;
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ivimage=(ImageView) findViewById(R.id.iv_image);
eturl=(EditText) findViewById(R.id.et_url);
}
public void click(View view){
final String text = eturl.getText().toString().trim();
if(TextUtils.isEmpty(text)){
Toast.makeText(getApplicationContext(), "资源路经不能为空", 0).show();
}
else{
new Thread(){
@Override
public void run() {
try {
URL url = new URL(text);
//System.out.println(url);
//1,获取链接资源的对象
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
//2,设置链接超时时间
connection.setConnectTimeout(2000);
//3,设置访问方式
connection.setRequestMethod("GET");
//connection.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 8.0; Windows NT 6.1; Trident/4.0; SLCC2; .NET CLR 2.0.50727; .NET CLR 3.5.30729; .NET CLR 3.0.30729; .NET4.0C; .NET4.0E)");
//获取访问时返回的状态码
int code = connection.getResponseCode();
if(code==200){
//获取服务端返回的资源流
InputStream is = connection.getInputStream();
/*//定义一个用于存储返回资源的输出流对象
ByteArrayOutputStream baos=new ByteArrayOutputStream();
//读取流中数据
int len=0;
byte[] buf=new byte[1024];
while((len=is.read(buf))!=-1){
baos.write(buf, 0, len);
}
baos.close();
is.close();*/
//将流进行封装
Bitmap bitmap = BitmapFactory.decodeStream(is);
//子线程不能更新view上的内容,需要将封装的流,以发送消息的方式发送给主线程
Message message = new Message();
//设置消息的内容,和状态码
message.obj=bitmap;
message.what=SUCCESS;
//通过hundler向主线程发送消息
handler.sendMessage(message);
}
else{
//Toast.makeText(getApplicationContext(), "没有正确的访问到资源", 0).show();
Message message = new Message();
message.what=ERROR;
handler.sendMessage(message);
}
} catch (Exception e) {
//Toast.makeText(getApplicationContext(), "获取资源错误", 0).show();
Message message = new Message();
message.what=LOAD;
handler.sendMessage(message);
e.printStackTrace();
}
}
}.start();
}
}
}
注意:应用程序无响应: ANR
需要添加一个访问网络的权限:android.permission.INTERNET
4.0以上的系统引入了一个新的异常:
android.os.NetworkOnMainThreadException
网络的操作不允许在主线程里面执行,
可能会消耗很长的时间,导致ANR异常的8.3. 获取XML产生,所以google在4.0以上的系统不允许
在主线程里面直接访问网络
08-17 02:54:17.154: W/System.err(1776): android.view.ViewRootImpl$CalledFromWrongThreadException: Only the original thread that created a view hierarchy can touch its views.
只有原始的线程创建的view对象 才可以操作他的view
只有UI线程 才可以修改界面 修改view对象里面的内容
在android操作系统里面有一个原则: 只有主线程(ui线程) 才可以修改view对象里面的内容.
子线程与主线程之间怎样实现消息的更新?
