Top-model Izabella participates in the competition. She wants to impress judges and show her mathematical skills.
Her problem is following: for given string, consisting of only 0 and 1, tell if it's possible to remove some digits in such a way, that remaining number is a representation of some positive integer, divisible by 64, in the binary numerical system.
In the only line given a non-empty binary string s with length up to100.
Print «yes» (without quotes) if it's possible to remove digits required way and «no» otherwise.
100010001
yes
100
no
In the first test case, you can get string 1 000 000 after removing two ones which is a representation of number64 in the binary numerical system.
You can read more about binary numeral system representation here: https://en.wikipedia.org/wiki/Binary_system
题意:给你只有0和1的串,问是否能删去一些0或者1是串变成1 000 000。
思路:只需要从最开始找到第一个1,输出后面有多少个0如果大于等于6个就满足条件。
#include<bits/stdc++.h>
using namespace std;
int main ()
{
char s[105];
int num[105] = {0};
scanf("%s",s);
int len = strlen(s);
int flag = 0;
for (int i = 0; i < len; i++){
if (s[i] == '1') {
int cnt = 0;
for (int j = i; j < len; j++){
if (s[j] == '0') cnt++;
}
if (cnt >= 6) flag = 1;
}
}
if (flag) printf("yes\n");
else printf("no\n");
}
Absent-minded Masha got set of n cubes for her birthday.
At each of 6 faces of each cube, there is exactly one digit from 0 to 9. Masha became interested what is the largest naturalx such she can make using her new cubes all integers from 1 tox.
To make a number Masha can rotate her cubes and put them in a row. After that, she looks at upper faces of cubes from left to right and reads the number.
The number can't contain leading zeros. It's not required to use all cubes to build a number.
Pay attention: Masha can't make digit 6 from digit 9 and vice-versa using cube rotations.
In first line integer n is given (1 ≤ n ≤ 3) — the number of cubes, Masha got for her birthday.
Each of next n lines contains 6 integersaij (0 ≤ aij ≤ 9) — number onj-th face of i-th cube.
Print single integer — maximum number x such Masha can make any integers from 1 tox using her cubes or 0 if Masha can't make even 1.
3 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7
87
3 0 1 3 5 6 8 1 2 4 5 7 8 2 3 4 6 7 9
98
In the first test case, Masha can build all numbers from 1 to 87, but she can't make 88 because there are no two cubes with digit 8.
题意:给出n个骰子,每个骰子固定6各面,问n个骰子组合,可以构成的最大的数。
思路:这题的构造其实非常巧妙。首先我们看一共有10个数字(0到9),最大有3个骰子,没一个骰子有4个数字是没有办法取到的,也就是说最多有12个数字没有办法取到,
除去00这种情况,至少有一种11,22,33,44,55,66,77,88,99取不到,也就是说答案必定是两位数。有了这个结论,我们在进行暴力求解很更加清晰。
#include<bits/stdc++.h>
using namespace std;
int cube[10][10];
int flag[100];
int main ()
{
int t; scanf("%d",&t);
for (int j = 1; j <= t; j ++){
for (int i = 1; i <= 6; i++) {
scanf("%d",&cube[j][i]);
}
}
for(int i = 1; i <= t; i++){
for (int j = 1; j <= 6; j++){
flag[cube[i][j]] = 1;
for (int k = 1; k <= t; k++){
if (k == i) continue;
for (int l = 1; l <= 6; l ++){
flag[cube[i][j]*10+cube[k][l]] = 1;
flag[cube[k][l]*10+cube[i][j]] = 1;
}
}
}
}
for (int i = 1; i <= 100; i++){
if (flag[i] == 0) {
printf("%d\n",i-1);
return 0;
}
}
}
During the breaks between competitions, top-model Izabella tries to develop herself and not to be bored. For example, now she tries to solve Rubik's cube 2x2x2.
It's too hard to learn to solve Rubik's cube instantly, so she learns to understand if it's possible to solve the cube in some state using 90-degrees rotation of one face of the cube in any direction.
To check her answers she wants to use a program which will for some state of cube tell if it's possible to solve it using one rotation, described above.
Cube is called solved if for each face of cube all squares on it has the same color.
https://en.wikipedia.org/wiki/Rubik's_Cube
In first line given a sequence of 24 integers ai (1 ≤ ai ≤ 6), where ai denotes color ofi-th square. There are exactly 4 occurrences of all colors in this sequence.
Print «YES» (without quotes) if it's possible to solve cube using one rotation and «NO» (without quotes) otherwise.
