代码:学生控制类对象可以管理学生对象,进行增删改查操作
# 学生信息的增删改查以及显示
class StudentModel:
"""
学生信息模型
"""
__slots__ = ("__sid", "__name", "__age", "__score")
def __init__(self, name="", age=0, score=0, sid=0):
"""
创建学生对象
:param sid:学生id,整形,学生的唯一标识
:param name:学生姓名,字符串类型
:param age:年龄 整形
:param score:成绩 浮点型
"""
self.sid = sid
self.name = name
self.age = age
self.score = score
@property
def sid(self):
return self.__sid
@sid.setter
def sid(self, sid):
if sid >= 0:
self.__sid = sid
else:
raise ValueError("sid should large than 0")
@property
def name(self):
return self.__name
@name.setter
def name(self, name):
if name is not None:
self.__name = name
else:
raise ValueError("name should not be None")
@property
def age(self):
return self.__age
@age.setter
def age(self, age):
if age >= 0:
self.__age = age
else:
raise ValueError("age should not be negative")
@property
def score(self):
return self.__score
@score.setter
def score(self, score):
if score >= 0:
self.__score = score
else:
raise ValueError("score should not be negative")
def print_info(self):
print("StuId:%d StuName:%s Age:%d Score:%d" % (self.sid, self.name, self.age, self.score))
class StudentManagerController:
"""
学生管理控制器,负责业务逻辑处理
"""