利用消息机制来实现子线程更新主线程
//当主线程中有任务式就调用handleMessage(Message msg)方法() 实时监控队列中是否有消息
public Looper getMainLooper() {
return null;
}
三,获取XML
package cn.guoqing.scanHtml;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import android.app.Activity;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
protected static final int SUCCESS = 1;
protected static final int ERROR = 2;
protected static final int LODE = 3;
TextView tvhtml=null;
EditText eturl=null;
Handler handler=new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case SUCCESS:
tvhtml.setText((String)msg.obj);
break;
case ERROR:
Toast.makeText(getApplicationContext(),"链接资源失败", 0).show();
break;
case LODE:
Toast.makeText(getApplicationContext(),"下载失败", 0).show();
break;
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvhtml=(TextView) findViewById(R.id.tv_html);
eturl=(EditText) findViewById(R.id.et_url);
}
public void click(View view){
final String path=eturl.getText().toString().trim();
if(TextUtils.isEmpty(path)){
Toast.makeText(this, "您输入的地址为空!", 0).show();
}
else{
new Thread(){
@Override
public void run() {
super.run();
try {
//获取资源的url
URL url = new URL(path);
//获取链接资源的connection
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(3000);
connection.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 8.0; Windows NT 6.1; Trident/4.0; SLCC2; .NET CLR 2.0.50727; .NET CLR 3.5.30729; .NET CLR 3.0.30729; .NET4.0C; .NET4.0E)");
int code = connection.getResponseCode();
if(code==200){
//获取服务器返回的流
InputStream is = connection.getInputStream();
ByteArrayOutputStream baos=new ByteArrayOutputStream();
byte[] buf=new byte[1024];
int len=0;
while((len=is.read(buf))!=-1){
baos.write(buf, 0, len);
}
baos.close();
String cintenthtml = new String (baos.toByteArray(),"gbk");
Message msg=new Message();
msg.obj=cintenthtml;
msg.what=SUCCESS;
handler.sendMessage(msg);
}else{
Message msg=new Message();
msg.what=ERROR;
handler.sendMessage(msg);
}
} catch (Exception e) {
Message msg=new Message();
msg.what=LODE;
handler.sendMessage(msg);
e.printStackTrace();
}
}
}.start();
}
}
}
四,乱码问题:
*************
五,SyncHttpClient 框架访问服务器端
package cn.guoqing.asynchttpLogin;
import java.net.URLEncoder;
import android.app.Activity;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.loopj.android.http.AsyncHttpClient;
import com.loopj.android.http.AsyncHttpResponseHandler;
import com.loopj.android.http.RequestParams;
@SuppressWarnings("all")
public class MainActivity extends Activity {
private EditText etusername=null;
private EditText etpassword=null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
etusername=(EditText) findViewById(R.id.et_username);
etpassword=(EditText) findViewById(R.id.et_password);
}
//GET方法登陆
public void getlogin(View view){
String username = etusername.getText().toString().trim();
String password = etpassword.getText().toString().trim();
if(TextUtils.isEmpty(username)&&TextUtils.isEmpty(password)){
Toast.makeText(this, "用户名或密码不能为空!", 0).show();
}
else{
try {
//1,获取url
String url="http://192.168.1.23:8080/LoginDemo/servlet/LoginDemo1?username="+URLEncoder.encode(username)+"&password="+URLEncoder.encode(password);
//2,获取浏览器
AsyncHttpClient client = new AsyncHttpClient();
//向浏览器中输入资源地址
client.get(url, new AsyncHttpResponseHandler(){//AsyncHttpResponseHandler返回结果处理器
@Override
public void onSuccess(String content) {
super.onSuccess(content);
Toast.makeText(getApplicationContext(), content, 0).show();
}
//content为 连成功后服务器端返回的数据
});
} catch (Exception e) {
Toast.makeText(getApplicationContext(), "链接服务器失败", 0).show();
e.printStackTrace();
}
}
}
//POST方法登陆
public void postlogin(View view){
String username = etusername.getText().toString().trim();
String password = etpassword.getText().toString().trim();
if(TextUtils.isEmpty(username)&&TextUtils.isEmpty(password)){
Toast.makeText(this, "用户名或密码不能为空!", 0).show();
