参考来源:点击打开链接
本文主要分为以下几个部分:
- Numpy--数组域的数组 (Array from numerical ranges)
- Numpy--索引和分片 (Indexing & slicing)
- Numpy--高级索引 (Advanced indexing)
- Numpy--广播(Broadcasting)
- Numpy--数组上的迭代 (Iterating over array)
- Numpy--数组处理 (Array manipulation)
一. Numpy --- 数值域的数组
本部分主要介绍如何从数值域创建数组:
代码及注释如下:
a1 = np.arange(3, 18, 3, dtype=float)
print('a1 = ', a1)
a2 = np.linspace(1, 2, 5, endpoint=False, retstep=True)
print('a2 = ', a2)
a3 = np.logspace(1.0, 2.0, num=10, base=2)
print('a3 = ', a3)
运行结果:

二. Numpy --- 索引和分片
可以通过 ‘索引’ 和 ‘分片’ 来更改ndarray的对象内容。
Three types of indexing methods are available − field access, basic slicing and advanced indexing.
实现索引的三种基本方法为:字段访问,基本分片和高级索引
分片是为了获取数组中的一部分
A Python slice object is constructed by giving start, stop, and step parameters to the built-in slice function. This slice object is passed to the array to extract a part of array.
代码及注释如下所示:
a1 = np.arange(10)
s1 = slice(2, 7, 2)
s2 = a1[2: 7: 2]
s3 = a1[5]
s4 = a1[5:]
s5 = a1[5:8]
print('s1 = ', s1, '\ns2 = ', s2, '\ns3 = ', s3, '\ns4 = ', s4, '\ns5 = ', s5)
a2 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print('a2 = ', a2, )
print(a2[1:])
print(a2[..., 1])
print(a2[1, ...])
print(a2[..., 1:])
运行结果:
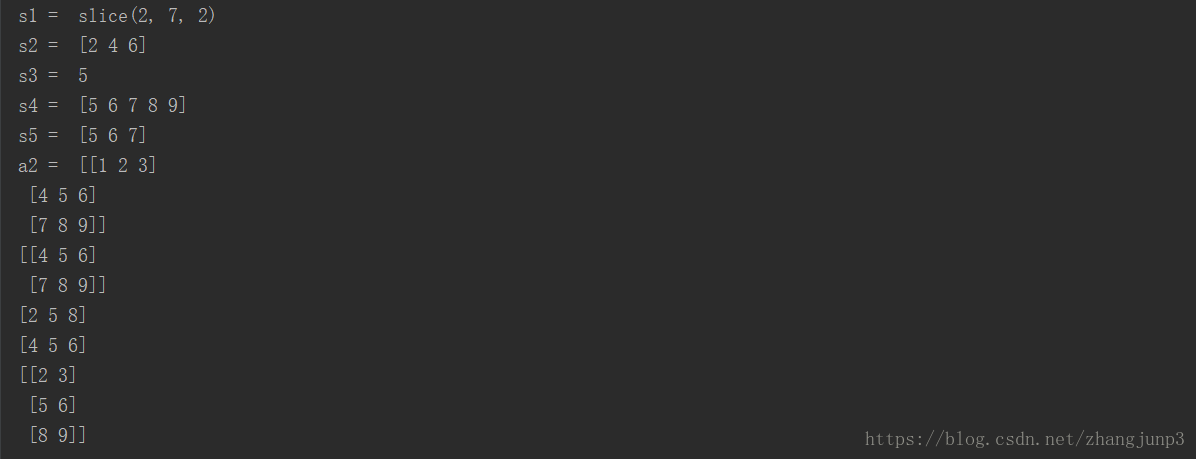
三. Numpy --- 高级索引
这一部分,我将介绍关于非元组序列,整形,布尔型,或至少包含一个序列元素的元组的索引。
Advanced indexing always returns a copy of the data. As against this, the slicing only presents a view.
高级索引包括整型和布尔型(Integer and Boolean)。
- 整型索引
实现:是基于 N 维索引来获取数组中任意元素。 每个整数数组表示该维度的下标值。
Selecting any arbitrary item in an array based on its Ndimensional index. Each integer array represents the number of indexes into that dimension.
