第一题
#include<iostream>
#include<cctype>
int main()
{
using namespace std;
char choice;
while ((choice = cin.get()) != '@')
{
if (islower(choice))
choice = toupper(choice);
else if (isupper(choice))
choice = tolower(choice);
if (!isdigit(choice))
cout << choice;
}
return 0;
}
第二题
#include<iostream>
#include<array>
const int SIZE = 10;
int main()
{
using namespace std;
array<double, SIZE>arr_dou;
unsigned i,count,count_dou;
double sum;
i = count = count_dou = 0;
sum = 0.0;
while (i < SIZE && cin >> arr_dou[i])
{
count++;
sum += arr_dou[i++];
}
sum /= count;
for (i = 0; i < SIZE; i++)
{
if (arr_dou[i] > sum)
count_dou++;
}
cout << fixed;// 使用一般方式输出浮点数,避免系统使用E表示法
cout.precision(2);//浮点数最多显示2位
cout.setf(ios_base::showpoint);//显示小数点和额外的零,即使不需要.
cout << "average value: " << sum
<< " A total of " << count_dou
<< " numbers are greater than the average.";
return 0;
}
第三题
#include<iostream>
void Menu();
//using namespace std;
int main()
{
using namespace std;
char choice;
Menu();
while (cin.get(choice))
{
switch (choice)
{
case 'c':
std::cout << "carnivore\n";
break;
case 'p':
std::cout << "pianist\n";
break;
case 't':
std::cout << "tree\n";//这里进行的输出没必要和书上的一样 书上说的是简单执行一个操作
break;
case 'g':
std::cout << "game\n";
break;
default:
cout << "Please enter a c, p, t, or g: ";
while ((choice = cin.get()) != '\n');//也可以这样使用
continue;
}
while ((choice = cin.get()) != '\n')//比如我们输入taa我们只对应第一个字符对多余字符进行丢弃操作 别忘了后面还有一个换行符
continue;
Menu();
}
return 0;
}
void Menu()
{
std::cout << "Please enter one of the following choices:\n";
std::cout << "c) carnivore p) pianist\n"
<< "t) tree g) game\n";
}
第四题
#include<iostream>
using namespace std;
const int strsize = 80;
const int SIZE{4};
void Menu();
struct bop
{
