#include <iostream>
using namespace std;
class mystring {
public:
//无参构造函数
mystring()
{
len=0;
str=nullptr;
}
//析构函数
~mystring()
{
if(str!=nullptr){
delete []str;
}
}
//有参构造函数
mystring(const char *_str)
{
len=mystrlen(_str);
str=new char[len+1];
mystrcpy(str,_str);
}
//拷贝构造函数
mystring(const mystring &operation)
{
len=operation.len;
str=new char[len+1];
mystrcpy(str,operation.str);
}
//拷贝复制函数
mystring &operator=(const mystring &operation)
{
if(this!=&operation){
if(str!=nullptr){
delete [](this->str);
}
len=operation.len;
str=new char[len+1];
mystrcpy(str,operation.str);
}
return *this;
}
//+运算符重载
const mystring &operator+(const mystring &operation)
{
char *p=new char[len+operation.len+1];
mystrcpy(p,str);
mystrcat(p,operation.str);
delete []str;
str=p;
len=mystrlen(str);
return *this;
}
//+=运算符重载
const mystring &operator+=(const mystring &operation)
{
char *p=new char[len+operation.len+1];
mystrcpy(p,str);
mystrcat(p,operation.str);
delete []str;
str=p;
len=mystrlen(str);
return *this;
}
//>运算符重载
bool operator>(const mystring &operation)
{
if(mystrcmp(this->str,operation.str)>0)
return true;
else
return false;
}
//>=运算符重载
bool operator>=(const mystring &operation)
{
if(mystrcmp(this->str,operation.str)>=0)
return true;
else
return false;
}
//<运算符重载
bool operator<(const mystring &operation)
{
if(mystrcmp(this->str,operation.str)<0)
return true;
else
return false;
}
//<=运算符重载
bool operator<=(const mystring &operation)
{
if(mystrcmp(this->str,operation.str)<=0)
return true;
else
return false;
}
//==运算符重载
bool operator==(const mystring &operation)
{
if(mystrcmp(this->str,operation.str)==0)
return true;
else
return false;
}
//!=运算符重载
bool operator!=(const mystring &operation)
{
if(mystrcmp(this->str,operation.str)!=0)
return true;
else
return false;
}
//[]去成员运算符重载
char operator[](const int index) const
{
return (this->str)[index];
}
//<<取值运算符重载
friend ostream &operator<<(ostream &,mystring &);
bool myEmpty()
{
return str==nullptr?true:false;
}
int mySize()
{
return len;
}
const char *myStr()
{
return (const char *)str;
}
private:
char *str;
int len;
char *mystrcpy(char *dest,const char *src)
{
char *ch=dest;
while(*src!='\0'){
*(ch++)=*(src++);
}
*ch='\0';
return dest;
}
int mystrlen(const char *src)
{
const char *ch=src;
while(*ch!='\0'){
ch++;
}
return ch-src;
}
char *mystrcat(char *dest,const char *src)
{
char *ch=dest;
while(*(++ch)!='\0');
while(*src!='\0'){
*(ch++)=*(src++);
}
*ch='\0';
return dest;
}
int mystrcmp(const char *dest,const char *src)
{
while(*dest!='\0' && *src!='\0' && *dest==*src){
dest++;
src++;
}
return *dest-*src;
}
};
ostream &operator<<(ostream &output,mystring &operation)
{
output<<operation.str;
return output;
}
int main()
{
mystring s1("hello"),s2(" world"),s3;
s3=s1+s2;
s1+=s2;
cout<<s1<<endl;
cout<<s3<<endl;
cout<<(s1>s3?"s1>s3":"s1<=s3")<<endl;
cout<<(s1==s3?"s1==s3":"s1!=s3")<<endl;
cout<<(s1<=s3?"s1<=s3":"s1>s3")<<endl;
cout<<s2[3]<<endl;
cout<<s2<<endl;
return 0;
}
2022.8.24 作业
于 2022-08-24 19:09:25 首次发布
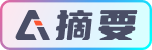