# 类变量,初始id值
__init_sid = 1000
def __init__(self):
self.__stu_list = []
@property
def stu_list(self):
"""
返回私有成员变量__stu_list,对外做成只读属性
:return:
"""
return self.__stu_list
def add_student(self, stu_info):
"""
添加一个新学生对象
:param stu_info: 没有编号的学生对象
:return:
"""
sid = self.__generate_id()
stu_info.sid = sid
self.__stu_list.append(stu_info)
def __generate_id(self):
"""
产生一个新sid
:return: 返回产生的新sid
"""
StudentManagerController.__init_sid += 1
return StudentManagerController.__init_sid
def delete_student(self, sid):
"""
根据传入编号,删除学生
:param sid: 传入编号
:return: -1标识没有此编号,0表示删除成功
"""
ret = False
for stu in self.__stu_list:
if stu.sid == sid:
self.__stu_list.remove(stu)
ret = True
break
return ret
def update_student(self, stu_info):
"""
根据学生编号,更改其余信息
:param stu_info: Student对象
:return: True修改成功,False修改失败
"""
sid = stu_info.sid
ret = False
for stu in self.__stu_list:
if stu.sid == sid:
ret = True
stu.name = stu_info.name
stu.age = stu_info.age
stu.score = stu_info.score
break
return ret
class StudentManagerView:
"""
视图类
"""
chooses = ["添加学生", "显示学生", "更新学生", "删除学生", "显示所有学生", "退出"]
def __init__(self):
self.__studentManagerController = StudentManagerController()
def __display_menu__(self):
print("*" * 50)
i = 1
