#include<iostream>
#include<mutex>
using namespace std;
//智能指针的模拟
//内部就是一个需要管理的空间
//构造函数赋值,析构函数清理
//指针要实现*,返回解引用后的空间,->返回指针
//auto_ptr的核心是拷贝构造,赋值运算符的管理权转移(参数一定要引用!!!因为要拿走它的资源)
//赋值运算符因为不是构造,要先判断不是给自己复制,在清理空间再复制,返回*this
template<class T>
class Auto_ptr{
public:
Auto_ptr(T* ptr=nullptr)
:_ptr(ptr)
{}
~Auto_ptr(){
if (_ptr != nullptr)
delete _ptr;
}
Auto_ptr(Auto_ptr<T>& a){
_ptr = a._ptr;
a._ptr = nullptr;
}
Auto_ptr<T>& operator=(Auto_ptr<T>& a){//返回的是智能指针,也就是整个智能指针对象(引用提高效率)
if (&a != this){ //也就是*this
if (this->_ptr)
delete _ptr;//delete
_ptr = a._ptr;
a._ptr = nullptr;
}
return *this;
}
T& operator*(){ return *_ptr; }//解引用当然拿到的是数值内容,是返回引用
T* operator->(){ return _ptr; }//箭头是指拿到内部的东西,也就是拿到指针
private:
T* _ptr;
};
//unique_ptr就是禁用了拷贝构造和赋值运算符的unique_ptr;
//shared_ptr需要自己在内部维护互斥锁指针和引用计数指针
//特殊点在于拷贝构造,赋值运算符计数+1,构造不用,析构要看情况析构
//计数加一要加锁,释放需要加锁(注意如果计数为0,解锁后才释放资源)
template <class T>
class Shared_ptr{
public:
Shared_ptr(T* ptr=nullptr)
:_ptr(ptr),
_mutex(new mutex),
_count(new int(1))
{}
~Shared_ptr(){
Release();
}
void countadd(){
_mutex->lock();
(*_count)++; //注意要解引用
_mutex->unlock();
}
Shared_ptr (const Shared_ptr<T>& p)
:_ptr(p._ptr),
_mutex(p._mutex),
_count(p.count)
{
countadd();
}
Shared_ptr<T>& operator=(Shared_ptr<T>& p){
if (this != &p){
Release();//释放this的智能指针的空间
_ptr = p.ptr;
_mutex = p._mutex;
_count = p._count;
countadd();
}
return *this;
}
T& operator*() { return *_ptr; }
T* operator->() { return _ptr; }
int UseCount() { return *_pRefCount; }
private:
void Release(){
bool m = flase;
_mutex->lock();
if ((--(*_count)) == 0){
delete _count;
delete _ptr;
m = true;
}
_mutex->unlock();
if (m){
delete _mutex;
}
}
T* _ptr;
mutex* _mutex;
int* _count;
};
【C++】智能指针的模拟实现
最新推荐文章于 2022-03-04 20:47:23 发布
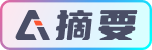