模板
<template>
<div>
<div class="table_box">
<Table :columns="tableColumns" :data="tableData" :height="tableHeight" v-if="tableColumns.length > 0">
<template slot-scope="{ index }" slot="orderNumber">
<strong v-if="isOrderNumberAdd">{{ ((current - 1) * pageSize) + index + 1 }}</strong>
<strong v-else>{{ index + 1 }}</strong>
</template>
<template v-for="(item, index) in customColumns" :slot="item" slot-scope="{ row, column }">
<Tooltip :content="row[item]" transfer placement="top" :key="index">
<span class="custom_column_span" :style="{width: column.width + 'px'}">
{{ row[item] }}
</span>
</Tooltip>
</template>
</Table>
</div>
<div class="paging_box" v-if="showPaging && total > 0">
<div class="total" v-if="showTotal">共<span>{{total}}</span>条数据</div>
<div v-else></div>
<Page
:total="total"
:page-size="pageSize"
:show-sizer="showSizer"
:current="current"
@on-change="handlePageChange"
@on-page-size-change="handlePageSizeChange"
:page-size-opts="pageSizeOpts"
/>
</div>
</div>
</template>
脚本
<script>
import lodash from 'lodash';
import { getSelectCloumnList } from 'api/pubApi';
export default {
props: {
customTableColumns: {
type: Array,
default: () => []
},
tableData: {
type: Array,
default: () => []
},
reqEntry: {
type: String,
required: true,
default: () => ''
},
tableHeight: {
type: Number,
default: () => 280
},
serviceName: {
type: String,
default: () => ''
},
columnAlign: {
type: String,
default: () => 'center'
},
showTotal: {
type: Boolean,
default: () => true
},
total: {
type: Number,
default: () => 10
},
showPaging: {
type: Boolean,
default: () => true
},
pageSize: {
type: Number,
default: () => 10
},
showSizer: {
type: Boolean,
default: () => true
},
current: {
type: Number,
default: () => 1
},
pageSizeOpts: {
type: Array,
default: () => [8, 16, 24, 32]
},
isOrderNumberAdd: {
type: Boolean,
default: () => true
},
customColumns: {
type: Array,
default: () => []
}
},
watch: {
reqEntry: {
handler () {
this.getTableColumn();
},
deep: true,
immediate: true
}
},
data () {
return {
tableColumns: [],
toolsColumns: [
{
title: '序号',
key: 'orderNumber',
code: 'orderNumber',
slot: 'orderNumber',
align: this.columnAlign,
width: 80,
},
],
}
},
methods: {
getTableColumn () {
if (!lodash.isEmpty(this.reqEntry)) {
const cloumnList = JSON.parse(sessionStorage.getItem(this.reqEntry));
if (cloumnList) {
this.tableColumns = cloumnList;
} else {
getSelectCloumnList(this.reqEntry, this.serviceName).then((res) => {
if (res && res.code === 200) {
const tableColumns = res.data;
if (tableColumns.length === 0) {
console.error(this.reqEntry, '列不能为空!');
return;
}
this.tableColumns = this.handleTableColumns(tableColumns);
sessionStorage.setItem(this.reqEntry, JSON.stringify(this.tableColumns));
}
});
}
}
},
handleTableColumns (tableColumns) {
let arr = [];
arr = tableColumns.map((item, index) => {
let column = {
key: item.code,
code: item.code,
title: item.title,
align: this.columnAlign,
width: Number(item.width) + 20,
operation: Number(item.operation)
};
if (this.customColumns.indexOf(item.code) >= 0) {
column.slot = item.code;
}
return column;
})
arr = [...this.toolsColumns, ...arr];
console.log(arr);
return arr;
},
handlePageChange (pageNo) {
this.$emit('page-change', pageNo);
},
handlePageSizeChange (pageSize) {
this.$emit('page-size-change', pageSize);
}
},
}
</script>
样式
<style lang="less" scoped>
// 表格公共样式修改
.table_box {
margin: 10px 20px 0px;
.custom_column_span {
display: inline-block;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
padding-right: 12px;
text-align: center;
}
}
/deep/ .ivu-table-wrapper {
border: 1px solid rgba(41, 99, 158, 1);
// min-height: 194px;
height: auto !important;
.ivu-table {
background-color: transparent;
color: #fff;
// border-left: 1px solid rgba(41, 99, 158, 1);