for choose in StudentManagerView.chooses:
print(" " * 10 + "%d) %s" % (i, choose))
i += 1
print("*" * 50)
def __select_menu__(self):
choose = int(input("请选择:"))
if choose == 1:
self.__add_student__()
elif choose == 2:
sid = int(input("请输入要显示学生的编号: "))
stu = self.__find_student__(sid)
if stu is not None:
stu.print_info()
else:
print("没有查找到学生")
elif choose == 3:
self.__update_student__()
elif choose == 4:
self.__delete_student__()
elif choose == 5:
self.__display_student()
elif choose == 6:
print("退出学生信息管理系统")
exit(0)
pass
else:
print("选择无效,请选择1~6")
def __add_student__(self):
while True:
name = input("请输入姓名:")
age = int(input("请输入年龄:"))
score = float(input("请输入成绩:"))
print("请确认输入学生信息:(姓名,年龄, 分数) (%s, %d, %f)" % (name, age, score))
ok = input("请确认(Y|N): ")
if ok.startswith('Y') or ok.startswith('y'):
stu = StudentModel(name, age, score)
self.__studentManagerController.add_student(stu)
print("添加成功")
break
else:
print("取消添加")
def __display_student__(self):
sid = int(input("请输入要显示学生的id"))
stu = self.__find_student__(sid)
if stu is not None:
stu.print_info()
else:
print("不存在输入id的学生")
def __find_student__(self, sid):
for stu in self.__studentManagerController.stu_list:
if stu.sid == sid:
return stu
else:
