前端电商商城项目分页器的原生js封装与使用详解

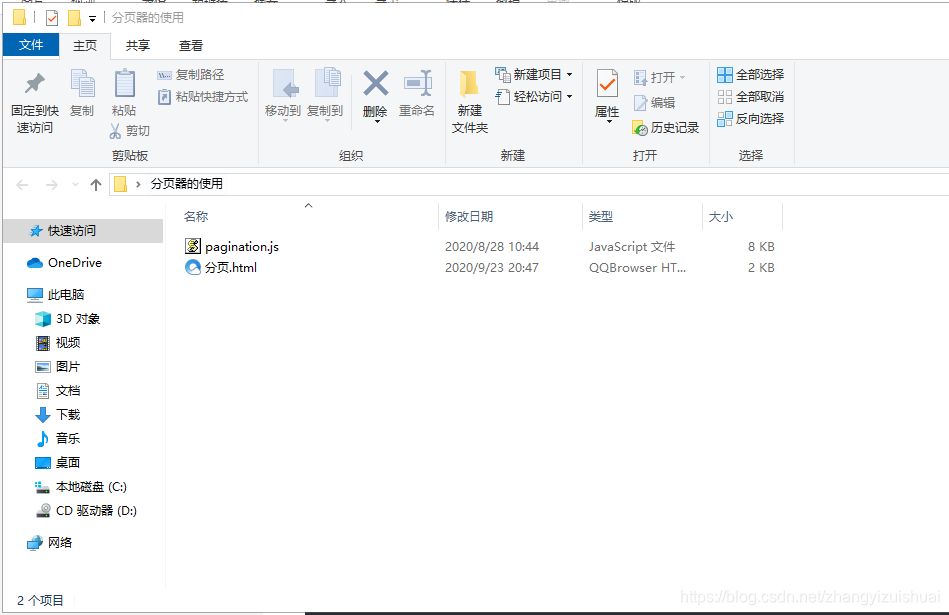
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>分页器的使用以及封装</title>
</head>
<style>
.box {
width: 800px;
height: 40px;
border: 1px red solid;
}
.box div{
user-select:none;
cursor: pointer;
}
</style>
<body>
<div class="box"></div>
</body>
<script src="./pagination.js"></script>
<!-- 上面封装的分液器js文件使用方法如下↓ -->
<!-- 因为是一个构造函数,所以需要new调用函数。 -->
<!-- 参数一:ele就是你想把分液器放在哪一个DOM元素上,
如果你想把分页器放在id为c的DOM元素上就填'#c'选择器写法就行 -->
<!-- 参数二是一个对象
对象包含的是分页信息
-->
<script>
var box = document.querySelector(".box")
new Pagination(box, {
pageInfo: {
pagenum: 1,
pagesize: 20,
total: 100,
totalpage: 5,
},
textInfo: {
first: "首页",
prev: "上一页",
next: "下一页",
last: "尾页",
},
change(n) {
console.log("n表示你选择的是第几页或者说你在输入框内填写的是第几页",n);
}
})
</script>
</html>
js封装代码如下js封装是上面代码中script标签内部引入的pagination.js
function Pagination(ele, options) {
if (!ele) {
throw new Error('方法必须传递参数,第一个为dom元素,第二个为对象');
}
this.ele = ele
this.options = options || {}
console.log(1);
this.default = {
pageInfo: {
pagenum: 1,
pagesize: 10,
total: 1000,
totalpage: 100
},
textInfo: {
first: 'first',
prev: 'prev',
list: '',
next: 'next',
last: 'last'
}
}
this.change = this.options.change || function () { }
this.list = null
this.init()
}
Pagination.prototype.init = function () {
this.setDefault()
this.setStyle()
this.dongcidaci()
}
Pagination.prototype.setDefault = function () {
if (this.options.pageInfo) {
for (let attr in this.options.pageInfo) {
this.default.pageInfo[attr] = this.options.pageInfo[attr]
}
}
if (this.options.textInfo) {
for (let attr in this.options.textInfo) {
this.default.textInfo[attr] = this.options.textInfo[attr]
}
}
}
Pagination.prototype.setStyle = function () {
this.ele.innerHTML = ''
setCss(this.ele, {
display: 'flex',
justifyContent: 'center',
alignItems: 'center'
})
this.createEle()
this.creteList()
this.go()
this.isDis()
this.change(this.default.pageInfo.pagenum)
}
Pagination.prototype.createEle = function () {
for (let attr in this.default.textInfo) {
const div = document.createElement('div')
div.className = attr
if (attr === 'list') {
this.list = div
setCss(div, {
display: 'flex',
justifyContent: 'center',
alignItems: 'center'
})
} else {
setCss(div, {
border: '1px solid #333',
padding: '0 5px',
margin: '0 5px'
})
}
div.innerHTML = this.default.textInfo[attr]
this.ele.appendChild(div)
}
}
Pagination.prototype.creteList = function () {
const pagenum = this.default.pageInfo.pagenum
const totalpage = this.default.pageInfo.totalpage
if (totalpage <= 9) {
for (let i = 1; i <= this.default.pageInfo.totalpage; i++) {
const p = this.crealeP(i)
this.list.appendChild(p)
}
} else {
if (pagenum < 5) {
for (let i = 1; i <= 5; i++) {
this.list.appendChild(this.crealeP(i))
}
const span = document.createElement('span')
span.innerHTML = '...'