代码以及注释如下:
a1 = np.array([[1, 2], [3, 4], [5, 6]])
print('a1 = ', a1)
selection = a1[[0, 1, 2], [0, 1, 0]]
print('\n', selection)
a2 = np.array([[0, 1, 2], [3, 4, 5], [6, 7, 8], [9, 10, 11]])
print('a2 = ', a2)
# Select rows and columns
Rows = np.array([[0, 0], [3, 3]])
Cols = np.array([[0, 2], [0, 2]])
print(' \n', a2[Rows, Cols])
print('\n', a2[1:4, 1:3])
print(' \n', a2[1:4, [1, 2]])
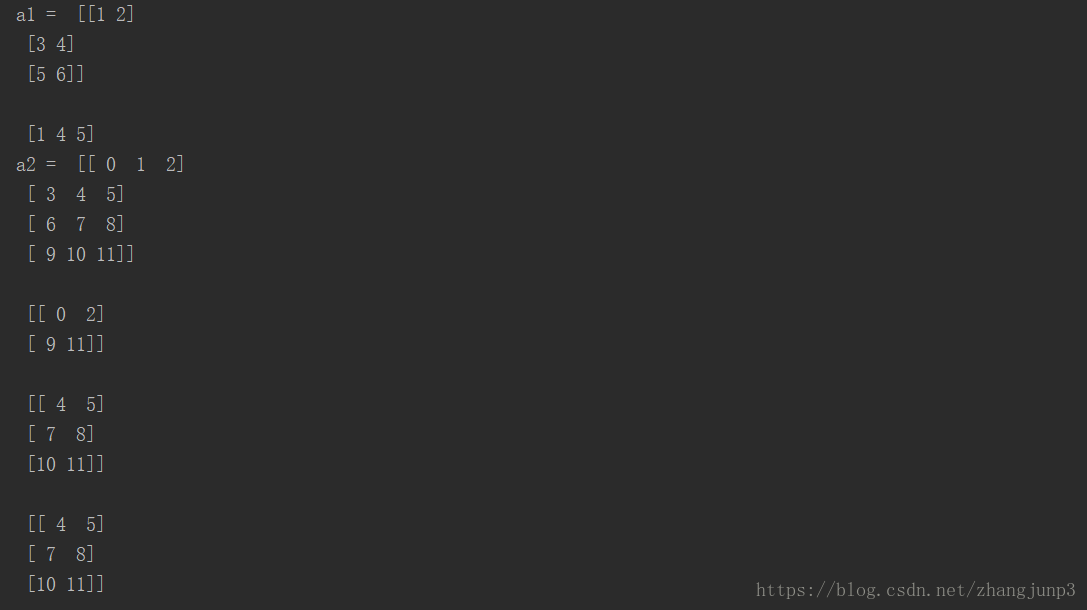
使用条件:
Used when the resultant object is meant to be the result of Boolean operations, such as comparison operators.
代码以及注释如下:
a1 = np.array([[0, 1, 2], [3, 4, 5], [6, 7, 8], [9, 10, 11]])
print('a1 = \n', a1)
print(' \n', a1[a1>5])
a2 = np.array([8, 8+9j, 9, 9+8j])
print('Complex elements in an array are: \n', a2[np.iscomplex(a2)])
运行结果如下:
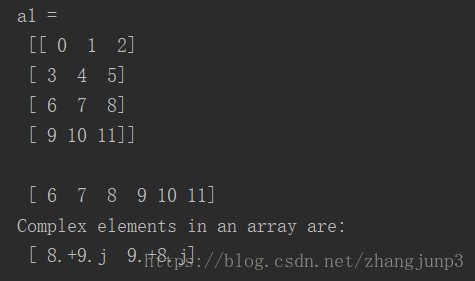
四. Numpy --- 广播
术语(Broadcasting)是指Numpy在算数运算期间可以处理不同形状数组的能力。
The ability of NumPy to treat arrays of different shapes during arithmetic operations.
代码以及注释如下:
a1 = np.array([1, 2, 3, 4])
a2 = np.array([10, 20, 30, 40])
print('similar-shape array multiply = \n', a1*a2)
a = np.array([[0.0, 0.0, 0.0], [10.0, 10.0, 10.0], [20.0, 20.0, 20.0], [30.0, 30.0, 30.0]])
b = np.array([1.0, 2.0, 3.0])
print('First array : \n', a)
print('Second array : \n', b)
print('First Array + Second Array: \n', a+b)
运行结果:
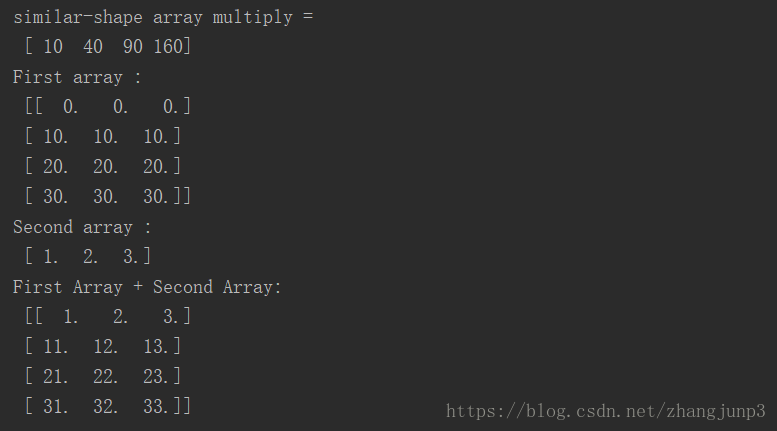
原理:
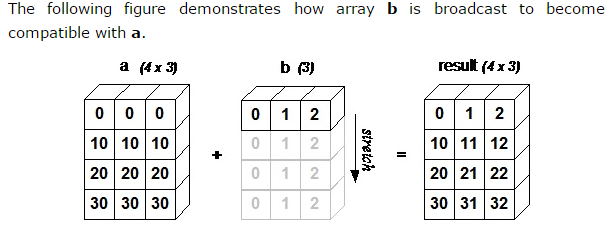
实现Broadcasting广播需要遵循的规则为:
- Array with smaller ndim than the other is prepended with '1' in its shape.
