#全局操作
#查看集群健康状况
GET /_cat/health?v
#查看节点情况
GET /_cat/nodes?v
#查看各个索引状态
GET /_cat/indices?v
#创建索引 PUT 索引名?pretty
PUT /movie_index
#删除索引
DELETE /movie_index
#查看某索引的分片情况
GET /_cat/shards/movie_index
#创建文档
PUT /movie_index/movie/1
{ "id":100,
"name":"operation red sea",
"doubanScore":8.5,
"actorList":[
{"id":1,"name":"zhang yi"},
{"id":2,"name":"hai qing"},
{"id":3,"name":"zhang han yu"}
]
}
PUT /movie_index/movie/2
{
"id":200,
"name":"operation meigong river",
"doubanScore":8.0,
"actorList":[
{"id":3,"name":"zhang han yu"}
]
}
PUT /movie_index/movie/3
{
"id":300,
"name":"incident red sea",
"doubanScore":5.0,
"actorList":[
{"id":4,"name":"zhang san feng"}
]
}
#查询某个索引中全部文档 size控制个数
GET /movie_index/_search
{
"size":3
}
#根据文档ID查看文档
GET /movie_index/movie/1?pretty
#根据文档ID删除文档
DELETE /movie_index/movie/3
#删除后并不是真的删除,新增记录标记删除,需要合并
#也可以手动执行 进行合并触发
POST /_forcemerge
#put 对已经存在的文档进行替换
PUT /movie_index/movie/3
{
"id":300,
"name":"incident red sea",
"doubanScore":5.0,
"actorList":[
{"id":4,"name":"zhang cuishan"}
]
}
#post新增操作,无法保证幂等性
#根据主键保证幂等性
#根据文档 id 更新文档 doc 固定写法
POST /movie_index/movie/3/_update?pretty
{
"doc": {"name":"wudang"}
}
#根据条件更新文档
POST /movie_index/_update_by_query
{
"query": {
"match":{
"actorList.id":1
}
},
"script": {
"lang": "painless",
"source":"for(int i=0;i<ctx._source.actorList.length;i++){if(ctx._source.actorList[i].id==1){ctx._source.actorList[i].name='tttt'}}"
}
}
#删除文档属性
POST /movie_index/movie/1/_update
{
"script" : "ctx._source.remove('name')"
}
#根据条件删除文档
POST /movie_index/_delete_by_query
{
"query":{
"match": {"id":100}
}
}
#根据条件删除文档
POST /movie_index/_delete_by_query
{
"query":{
"match_all": {}
}
}
GET /movie_index/_search
#批处理 在索引中批量创建两个文档
POST /movie_index/movie/_bulk
{"index":{"_id":66}}
{"id":300,"name":"incident red sea","doubanScore":5.0,"actorList":[{"id":4,"name":"zhang cuishan"}]}
{"index":{"_id":88}}
{"id":300,"name":"incident red sea","doubanScore":5.0,"actorList":[{"id":4,"name":"zhang cuishan"}]}
#批处理 在一个批量操作中,先更新第一个文档(ID 为 66),再删除第二个文档(ID 为 88)
pOST /movie_index/movie/_bulk
{"update":{"_id":"66"}}
{"doc": { "name": "wudangshanshang" } }
{"delete":{"_id":"88"}}
#----------------------------------查询操作
#查询出当前索引全部数据
GET /movie_index/_search?q=_id:66
GET /movie_index/_search
#根据电影名字进行查询
GET /movie_index/_search
{
"query": {
"match": {
"name": "operation red sea"
}
}
}
#按照分词子属性进行查询
GET /movie_index/_search
{
"query": {
"match": {
"actorList.name": "zhang han yu"
}
}
}
#按短语查询(相当于like %%)
GET /movie_index/_search
{
"query": {
"match_phrase": {
"actorList.name": "zhang han yu"
}
}
}
