UITableViewCell类能够显示出各种各样的风格,但有时候我们需要适应不同的显示模式下的显示。今天的文章中,我们将使用table view去显示一系列自定义的cell。
启动Xcode,选择"Create a new Xcode project",然后选择空应用程序模板,点击Next。命名为 CustomCells,然后照下图那样设置。
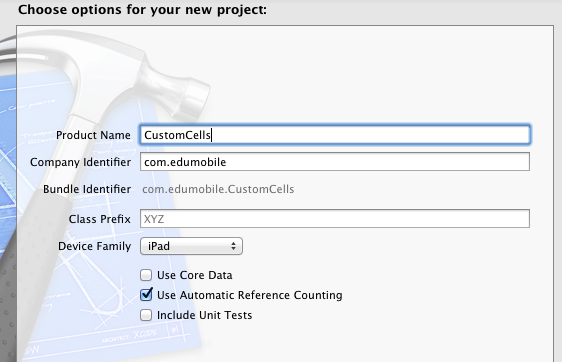
点击Next,选择项目的存放路径,最后点击Create。
这里需要添加两个文件,UITableViewController以及custom cell对应的xib文件。
Choose File | New > File ,然后添加一个名为 TableViewController 的UITableViewController。
如图:
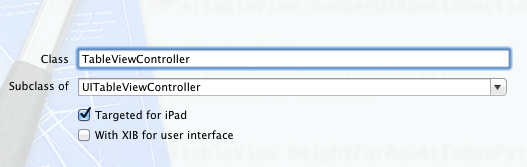
对于这个controller,我们并不需要xib文件,所以直接点击Next创建。
重新创建文件,这次我们是创建一个空的 xib 文件,如下图:
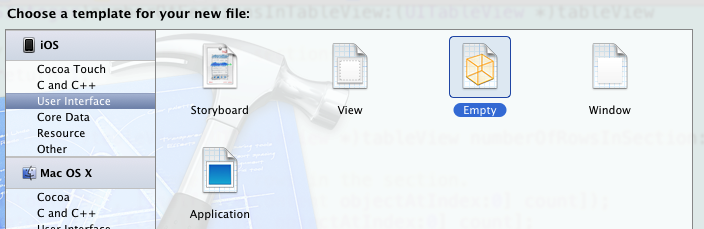
点击Next,确保Device Family被设置为iPad,再点击Next,在默认路径下保存为 CellNib 文件。
接着打开 CellNib.xib 文件。在上面拖放几个 label:
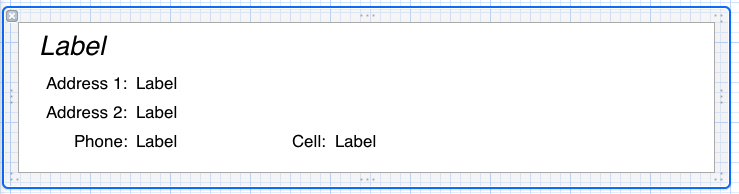
这里第一个Label的字体大小是27,字体是System Italic。而其他的Label全部都是默认设置。
下一步就是为文本依然是"Label"的Label设置tag。
将第一个大字体的Label设置tag=1,然后设置Address1,Address2,Phone,Cell右边的Label的tag分别为2,3,4,5。
接着需要修改xib的File's Owner的所属类。这里选择为 TableViewController。
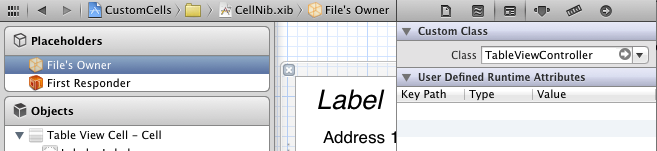
打开 TableViewController.h 然后添加这些属性:
#import @interface TableViewController : UITableViewController
@property (nonatomic, strong) NSArray *cellContent;
@property (nonatomic, strong) IBOutlet UITableViewCell *customCell;@end
这个演示中,我们定义一个数组来记录所有cell的内容,还需要如下图那样,设置设置好 customCell的outlet。
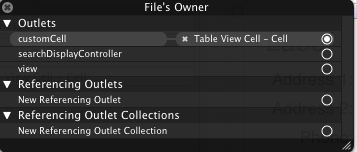
现在打开TableViewController.m做出如下更改:
#import "TableViewController.h"@interface TableViewController ()@end
@implementation TableViewController
@synthesize cellContent, customCell;
- (NSArray *)cellContent
{
cellContent = [[NSArray alloc] initWithObjects:
[NSArray arrayWithObjects:@"Alex Ander",
@"213 4th St.", @"Apt. 17", @"555-555-5555", @"111-111-1111", nil],
[NSArray arrayWithObjects:@"Jane Doe",
@"4 Any Ave.", @"Suite 2", @"123-456-7890", @"098-765-4321", nil],
[NSArray arrayWithObjects:@"Bill Smith",
@"63 Smith Dr.", @"", @"678-765-1236", @"987-234-4987", nil],
[NSArray arrayWithObjects:@"Mike Taylor",
@"3145 Happy Ct.", @"", @"654-321-9871", @"654-385-1594", nil],
[NSArray arrayWithObjects:@"Nancy Young",
@"98 W. 98th St.", @"Apt. 3", @"951-753-9871", @"951-654-3557", nil],
nil];
return cellContent;
}
- (id)initWithStyle:(UITableViewStyle)style
{
self = [super initWithStyle:style];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
// Uncomment the following line to preserve selection between presentations.
