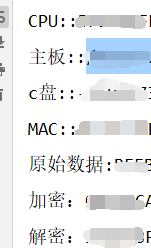
package com.RuoYi.RuoYiPojo;
import java.applet.Applet;
import java.awt.*;
import java.awt.Graphics;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileWriter;
import java.io.InputStreamReader;
import java.net.InetAddress;
import java.net.NetworkInterface;
public class HardWareUtils extends Applet {
public HardWareUtils() throws HeadlessException {
}
private static final long serialVersionUID = 1L;
@Override
public void paint(Graphics paint) {
super.paint(paint);
paint.drawString("获取硬件信息", 10, 10);
paint.drawString("CPU SN:" + HardWareUtils.getCPUSerial(), 10, 30);
paint.drawString("主板 SN:" + HardWareUtils.getMotherboardSN(), 10, 50);
paint.drawString("C盘 SN:" + HardWareUtils.getHardDiskSN("c"), 10, 70);
paint.drawString("MAC SN:" + HardWareUtils.getMac(), 10, 90);
}
/**
* 获取主板序列号30
* @return
* <p>
*/
public static String getMotherboardSN() {
String result = "";
try {
File file = File.createTempFile("realhowto", ".vbs");
file.deleteOnExit();
FileWriter fw = new java.io.FileWriter(file);
String vbs = "Set objWMIService = GetObject(\"winmgmts:\\\\.\\root\\cimv2\")\n"
+ "Set colItems = objWMIService.ExecQuery _ \n"
+ " (\"Select * from Win32_BaseBoard\") \n"
+ "For Each objItem in colItems \n"
+ " Wscript.Echo objItem.SerialNumber \n"
+ " exit for ' do the first cpu only! \n" + "Next \n";
fw.write(vbs);
fw.close();
Process p = Runtime.getRuntime().exec("cscript //NoLogo " + file.getPath());
BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
while ((line = input.readLine()) != null) {
result += line;
}
input.close();
} catch (Exception e) {
e.printStackTrace();
}
return result.trim();
}
/**
* 获取硬盘序列号
*@paramdrive
* 盘符
*@return
* <p>
*/
public static String getHardDiskSN(String drive) {
String result = "";
try {
File file = File.createTempFile("realhowto", ".vbs");
file.deleteOnExit();
FileWriter fw = new java.io.FileWriter(file);
String vbs = "Set objFSO = CreateObject(\"Scripting.FileSystemObject\")\n"
+ "Set colDrives = objFSO.Drives\n"
+ "Set objDrive = colDrives.item(\""
+ drive + "\")\n"
+ "Wscript.Echo objDrive.SerialNumber"; //see note
fw.write(vbs);
fw.close();
Process p = Runtime.getRuntime().exec("cscript //NoLogo " + file.getPath());
BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
while ((line = input.readLine()) != null) {
result += line;
}
input.close();
} catch (Exception e) {
e.printStackTrace();
}
return result.trim();
}
/**
* 101 * 获取CPU序列号102 *103 *@return
* <p>
* 104
*/
public static String getCPUSerial() {
String result = "";
try {
File file = File.createTempFile("tmp", ".vbs");
file.deleteOnExit();
FileWriter fw = new java.io.FileWriter(file);
String vbs = "Set objWMIService = GetObject(\"winmgmts:\\\\.\\root\\cimv2\")\n"
+ "Set colItems = objWMIService.ExecQuery _ \n"
+ " (\"Select * from Win32_Processor\") \n"
+ "For Each objItem in colItems \n"
+ " Wscript.Echo objItem.ProcessorId \n"
+ " exit for ' do the first cpu only! \n" + "Next \n";
fw.write(vbs);
fw.close();
Process p = Runtime.getRuntime().exec(
"cscript //NoLogo " + file.getPath());
BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
while ((line = input.readLine()) != null) {
result += line;
}
input.close();
file.delete();
} catch (Exception e) {
e.fillInStackTrace();
}
if (result.trim().length() < 1 || result == null) {
