1-中断interrupt机制
1.1-interrupt介绍
首先,一个线程不应该由其他线程来强制中断或停止,而是应该由线程自己自行停止。所以,Thread.stop,Thread.suspend,Thread.resume都已经被废弃了。
其次在Java中没有办法立即停止一条线程,然而停止线程却显得尤为重要,如取消一个耗时操作。
因此,Java提供了一种用于停止线程的协商机制——中断。
中断只是一种协作协商机制,Java没有给中断增加任何语法,中断的过程完全需要程序员自己实现。若要中断一个线程,你需要手动调用该线程的interrupt方法,该方法也仅仅是将线程对象的中断标识设成true;接着你需要自己写代码不断地检测当前线程的标识位,如果为true,表示别的线程要求这条线程中断,此时究竟该做什么需要你自己写代码实现。
每个线程对象中都有一个标识,用于表示线程是否被中断;该标识位为true表示中断,为false表示未中断;通过调用线程对象的interrupt方法将该线程的标识位设为true;可以在别的线程中调用,也可以在自己的线程中调用。
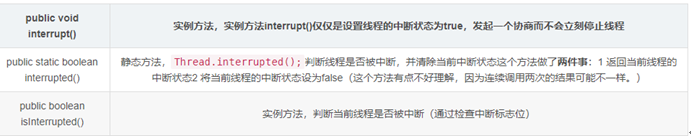
1.2-interrupt代码演示
@Slf4j
public class TestInterrupt2 {
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
for (int i = 0; i < 300; i++) {
log.info("---------" + i);
}
log.info("aftert1.interrupt()---第2次----" + Thread.currentThread().isInterrupted());
}, "t1");
t1.start();
log.info("beforet1.interrupt()----" + t1.isInterrupted());
t1.interrupt();
try {
TimeUnit.MILLISECONDS.sleep(5);
} catch (InterruptedException e) {
log.error("InterruptedException1={}",e);
}
log.info("aftert1.interrupt()---第1次---" + t1.isInterrupted());
try {
TimeUnit.MILLISECONDS.sleep(300);
} catch (InterruptedException e) {
log.error("InterruptedException2={}",e);
}
log.info("aftert1.interrupt()---第3次---" + t1.isInterrupted());
}
}
控制台输出:
15:22:08.083 [main] INFO com.ycmy2023.interrupt.TestInterrupt2 - beforet1.interrupt()----false
15:22:08.083 [t1] INFO com.ycmy2023.interrupt.TestInterrupt2 - ---------0
15:22:08.086 [t1] INFO com.ycmy2023.interrupt.TestInterrupt2 - ---------1
省略无用日志
15:22:08.092 [t1] INFO com.ycmy2023.interrupt.TestInterrupt2 - ---------250
15:22:08.094 [main] INFO com.ycmy2023.interrupt.TestInterrupt2 - aftert1.interrupt()---第1次---true
省略无用日志,发现t1线程继续运行,并没有停止;
15:22:08.095 [t1] INFO com.ycmy2023.interrupt.TestInterrupt2 - ---------251
15:22:08.096 [t1] INFO com.ycmy2023.interrupt.TestInterrupt2 - ---------299
15:22:08.096 [t1] INFO com.ycmy2023.interrupt.TestInterrupt2 - aftert1.interrupt()---第2次----true
15:22:08.409 [main] INFO com.ycmy2023.interrupt.TestInterrupt2 - aftert1.interrupt()---第3次---false//中断不活动的线程不会产生任何影响,线程结束后应该是自动变为了false
@Slf4j
public class TestInterrupt3 {
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
while (true) {
if (Thread.currentThread().isInterrupted()) {
log.info(Thread.currentThread().getName() + "中断标志位:" + Thread.currentThread().isInterrupted() + "程序终止");
break;
}
try {
TimeUnit.MILLISECONDS.sleep(200);
} catch (InterruptedException e) {
log.error("InterruptedException,e={}",e);
//Thread.currentThread().interrupt();//如果注释掉这个代码,线程t1不会终止,假如加了这个,程序可以终止
}
log.info("-----TestInterrupt3");
}
}, "t1");
t1.start();
try {
TimeUnit.MILLISECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
Thread t2 = new Thread(() -> {
t1.interrupt();
}, "t2");
t2.start();
}
}
控制台输出:程序不会停止
15:36:50.703 [t1] ERROR com.ycmy2023.interrupt.TestInterrupt3 - InterruptedException,e={}
java.lang.InterruptedException: sleep interrupted
at java.lang.Thread.sleep(Native Method)
at java.lang.Thread.sleep(Thread.java:340)
at java.util.concurrent.TimeUnit.sleep(TimeUnit.java:386)
