-
ES6 环境搭建
目前各大浏览器基本上都支持 ES6 的新特性,其中 Chrome 和 Firefox 浏览器对 ES6 新特性最友好,IE7~11 基本不支持 ES6
-
Let,const
let 声明的变量只在 let 命令所在的代码块内有效。
const 声明一个只读的常量,一旦声明,常量的值就不能改变。
-
对象
-
解构对象
const student = { name: '张三', age: 18 } const { name, age } = student console.log(name, age)
-
给Object扩展新的方法(key,value,entries)
const Person = { name: '张三', age: '18', sex: '0' } console.log(Object.keys(Person)) console.log(Object.values(Person)) console.log(Object.entries(Person)) const Student = {} console.log(Object.keys(Student).length)//可以通过length来判断对象是否为空
-
简写
const name = '张三' const age = 18 //以前 const person = { name: name, age: age } //现在 const person1 = { name, age } console.log(person) //{name: "张三", age: 18} console.log(person1) //{name: "张三", age: 18}
-
对象合并...
const Person = { name: '张三', age: '18', sex: '0' } const Skill = { skill: '会写代码' } const all = { ...Person, ...Skill } const obj = {} Object.assign(obj, Person, Skill) console.log(all) console.log(obj)
-
-
字符串
let name = "Mike"; let age = 27; let info = `My Name is ${name},I'm ${age+1} years old next year.` console.log(info);
-
数组
- 对象转数组
let str = 'hello' console.log(Array.from(str)) //(5) ["h", "e", "l", "l", "o"] const student = { 0: '张三', 1: 18, length: 2 }//这里必须具备length属性 const arr = Array.from(student) console.log(arr) //(2) ["张三", 18]
- 一组值转数据
console.log(Array.of(1, 2, 3, 4)); // [1, 2, 3, 4]
- 扩展方法--查找find
注:查找数组内元素,找到第一个符合条件的数组成员,返回该成员的值,如果没有找到,返回undefinedlet arr = Array.of(1, 2, 3, 4); console.log(arr.find(item => item > 2));
- 扩展方法--查找findIndex()
let arr = Array.of('张三', '李四', '王五', '李四') console.log(arr.findIndex(item => item == '李四')) // 1
注:查找数组内元素,找到第一个符合条件的数组成员,返回该成员的下标(index), 没找到返回-1
- 包含includes()
console.log([1, 2, 3].includes(1)) // true
注:数组是否包含指定值。
-
合并数据
const arr1 = [1, 2, 3] const arr2 = [4, 5, 6] const arr = [...arr1, ...arr2] console.log(arr) //[1, 2, 3, 4, 5, 6]
- 对象转数组
-
函数
- 给参数设置默认值
function student (name, age = 18) { console.log(name + ',' + age) } student('张三') // 张三,18
- 不定参数(不确定参数个数)
function student (...values) { console.log(values) } student('张三', '李四') //["张三", "李四"]
- 箭头函数(基本语法【参数=>函数体】)
var sum = (a, b) => a + b console.log(sum(6, 2)) //8
var Person = { age: 18, sayHello: function () { setTimeout(function () { console.log(this.age) }) } } var age = 20 Person.sayHello() // 22,这里为啥是22,不是20,会在下一篇博客讲 Person1 = { age: 18, sayHello: function () { setTimeout(() => { console.log(this.age) }) } } var age = 22 Person1.age = 20 Person1.sayHello() // 20
注:当我们需要维护一个 this 上下文的时候,就可以使用箭头函数。
- 给参数设置默认值
-
类
- 类声明
class Person { constructor(name) { // 构造函数,默认返回实例对象 this this.name = name; } eat(){ console.log(this.name+'在吃饭') } }
- 类的实例化
new Person('张三').eat() //张三在吃饭
- 类的继承,子类必须在构造函数中写super
class Person{ constructor(){} } class Student extends Person{ constructor(){ super() } }
- 类声明
-
模块化
- 在导入js时,定义一个type="module",通过export定义导出,通过import定义导入(但是这种两边的变量名必须一样)
//common.js export function sum (a, b) { return a + b } //html中 <script type="module"> import { sum } from './index.js' console.log(sum(1, 3)) </script>
- export default function(){}(这种导出就是用户在导入的时候自定义),import * as 别名 from './common.js'(全部导入)
注:在一个文件或模块中,export、import 可以有多个,export default 仅有一个。//common.js export function sum (a, b) { return a + b } const name = '张三' const age = '18' export default { name, age } // html中 import * as obj from './common.js' console.log(obj.default.name) //张三 console.log(obj.default.age) //18 console.log(obj.sum(1, 3)) //4
- 在导入js时,定义一个type="module",通过export定义导出,通过import定义导入(但是这种两边的变量名必须一样)
-
Promise对象
- 状态特点
Promise 异步操作有三种状态:pending(进行中)、fulfilled(已成功)和 rejected(已失败)除了异步操作的结果,任何其他操作都无法改变这个状态 - then方法
通过 .then 形式添加的回调函数,不论什么时候,都会被调用,可以添加多个回调函数(最好保持扁平化,不要嵌套 Promise)new Promise((resolve, reject) => { if (true) {//异步成功后 resolve('成功') //将Promise的状态由padding改为fulfilled } else { reject('失败') //将Promise的状态由padding改为rejected } }) .then( response => { console.log(response) //resolve回调 }, error => { console.log(error) // reject回调 } ) .then(response => { console.log(response) //undefined return Promise.resolve('resolve') }) .then(response => { console.log(response) // resolve })
- 状态特点
- async await
ES6语法详解
最新推荐文章于 2024-06-10 16:23:30 发布
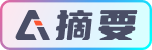