/*
---------------------------------
1.创建过程
2.操作过程
3.显示过程
---------------------------------
创建链表 (创建表头)
创建节点
插入节点 (表头,表尾,指定位置)
判断链表是否为空
删除节点 (表头,表尾,指定位置)
打印链表
----------------------------------
*/
#include<iostream>
using namespace std;
typedef struct SingleList
{
//数据域
int data;
//指针域
struct SingleList* next;
}SINGLELIST,*LPSINGLELIST;
/*
-------------------------------------------------
功能独立----函数功能划分明确
-------------------------------------------------
*/
//创建表
LPSINGLELIST CreateList()
{
//创建过程----初始化基本数据成员
// 申请内存
LPSINGLELIST List = new SINGLELIST;
// 初始化基本数据成员
List->next = nullptr; //表头不需要初始化数据域
return List;
}
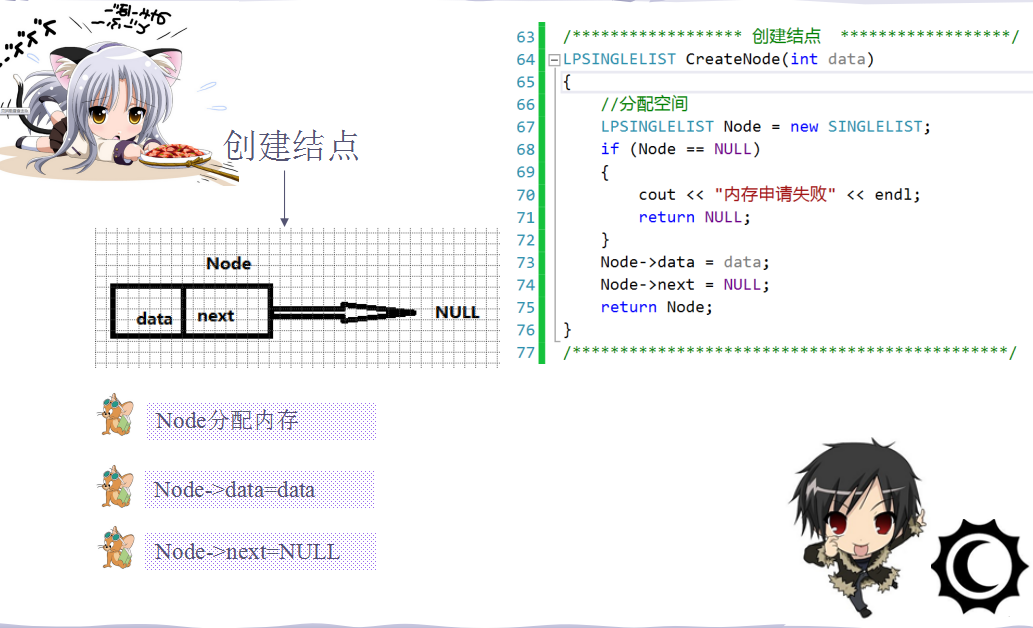
//创建节点
LPSINGLELIST CreateNode(int data)
{
// 申请内存
LPSINGLELIST Node = new SINGLELIST;
// 初始化基本数据成员
Node->data = data;
Node->next = nullptr; //表头不需要初始化数据域
return Node;
}
//判断为空---和创建过程相比较
bool IsEmptyList(LPSINGLELIST List)
{
if (List->next == nullptr)
return 1;
return 0;
}
void InsertHeadNode(LPSINGLELIST List, int data)
{
//插入操作 ---创建插入的结点
LPSINGLELIST newNode = CreateNode(data);
//先连后断
newNode->next = List->next;
List->next = newNode;
}
void InsertTailNode(LPSINGLELIST List, int data)
{
LPSINGLELIST tailNode = List;
while (tailNode->next != NULL)
{
tailNode = tailNode->next;
}
LPSINGLELIST newNode = CreateNode(data);
tailNode->next = newNode;
}
void InsertAppoinNode(LPSINGLELIST List, int data, int item)
{
LPSINGLELIST frontNode = List;
LPSINGLELIST tailNode = List->next;
while (tailNode->data != item)
{
frontNode = tailNode;
tailNode = frontNode->next;
if (tailNode== nullptr)
{
cout << "为找指定位置" << endl;
return;
}
}
LPSINGLELIST newNode = CreateNode(data);
frontNode->next = newNode;
newNode->next = tailNode;
}
void DeleteHeadNode(LPSINGLELIST List)
{
if (IsEmptyList(List))
{
cout << "链表为空无法删除" << endl;
return;
}
LPSINGLELIST headNode = List->next;
List->next = headNode->next;
delete headNode;
}
void DeleteAppoinNode(LPSINGLELIST List, int item)
{
LPSINGLELIST frontNode = List;
LPSINGLELIST tailNode = List->next;
while (tailNode->data != item)
{
frontNode = tailNode;
tailNode = frontNode->next;
if (tailNode == nullptr)
{
cout << "为找指定位置" << endl;
return;
}
}
frontNode->next = tailNode->next;
delete tailNode;
}
void DeleteTailNode(LPSINGLELIST List)
{
//找到表尾
if (IsEmptyList(List))
{
cout << "链表为空无法删除" << endl;
return;
}
LPSINGLELIST frontNode = List;
LPSINGLELIST tailNode = List->next;
while (tailNode->next != nullptr)
{
frontNode = tailNode;
tailNode = frontNode->next;
}
frontNode->next = nullptr;
delete tailNode;
}
void PrintList(LPSINGLELIST List)
{
LPSINGLELIST nextNode = List->next;
while (nextNode)
{
cout << nextNode->data<<" ";
nextNode = nextNode->next;
}
}
int main()
{
LPSINGLELIST List = CreateList(); //创建链表
cout << "InsertHeadNode:" << endl;
InsertHeadNode(List, 1);
InsertHeadNode(List, 2);
InsertHeadNode(List, 3);
PrintList(List);
cout <<endl <<"InsertTailNode:" << endl;
InsertTailNode(List, 5);
InsertTailNode(List, 6);
PrintList(List);
cout << endl << "InsertAppoinNode:" << endl;
InsertAppoinNode(List, 4, 5);
PrintList(List);
cout << endl << "DeleteHeadNode:" << endl;
DeleteHeadNode(List);
PrintList(List);
cout << endl << "DeleteTailNode:" << endl;
DeleteTailNode(List);
PrintList(List);
cout << endl << "DeleteAppoinNode:" << endl;
DeleteAppoinNode(List, 4);
PrintList(List);
system("pause");
return 0;
}
完整版本:
/*