}
else{
try {
//1,获取url
String url="http://192.168.1.23:8080/LoginDemo/servlet/LoginDemo1";
//2,获取浏览器
AsyncHttpClient client = new AsyncHttpClient();
//向浏览器中输入资源地址并经请求体中的数据进行封装
RequestParams params = new RequestParams();
params.put("username", username);
params.put("password", password);
client.post(url, params, new AsyncHttpResponseHandler(){
@Override
public void onSuccess(String content) {
super.onSuccess(content);
Toast.makeText(getApplicationContext(), content, 0).show();
}
});
} catch (Exception e) {
Toast.makeText(getApplicationContext(), "链接服务器失败", 0).show();
e.printStackTrace();
}
}
}
}
六,SmartImageView获取服务器端的图片,并在指定的SmartImageView视图中显示
注意:在layOut中的视图标签中要用smartImageView 代替imageView
<com.loopj.android.image.SmartImageView
android:id="@+id/iv_image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="20" />
代码:
package cn.guoqing.smartImageView;
import android.app.Activity;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.loopj.android.image.SmartImageView;
public class MainActivity extends Activity {
private SmartImageView siv=null;
private EditText edurl=null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
siv=(SmartImageView) findViewById(R.id.iv_image);
edurl=(EditText) findViewById(R.id.et_url);
}
public void click(View view){
String url=edurl.getText().toString().trim();
if(TextUtils.isEmpty(url)){
Toast.makeText(getApplicationContext(), "路径不能为空!", 1).show();
}
}
}
}
1,联系人数据在contact2数据库中的存储方式:
(图contact)
2,实现获取联系人数据的代码
package cn.guoqing.readContacts.service;
import java.util.ArrayList;
import java.util.List;
import cn.guoqing.readContacts.domain.Contact;
import android.content.ContentResolver;
import android.content.Context;
import android.database.Cursor;
import android.net.Uri;
/**
* 从系统的数据库中获取用户的记录信息
* @author YGQ
*
*/
public class ReadContactsService {
public static List<Contact> getContactInfos(Context context){
//1.获取内容解析者
ContentResolver resolver = context.getContentResolver();
//2,获取raw_contacts表的URI --->暗号
Uri uri_contact = Uri.parse("content://com.android.contacts/data");
Uri uri=Uri.parse("content://com.android.contacts/raw_contacts");
//3,查询raw_contacts表获取联系人的id
Cursor cursor = resolver.query(uri, new String[]{"contact_id"}, null, null, null);
// System.out.println(cursor.getCount());
//遍历结果集中的用户id
//用于存储联系人的集合
ArrayList<Contact> list=new ArrayList<Contact>();
while(cursor.moveToNext()){
String contact_id = cursor.getString(0);
//利用联系人的id查询data表,获取联系人的详细记录
Contact contact = new Contact();;
Cursor cursor2 = resolver.query(uri_contact, new String[]{"mimetype","data1"}, "raw_contact_id=?", new String[]{contact_id}, null);
// System.out.println(cursor2.getCount());
while(cursor2.moveToNext()){
String mimetype = cursor2.getString(0);
String data1 = cursor2.getString(1);
//判断mimetypq的类型,看是否为姓名,电话,email
if("vnd.android.cursor.item/phone_v2".equals(mimetype)){
contact.setPhone(data1);
}
if("vnd.android.cursor.item/email_v2".equals(mimetype)){
contact.setEmail(data1);
}
if("vnd.android.cursor.item/name".equals(mimetype)){
contact.setName(data1);
}
}
//将一个用户的记录填充到contact对象中
list.add(contact);
}
//返回所用的用户记录信息
return list;
}
}
二,从互联网上查看图片
package cn.guoqing.picnet;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends Activity {
protected static final int SUCCESS = 1;
protected static final int ERROR = 2;
protected static final int LOAD = 3;
ImageView ivimage=null;
EditText eturl=null;
@SuppressLint("HandlerLeak")
@SuppressWarnings("all")
private Handler handler =new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case SUCCESS:
Bitmap bitmap=(Bitmap) msg.obj;
ivimage.setImageBitmap(bitmap);
break;
case ERROR:
Toast.makeText(getApplicationContext(), "链接服务器获取资源错误", 0).show();
break;
case LOAD:
Toast.makeText(getApplicationContext(), "下载图片失败!", 0).show();
break;