#不分词,通过精准搜索匹配,term精准匹配,使用keyword完成
GET /movie_index/_search
{
"query": {
"term": {
"actorList.name.keyword": {
"value": "zhang han yu"
}
}
}
}
#容错匹配
GET /movie_index/_search
{
"query": {
"fuzzy": {
"name": "operati"
}
}
}
#过滤,先匹配,再过滤
GET /movie_index/_search
{
"query": {
"match": {
"name": "red"
}
}
,"post_filter": {
"term": {
"actorList.id": 3
}
}
}
#过滤-推荐使用 匹配和过滤同时进行
GET /movie_index/_search
{
"query": {
"bool": {
"must": [
{"match": {
"name": "red"
}}
],
"filter": {
"term": {
"actorList.id": "3"
}
}
}
}
}
#范围查询 豆瓣评分在6-9之间的查询出来
GET /movie_index/_search
{
"query": {
"range": {
"doubanScore": {
"gte": 6,
"lte": 9
}
}
}
}
#按照豆瓣评分降序排序
GET /movie_index/_search
{
"query": {
"match": {
"name": "red"
}
},
"sort": [
{
"doubanScore": {
"order": "desc"
}
}
]
}
#分页查询 从什么开始,显示几条
GET /movie_index/_search
{
"from": 0,
"size": 2
}
#查询指定字段
GET /movie_index/_search
{
"_source": ["id","name"]
}
#高亮,对命中的词进行高亮显示
GET /movie_index/_search
{
"query": {
"match": {
"name": "red"
}
},
"highlight": {
"fields": {"name": {}},
"pre_tags": "<a>",
"post_tags": "</a>"
}
}
#--------------聚合函数----------------
#需求一,取出每个演员共参演了多少电影
#aggs 表示聚合
#term 精准匹配
#terms 聚合操作,相当于group by
GET /movie_index/movie/_search
{
"aggs": {
"myAggs": {
"terms": {
"field": "actorList.name.keyword",
"size": 10
}
}
}
}
#需求二,每个演员参演电影的平均分,并按评分排序
GET /movie_index/_search
{
"aggs": {
"groupByname": {
"terms": {
"field": "actorList.name.keyword",
"size": 10
, "order": {
"avg_score": "asc"
}
},
"aggs": {
"avg_score": {
"avg": {
"field": "doubanScore"
}
}
}
}
}
}
}
#中文分词器 ik_smart 简易分词器
#ik_max_word
GET /_analyze
{
"text": "无卡卡",
"analyzer": "ik_max_word"
}
#mapping
#直接创建Document,建立文档的时候自动创建index,同时mapping会自动定义
PUT /movie_chn_1/movie/1
{ "id":1,
"name":"红海行动",
"doubanScore":8.5,
"actorList":[
{"id":1,"name":"张译"},
{"id":2,"name":"海清"},
{"id":3,"name":"张涵予"}
]
}
PUT /movie_chn_1/movie/2
{
"id":2,
"name":"湄公河行动",
"doubanScore":8.0,
"actorList":[
{"id":3,"name":"张涵予"}
]
}
PUT /movie_chn_1/movie/3
{
"id":3,
"name":"红海事件",
"doubanScore":5.0,
"actorList":[
{"id":4,"name":"张三丰"}
]
}
GET /movie_chn_1/_search
#查看自动定义的mapping
GET /movie_chn_1/_mapping
GET /movie_chn_1/movie/_search
{
"query": {
"match": {
"name": "海行"
}
}
}
#基于中文分词搭建索引-手动定义 mapping
#定义 Index,指定 mapping
PUT movie_chn_2
{
"mappings": {
"movie":{
"properties": {
"id":{
"type": "long"
},
"name":{
"type": "text",
"analyzer": "ik_smart"
},
"doubanScore":{
"type": "double"
},
"actorList":{
"properties": {
"id":{
"type":"long"
},
"name":{
"type":"keyword"
}
}
}
}
}
}
}
#向 Index 中放入 Document
PUT /movie_chn_2/movie/1
{ "id":1,
"name":"红海行动",