char fullname[strsize];
char title[strsize];
char bopname[strsize];
int preference;
};
int main()
{
char choice;
bop bop_name[SIZE]
{
{"Davis","Registered Architect","Harper",1},
{"Miller", "Builders", "Sylvia", 0},
{"Smith","veterinarian","Ashley",2},
{"Garcia","teacher","Astrid",0 }
};
cout << "Benevolent Order of Programmers Report\n";
Menu();
cout << "Enter your choice: ";
while ((choice = cin.get()) != 'q')
{
switch (choice)
{
case 'a':
cout << "Wimp Macho\n";//也可以放在switch外面
for (unsigned i = 0; i < SIZE; i++)
{
cout << bop_name[i].fullname << endl;
}
break;
case 'b':
cout << "Wimp Macho\n";
for (unsigned i = 0; i < SIZE; i++)
{
cout << bop_name[i].title << endl;
}
break;
case 'c':
cout << "Wimp Macho\n";
for (unsigned i = 0; i < SIZE; i++)
{
cout << bop_name[i].bopname << endl;
}
break;
case 'd':
cout << "Wimp Macho\n";
for (unsigned i = 0; i < SIZE; i++)
{
if (bop_name[i].preference == 0)
cout << bop_name[i].fullname << endl;
else if (bop_name[i].preference == 1)
cout << bop_name[i].title << endl;
else
cout << bop_name[i].bopname << endl;
}
break;
default:
cout << "Please enter a a, b, c, d (q is quit): ";
while ((choice = cin.get()) != '\n');//丢弃字符
//没有大括号while后面有分号请注意
continue;
}
while ((choice = cin.get()) != '\n');//丢弃字符
cout << "Next choice: ";
}
cout << "Bye!";
return 0;
}
void Menu()
{
cout << "a.display by name b.display by title\n"
<< "c.display by bopname d.display by preference\n"
<< "q.quit\n";
}
第五题
#include<iostream>
const double ZERO_DOU = 0.00;
const double tvarps_d = 0.10;
const double tvarps_o = 0.15;
const double tvarps_u = 0.20;
const int tvarps_i{ 10000 };
const int tvarps_n{ 20000 };
const int tvarps_t{ 35000 };
int main()
{
double tvarps;
double tvarps_dou;
