概述
- 如果在一个系统中对象之间的联系呈现为网状结构。对象之间存在大量的多对多联系,将导致系统非常复杂,这些对象既会影响别的对象,也会被别的对象所影响,这些对象称为同事对象。
- 中介者模式可以使对象之间的关系数量急剧减少,通过引入中介者对象,可以将系统的网状结构变成以中介者为中心的星形结构。在这个星形结构中,同事对象不再直接与另一个对象联系,它通过中介者对象与另一个对象发生相互作用。中介者对象的存在保证了对象结构上的稳定,也就是说,系统的结构不会因为新对象的引入带来大量的修改工作。
- 如果在一个系统中对象之间存在多对多的相互关系,我们可以将对象之间的一些交互行为从各个对象中分离出来,并集中封装在一个中介者对象中,并由该中介者进行统一协调,这样对象之间多对多的复杂关系就转化为相对简单的一对多关系。通过引入中介者来简化对象之间的复杂交互,中介者模式是“迪米特法则”的一个典型应用。
- 定义:用一个中介对象(中介者)来封装一系列的对象交互,中介者使各对象不需要显式地相互引用,从而使其耦合松散,而且可以独立地改变它们之间的交互。
- 中介者模式又称为调停者模式。
- 是一种对象行为型模式。
- 学习难度:★★★☆☆
- 使用频率:★★☆☆☆
优缺点
- 优点
- 中介者对象使各对象不用显式的相互作用,从而使其耦合松散,而且可以独立改变他们之间的交互
- 减少依赖,耦合松散
- 缺点
类图
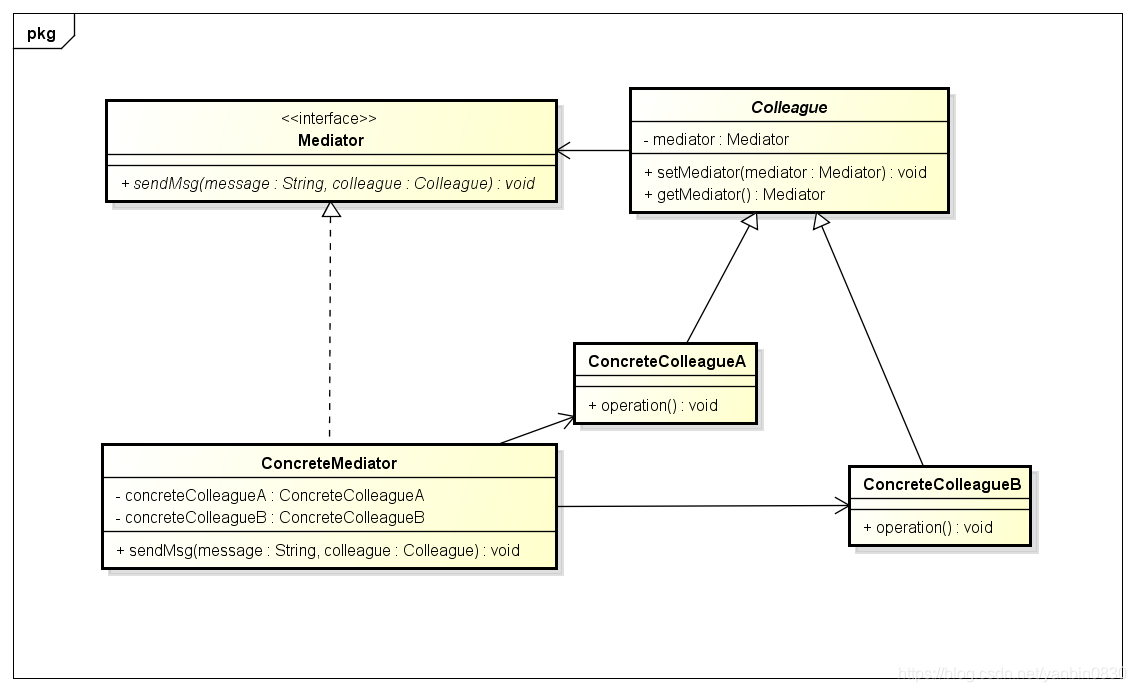
组成角色
- 抽象中介者角色(Mediator)
- 具体中介者角色(ConcreteMediator)
- 同事角色(Colleague)
- 具体同事角色(ConcreteColleague)
Code Example
抽象中介者角色(Mediator)
public interface Mediator {
public void sendMsg(String message, Colleague colleague);
}
具体中介者角色(ConcreteMediator)
public class ConcreteMediator implements Mediator {
private Colleague colleagueA;
private Colleague colleagueB;
public ConcreteMediator(Colleague colleagueA, Colleague colleagueB) {
this.colleagueA = colleagueA;
this.colleagueB = colleagueB;
}
@Override
public void sendMsg(String message, Colleague colleague) {
if (colleagueA == colleague) {
colleagueB.notify(message);
} else if (colleagueB == colleague) {
colleagueA.notify(message);
}
}
}
同事角色(Colleague)
- 每个同事角色都知道中介者角色
- 包含自有方法,只处理内部的逻辑的方法
- 依赖方法,依赖中介者处理逻辑的方法
public abstract class Colleague {
private Mediator mediator;
public Mediator getMediator() {
return mediator;
}
public void setMediator(Mediator mediator) {
this.mediator = mediator;
}
public abstract void sendMsg();
public abstract void notify(String message);
}
具体同事角色(ConcreteColleague)
public class ConcreteColleagueA extends Colleague {
public void sendMsg() {
getMediator().sendMsg("通知B,想揍他", this);
}
public void notify(String message) {
System.out.println("A接受到通知:" + message);
}
}
public class ConcreteColleagueB extends Colleague {
public void sendMsg() {
getMediator().sendMsg("通知A,有问题", this);
}
public void notify(String message) {
System.out.println("B接受到通知:" + message);
}
}
客户端
public class MediatorPattern {
public static void main(String[] args) {
Colleague colleagueA = new ConcreteColleagueA();
Colleague colleagueB = new ConcreteColleagueB();
Mediator mediator = new ConcreteMediator(colleagueA, colleagueB);
colleagueA.setMediator(mediator);
colleagueB.setMediator(mediator);
colleagueA.sendMsg();
colleagueB.sendMsg();
}
}