目录
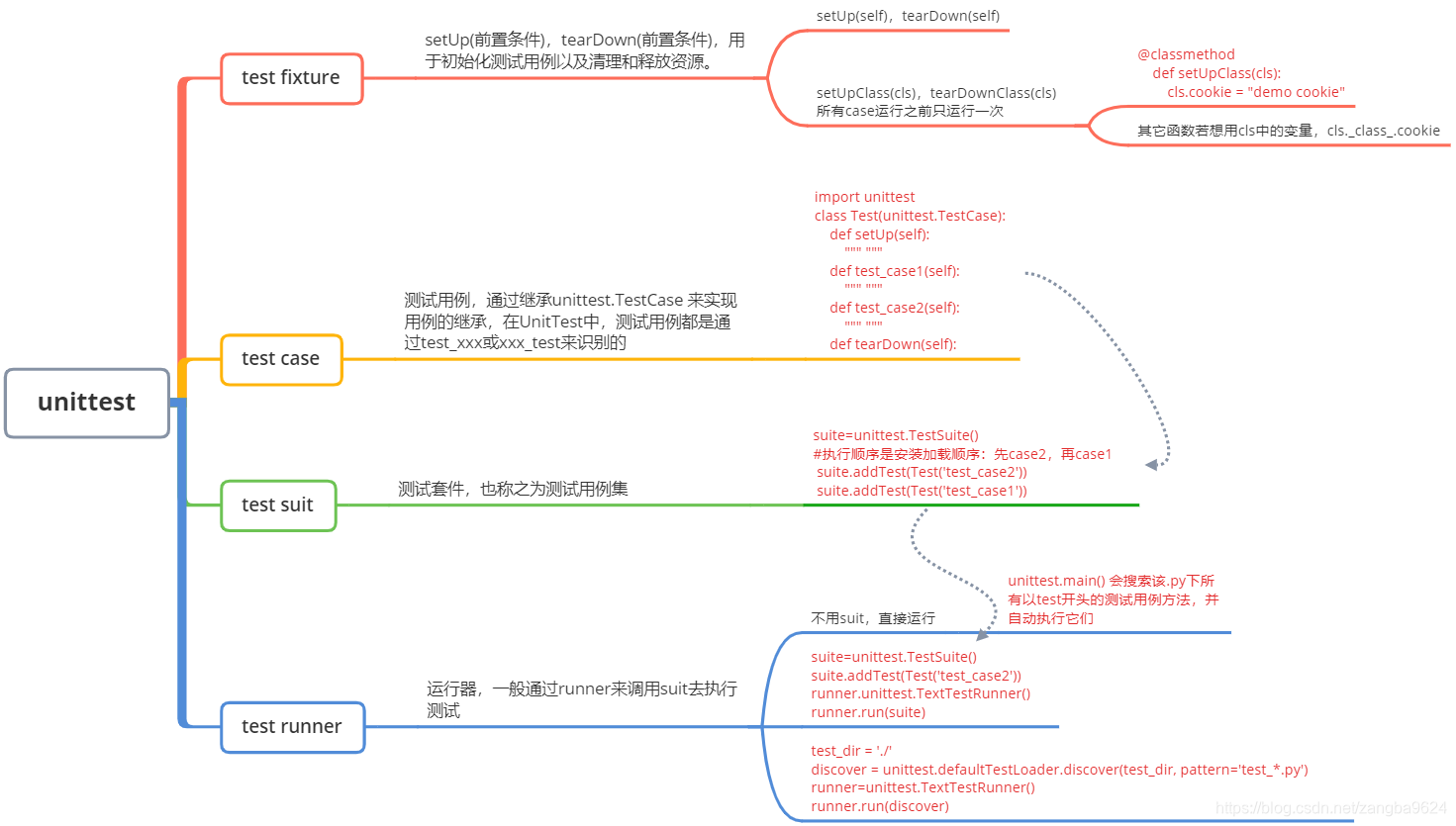
1、test case
# coding=utf-8
import unittest
#1.定义测试类,父类为unittest.TestCase。
#可继承unittest.TestCase的方法,如setUp和tearDown方法,不过此方法可以在子类重写,覆盖父类方法。
#可继承unittest.TestCase的各种断言方法。
class Test(unittest.TestCase):
#2.定义setUp()方法用于测试用例执行前的初始化工作。
#注意,所有类中方法的入参为self,定义方法的变量也要“self.变量”
#注意,输入的值为字符型的需要转为int型
def setUp(self):
self.number=raw_input('Enter a number:')
self.number=int(self.number)
#3.定义测试用例,以“test_”开头命名的方法
#可使用unittest.TestCase类下面的各种断言方法用于对测试结果的判断
def test_case1(self):
print self.number
self.assertEqual(self.number,10,msg='Your input is not 10')
def test_case2(self):
print self.number
self.assertEqual(self.number,20,msg='Your input is not 20')
@unittest.skip('暂时跳过用例3的测试')
def test_case3(self):
print self.number
self.assertEqual(self.number,30,msg='Your input is not 30')
#4.定义tearDown()方法用于测试用例执行之后的善后工作。
def tearDown(self):
print 'Test over'
2、test suite /test runner
method 1:#直接运行该文
if __name__=='__main__':
unittest.main() ##搜索该模块下所有以test开头的测试用例方法,并自动执行它们。执行顺序是命名顺序
method 2: #添加多条测试用例
if __name__=='__main__':
#实例化测试套件
suite=unittest.TestSuite()
#将测试用例加载到测试套件中。执行顺序是安装加载顺序
suite.addTest(Test('test_case1'))
suite.addTest(Test('test_case2'))
runner=unittest.TextTestRunner()
#使用run()方法运行测试套件(即运行测试套件中的所有用例)
runner.run(suite)
if __name__=='__main__'
suite.addTest([Test('test_case1'),Test('test_case2')])
#执行测试用例
#实例化TextTestRunner类
runner=unittest.TextTestRunner()
#使用run()方法运行测试套件(即运行测试套件中的所有用例)
runner.run(suite)
method 3: 添加整个测试类
if __name__=='__main__':
suite=unittest.TestSuite(unittest.makeSuite(Test)) #Test为类名
runner=unittest.TextTestRunner()
runner.run(suite)
import '文件名'
if __name__=='___main_':
loader = unittest.TestLoader() # 创建一个加载对象
suite.addTest(loader.loadTestsFromTestCase(文件名.类名))
method 4: 添加整个模块
import test_register
if __name__='__main__'
loader = unittest.TestLoader() # 创建一个加载对象
suite.addTest(loader.loadTestsFromModule(test_register))
method5 : 运行文件中的测试用例(和方法4类似)
#简化了method 2中先要创建测试套件然后再依次加载测试用例)
if __name__=='__main__':
test_dir = './'
discover = unittest.defaultTestLoader.discover(test_dir, pattern='test_*.py')
#执行测试用例
#实例化TextTestRunner类
runner=unittest.TextTestRunner()
#使用run()方法运行测试套件(即运行测试套件中的所有用例)
runner.run(discover)
3 生成测试报告
3.1 HTMLTestRunner
test runner可以生成相应的测试报告。测试报告有两种展示形式,一种是text文本,一种是html格式。
html格式的就是HTMLTestRunner了, HTMLTestRunner 是 Python 标准库的 unittest 框架的一个扩展,它可以生成一个直观清晰的 HTML 测试报告。
使用的前提就是要载
HTMLTestRunner.py
,下载完后放在python的安装目录下的scripts目录下即可。
text文本相对于html来说过于简陋,与控制台输出的没有什么区别,也几乎没有人使用,这里不作演示,使用方法是一样的。我们结合前面的测试套件来演示一下如何生成html格式的测试报告:
from HTMLTestRunner import HTMLTestRunner ####下面这句替代上文中的
#####下面语句替代runner=unittest.TextTestRunner()
runner = HTMLTestRunner(stream=open("report.html", "wb"), # 打开一个报告文件,将句柄传给
stream tester="miki", # 报告中显示的测试人员
description="注册接口测试报告" # 报告中显示的描述信息
title="自动化测试报告") # 报告的标题
还有一个参数
* verbosity :表示测试报告信息的详细程度,一共三个值,默认是2
* 0 (静默模式):你只能获得总的测试用例数和总的结果,如:总共100个 失败10 成功90
* 1 (默认模式):类似静默模式,只是在每个成功的用例前面有个. 每个失败的用例前面有个F
* 2 (详细模式):测试结果会显示每个测试用例的所有相关的信息
3.2 BeautifulReport
from BeautifulReport import BeautifulReport
import unittest
# 1: 加载测试用例
def all_test():
case_path = os.path.join(cur_path, "test_case")
suite = unittest.TestLoader().discover(
start_dir=case_path,
pattern="test_04*.py",
top_level_dir=None)
return suite
# 2: 执行测试用例
def run():
result = BeautifulReport(all_test())
now = time.strftime("%Y_%m_%d_%H_%M_%S")
# 测试报告路径
file_name = 'SAIC_model_test' + now + "-report.html"
result.report(
filename=file_name,
report_dir=os.path.join(
cur_path,
'report'),
description='测试报告')
4、装饰器
unittest.skip():装饰器,当运行用例时,有些用例可能不想执行等,可用装饰器暂时屏蔽该条测试用例。一种常见的用法就是比如说想调试某一个测试用例,想先屏蔽其他用例就可以用装饰器屏蔽。
@unittest.skip(reason): skip(reason)装饰器:无条件跳过装饰的测试,并说明跳过测试的原因。
@unittest.skipIf(reason): skipIf(condition,reason)装饰器:条件为真时,跳过装饰的测试,并说明跳过测试的原因。
@unittest.skipUnless(reason): skipUnless(condition,reason)装饰器:条件为假时,跳过装饰的测试,并说明跳过测试的原因。
@unittest.expectedFailure(): expectedFailure()测试标记为失败。
代码如下:
class Test(unittest.TestCase):
@unittest.skip(u"无条件跳过此用例")
def test_1(self):
print("测试1")
@unittest.skipIf(True, u"为True的时候跳过")
def test_2(self):
print("测试2")
@unittest.skipUnless(False, u"为False的时候跳过")
def test_3(self):
print("测试3")
@unittest.expectedFailure
def test_4(self):
print("测试4")
self.assertEqual(2, 4, msg=u"判断相等")
if __name__ == '__main__':
unittest.main()
5、断言
常用:
在python基础中,我们有讲过一个assert断言,使用方法比较简单,即
assert 表达式, 提示信息
,而unittest框架中也提供了一个自带的断言方式,主要有以下几种:
方法
|
检查
|
assertEqual(a, b,msg=None)
|
a ==b
|
assertNotEqual(a, b)
|
a !=b
|
assertTrue(x)
|
bool(x) is True
|
assertFalse(x)
|
Bool(x) is False
|
assertIs(a, b)
|
a is b
|
assertIsNot(a, b)
|
a is not b
|
assertIsNone(x)
|
x is None
|
assertIsNotNone(x)
|
x is not None
|
assertIn(a, b)
|
a in b
|
assertNotIn(a, b)
|
a not in b
|
assertIsInstance(a, b)
|
isinstance(a,b)
|
assertNotIsInstance(a, b)
|
not isinstance(a,b)
|
如果断言失败即不通过就会抛出一个 AssertionError 断言错误,成功则标识为通过,以上几种方式都有一个共同点,就是都有一个msg参数(表中只列了一个,其实都有),默认是None,即 msg = None ,如果指定msg参数的值,则将该信息作为失败的错误信息返回。
assertAlmostEqual
(first, second[, places, ...])
