在Android开发中,有一些控件布局往往会多次用到,这时就可以用自定义view的方法对其进行封装,以便复用。下面以登录页面中的输入框为例进行介绍~
1 自定义属性
- 在res中的values目录下新建一个资源文件并命名为attrs.xml,用来存放我们自定义的属性
- 确定自定义控件中可配置的属性,以输入框为例,即为icon:输入框前面的图标 hint:输入框里的提示 password:是否需要密文显示
- 在attrs文件中声明样式,format里面的内容表示该属性接收什么类型的内容
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- 声明样式-->
<declare-styleable name="inputView">
<!-- icon:输入框前面的图标 hint:输入框里的提示 password:是否需要密文显示 -->
<attr name="input_icon" format="reference"></attr>
<attr name="input_hint" format="string"></attr>
<attr name="is_password" format="boolean"></attr>
</declare-styleable>
</resources>
2 自定义控件
在定义完属性之后需要在自定义控件中使用提供的若干可配置属性,具体步骤如下:
-
新建一个Java文件,命名为InputView.java,表示我们自定义的控件
-
让InputView继承FrameLayout,并重写所有的构造方法
-
新建布局文件,命名为input_view.xml,包含ImageView和EditText
-
在InputView类中新建一个init方法,用来初始化,并在构造方法中调用init方法
其中init方法所应包含的内容:(1)获取自定义属性:调用context.obtainStyledAttributes方法,第二个参数是我们自定义的输入控件的属性样式名,即R.styleable.inputView
(2)绑定layout布局:传入之前创建的input_view布局
(3)将布局与属性相关联 -
在activity_login.xml主布局文件中调用之即可
input_view.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:gravity="center_vertical"
android:layout_width="match_parent"
android:layout_height="44dp"
android:paddingLeft="@dimen/marginSize"
android:paddingRight="@dimen/marginSize">
<ImageView
android:id="@+id/iv_icon"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/user"/>
<EditText
android:id="@+id/et_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="用户名"
android:background="@null"
android:paddingRight="@dimen/marginSize"
android:paddingLeft="@dimen/marginSize"
android:textSize="18sp"/>
</LinearLayout>
InputView.java代码
public class InputView extends FrameLayout {
private int inputIcon;
private String inputHint;
private boolean isPassword;
private View mView;
private ImageView ivIcon;
private EditText etInput;
public InputView(@NonNull Context context) {
super(context);
init(context,null);
}
public InputView(@NonNull Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
init(context,attrs);
}
public InputView(@NonNull Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init(context,attrs);
}
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP)
public InputView(@NonNull Context context, @Nullable AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