while (std::cin >> tvarps && tvarps >= 0)
{
if (tvarps < 5001)
tvarps_dou = 0;
else if (tvarps >= 5001 && tvarps <= 15000)
tvarps_dou = (tvarps - 5000) * tvarps_d;
else if (tvarps >= 15001 && tvarps <= 35000)
tvarps_dou = tvarps_i * tvarps_o + (tvarps - 15000) * tvarps_o;
else
tvarps_dou = tvarps_i * tvarps_d + tvarps_n * tvarps_o + (tvarps - 35000) * tvarps_u;
std::cout << tvarps_dou << std::endl;
}
return 0;
}
第六题
#include<iostream>
#include<string>
struct organ
{
std::string name;
double amount;
};
const int SIZE{ 3 };
int main()
{
using namespace std;
organ str_name[SIZE];
int i, count_s;
i = count_s = 0;
while (i < SIZE)
{
cout << "Please enter the name of the donor: ";
getline(cin, str_name[i].name);
cout << "Please enter the donation amount: ";
cin >> str_name[i].amount;
str_name[i].amount > 9999 ? count_s++ : count_s;
i++;
cin.get();
}
cout << "\nGrand Patrons\n";
if (count_s != 0)
{
for (i = 0; i < SIZE; i++)
if(str_name[i].amount > 9999)
cout << str_name[i].name << endl
<< str_name[i].amount << endl;
}
else
cout << "none\n";
cout.put('\n');
cout << "Patrons\n";
if (count_s == 0 || count_s != SIZE)
{
for (i = 0; i < SIZE; i++)
if (str_name[i].amount < 10000)
cout << str_name[i].name << endl
<< str_name[i].amount << endl;
}
else
cout << "none\n";
return 0;
}
第七题
#include<iostream>
#include<cctype>
int const SIZE{ 80 };
int main()
{
using namespace std;
char str[SIZE];
int count_y, count_f;
count_y = count_f = 0;
while (cin >> str)
{
if (str[1] == '\0' && str[0] == 'q')
break;
/*if (isalpha(str[0]))
{
if (str[0] == 'a' || str[0] == 'A')
count_y++;
else if (str[0] == 'e' || str[0] == 'E')
count_y++;
else if (str[0] == 'u' || str[0] == 'U')
count_y++;
else if (str[0] == 'i' || str[0] == 'I')
