#include<iostream>
#include<vector>
#include<functional>
using namespace std;
template <class T>
struct Less{//注意名字不可以小写,会和库里的重复,
bool operator()(const T&left, const T& right){
return left < right;
}
};
template <class T>
struct Greater{
bool operator()(const T&left, const T& right){
return left > right;
}
};
//第三参数compare为仿函数,默认构造是less(小堆)
template <class T, class Container = vector<T>, class Compare = Less<T> >
//用less是调用#include<functional>库里的less
class priority_queue
{
public:
priority_queue(){};
template<class InputIterator>
priority_queue(InputIterator first, InputIterator last) :c(first, last)
{
int n = c.size();
int start = n / 2 - 1; //建堆是从非叶子结点开始的
while (start >= 0)
{
AdjustDown(start);
start--;
}
}
bool empty() const{
return c.empty();
}
size_t size() const{
return c.size();
}
const T& top() const{
return c.front();
}
void push(const T& x){//尾插向上调整
c.push_back(x);
AdjustUP(c.size() - 1);
}
void pop(){//每次删除堆顶的元素,就是和最后的交换尾删,最后的再向下调整
if (empty()){
return;
}
swap(c.front(), c.back());
c.pop_back();
AdjustDown(0);
}
private:
Container c;
Compare comp;
//向上调整
private:
void AdjustUP(int child){//根据数组小标元素调整
while (child){
int partent = ((child - 1) / 2);
if (comp(c[partent], c[child])){
swap(c[partent], c[child]);
child = partent;
partent = ((child - 1) / 2);
}
else{
return;
}
}
}
void AdjustDown(int start){
int partent = start;
int child = partent * 2 + 1;
while (child < c.size()){
if (child + 1 < c.size() && comp(c[child], c[child + 1])){
child++;
}
if (comp(c[partent], c[child])){
T tmp = c[partent];
c[partent] = c[child];
c[child] = tmp;
partent = child;
child = partent * 2 + 1;
}
else
break;
}
}
};
int main(){
vector<int> v{ 3, 2, 6, 1, 2, 9, 8 };
priority_queue<int> q(v.begin(),v.end());
cout << q.top() << endl;
system("pause");
return 0;
}
模拟实现priority_queue
最新推荐文章于 2021-09-15 21:09:33 发布
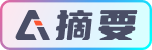