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ivimage=(ImageView) findViewById(R.id.iv_image);
eturl=(EditText) findViewById(R.id.et_url);
}
public void click(View view){
final String text = eturl.getText().toString().trim();
if(TextUtils.isEmpty(text)){
Toast.makeText(getApplicationContext(), "资源路经不能为空", 0).show();
}
else{
new Thread(){
@Override
public void run() {
try {
URL url = new URL(text);
//System.out.println(url);
//1,获取链接资源的对象
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
//2,设置链接超时时间
connection.setConnectTimeout(2000);
//3,设置访问方式
connection.setRequestMethod("GET");
//connection.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 8.0; Windows NT 6.1; Trident/4.0; SLCC2; .NET CLR 2.0.50727; .NET CLR 3.5.30729; .NET CLR 3.0.30729; .NET4.0C; .NET4.0E)");
//获取访问时返回的状态码
int code = connection.getResponseCode();
if(code==200){
//获取服务端返回的资源流
InputStream is = connection.getInputStream();
/*//定义一个用于存储返回资源的输出流对象
ByteArrayOutputStream baos=new ByteArrayOutputStream();
//读取流中数据
int len=0;
byte[] buf=new byte[1024];
while((len=is.read(buf))!=-1){
baos.write(buf, 0, len);
}
baos.close();
is.close();*/
//将流进行封装
Bitmap bitmap = BitmapFactory.decodeStream(is);
//子线程不能更新view上的内容,需要将封装的流,以发送消息的方式发送给主线程
Message message = new Message();
//设置消息的内容,和状态码
message.obj=bitmap;
message.what=SUCCESS;
//通过hundler向主线程发送消息
handler.sendMessage(message);
}
else{
//Toast.makeText(getApplicationContext(), "没有正确的访问到资源", 0).show();
Message message = new Message();
message.what=ERROR;
handler.sendMessage(message);
}
} catch (Exception e) {
//Toast.makeText(getApplicationContext(), "获取资源错误", 0).show();
Message message = new Message();
message.what=LOAD;
handler.sendMessage(message);
e.printStackTrace();
}
}
}.start();
}
}
}
注意:应用程序无响应: ANR
需要添加一个访问网络的权限:android.permission.INTERNET
4.0以上的系统引入了一个新的异常:
android.os.NetworkOnMainThreadException
网络的操作不允许在主线程里面执行,
可能会消耗很长的时间,导致ANR异常的8.3. 获取XML产生,所以google在4.0以上的系统不允许
在主线程里面直接访问网络
08-17 02:54:17.154: W/System.err(1776): android.view.ViewRootImpl$CalledFromWrongThreadException: Only the original thread that created a view hierarchy can touch its views.
只有原始的线程创建的view对象 才可以操作他的view
只有UI线程 才可以修改界面 修改view对象里面的内容
在android操作系统里面有一个原则: 只有主线程(ui线程) 才可以修改view对象里面的内容.
子线程与主线程之间怎样实现消息的更新?
利用消息机制来实现子线程更新主线程
//当主线程中有任务式就调用handleMessage(Message msg)方法() 实时监控队列中是否有消息
public Looper getMainLooper() {
return null;
}
三,获取XML
package cn.guoqing.scanHtml;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import android.app.Activity;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
protected static final int SUCCESS = 1;
protected static final int ERROR = 2;
protected static final int LODE = 3;
TextView tvhtml=null;
EditText eturl=null;
Handler handler=new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case SUCCESS:
tvhtml.setText((String)msg.obj);
break;
case ERROR:
Toast.makeText(getApplicationContext(),"链接资源失败", 0).show();
break;
case LODE:
Toast.makeText(getApplicationContext(),"下载失败", 0).show();
break;
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvhtml=(TextView) findViewById(R.id.tv_html);
eturl=(EditText) findViewById(R.id.et_url);
}
public void click(View view){
final String path=eturl.getText().toString().trim();
if(TextUtils.isEmpty(path)){
Toast.makeText(this, "您输入的地址为空!", 0).show();
}
else{
new Thread(){
@Override
public void run() {
super.run();
try {
//获取资源的url
URL url = new URL(path);
//获取链接资源的connection
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(3000);
connection.setRequestProperty("User-Agent", "Mozilla/4.0 (compatible; MSIE 8.0; Windows NT 6.1; Trident/4.0; SLCC2; .NET CLR 2.0.50727; .NET CLR 3.5.30729; .NET CLR 3.0.30729; .NET4.0C; .NET4.0E)");
int code = connection.getResponseCode();
if(code==200){
//获取服务器返回的流
InputStream is = connection.getInputStream();
ByteArrayOutputStream baos=new ByteArrayOutputStream();
byte[] buf=new byte[1024];