count_y++;
else if (str[0] == 'o' || str[0] == 'O')
count_y++;
else
{
count_f++;
continue;
}
continue;
}*/
//可以自己选择一个测试
if (isalpha(str[0]))
{
switch (str[0])
{
case 'a':
case 'e':
case 'o':
case 'i':
case 'u':
case 'A':
case 'E':
case 'O':
case 'I':
case 'U':count_y++; break;
default:count_f++; break;
}
}
}
cout << count_y << " words beginning with vowels\n"
<< count_f << " words beginning with consonants\n";
return 0;
}
第八题
跟着图片进行创建文本
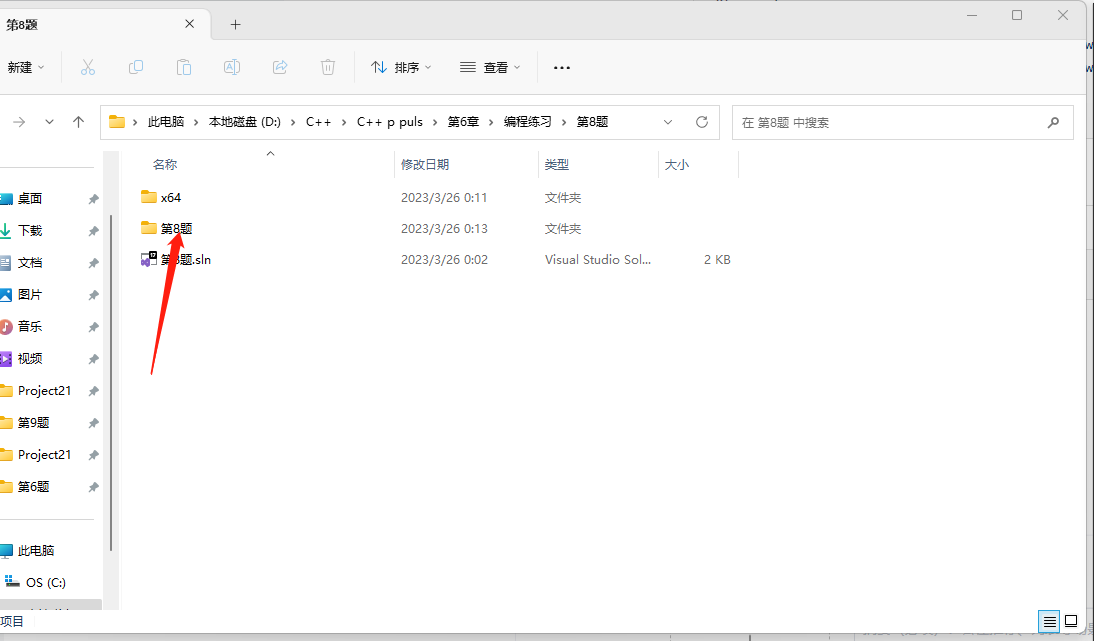
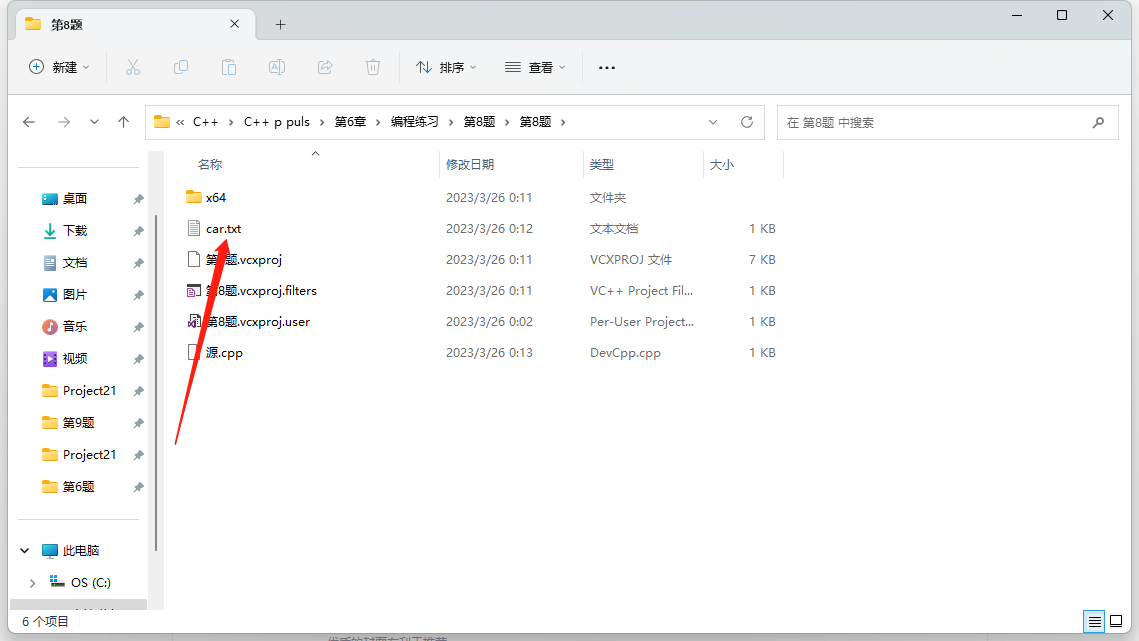
#include<iostream>
#include<fstream>
#include<cstdlib>
int main()
{
using namespace std;
ifstream ifFile;
char choice;
int count = 0;
ifFile.open("car.txt");//和文件建立联系
if (!ifFile.is_open())//查看是否打开文件
{
exit(1);
}
ifFile.get(choice);
while (ifFile.good())
{
++count;//包含了换行符 if(choice != '\n')不读取换行符就使用这个条件 加上换行符就多个字符
ifFile.get(choice);
}
if (ifFile.eof())//查看是否读到文件结尾
cout << "End of file reached.\n";
else if (ifFile.fail())//是否读取出错
cout << "error";
cout << count;
return 0;
}
第九题
跟着第八题步骤创建文本 下面图片为文本内容
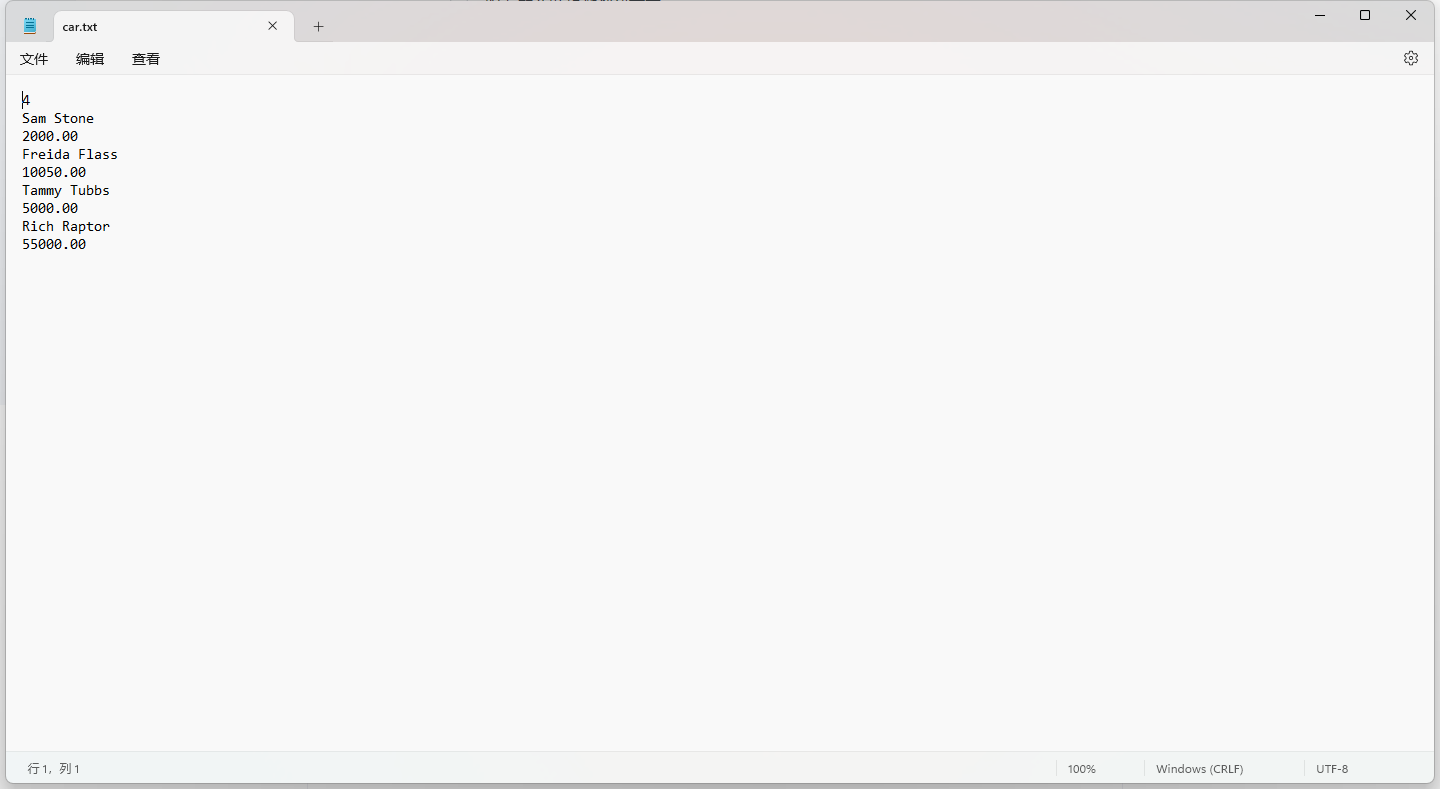
#include<iostream>
#include<fstream>
#include<cstdlib>
#include<string>
struct organ
{
std::string name;
double amount;
};
int main()
{
using namespace std;
ifstream inFile;
int temp;
organ str_name[4];
int i = 0;
inFile.open("car.txt");
if (!inFile.is_open())//查看文件是否打开
{
exit(1);
}
inFile >> temp;
inFile.get();
while (inFile.good() && i < 4)
{
getline(inFile, str_name[i].name);
inFile >> str_name[i++].amount;
inFile.get();//丢弃换行符
}
cout << temp << endl;;
cout << fixed;
cout.precision(2);
cout.setf(ios_base::showpoint);
for (i = 0; i < 4; i++)
{
cout << str_name[i].name << endl
<< str_name[i].amount << endl;
}
return 0;
}