QEMU’s virtual network
Packet Inspection
tcpdump -XXnr qemu.pcap
Debugging the E1000
The Network Server
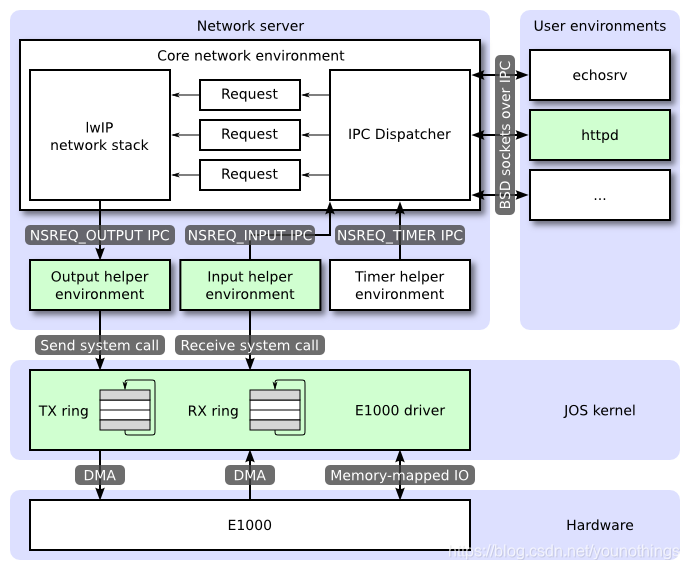
Part A: Initialization and transmitting packets
Exercise1
if (tf->tf_trapno == IRQ_OFFSET + IRQ_TIMER) {
lapic_eoi();
// Add time tick increment to clock interrupts.
// Be careful! In multiprocessors, clock interrupts are
// triggered on every CPU.
// LAB 6: Your code here.
if (thiscpu->cpu_id == 0) {
time_tick();
}
sched_yield();
return;
}
// Return the current time.
static int
sys_time_msec(void)
{
return time_msec();
}
case SYS_time_msec:
return sys_time_msec();
The Network Interface Card
Exercise2
Browse Intel’s Software Developer’s Manual for the E1000.
PCI Interface
E1000 is a PCI device
A PCI device needs to be discovered and initialized before it can be used. Discovery is the process of walking the PCI bus looking for attached devices. Initialization is the process of allocating I/O and memory space as well as negotiating the IRQ line for the device to use.
Exercise3
#define E1000_VEN_ID 0x8086
#define E1000_DEV_ID_82540EM 0x100E
int
e1000_attach(struct pci_func *pcif)
{
pci_func_enable(pcif);
return 0;
}
// pci_attach_class matches the class and subclass of a PCI device
struct pci_driver pci_attach_class[] = {
{ PCI_CLASS_BRIDGE, PCI_SUBCLASS_BRIDGE_PCI, &pci_bridge_attach },
{ 0, 0, 0 },
};
Memory-mapped I/O
Software communicates with the E1000 via memory-mapped I/O (MMIO). You’ve seen this twice before in JOS: both the CGA console and the LAPIC are devices that you control and query by writing to and reading from “memory”. But these reads and writes don’t go to DRAM; they go directly to these devices.
Exercise4
volatile void *e1000_base;
int
e1000_attach(struct pci_func *pcif)
{
pci_func_enable(pcif);
e1000_base = mmio_map_region(pcif->reg_base[0], pcif->reg_size[0]);
return 0;
}
#define E1000_STATUS 0x00008 /* Device Status - RO */
#define E1000_REG(offset) (*(volatile uint32_t *)(e1000_base + offset))
cprintf("e1000: status 0x%08x\n", E1000_REG(E1000_STATUS));
DMA
You could imagine transmitting and receiving packets by writing and reading from the E1000’s registers, but this would be slow and would require the E1000 to buffer packet data internally. Instead, the E1000 uses Direct Memory Access or DMA to read and write packet data directly from memory without involving the CPU. The driver is responsible for allocating memory for the transmit and receive queues, setting up DMA descriptors, and configuring the E1000 with the location of these queues, but everything after that is asynchronous. To transmit a packet, the driver copies it into the next DMA descriptor in the transmit queue and informs the E1000 that another packet is available; the E1000 will copy the data out of the descriptor when there is time to send the packet. Likewise, when the E1000 receives a packet, it copies it into the next DMA descriptor in the receive queue, which the driver can read from at its next opportunity.