|
适用于小数,
place
是应最少几位相等布尔值才为
1
(默认为
7
),如果在
place
位以内不同则断言失败。
|
assertDictContainsSubset
(expected, actual[, msg])
|
检查实际是否超预期
|
assertDictEqual
(d1, d2[, msg])
|
前后字典是否相同
|
assertEqual
(first, second[, msg])
|
前后两个数不等的话,失败
|
assertFalse
(expr[, msg])
|
检查表达式是否为假
|
assertGreater
(a, b[, msg])
|
和
self.assertTrue(a > b)
用法一样
,
但是多了设置条件
.
|
assertGreaterEqual
(a, b[, msg])
|
和
self.assertTrue(a >
=
b)
用法一样
,
但是多了设置条件
.
|
assertIn
(member, container[, msg])
|
self.assertTrue(a in b)
|
assertIs
(expr1, expr2[, msg])
|
assertTrue(a is b)
|
assertIsInstance
(obj, cls[, msg])
|
I
sinstance(a,b)
|
assertIsNone
(obj[, msg])
|
O
bj is none
.
|
assertIsNot
(expr1, expr2[, msg])
|
a is not b
.
|
assertIsNotNone
(obj[, msg])
|
O
bj is not none.
|
assertItemsEqual
(expected_seq, actual_seq[, msg])
|
一个无序的序列特异性的比较。
|
assertLess
(a, b[, msg])
|
Just like self.assertTrue(a < b), but with a nicer default message.
|
assertLessEqual
(a, b[, msg])
|
Just like self.assertTrue(a <= b), but with a nicer default message.
|
assertListEqual
(list1, list2[, msg])
|
L
ist1
与
list2
是否相等
.
|
assertMultiLineEqual
(first, second[, msg])
|
断言,
2
个多行字符串是相等的
|
assertNotAlmostEqual
(first, second[, ...])
|
Fail if the two objects are equal as determined by their difference rounded to the given number of decimal places (default 7) and comparing to zero, or by comparing that the between the two objects is less than the given delta.
|
assertNotAlmostEquals
(first, second[, ...])
|
Fail if the two objects are equal as determined by their difference rounded to the given number of decimal places (default 7) and comparing to zero, or by comparing that the between the two objects is less than the given delta.
|
assertNotEqual
(first, second[, msg])
|
Fail if the two objects are equal as determined by the ‘==’
|
assertNotEquals
(first, second[, msg])
|
Fail if the two objects are equal as determined by the ‘==’
|
assertNotIn
(member, container[, msg])
|
Just like self.assertTrue(a not in b), but with a nicer default message.
|
assertNotIsInstance
(obj, cls[, msg])
|
Included for symmetry with assertIsInstance.
|
assertNotRegexpMatches
(text, unexpected_regexp)
|
如果文本匹配正则表达式,将失败。
|
assertRaises
(excClass[, callableObj])
|
除非
excclass
类抛出异常失败
|
assertRaisesRegexp
(expected_exception, ...)
|
认为在引发异常的情况下消息匹配一个正则表达式。
|
assertRegexpMatches
(text, expected_regexp[, msg])
|
测试失败,除非文本匹配正则表达式。
|
assertSequenceEqual
(seq1, seq2[, msg, seq_type])
|
有序序列的相等断言
(like lists and tuples).
|
assertSetEqual
(set1, set2[, msg])
|
A set-specific equality assertion.
|
assertTrue
(expr[, msg])
|
Check that the expression is true.
|
assertTupleEqual
(tuple1, tuple2[, msg])
|
A tuple-specific equality assertion.
|
assert_
(expr[, msg])
|
Check that the expression is true.
|