int len=0;
while((len=is.read(buf))!=-1){
baos.write(buf, 0, len);
}
baos.close();
String cintenthtml = new String (baos.toByteArray(),"gbk");
Message msg=new Message();
msg.obj=cintenthtml;
msg.what=SUCCESS;
handler.sendMessage(msg);
}else{
Message msg=new Message();
msg.what=ERROR;
handler.sendMessage(msg);
}
} catch (Exception e) {
Message msg=new Message();
msg.what=LODE;
handler.sendMessage(msg);
e.printStackTrace();
}
}
}.start();
}
}
}
四,乱码问题:
*************
五,SyncHttpClient 框架访问服务器端
package cn.guoqing.asynchttpLogin;
import java.net.URLEncoder;
import android.app.Activity;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.loopj.android.http.AsyncHttpClient;
import com.loopj.android.http.AsyncHttpResponseHandler;
import com.loopj.android.http.RequestParams;
@SuppressWarnings("all")
public class MainActivity extends Activity {
private EditText etusername=null;
private EditText etpassword=null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
etusername=(EditText) findViewById(R.id.et_username);
etpassword=(EditText) findViewById(R.id.et_password);
}
//GET方法登陆
public void getlogin(View view){
String username = etusername.getText().toString().trim();
String password = etpassword.getText().toString().trim();
if(TextUtils.isEmpty(username)&&TextUtils.isEmpty(password)){
Toast.makeText(this, "用户名或密码不能为空!", 0).show();
}
else{
try {
//1,获取url
String url="http://192.168.1.23:8080/LoginDemo/servlet/LoginDemo1?username="+URLEncoder.encode(username)+"&password="+URLEncoder.encode(password);
//2,获取浏览器
AsyncHttpClient client = new AsyncHttpClient();
//向浏览器中输入资源地址
client.get(url, new AsyncHttpResponseHandler(){//AsyncHttpResponseHandler返回结果处理器
@Override
public void onSuccess(String content) {
super.onSuccess(content);
Toast.makeText(getApplicationContext(), content, 0).show();
}
//content为 连成功后服务器端返回的数据
});
} catch (Exception e) {
Toast.makeText(getApplicationContext(), "链接服务器失败", 0).show();
e.printStackTrace();
}
}
}
//POST方法登陆
public void postlogin(View view){
String username = etusername.getText().toString().trim();
String password = etpassword.getText().toString().trim();
if(TextUtils.isEmpty(username)&&TextUtils.isEmpty(password)){
Toast.makeText(this, "用户名或密码不能为空!", 0).show();
}
else{
try {
//1,获取url
String url="http://192.168.1.23:8080/LoginDemo/servlet/LoginDemo1";
//2,获取浏览器
AsyncHttpClient client = new AsyncHttpClient();
//向浏览器中输入资源地址并经请求体中的数据进行封装
RequestParams params = new RequestParams();
params.put("username", username);
params.put("password", password);
client.post(url, params, new AsyncHttpResponseHandler(){
@Override
public void onSuccess(String content) {
super.onSuccess(content);
Toast.makeText(getApplicationContext(), content, 0).show();
}
});
} catch (Exception e) {
Toast.makeText(getApplicationContext(), "链接服务器失败", 0).show();
e.printStackTrace();
}
}
}
}
六,SmartImageView获取服务器端的图片,并在指定的SmartImageView视图中显示
注意:在layOut中的视图标签中要用smartImageView 代替imageView
<com.loopj.android.image.SmartImageView
android:id="@+id/iv_image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="20" />
代码:
package cn.guoqing.smartImageView;
import android.app.Activity;
import android.os.Bundle;
import android.text.TextUtils;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.loopj.android.image.SmartImageView;
public class MainActivity extends Activity {
private SmartImageView siv=null;
private EditText edurl=null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
siv=(SmartImageView) findViewById(R.id.iv_image);
edurl=(EditText) findViewById(R.id.et_url);
}
public void click(View view){
String url=edurl.getText().toString().trim();
if(TextUtils.isEmpty(url)){
Toast.makeText(getApplicationContext(), "路径不能为空!", 1).show();
}
else{
//url,R.drawable.fail:下载失败时显示的图片呢;R.drawable.loading:下载时显示的图片
siv.setImageUrl(url,R.drawable.fail, R.drawable.loading);}
}
}