result = "无CPU_ID被读取";
}
return result.trim();
}
/**
* 139 * 获取MAC地址
*/
public static String getMac() {
try {
byte[] mac = NetworkInterface.getByInetAddress(InetAddress.getLocalHost()).getHardwareAddress();
StringBuffer sb = new StringBuffer();
for (int i = 0; i < mac.length; i++) {
if (i != 0) {
sb.append("-");
}
String s = Integer.toHexString(mac[i] & 0xFF);
sb.append(s.length() == 1 ? 0 + s : s);
}
return sb.toString().toUpperCase();
} catch (Exception e) {
return "";
}
}
public static void main(String[] args) throws Exception {
System.out.println("CPU::" + getCPUSerial());//CPU
System.out.println("主板::" + getMotherboardSN());//主板
System.out.println("c盘::" + getHardDiskSN("c"));//c盘
System.out.println("MAC::" + getMac());//MAC
String msg = getCPUSerial()
+ getMotherboardSN().replace(".", "")
+ getHardDiskSN("c")
+ getMac().replace("-", "");
System.out.println("原始数据:" + msg);
String encode = DesUtils.encode(msg);
System.out.println("加密:" + encode);
String decode = DesUtils.decode(encode);
System.out.println("解密:" + decode);
}
}
package com.RuoYi.RuoYiPojo;
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.DESKeySpec;
import java.security.SecureRandom;
public class DesUtils {
private static final String DES = "DES";
private static final String KEY = "4YztMHI7PsT4rLZN";
private DesUtils() {}
private static byte[] encrypt(byte[] src, byte[] key) throws Exception {
SecureRandom sr = new SecureRandom();
DESKeySpec dks = new DESKeySpec(key);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(DES);
SecretKey secretKey = keyFactory.generateSecret(dks);
Cipher cipher = Cipher.getInstance(DES);
cipher.init(Cipher.ENCRYPT_MODE, secretKey, sr);
return cipher.doFinal(src);
}
private static byte[] decrypt(byte[] src, byte[] key) throws Exception {
SecureRandom sr = new SecureRandom();
DESKeySpec dks = new DESKeySpec(key);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance(DES);
SecretKey secretKey = keyFactory.generateSecret(dks);
Cipher cipher = Cipher.getInstance(DES);
cipher.init(Cipher.DECRYPT_MODE, secretKey, sr);
return cipher.doFinal(src);
}
private static String byte2hex(byte[] b) {
String hs = "";
String temp = "";
for (int n = 0; n < b.length; n++) {
temp = (java.lang.Integer.toHexString(b[n] & 0XFF));
if (temp.length() == 1)
hs = hs + "0" + temp;
else
hs = hs + temp;
}
return hs.toUpperCase();
}
private static byte[] hex2byte(byte[] b) {
if ((b.length % 2) != 0)
throw new IllegalArgumentException("length not even");
byte[] b2 = new byte[b.length / 2];
for (int n = 0; n < b.length; n += 2) {
String item = new String(b, n, 2);
b2[n / 2] = (byte) Integer.parseInt(item, 16);
}
return b2;
}
private static String decode(String src, String key) {
String decryptStr = "";
try {
byte[] decrypt = decrypt(hex2byte(src.getBytes()), key.getBytes());
decryptStr = new String(decrypt);
} catch (Exception e) {
e.printStackTrace();
}
return decryptStr;
}
private static String encode(String src, String key){
byte[] bytes = null;
String encryptStr = "";
try {
bytes = encrypt(src.getBytes(), key.getBytes());
} catch (Exception ex) {
ex.printStackTrace();
}
if (bytes != null)
encryptStr = byte2hex(bytes);
return encryptStr;
}
/**
* 解密
*/
public static String decode(String src) {
return decode(src, KEY);
}
/**
* 加密
*/
public static String encode(String src) {
return encode(src, KEY);
}
}