"doubanScore":8.5,
"actorList":[
{"id":1,"name":"张译"},
{"id":2,"name":"海清"},
{"id":3,"name":"张涵予"}
]
}
PUT /movie_chn_2/movie/2
{
"id":2,
"name":"湄公河行动",
"doubanScore":8.0,
"actorList":[
{"id":3,"name":"张涵予"}
]
}
PUT /movie_chn_2/movie/3
{
"id":3,
"name":"红海事件",
"doubanScore":5.0,
"actorList":[
{"id":4,"name":"张三丰"}
]
}
GET /movie_chn_2/movie/_search
{
"query": {
"match": {
"name": "海行"
}
}
}
#索引数据拷贝
POST _reindex
{
"source": {
"index": "my_index_name"
},
"dest": {
"index": "my_index_name_new"
}
}
PUT 索引名
{
"aliases": {
"索引别名": {}
}
}
#创建索引的时候,手动 mapping,并指定别名
PUT movie_chn_3
{
"aliases": {
"movie_chn_3_aliase": {}
},
"mappings": {
"movie":{
"properties": {
"id":{
"type": "long"
},
"name":{
"type": "text",
"analyzer": "ik_smart"
},
"doubanScore":{
"type": "double"
},
"actorList":{
"properties": {
"id":{
"type":"long"
},
"name":{
"type":"keyword"
}
}
}
}
}
}
}
GET /movie_chn_3_a2/_search
#为已存在的索引增加别名
POST _aliases
{
"actions": [
{ "add":{ "index": "索引名", "alias": "索引别名" }}
]
}
#给 movie_chn_3 添加别名
POST _aliases
{
"actions": [
{ "add":{ "index": "movie_chn_3", "alias": "movie_chn_3_a2" }}
]
}
#查询别名列表
GET _cat/aliases?v
#删除某个索引的别名
POST _aliases
{
"actions": [
{ "remove": { "index": "movie_chn_3", "alias": "movie_chn_3_a2" }}
]
}
#使用场景-给多个索引分组
POST _aliases
{
"actions": [
{ "add": { "index": "movie_chn_1", "alias": "movie_chn_query" }},
{ "add": { "index": "movie_chn_2", "alias": "movie_chn_query" }}
]
}
GET movie_chn_query/_search
#给索引的一个子集创建视图
POST _aliases
{
"actions": [
{
"add":
{
"index": "movie_chn_1",
"alias": "movie_chn_1_sub_query",
"filter": {
"term": { "actorList.id": "4"}
}
}
}
]
}
GET movie_chn_1_sub_query/_search
#在运行的集群中可以无缝的从一个索引切换到另一个索引
POST /_aliases
{
"actions": [
{ "remove": { "index": "movie_chn_1", "alias": "movie_chn_query" }},
{ "remove": { "index": "movie_chn_2", "alias": "movie_chn_query" }},
{ "add": { "index": "movie_chn_3", "alias": "movie_chn_query" }}
]
}
#创建索引模板
PUT _template/template_movie2020
{
"index_patterns": ["movie_test*"],
"settings": {
"number_of_shards": 1
},
"aliases" : {
"{index}-query": {},
"movie_test-query":{}
},
"mappings": {
"_doc": {
"properties": {
"id": {
"type": "keyword"
},
"movie_name": {
"type": "text",
"analyzer": "ik_smart"
}
}
}
}
}
#测试 向索引中添加数据
POST movie_test_202011/_doc
{
"id":"333",
"name":"zhang3"
}
GET /_cat/aliases
GET movie_test_202011-query/_mapping
GET movie_test_202011/_search
# 查看系统中已有的模板清单
GET /_cat/templates
#查看某个模板详情
GET _template/template_movie2020
#或者
GET _template/template_movie*
ES操作命令
最新推荐文章于 2024-10-14 11:59:05 发布
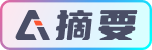