The pointers to these arrays as well as the addresses of the packet buffers in the descriptors must all be physical addresses because hardware performs DMA directly to and from physical RAM without going through the MMU.
Transmitting Packets
C Structures
Exercise5
#define E1000_TCTL 0x00400 /* TX Control - RW */
#define E1000_TIPG 0x00410 /* TX Inter-packet gap -RW */
#define E1000_TDBAL 0x03800 /* TX Descriptor Base Address Low - RW */
#define E1000_TDBAH 0x03804 /* TX Descriptor Base Address High - RW */
#define E1000_TDLEN 0x03808 /* TX Descriptor Length - RW */
#define E1000_TDH 0x03810 /* TX Descriptor Head - RW */
#define E1000_TDT 0x03818 /* TX Descripotr Tail - RW */
/* Transmit Control */
#define E1000_TCTL_EN 0x00000002 /* enable tx */
#define E1000_TCTL_PSP 0x00000008 /* pad short packets */
#define E1000_TCTL_CT 0x00000ff0 /* collision threshold */
#define E1000_TCTL_COLD 0x003ff000 /* collision distance */
/* Collision related configuration parameters */
#define E1000_COLLISION_THRESHOLD 0x10
#define E1000_CT_SHIFT 4
/* Collision distance is a 0-based value that applies to half-duplex-capable hardware only. */
#define E1000_COLLISION_DISTANCE 0x40
#define E1000_COLD_SHIFT 12
/* Default values for the transmit IPG register */
#define E1000_DEFAULT_TIPG_IPGT 10
#define E1000_DEFAULT_TIPG_IPGR1 4
#define E1000_DEFAULT_TIPG_IPGR2 6
#define E1000_TIPG_IPGT_MASK 0x000003FF
#define E1000_TIPG_IPGR1_MASK 0x000FFC00
#define E1000_TIPG_IPGR2_MASK 0x3FF00000
#define E1000_TIPG_IPGR1_SHIFT 10
#define E1000_TIPG_IPGR2_SHIFT 20
#define TX_BUF_SIZE 1536 // 16-byte aligned for performance
#define NTXDESC 64
static struct e1000_tx_desc e1000_tx_queue[NTXDESC] __attribute__((aligned(16)));
static uint8_t e1000_tx_buf[NTXDESC][TX_BUF_SIZE];
static void
e1000_tx_init()
{
// initialize tx queue
int i;
memset(e1000_tx_queue, 0, sizeof(e1000_tx_queue));
for (i = 0; i < NTXDESC; i++) {
e1000_tx_queue[i].addr = PADDR(e1000_tx_buf[i]);
}
// initialize transmit descriptor registers
E1000_REG(E1000_TDBAL) = PADDR(e1000_tx_queue);
E1000_REG(E1000_TDBAH) = 0;
E1000_REG(E1000_TDLEN) = sizeof(e1000_tx_queue);
E1000_REG(E1000_TDH) = 0;
E1000_REG(E1000_TDT) = 0;
// initialize transmit control registers
E1000_REG(E1000_TCTL) &= ~(E1000_TCTL_CT | E1000_TCTL_COLD);
E1000_REG(E1000_TCTL) |= E1000_TCTL_EN | E1000_TCTL_PSP |
(E1000_COLLISION_THRESHOLD << E1000_CT_SHIFT) |
(E1000_COLLISION_DISTANCE << E1000_COLD_SHIFT);
E1000_REG(E1000_TIPG) &= ~(E1000_TIPG_IPGT_MASK | E1000_TIPG_IPGR1_MASK | E1000_TIPG_IPGR2_MASK);
E1000_REG(E1000_TIPG) |= E1000_DEFAULT_TIPG_IPGT |
(E1000_DEFAULT_TIPG_IPGR1 << E1000_TIPG_IPGR1_SHIFT) |
(E1000_DEFAULT_TIPG_IPGR2 << E1000_TIPG_IPGR2_SHIFT);
}
int
e1000_attach(struct pci_func *pcif)
{
pci_func_enable(pcif);
e1000_base = mmio_map_region(pcif->reg_base[0], pcif->reg_size[0]);
e1000_tx_init();
return 0;
}