- Size in each dimension of the output shape is maximum of the input sizes in that dimension.
- An input can be used in calculation, if its size in a particular dimension matches the output size or its value is exactly 1.
- If an input has a dimension size of 1, the first data entry in that dimension is used for all calculations along that dimension.
若上述规则产生有效结果,并且满足以下条件之一,那么数组被称为可广播的。
- Arrays have exactly the same shape.
- Arrays have the same number of dimensions and the length of each dimension is either a common length or 1.
- Array having too few dimensions can have its shape prepended with a dimension of length 1, so that the above stated property is true.
五. Numpy --- 数组上的迭代
Numpy包含一个迭代对象:numpy.nditer. 可以实现多维数组的迭代。
代码以及注释如下:
a1 = (np.arange(0, 60, 5)).reshape(3, 4)
print('Original array is :\n', a1)
print('Array after iter: \n')
for x in np.nditer(a1):
print(x, end=' ')
print('\nTranspose of the original array a1 is : \n', a1.T)
print('Array after iter :\n')
for y in np.nditer(a1.T):
print(y, end=' ')
运行结果:
- 更改数组值
nditer的另一个可选的参数为op_flags,其默认值为只读(read-only),但是(read-only)或者(write-only)的模式是可以切换的。这样就可以实现通过迭代来改变数组中的元素值。
This will enable modifying array elements using this iterator.
代码以及注释如下所示:
a1 = (np.arange(0, 60, 5)).reshape(3,4)
print('Original array is :\n', a1)
for x in np.nditer(a1, op_flags=['readwrite']):
x[...] = 2*x
print('Modified array is : \n', a1)
运行结果为:
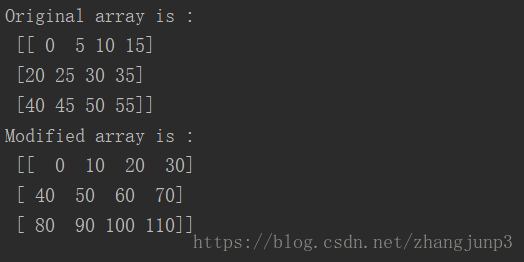
- 外部循环
关于Numpy的flags参数,可以更改以下值:
- c_index --- C_order index can be racked
- f_index --- Fortran_order index is tracked
- multi-index --- Type of indexes with one per iteration can be tracked
- external_loop --- Causes values given to be one-dimensional arrays with multiple values instead of zero-dimensional array
代码以及注释如下所示:
a1 = (np.arange(0, 60, 5)).reshape(3, 4)
print('Original array is :\n', a1)
print('Modified array is: \n')
for x in np.nditer(a1, flags=['external_loop'], order='F'):
print(x, end=' ')
运行结果:
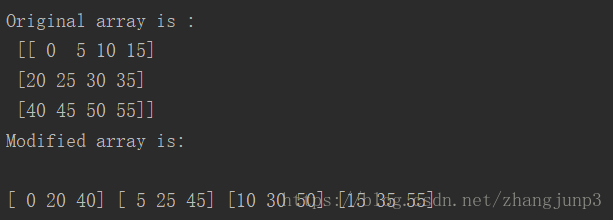
六. Numpy --- 数组处理
- 修改形状 (Changing shape)
- reshape() --- Gives a new shape to an array without changing its data
- flat() --- A 1-D iterator over the array
- flatten() --- Returns a copy of the array collapsed into one dimension
- ravel() --- Returns a contiguous flattened array
- 转置操作 (Transpose operation)
- transpose() --- Permutes the dimensions of an array
- ndarray.T() --- Same as self.transpose()
- rollaxis() --- Rolls the specified axis backwards
- swapaxes() --- Interchanges the two axes of an array
- 修改维度 (Changing dimensions)
- broadcast() --- Produces an object that mimics broadcasting
- broadcast_to() --- Broadcasts an array to a new shape
- expand_dims() --- Expands the shape of an array
- squeeze() --- Removes single-dimensional entries from the shape of an array
- 数组连接(Joining arrays)
- concatenate() --- Joins a sequence of arrays along an existing axis
- stack() --- Joins a sequence of arrays along a new axis
- hstack() --- Stacks arrays in sequence horizontally (column wise)
- vstack() --- Stacks arrays in sequence vertically (row wise)
- 数组分割(Splitting arrays)
- split() --- Splits an array into multiple sub-arrays
- hsplit() --- Splits an array into multiple sub-arrays horizontally (column-wise)
- vsplit() --- Splits an array into multiple sub-arrays vertically (row-wise)
- 添加、删除元素(Adding/removing elements)
- resize() --- Returns a new array with the specified shape
- append() --- Appends the values to the end of an array
- insert() --- Inserts the values along the given axis before the given indices
- delete() --- Returns a new array with sub-arrays along an axis deleted
- unique() --- Finds the unique elements of an array