2 5 4 6 1 3 6 2 5 5 1 2 3 5 3 1 1 2 4 6 6 4 3 4
NO
5 3 5 3 2 5 2 5 6 2 6 2 4 4 4 4 1 1 1 1 6 3 6 3
YES
In first test case cube looks like this:

In second test case cube looks like this:
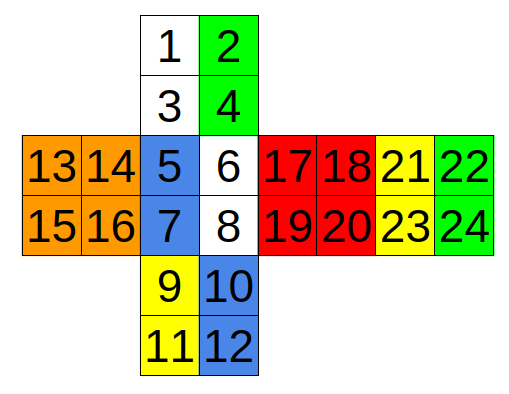
It's possible to solve cube by rotating face with squares with numbers 13, 14, 15, 16.
题意:二阶魔方,只转一次,问是否能还原。
思路:模拟即可。考虑3种情况,左右边如果都是涂好的颜色(如例2) 就考虑其他四个面是否能通过转一次就是转出来。其他同理。
#include<bits/stdc++.h>
using namespace std;
int a[30];
int main ()
{
for (int i = 1; i <= 24; i++){
scanf("%d",&a[i]);
}
int flag = 0;
if(a[13]==a[14]&&a[14]==a[15]&&a[15]==a[16]&&a[17]==a[18]&&a[18]==a[19]&&a[19]==a[20]) // 左右
{
if (a[1]==a[3] && a[3]==a[6] && a[6]==a[8]){
if (a[2]==a[4]&&a[4]==a[22]&&a[22]==a[24]){
if (a[9]==a[11]&&a[11]==a[21]&&a[21]==a[23])
if (a[5]==a[7]&&a[7]==a[10]&&a[10]&&a[12]) flag = 1;
}
}
if (a[11]==a[9] && a[9]==a[6] && a[6]==a[8]){
if (a[2]==a[4]&&a[4]==a[5]&&a[5]==a[7]){
if (a[1]==a[3]&&a[3]==a[21]&&a[21]==a[23]) {
if (a[10]==a[12] && a[12]==a[22]&&a[22]==a[24])flag = 1;
}
}
}
}
if(a[1]==a[2]&&a[2]==a[3]&&a[3]==a[4]&&a[9]==a[10]&&a[10]==a[11]&&a[11]==a[12]) // 左右
{
if (a[5]==a[6] && a[6]==a[15] && a[15]==a[16]){
if (a[7]==a[8]&&a[8]==a[17]&&a[17]==a[18]){
if (a[19]==a[20]&&a[20]==a[21]&&a[21]==a[22])
if (a[13]==a[14]&&a[14]==a[23]&&a[23]&&a[24]) flag = 1;
}
}
if (a[7]==a[8] && a[8]==a[13] && a[13]==a[14]){
if (a[19]==a[20]&&a[20]==a[5]&&a[5]==a[6]){
if (a[17]==a[18]&&a[18]==a[23]&&a[23]==a[24]){
if (a[15]==a[16]&&a[16]==a[21]&&a[21]==a[22]) flag = 1;
}
}
}
}
if(a[5]==a[6]&&a[6]==a[7]&&a[7]==a[8]&&a[21]==a[22]&&a[22]==a[23]&&a[23]==a[24]) // 左右
{
if (a[9]==a[10] && a[10]==a[18] && a[18]==a[20]){
if (a[17]==a[19]&&a[19]==a[1]&&a[1]==a[2]){
if (a[3]==a[4]&&a[4]==a[13]&&a[13]==a[15])
if (a[11]==a[12]&&a[14]==a[12]&&a[14]&&a[16]) flag = 1;
}
}
if (a[3]==a[4] && a[4]==a[18] && a[18]==a[20]){
if (a[11]==a[12]&&a[12]==a[17]&&a[17]==a[19]){
if (a[9]==a[10]&&a[10]==a[13]&&a[13]==a[15]){
if (a[1]==a[2]&&a[2]==a[14]&&a[14]==a[16]) flag = 1;
}
}
}
}
if (flag == 1) printf("YES\n");
else printf("NO\n");
}