package com.hyzs.szcg.doc.utils; import java.awt.image.BufferedImage; import java.io.ByteArrayInputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.*; import java.util.List; import java.util.regex.Matcher; import java.util.regex.Pattern; import com.hyzs.gz.common.core.exception.CommonException; import lombok.extern.slf4j.Slf4j; import org.apache.commons.lang.StringUtils; import org.apache.poi.openxml4j.exceptions.InvalidFormatException; import org.apache.poi.util.Units; import org.apache.poi.xwpf.usermodel.*; import sun.misc.BASE64Decoder; import javax.imageio.ImageIO; /** * * @Title: WordExport.java * @Description: 导出word文档 * @author zzh * @version 1.0 */ @Slf4j public class PoiExportWord { private String templatePath; private XWPFDocument doc = null; private FileInputStream is = null; private OutputStream os = null; public PoiExportWord(String templatePath) { this.templatePath = templatePath; } public void init() throws IOException { File file=new File(this.templatePath); if(file.exists()){ is = new FileInputStream(file); doc = new XWPFDocument(is); }else{ log.error("io error -> 文件模板不存在》"+this.templatePath); throw CommonException.exception("io error 模板文件不存在"); } } /** * 替换掉占位符 * @param params * @return * @throws Exception */ public boolean export(Map<String,Object> params) throws Exception{ this.replaceInPara(doc, params); return true; } /** * 替换掉表格中的占位符 * @param params * @param tableIndex * @return * @throws Exception */ public boolean export(Map<String,Object> params,int tableIndex) throws Exception{ this.replaceInTable(doc, params,tableIndex); return true; } /** * 生成word文档 * @param outDocPath * @return * @throws IOException */ public boolean generate(String outDocPath) throws IOException{ os = new FileOutputStream(outDocPath); doc.write(os); this.close(os); this.close(is); return true; } /** * 替换表格里面的变量 * * @param doc * 要替换的文档 * @param params * 参数 * @throws Exception */ private void replaceInTable(XWPFDocument doc, Map<String, Object> params,int tableIndex) throws Exception { List<XWPFTable> tableList = doc.getTables(); if(tableList.size()>tableIndex){ for (int i=0;i<tableList.size();i++){ XWPFTable table = tableList.get(i); List<XWPFTableRow> rows; List<XWPFTableCell> cells; List<XWPFParagraph> paras; rows = table.getRows(); for (XWPFTableRow row : rows) { cells = row.getTableCells(); for (XWPFTableCell cell : cells) { paras = cell.getParagraphs(); for (XWPFParagraph para : paras) { this.replaceInPara(para, params); } } } } } } /** * 替换段落里面的变量 * * @param doc * 要替换的文档 * @param params * 参数 * @throws Exception */ private void replaceInPara(XWPFDocument doc, Map<String, Object> params) throws Exception { Iterator<XWPFParagraph> iterator = doc.getParagraphsIterator(); XWPFParagraph para; while (iterator.hasNext()) { para = iterator.next(); this.replaceInPara(para, params); } } /** * 替换段落里面的变量 * * @param para * 要替换的段落 * @param params * 参数 * @throws Exception * @throws IOException * @throws */ private boolean replaceInPara(XWPFParagraph para, Map<String, Object> params) throws Exception { boolean data = false; List<XWPFRun> runs; //有符合条件的占位符 if (this.matcher(para.getParagraphText()).find()) { runs = para.getRuns(); data = true; Map<Integer,String> tempMap = new TreeMap<>(); for (int i = 0; i < runs.size(); i++) { XWPFRun run = runs.get(i); String runText = run.toString(); //以"$"开头 boolean begin = runText.indexOf("$")>-1; boolean end = runText.indexOf("}")>-1; if(begin && end){ tempMap.put(i, runText); fillBlock(para, params, tempMap, i); continue; }else if(begin && !end){ tempMap.put(i, runText); continue; }else if(!begin && end){ tempMap.put(i, runText); fillBlock(para, params, tempMap, i); continue; }else{ if(tempMap.size()>0){ tempMap.put(i, runText); continue; } continue; } } } else if (this.matcherRow(para.getParagraphText())) { runs = para.getRuns(); data = true; } return data; } /** * 填充run内容 * @param para * @param params * @param tempMap * @param * @param * @throws * @throws IOException * @throws Exception */ @SuppressWarnings("rawtypes") private void fillBlock(XWPFParagraph para, Map<String, Object> params, Map<Integer, String> tempMap, int index) throws InvalidFormatException, IOException, Exception { Matcher matcher; if(tempMap!=null&&tempMap.size()>0){ String wholeText = ""; List<Integer> tempIndexList = new ArrayList<Integer>(); for(Map.Entry<Integer, String> entry :tempMap.entrySet()){ tempIndexList.add(entry.getKey()); wholeText+=entry.getValue(); } if(wholeText.equals("")){ return; } matcher = this.matcher(wholeText); if (matcher.find()) { boolean isPic = false;//图片标识 boolean isBasePic = false;//base64图片标识 boolean isUnderLine=false;//下划线标识 boolean checkbox=false;//复选框标识 ByteArrayInputStream inputStream=null; int width = 0; int height = 0; int picType = 0; String path = null; String keyText = matcher.group().substring(2,matcher.group().length()-1); String newRunText = ""; Object value = params.get(keyText); if(value instanceof String){ newRunText = matcher.replaceFirst(String.valueOf(value)); }else if(value instanceof Map){ Map pic = (Map)value; if(pic.containsKey("fileSuffix")&&pic.containsKey("filePath")){ //插入图片 isPic = true; path = pic.get("filePath").toString(); //获取图片大小 BufferedImage sourceImg = ImageIO.read(getPicStream(path)); width=sourceImg.getWidth(); height=sourceImg.getHeight(); if(width>200){ width=200; } if(height>200){ height=200; } if(keyText.endsWith("Sign")||keyText.endsWith("_sign")){//签名限制大小 width=50; height=30; } picType=sourceImg.getType(); }else if(pic.containsKey("personalSignature")){ // base64字符串的 图片形式 isBasePic=true; //将字符串转换为byte数组 String file= (String) pic.get("personalSignature"); file=file.replaceFirst("data:image/png;base64,",""); byte[] bytes = new BASE64Decoder().decodeBuffer(file.trim()); //转化为输入流 inputStream = new ByteArrayInputStream(bytes); //获取图片大小 BufferedImage sourceImg = ImageIO.read(new ByteArrayInputStream(bytes)); width = sourceImg.getWidth(); height = sourceImg.getHeight(); if(width>200){ width=200; } if(height>200){ height=200; } if(keyText.endsWith("Sign")||keyText.endsWith("_sign")){//签名限制大小 width=50;
java poi生成word
最新推荐文章于 2022-11-05 15:15:32 发布
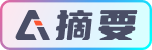