return None
def __update_student__(self):
sid = int(input("请输入要修改学生的编号:"))
stu = self.__find_student__(sid)
if stu is None:
print("输入编号的学生不存在")
return
print("所要更改的学生信息为:")
stu.print_info()
name = input("请输入姓名:")
age = int(input("请输入年龄:"))
score = float(input("请输入成绩:"))
print("请确认更改学生的信息:(编号,姓名,年龄, 分数) (%d,%s, %d, %f)" % (stu.sid, name, age, score))
ok = input("请确认(Y|N): ")
if ok.startswith('Y') or ok.startswith('y'):
stu_new = StudentModel(name, age, score, stu.sid)
self.__studentManagerController.update_student(stu_new)
print("学生信息更改成功")
else:
print("取消修改学生信息")
def __delete_student__(self):
sid = int(input("请输入需要删除的学生编号:"))
stu = self.__find_student__(sid)
if stu is None:
print("没有找到要删除的学生")
return
print("你要删除的学生信息:")
stu.print_info()
ok = input("请确认你是否要删除(Y|N): ")
if ok.startswith('Y') or ok.startswith('y'):
if self.__studentManagerController.delete_student(sid):
print("删除成功")
else:
print("删除失败")
else:
print("取消删除")
def __display_student(self):
stulist = self.__studentManagerController.stu_list
for stu in stulist:
stu.print_info()
def main(self):
while True:
self.__display_menu__()
self.__select_menu__()
pass
"""
测试代码
"""
if __name__ == "__main__":