at com.ycmy2023.interrupt.TestInterrupt3.lambda$main$0(TestInterrupt3.java:17)
at java.lang.Thread.run(Thread.java:748)
15:36:50.706 [t1] INFO com.ycmy2023.interrupt.TestInterrupt3 - -----TestInterrupt3
15:36:50.913 [t1] INFO com.ycmy2023.interrupt.TestInterrupt3 - -----TestInterrupt3
15:36:51.121 [t1] INFO com.ycmy2023.interrupt.TestInterrupt3 - -----TestInterrupt3
15:36:51.327 [t1] INFO com.ycmy2023.interrupt.TestInterrupt3 - -----TestInterrupt3
//Thread.currentThread().interrupt(); 这个注释去掉:
控制台输出:程序会停止
15:39:41.983 [t1] ERROR com.ycmy2023.interrupt.TestInterrupt3 - InterruptedException,e={}
java.lang.InterruptedException: sleep interrupted
at java.lang.Thread.sleep(Native Method)
at java.lang.Thread.sleep(Thread.java:340)
at java.util.concurrent.TimeUnit.sleep(TimeUnit.java:386)
at com.ycmy2023.interrupt.TestInterrupt3.lambda$main$0(TestInterrupt3.java:17)
at java.lang.Thread.run(Thread.java:748)
15:39:41.986 [t1] INFO com.ycmy2023.interrupt.TestInterrupt3 - -----TestInterrupt3
15:39:41.986 [t1] INFO com.ycmy2023.interrupt.TestInterrupt3 - t1中断标志位:true程序终止
t1中断标志位默认是false
t2----->t1发出了中断协商,t2调用t1.interrupt(),中断标志位true
中断标志位true,正常情况下,程序停止
中断标志位true,异常情况下,InterruptedException,将会把中断状态清除,并且将收到InterruptedException。中断标志位false导致无限循环。
在catch块中,需要再次给中断标志位设置为true,调用停止
1.3-interrupt总结
具体来说,当对一个线程,调用interrupt()时:
(1)如果线程处于正常活动状态,那么会将该线程的中断标志设置为true,仅此而已。被设置中断标志的线程将继续正常运行,不受影响。所以,interrupt()并不能真正的中断线程,需要被调用的线程自己进行配合才行 比如https://blog.csdn.net/ycmy2017/article/details/129078824?spm=1001.2014.3001.5501 中的两阶段模式。
(2)如果线程处于被阻塞状态(例如处于sleep,wait,join等状态),在别的线程中调用当前线程对象的interrupt方法,那么线程将立即退出被阻塞状态(中断状态将被清除),并抛出一个InterruptedException异常。
2-LockSupport
2.1-LockSupport介绍
Basic thread blocking primitives for creating locks and other synchronization classes.
官方解释:用于创建锁和其他同步类的基本线程阻塞原语。
核心就是park()和unpark()方法
park()方法是阻塞线程;
unpark()方法是解除阻塞线程
2.2-LockSupport代码演示
@Slf4j
public class TestLockSupport {
public static void main(String[]args){
Thread t1=new Thread(()->{
log.info(Thread.currentThread().getName()+"---come in");
LockSupport.park();
log.info(Thread.currentThread().getName()+"---被唤醒了");
},"t1");
t1.start();
new Thread(()->{
LockSupport.unpark(t1);
log.info(Thread.currentThread().getName()+"---发出通知,去唤醒t1");
},"t2").start();
}
}
控制台输出:
15:50:53.434 [t1] INFO com.ycmy2023.lockSupport.TestLockSupport - t1---come in
15:50:53.434 [t2] INFO com.ycmy2023.lockSupport.TestLockSupport - t2---发出通知,去唤醒t1
15:50:53.437 [t1] INFO com.ycmy2023.lockSupport.TestLockSupport - t1---被唤醒了
通过park()和unpark(thread)方法来实现阻塞和唤醒线程的操作;
LockSupport是用来创建锁和其他同步类的基本线程阻塞原语。
LockSupport类使用了一种名为Permit(许可)的概念来做到阻塞和唤醒线程的功能,每个线程都有一个许可(permit),
permit(许可)只有两个值1和0,默认是0。0是阻塞,1是唤醒
可以把许可看成是一种(0,1)信号量(Semaphore),但与Semaphore不同的是,许可的累加上限是1。
2.3-LockSupport总结
LockSupport是用来创建锁和其他同步类的基本线程阻塞原语。
LockSupport是一个线程阻塞工具类,所有的方法都是静态方法,可以让线程在任意位置阻塞,阻塞之后也有对应的唤醒方法。归根结底,LockSupport调用的Unsafe中的native代码。
LockSupport提供park()和unpark()方法实现阻塞线程和解除线程阻塞的过程
LockSupport和每个使用它的线程都有一个许可(permit)关联。
每个线程都有一个相关的permit,permit最多只有一个,重复调用unpark也不会积累凭证。
形象的理解
线程阻塞需要消耗凭证(permit),这个凭证最多只有1个。
当调用方法时
*如果有凭证,则会直接消耗掉这个凭证然后正常退出;
*如果无凭证,就必须阻塞等待凭证可用;