UIBlurEffect
// 对应下图的效果
typedef NS_ENUM(NSInteger, UIBlurEffectStyle) {
UIBlurEffectStyleExtraLight, // 额外亮
UIBlurEffectStyleLight, // 亮
UIBlurEffectStyleDark, // 黑色
UIBlurEffectStyleExtraDark, // 额外黑,iOS上不能用
UIBlurEffectStyleRegular, // 常规
UIBlurEffectStyleProminent, // 适应用户界面风格
} NS_ENUM_AVAILABLE_IOS(8_0);
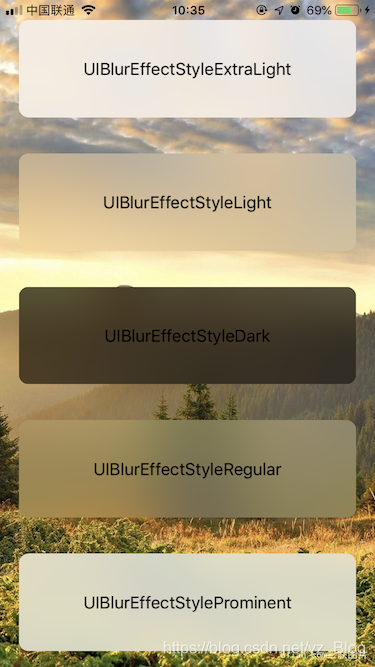
/*
UIBlurEffect将提供一个模糊的效果,它似乎已经应用于UIVisualEffectView后面的内容层。
添加到contentView的视图具有模糊的视觉效果,不会使自身模糊。
*/
NS_CLASS_AVAILABLE_IOS(8.0) @interface UIBlurEffect : UIVisualEffect
/* 初始化一个UIBlurEffect对象,并指定模糊效果 */
+ (UIBlurEffect *)effectWithStyle:(UIBlurEffectStyle)style;
@end
示例
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
UIImageView * imageView = [[UIImageView alloc] init];
imageView.frame = self.view.bounds;
imageView.image = [UIImage imageNamed:@"wallpaper"];
[self.view addSubview:imageView];
UIVisualEffectView * extraLightView = [self effectViewFromStyle:UIBlurEffectStyleExtraLight];
[imageView addSubview:extraLightView];
UIVisualEffectView * lightView = [self effectViewFromStyle:UIBlurEffectStyleLight];
[imageView addSubview:lightView];
UIVisualEffectView * darkView = [self effectViewFromStyle:UIBlurEffectStyleDark];
[imageView addSubview:darkView];
UIVisualEffectView * regularView = [self effectViewFromStyle:UIBlurEffectStyleRegular];
[imageView addSubview:regularView];
UIVisualEffectView * prominentView = [self effectViewFromStyle:UIBlurEffectStyleProminent];
[imageView addSubview:prominentView];
}
- (UIVisualEffectView *)effectViewFromStyle:(UIBlurEffectStyle)style{
CGRect rect = self.view.bounds;
NSString * labelText = @"";
NSInteger index = 0;
switch (style) {
case UIBlurEffectStyleExtraLight:
labelText = @"UIBlurEffectStyleExtraLight";
index = 0;
break;
case UIBlurEffectStyleLight:
labelText = @"UIBlurEffectStyleLight";
index = 1;
break;
case UIBlurEffectStyleDark:
labelText = @"UIBlurEffectStyleDark";
index = 2;
break;
case UIBlurEffectStyleRegular:
labelText = @"UIBlurEffectStyleRegular";
index = 3;
break;
case UIBlurEffectStyleProminent:
labelText = @"UIBlurEffectStyleProminent";
index = 4;
break;
default: break;
}
// 创建UIBlurEffect类的对象blur
UIBlurEffect *blurForHeadImage = [UIBlurEffect effectWithStyle:style];
// 创建UIVisualEffectView的对象visualView, 以blur为参数
UIVisualEffectView * visualViewForHeadImage = [[UIVisualEffectView alloc] initWithEffect:blurForHeadImage];
visualViewForHeadImage.layer.cornerRadius = 10;
visualViewForHeadImage.clipsToBounds = YES;
visualViewForHeadImage.frame = CGRectMake(rect.size.width * 0.05,
20 + index * ((rect.size.height - 100) * 0.2 + 20),
rect.size.width * 0.9,
(rect.size.height - 100 - 80) * 0.2);
UILabel * label = [UILabel new];
label.frame = visualViewForHeadImage.bounds;
label.textAlignment = NSTextAlignmentCenter;
[visualViewForHeadImage.contentView addSubview:label];
label.text = labelText;
return visualViewForHeadImage;
}