# stu = StudentModel("xrd", 12, 80)
# stu.print_info()
#
# # 创建学生管理控制器
# stuManager = StudentManagerController()
#
# # 测试添加学生
# stuManager.add_student(stu)
# stu = StudentModel("xrr", 15, 70)
# stu.print_info()
# stuManager.add_student(stu)
# stu = StudentModel("xps", 5, 90)
# stu.print_info()
# stuManager.add_student(stu)
#
# print("====================================================")
# stulist = stuManager.stu_list
# for stu in stulist:
# stu.print_info()
#
# # 测试删除学生
# print("====================================================")
# if stuManager.delete_student(1002) == 0:
# print("删除成功")
# else:
# print("删除失败")
# stulist = stuManager.stu_list
# for stu in stulist:
# stu.print_info()
#
# # 测试修改学生信息
# print("====================================================")
# stu_old = stuManager.stu_list[0]
# stu_new = StudentModel("x-ray", 18, 100, stu_old.sid)
#
# if stuManager.update_student(stu_new):
# print("修改成功")
# stulist = stuManager.stu_list
# for stu in stulist:
# stu.print_info()
# else:
# print("修改失败")
view = StudentManagerView()
view.main()
测试结果:
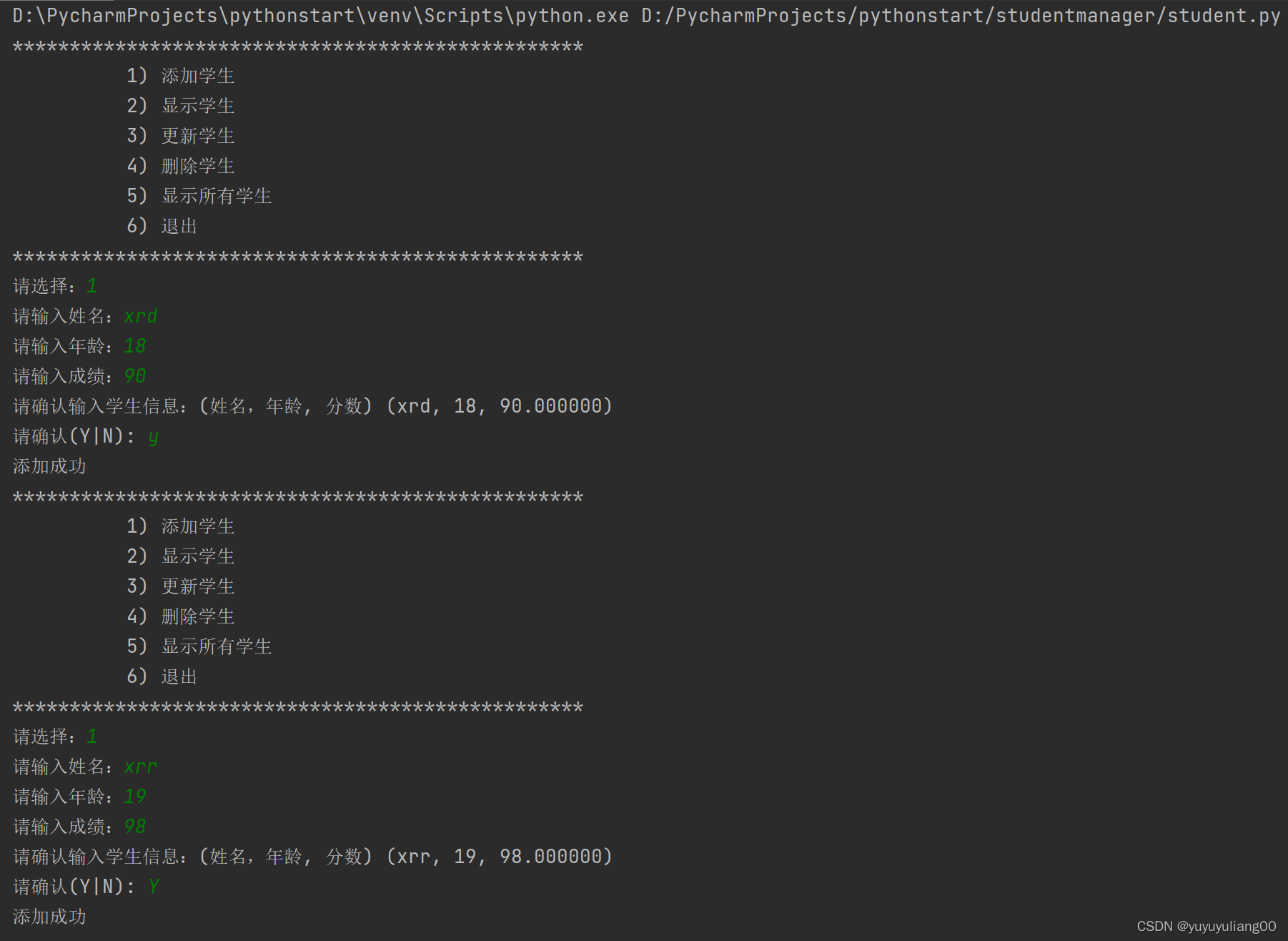
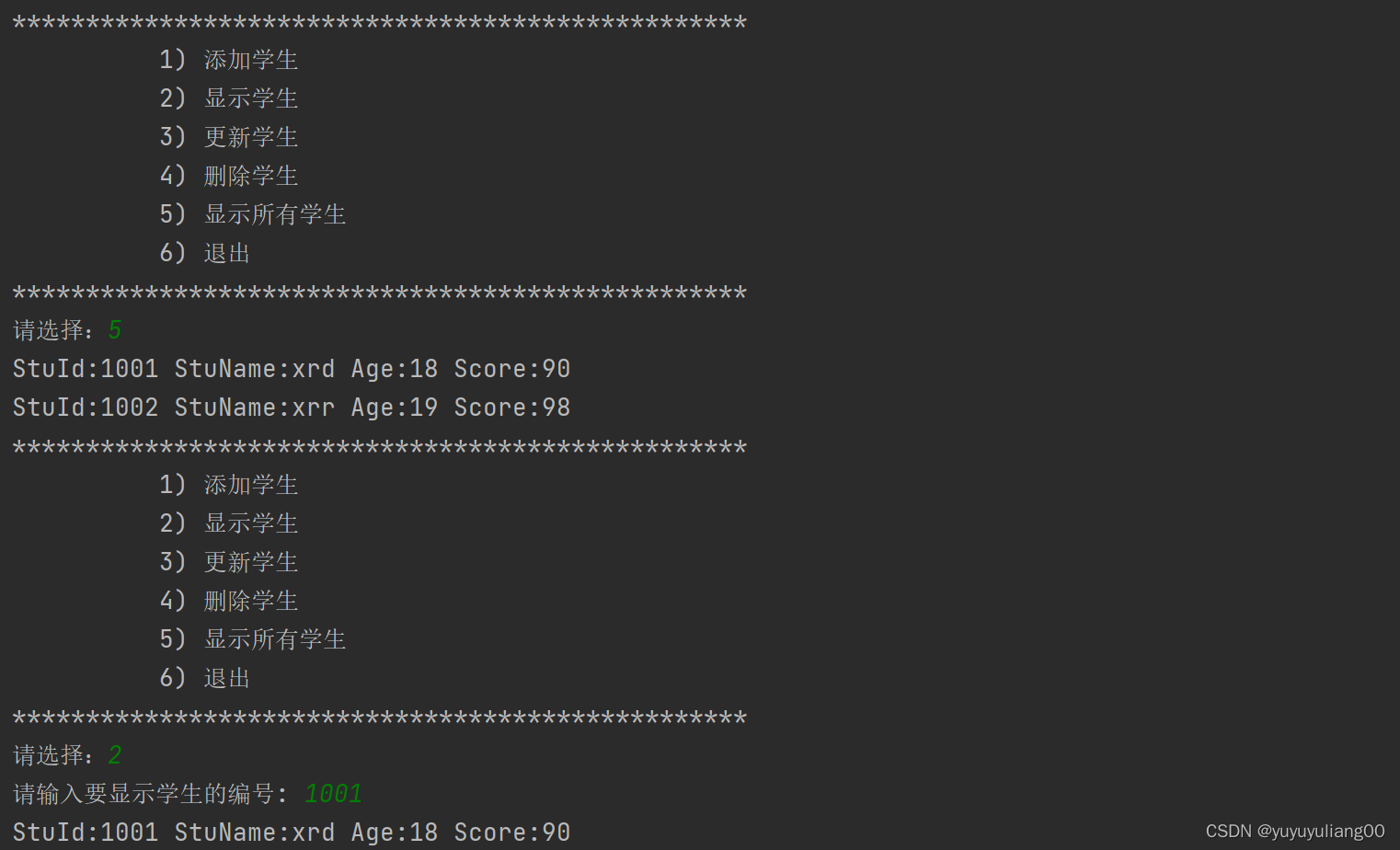
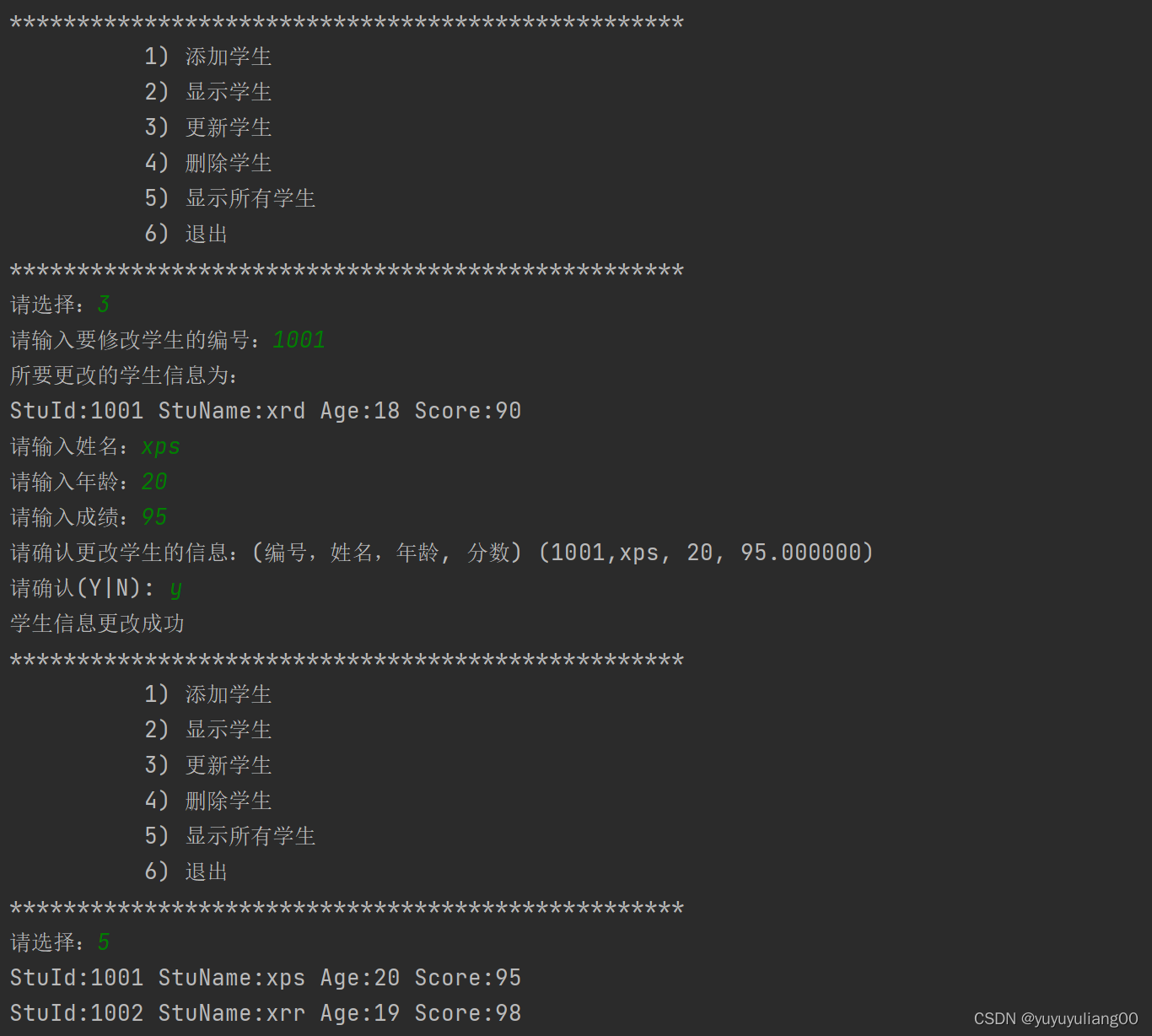
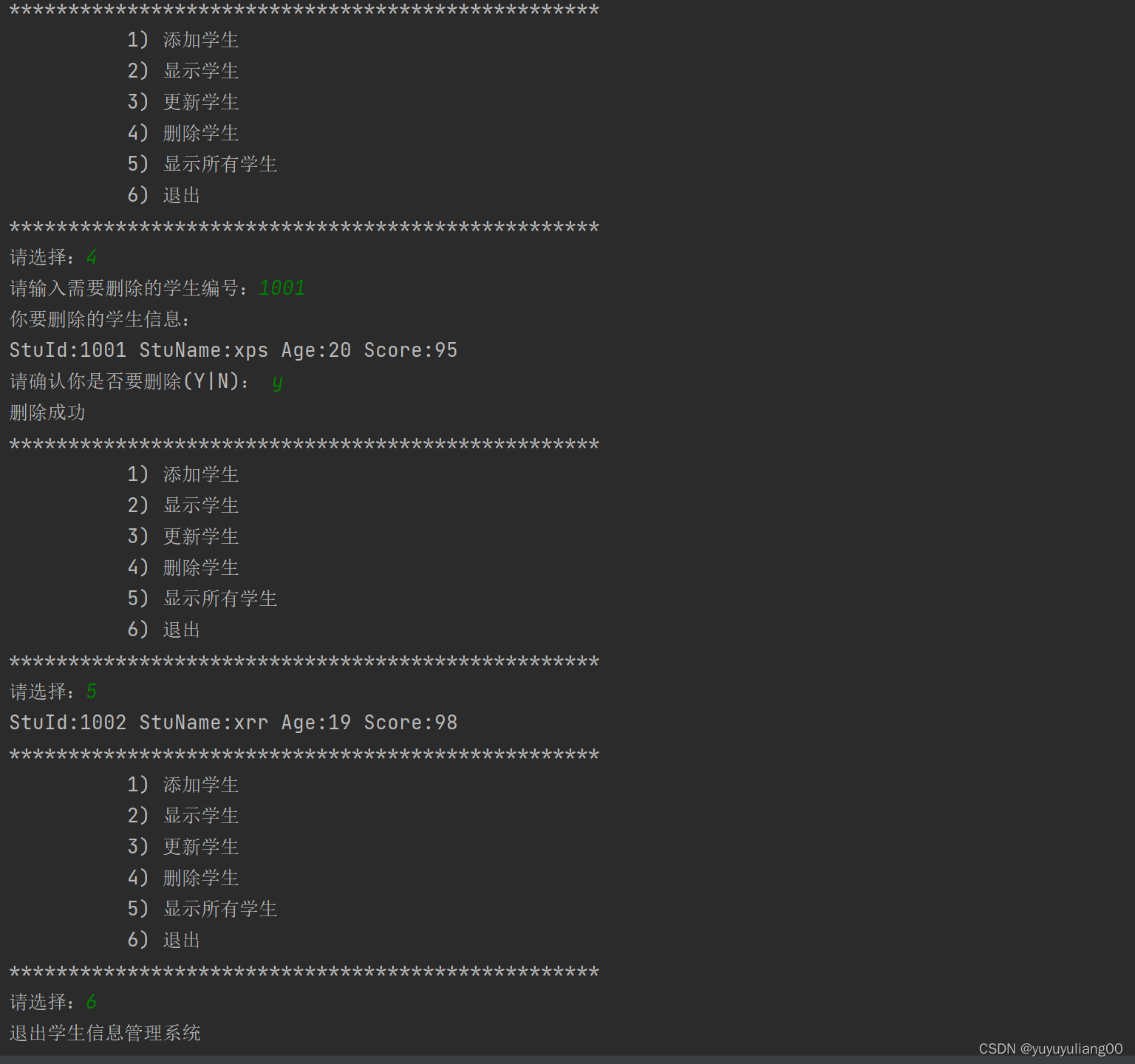