---------------------------------
1.创建过程
2.操作过程
3.显示过程
---------------------------------
创建链表 (创建表头)
创建节点
插入节点 (表头,表尾,指定位置)
判断链表是否为空
删除节点 (表头,表尾,指定位置)
打印链表
----------------------------------
*/
#include<iostream>
using namespace std;
typedef struct SingleList
{
//数据域
int data;
//指针域
struct SingleList* next;
}SINGLELIST,*LPSINGLELIST;
/*
定义别的名
SINGLIST ------struct SingleList
LPSINGLELIST ----struct SingleList*
*/
/*
-------------------------------------------------
功能独立----函数功能划分明确
-------------------------------------------------
*/
LPSINGLELIST CreateList()
{
//创建过程----初始化基本数据成员
// 申请内存
LPSINGLELIST List = new SINGLELIST;
// 初始化基本数据成员
List->next = nullptr; //表头不需要初始化数据域
return List;
}
LPSINGLELIST CreateNode(int data)
{
// 申请内存
LPSINGLELIST Node = new SINGLELIST;
// 初始化基本数据成员
Node->data = data;
Node->next = nullptr; //表头不需要初始化数据域
return Node;
}
//判断为空---和创建过程相比较
bool IsEmptyList(LPSINGLELIST List)
{
if (List->next == nullptr)
return 1;
return 0;
}
void InsertHeadNode(LPSINGLELIST List, int data)
{
//插入操作 ---创建插入的结点
LPSINGLELIST newNode = CreateNode(data);
//先连后断
newNode->next = List->next;
List->next = newNode;
}
void InsertTailNode(LPSINGLELIST List, int data)
{
LPSINGLELIST tailNode = List;
while (tailNode->next != NULL)
{
tailNode = tailNode->next;
}
LPSINGLELIST newNode = CreateNode(data);
tailNode->next = newNode;
}
void InsertAppoinNode(LPSINGLELIST List, int data, int item)
{
LPSINGLELIST frontNode = List;
LPSINGLELIST tailNode = List->next;
while (tailNode->data != item)
{
frontNode = tailNode;
tailNode = frontNode->next;
if (tailNode== nullptr)
{
cout << "为找指定位置" << endl;
return;
}
}
LPSINGLELIST newNode = CreateNode(data);
frontNode->next = newNode;
newNode->next = tailNode;
}
void PrintList(LPSINGLELIST List)
{
LPSINGLELIST nextNode = List->next;
while (nextNode)
{
cout << nextNode->data<<" ";
nextNode = nextNode->next;
}
}
void DeleteHeadNode(LPSINGLELIST List)
{
if (IsEmptyList(List))
{
cout << "链表为空无法删除" << endl;
return;
}
LPSINGLELIST headNode = List->next;
List->next = headNode->next;
delete headNode;
}
void DeleteTailNode(LPSINGLELIST List)
{
//找到表尾
if (IsEmptyList(List))
{
cout << "链表为空无法删除" << endl;
return;
}
LPSINGLELIST frontNode = List;
LPSINGLELIST tailNode = List->next;
while (tailNode->next != nullptr)
{
frontNode = tailNode;
tailNode = frontNode->next;
}
frontNode->next = nullptr;
delete tailNode;
}
void DeleteAppoinNode(LPSINGLELIST List, int item)
{
LPSINGLELIST frontNode = List;
LPSINGLELIST tailNode = List->next;
while (tailNode->data != item)
{
frontNode = tailNode;
tailNode = frontNode->next;
if (tailNode == nullptr)
{
cout << "为找指定位置" << endl;
return;
}
}
frontNode->next = tailNode->next;
delete tailNode;
}
int main()
{
LPSINGLELIST List = CreateList(); //创建链表
cout << "InsertHeadNode:" << endl;
InsertHeadNode(List, 1);
InsertHeadNode(List, 2);
InsertHeadNode(List, 3);
PrintList(List);
cout <<endl <<"InsertTailNode:" << endl;
InsertTailNode(List, 5);
InsertTailNode(List, 6);
PrintList(List);
cout << endl << "InsertAppoinNode:" << endl;
InsertAppoinNode(List, 4, 5);
PrintList(List);
cout << endl << "DeleteHeadNode:" << endl;
DeleteHeadNode(List);
PrintList(List);
cout << endl << "DeleteTailNode:" << endl;
DeleteTailNode(List);
PrintList(List);
cout << endl << "DeleteAppoinNode:" << endl;
DeleteAppoinNode(List, 4);
PrintList(List);
system("pause");
return 0;
}