效果截图:
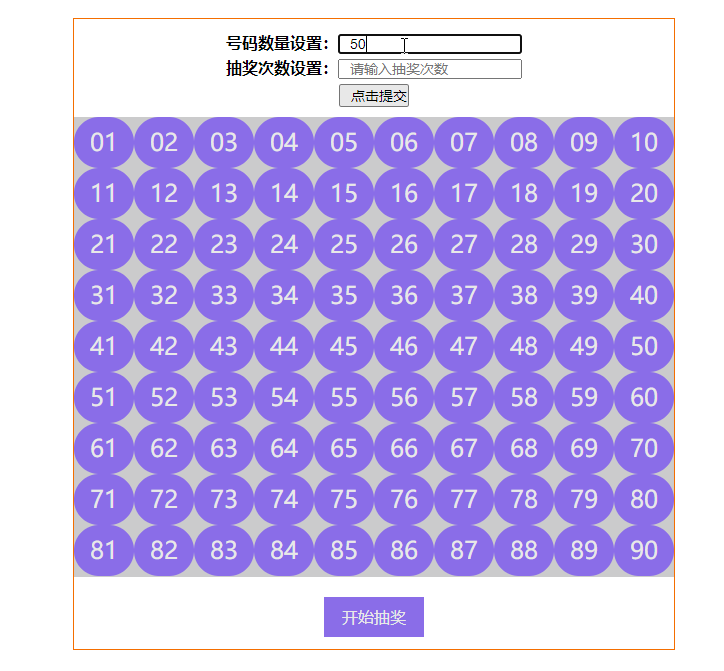
效果说明
- 设置最大号码个数为90,抽奖次数无限制
- 当输入格式不正确时,会设置一个默认的值(号码个数默认为90,抽奖次数默认为1)
- 当输入无误时,点击提交,即可开始抽奖
- 在抽奖过程中,可点击提交按钮重置号码个数及抽奖次数
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>抽奖系统的实现</title>
</head>
<style>
* {
padding: 0;
margin: 0;
}
li {
list-style: none;
}
#box {
position: relative;
margin: 50px auto;
width: 600px;
height: 630px;
border: 1px solid #f46e00;
}
#show {
float: left;
width: 600px;
height: 460px;
background: #cccccc;
}
#start {
float: left;
width: 100px;
height: 40px;
color: #ededed;
user-select: none;
line-height: 40px;
text-align: center;
margin-left: 250px;
margin-top: 20px;
}
.start {
background: #8a6de9;
}
.list {
float: left;
width: 60px;
height: 51px;
font-size: 24px;
border-radius: 60px;
line-height: 50px;
background: #8a6de9;
text-align: center;
color: #e8e8e8;
}
.stop {
background: #888888;
}
.select {
color: #8a6de9;
background: #e8e8e8;
}
.lucky {
display: none;
position: absolute;
left: 0;
top: 98px;
width: 600px;
height: 460px;
line-height: 460px;
text-align: center;
font-size: 56px;
color: #0f0;
background: rgba(0, 0, 0, .7);
}
input::-webkit-outer-spin-button,
input::-webkit-inner-spin-button {
-webkit-appearance: none;
appearance: none;
margin: 0;
}
input {
-moz-appearance: textfield;
font-size: 14px;
padding-left: 10px;
}
input:last-child {
margin-top: 5px;
}
</style>
<body>
<div id="box">
<div style="margin-top: 10px;margin-bottom: 10px;text-align: center">
<div><strong>号码数量设置:</strong><input id="num" title="" type="number" value="" placeholder="号码个数(默认为90)"/></div>
<div><strong>抽奖次数设置:</strong><input id="numClick" title="" type="number" value="" placeholder="请输入抽奖次数"/></div>
<div><input id="submit" type="submit" value="点击提交"></div>
</div>
<div id="show"></div>
<p id="start" class="start">开始抽奖</p>
<div class="lucky"></div>
</div>
<script>
let show = document.getElementById('show');
let start = document.getElementById('start');
let lists = document.getElementsByClassName('list');
let lucky = document.getElementsByClassName('lucky')[0];
let colNum = document.getElementById('num');
let submit = document.getElementById('submit');
let numClick = document.getElementById('numClick');
let cjNum = 3;
let src = '';
let num = 0;
let clickNum = 0;
let maxNum = 90;
let interval = 0;
function getNum() {
src = '';
for (let i = 1; i <= maxNum; i++) {
if (i < 10) {
i = '0' + i;
}
src = src + '<li class="list">' + i + '</li>';
}
show.innerHTML = src;
}
getNum();
submit.onclick = function () {
lucky.style.display = 'none';
start.innerText = '开始抽奖';
if (numClick.value <= 0) {
numClick.value = 1;
alert('至少有一次抽奖');
}
cjNum = numClick.value;
clickNum = 0;
while (show.hasChildNodes()) {
show.removeChild(show.firstChild);
}
if (colNum.value === '' || colNum.value > 90) {
maxNum = 90;
alert('最大值为90,即将为您修改为90');
colNum.value = 90;
} else if (colNum.value < 10) {
maxNum = 10;
alert('最小值为10,即将为您修改为10');
colNum.value = 10;
} else {
maxNum = colNum.value;
}
getNum();
};
start.onclick = function () {
if (clickNum < cjNum) {
if (start.innerText === '停止') {
start.innerText = '再来一次';
clearInterval(interval);
start.setAttribute('class', 'start');
if (num < 10) {
num = '0' + num;
}
lucky.style.display = 'block';
lucky.innerText = "中奖号码为:" + num;
} else {
clickNum++;
interval = 0;
clearInterval(interval);
lucky.style.display = 'none';
start.innerText = '停止';
start.setAttribute('class', 'stop');
interval = setInterval(function () {
num = Math.ceil(Math.random() * maxNum);
for (let i = 0; i < lists.length; i++) {
lists[i].setAttribute('class', 'list');
if (num - 1 === i) {
lists[i].setAttribute('class', 'select list');
}
}
}, 10)
}
} else {
start.innerText = '次数已用完';
start.style.background = 'rgba(0,0,0,0.7)';
start.style.color = '#fff137';
clearInterval(interval);
if (num < 10) {
num = '0' + num;
}
lucky.style.display = 'block';
lucky.innerText = "中奖号码为:" + num;
}
}
</script>
</body>
</html>