this.list.appendChild(span)
for (let i = totalpage - 1; i <= totalpage; i++) {
this.list.appendChild(this.crealeP(i))
}
} else if (pagenum === 5) {
for (let i = 1; i <= 7; i++) {
this.list.appendChild(this.crealeP(i))
}
const span = document.createElement('span')
span.innerHTML = '...'
this.list.appendChild(span)
for (let i = totalpage - 1; i <= totalpage; i++) {
this.list.appendChild(this.crealeP(i))
}
} else if (pagenum > 5 && pagenum < totalpage - 4) {
for (let i = 1; i <= 2; i++) {
this.list.appendChild(this.crealeP(i))
}
const span = document.createElement('span')
span.innerHTML = '...'
this.list.appendChild(span)
for (let i = pagenum - 2; i <= pagenum + 2; i++) {
this.list.appendChild(this.crealeP(i))
}
const span2 = document.createElement('span')
span2.innerHTML = '...'
this.list.appendChild(span2)
for (let i = totalpage - 1; i <= totalpage; i++) {
this.list.appendChild(this.crealeP(i))
}
} else if (pagenum === totalpage - 4) {
for (let i = 1; i <= 2; i++) {
this.list.appendChild(this.crealeP(i))
}
const span = document.createElement('span')
span.innerHTML = '...'
this.list.appendChild(span)
for (let i = totalpage - 6; i <= totalpage; i++) {
this.list.appendChild(this.crealeP(i))
}
} else if (pagenum > totalpage - 4) {
for (let i = 1; i <= 2; i++) {
this.list.appendChild(this.crealeP(i))
}
const span = document.createElement('span')
span.innerHTML = '...'
this.list.appendChild(span)
for (let i = totalpage - 4; i <= totalpage; i++) {
this.list.appendChild(this.crealeP(i))
}
}
}
}
Pagination.prototype.crealeP = function (i) {
const p = document.createElement('p')
p.innerHTML = i
setCss(p, {
border: '1px solid #333',
margin: '0 5px',
padding: '0 5px'
})
if (i === this.default.pageInfo.pagenum) {
setCss(p, {
backgroundColor: 'orange'
})
}
return p
}
Pagination.prototype.go = function () {
const inp = document.createElement('input')
const btn = document.createElement('button')
setCss(inp, {
outline: 'none',
width: '50px',
height: '20px'
})
inp.value = this.default.pageInfo.pagenum
inp.type = 'number'
inp.setAttribute('min', '1')
inp.setAttribute('max', this.default.pageInfo.totalpage)
setCss(btn, {
outline: 'none',
width: '30px',
height: '24px',
marginLeft: '5px'
})
btn.innerHTML = 'go'
this.ele.appendChild(inp)
this.ele.appendChild(btn)
}
Pagination.prototype.isDis = function () {
if (this.default.pageInfo.pagenum === 1) {
this.ele.children[0].style.backgroundColor = '#ccc'
this.ele.children[1].style.backgroundColor = '#ccc'
}
if (this.default.pageInfo.pagenum === this.default.pageInfo.totalpage) {
this.ele.children[3].style.backgroundColor = '#ccc'
this.ele.children[4].style.backgroundColor = '#ccc'
}
}
Pagination.prototype.dongcidaci = function () {
this.ele.addEventListener('click', e => {
e = e || window.event
const target = e.target
if (target.className === 'first' && this.default.pageInfo.pagenum !== 1) {
this.default.pageInfo.pagenum = 1
this.setStyle()
}
if (target.className === 'prev' && this.default.pageInfo.pagenum !== 1) {
this.default.pageInfo.pagenum--
this.setStyle()
}
if (target.className === 'next' && this.default.pageInfo.pagenum !== this.default.pageInfo.totalpage) {
this.default.pageInfo.pagenum++
this.setStyle()
}
if (target.className === 'last' && this.default.pageInfo.pagenum !== this.default.pageInfo.totalpage) {
this.default.pageInfo.pagenum = this.default.pageInfo.totalpage
this.setStyle()
}
if (target.nodeName === 'P' && target.innerHTML - 0 !== this.default.pageInfo.pagenum) {
this.default.pageInfo.pagenum = target.innerHTML - 0
this.setStyle()
}
if (target.nodeName === 'BUTTON' && target.previousElementSibling.value - 0 !== this.default.pageInfo.pagenum) {
this.default.pageInfo.pagenum = target.previousElementSibling.value - 0
this.setStyle()
}
})
}
function setCss(ele, options) {
for (let attr in options) {
ele.style[attr] = options[attr]
}
}