import java.util.Stack;
public class Iterator1
{
//先序遍历
public void preIterator(BiTree root)
{
if(root == null)
return;
Stack<BiTree> stack = new Stack<BiTree>();
BiTree p = root;
while(!stack .empty() || p != null )
{
while(p != null)
{
System.out.print(p.val + "-->");//若节点不为空先访问再压栈(每个节点都可以看成根节点)
stack.push(p);
p = p.left;//将当前节点置为p的左孩子,若不为空继续访问并压栈
}
//当p为空时,说明根节点和左孩子打印遍历完毕了,接下来出栈遍历右孩子
if(!stack.empty())
{
p = stack.pop();
p = p.right;
}
}
}
//中序遍历
public void inIterator(BiTree root)
{
if(root == null)
return;
Stack<BiTree> stack = new Stack<BiTree>();
BiTree p = root;//让p指向根节点
while(!stack .empty() || p != null )
{
//一直遍历到左子树最下边,边遍历边保存根节点到栈中(每个节点都可以看成一个新的子树的根节点)
while(p != null)
{
stack.push(p);//若节点的左孩子不为空,将左孩子压栈,因为需要借助遍历过的节点进入右子树
p = p.left;
}
//当p为空时,说明已经到达左子树最下边,这时需要出栈了
if(stack != null)
{
p = stack.pop();
System.out.print(p.val + "-->");//访问根节点
p = p.right;//进入右子树,此时p是右子树的根节点(开始新一轮的遍历)
}
}
}
//后序遍历
public void postIterator(BiTree root)
{
if(root == null)
return;
Stack<BiTree> stack = new Stack<BiTree>();
BiTree pre = null;//当前节点的之前访问的节点
BiTree current;
stack.push(root);
while(!stack.empty())
{
current = stack.peek();
if((current.left == null && current.right == null) || //当前节点是叶子节点,可以直接访问该节点
(pre != null &&(pre == current.left|| pre == current.right)))
//当前一个节点不为空并且是当前节点的左孩子或者右孩子,当是左孩子时说明当前节点右孩子为空,当是右孩子时,说明左右孩子都访问过了,且都不为空
{
System.out.print(current.val + "-->");
stack.pop();
pre = current;
}
else //当前节点为栈顶元素 如果当前节点不是叶子节点,在当前节点之前访问的那个节点不是当前节
二叉树的非递归遍历实现
最新推荐文章于 2020-10-27 09:53:11 发布
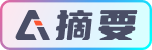