init(context,attrs);
}
/**
* 初始化方法
* @param context
* @param attrs
*/
private void init(Context context,AttributeSet attrs){
if(attrs==null) return;
//获取自定义属性
TypedArray typedArray=context.obtainStyledAttributes(attrs, R.styleable.inputView);
inputIcon=typedArray.getResourceId(R.styleable.inputView_input_icon,R.mipmap.ic_launcher);
inputHint=typedArray.getString(R.styleable.inputView_input_hint);
isPassword=typedArray.getBoolean(R.styleable.inputView_is_password,false);
typedArray.recycle();
//绑定layout布局
mView= LayoutInflater.from(context).inflate(R.layout.input_view,this,false);
ivIcon=mView.findViewById(R.id.iv_icon);
etInput=mView.findViewById(R.id.et_input);
//布局关联属性
ivIcon.setImageResource(inputIcon);
etInput.setHint(inputHint);
etInput.setInputType(isPassword? InputType.TYPE_CLASS_TEXT|InputType.TYPE_TEXT_VARIATION_PASSWORD:InputType.TYPE_CLASS_TEXT);
addView(mView);
}
/**
* 返回输入内容
* @return
*/
public String getInput(){
return etInput.getText().toString().trim();
}
}
activity_login.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<include layout="@layout/nav_bar"/>
<de.hdodenhof.circleimageview.CircleImageView
android:id="@+id/default_picture"
android:layout_width="150dp"
android:layout_height="150dp"
android:layout_marginTop="@dimen/marginSize"
android:layout_gravity="center_horizontal"
android:src="@drawable/logo" />
<com.example.viewdefinedtest.view.InputView
android:layout_width="match_parent"
android:layout_height="44dp"
android:layout_marginTop="@dimen/marginSize"
app:input_icon="@drawable/user"
app:input_hint="用户名"
app:is_password="false">
</com.example.viewdefinedtest.view.InputView>
<View style="@style/line" />
<com.example.viewdefinedtest.view.InputView
android:layout_width="match_parent"
android:layout_height="44dp"
android:layout_marginTop="@dimen/marginSize"
app:input_icon="@drawable/password"
app:input_hint="密码"
app:is_password="true">
</com.example.viewdefinedtest.view.InputView>
<View style="@style/line" />
<Button
style="@style/commitBtn"
android:text="登录"
android:layout_marginTop="@dimen/marginSize"/>
</LinearLayout>
注:里面的button和分割线line也是自定义样式,这里不重点讲
到此,就完成了自定义输入控件了。
(ps:该内容的学习来自于慕课网~)
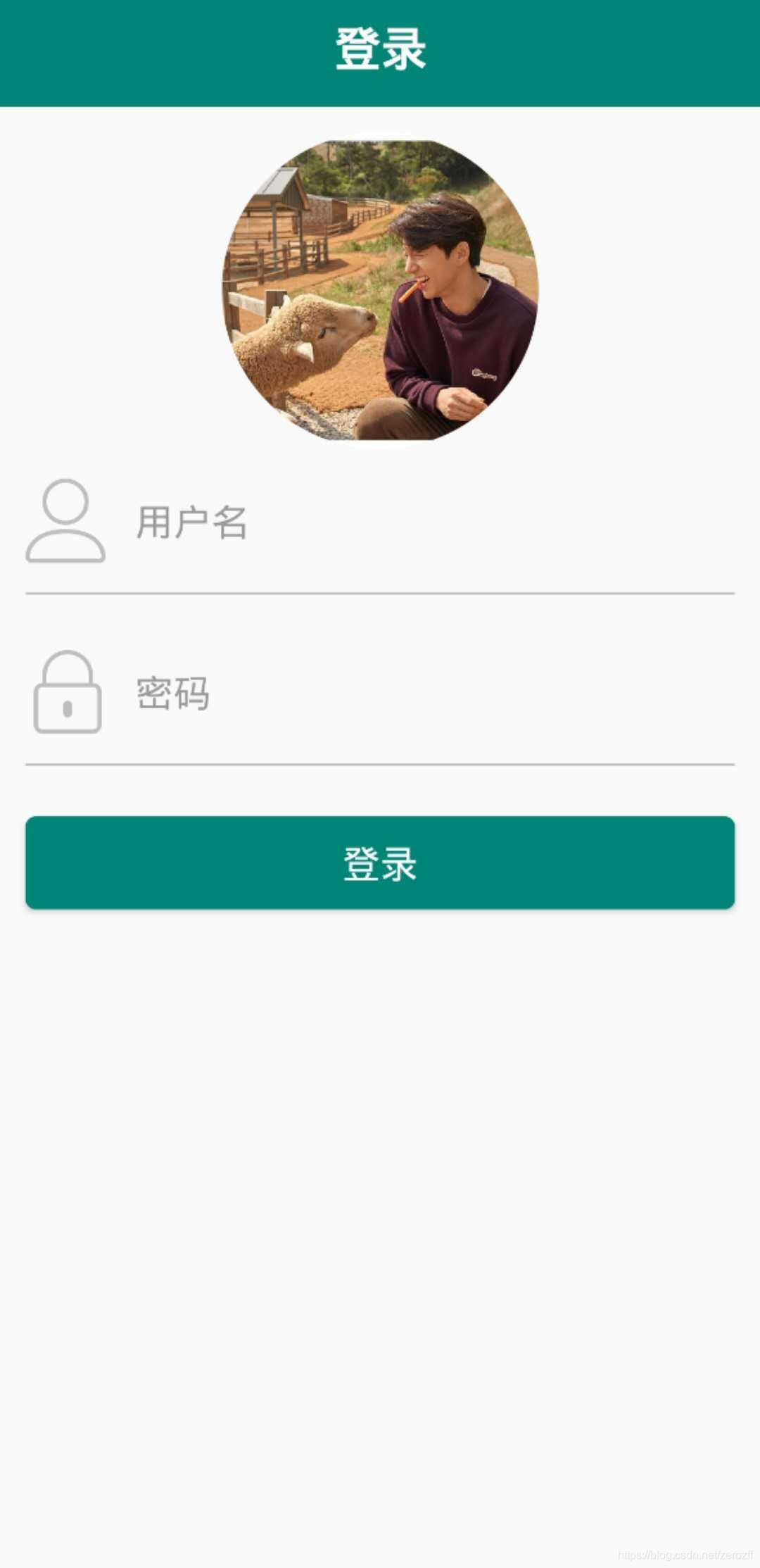