border-top: 1px solid rgba(41, 99, 158, 1);
overflow: hidden;
&::before {
height: 0;
}
th, td {
padding: 0px 0;
height: 30px;
border-bottom-color: rgba(41, 99, 158, 1);
border-right: 1px solid rgba(41, 99, 158, 1);
font-size: 12px;
&:last-child {
border-right: 0;
}
}
th {
font-weight: normal;
background-color: rgba(28, 98, 172, 1);
}
td {
background-color: transparent;
.ivu-table-cell {
padding-left: 8px;
padding-right: 8px;
span {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
}
}
.ivu-table-body {
tr:nth-child(2n) td {
background: rgba(4, 41, 88, 1);
}
}
// 暂无数据设置
.ivu-table-tip table {
min-height: 180px;
table-layout: auto;
}
}
}
// 分页
.paging_box {
display: flex;
align-items: center;
justify-content: space-between;
padding: 0 20px;
height: 30px;
.total {
font-size: 14px;
color: #fff;
letter-spacing: 1px;
span {
color: #035ede;
letter-spacing: 0;
padding: 0 2px;
}
}
/deep/ .ivu-page {
margin-bottom: 0;
li {
width: 18px;
height: 22px;
min-width: 18px;
font-size: 12px;
line-height: 2em;
a {
margin: 0;
color: #fff;
}
}
.ivu-page-item {
background-color: #11438d;
border: 1px solid #0750a9;
border: 0;
font-weight: normal;
&.ivu-page-item-active {
border: 1px solid #0750a9;
box-sizing: border-box;
line-height: 1.9em;
background-color: #286ab9;
}
}
.ivu-page-next, .ivu-page-prev {
// background-color: transparent;
border: 0;
background-color: #11438d;
border: 1px solid #0750a9;
line-height: 1.8em;
}
.ivu-page-options {
margin-left: 5px;
.ivu-page-options-sizer {
margin-right: 0;
.ivu-select-selection {
height: 22px;
// background-color: transparent;
background-color: #11438d;
border: 1px solid #0750a9;
color: #fff;
position: relative;
top: -4px;
.ivu-select-selected-value {
line-height: 20px;
font-size: 12px;
}
.ivu-select-arrow {
color:#fff;
font-size: 12px;
font-weight: normal;
}
}
.ivu-select-placeholder {
height: 22px;
line-height: 1.7em;
font-size: 12px;
}
/deep/ .ivu-select-dropdown {
background-color: #286ab9;
padding-top: 6px;
.ivu-select-item {
width: 100%;
padding: 0px 8px;
font-size: 12px !important;
height: 18px;
line-height: 1.6em;
color: #fff;
&:hover {
background-color: #225594;
}
&.ivu-select-item-focus {
background-color: #225594;
}
}
}
}
}
}
}
</style>
<style lang="less">
::-webkit-scrollbar {
width: 7px;
height: 7px;
// display:none;
}
::-webkit-scrollbar-thumb {
// background-color: #bbb;
background-color: rgba(28, 98, 172, 1);
border-radius: 10px;
border: 0;
cursor: pointer;
}
::-webkit-scrollbar-thumb:hover {
// background-color: #aaa;
background: rgba(14, 76, 143, 1);
}
::-webkit-scrollbar-corner {
background-color: transparent;
}
.ivu-tooltip-inner {
padding: 4px 8px;
white-space: normal;
text-align: justify;
background: rgba(8,31,130, 0.9);
box-shadow: 0 0 1px 1px rgba(58,219,248,.8);
}
</style>
效果图
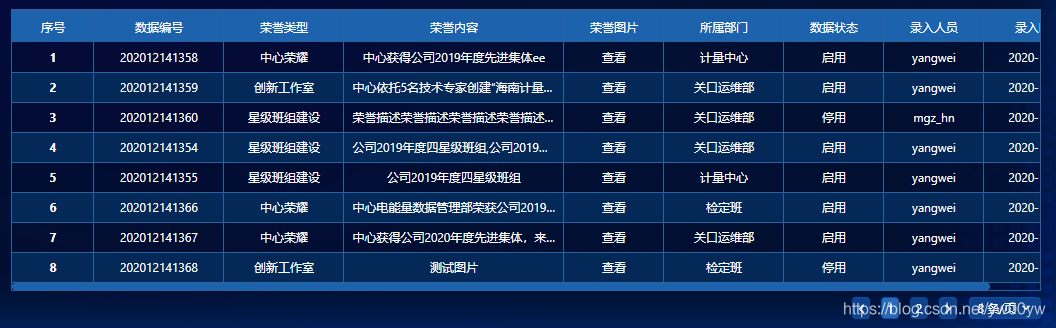
使用方式
<BigCustomTable
class="custom_table"
serviceName="hnksh"
reqEntry="ResHdry"
:tableData="tableDatalist"
:current="pageNo"
:pageSize="pageSize"
:total="total"
:tableHeight="219"
@page-change="handlePageChange"
@page-size-change="handlePageSizeChange"
:pageSizeOpts="[6, 12, 18, 24]"
>
</BigCustomTable>
import BigCustomTable from '_c/BigCustomTable';
import { getHonorList } from 'api/screenApi/gloryAchievementInnovation.js';
data () {
return {
moreParamsMeter: {},
pageNo: 1,
pageSize: 6,
total: 0,
tableDatalist: [],
}
},
methods: {
getTableData() {
getHonorList(this.moreParamsMeter, this.pageNo, this.pageSize).then((res) => {
if (res.code === 200) {
this.tableDatalist = res.data.list;
this.total = res.data.total - 0;
}
});
},
handlePageChange (val) {
this.pageNo = val;
this.getTableData();
},
handlePageSizeChange (val) {
this.pageSize = val;
if (this.pageNo !== 1) {
return;
}
this.getTableData();
},
},