// self.clearsSelectionOnViewWillAppear = NO;
// Uncomment the following line to display an Edit button in the navigation bar for this view controller.
// self.navigationItem.rightBarButtonItem = self.editButtonItem;
}
- (void)viewDidUnload
{
[super viewDidUnload];
// Release any retained subviews of the main view.
// e.g. self.myOutlet = nil;
}
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
return YES;
}
#pragma mark – Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return [[self.cellContent objectAtIndex:0] count];
}
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
return 149.0f;
}
- (void)tableView:(UITableView *)tableView willDisplayCell:(UITableViewCell *)cell forRowAtIndexPath:(NSIndexPath *)indexPath
{
cell.backgroundColor = [UIColor colorWithRed:1 green:1 blue:.75 alpha:1];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
if (cell == nil) {
[[NSBundle mainBundle] loadNibNamed:@"CellNib" owner:self options:nil];
cell = self.customCell;
self.customCell = nil;
}
// Configure the cell…
UILabel *textTarget;
textTarget = (UILabel *)[cell viewWithTag:1]; //name
textTarget.text = [[self.cellContent objectAtIndex:indexPath.row] objectAtIndex:0];
textTarget = (UILabel *)[cell viewWithTag:2]; //addr1
textTarget.text = [[self.cellContent objectAtIndex:indexPath.row] objectAtIndex:1];
textTarget = (UILabel *)[cell viewWithTag:3]; //addr2
textTarget.text = [[self.cellContent objectAtIndex:indexPath.row] objectAtIndex:2];
textTarget = (UILabel *)[cell viewWithTag:4]; //phone
textTarget.text = [[self.cellContent objectAtIndex:indexPath.row] objectAtIndex:3];
textTarget = (UILabel *)[cell viewWithTag:5]; //cellPhone
textTarget.text = [[self.cellContent objectAtIndex:indexPath.row] objectAtIndex:4];
return cell;
}
#pragma mark – Table view delegate
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
// Navigation logic may go here. Create and push another view controller.
/*
<#DetailViewController#> *detailViewController = [[<#DetailViewController#> alloc] initWithNibName:@"<#Nib name#>" bundle:nil];
// …
// Pass the selected object to the new view controller.
[self.navigationController pushViewController:detailViewController animated:YES];
*/
}@end
#import
#import "TableViewController.h"@interface AppDelegate : UIResponder @property (strong, nonatomic) UIWindow *window;
@property (strong, nonatomic) TableViewController *tableViewController;
@property (strong, nonatomic) UINavigationController *navController;@end
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
self.window = [[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
// Override point for customization after application launch.
self.tableViewController = [[TableViewController alloc] initWithStyle:UITableViewStylePlain];
self.tableViewController.title = @"Table View";
self.navController = [[UINavigationController alloc]
initWithRootViewController:self.tableViewController];
[self.window addSubview:self.navController.view];
[self.window makeKeyAndVisible];
return YES;
OK,现在运行程序,特别注意一下tableViewController的默认cell已经被我们的自定义 cell 替代。
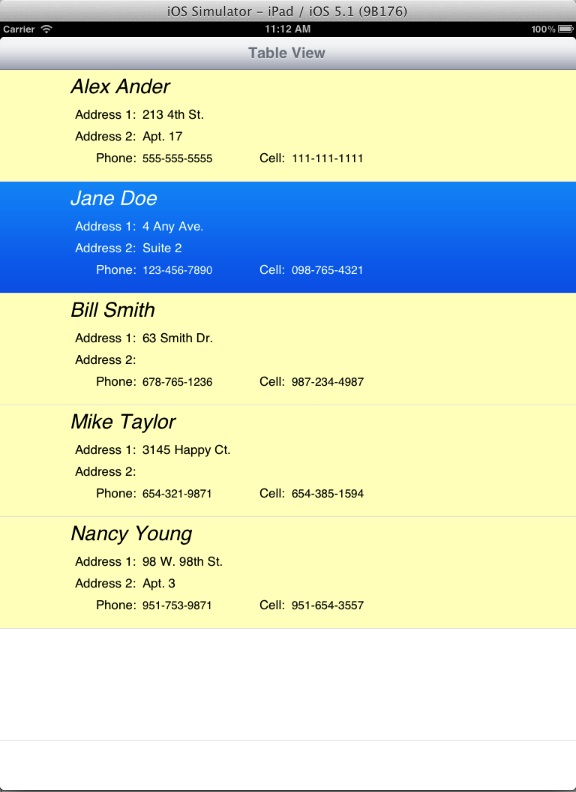
用这种方式创建的 TableViewCell 能够包含任意控件而不仅仅只是Label与Image,也因为Files's Owner属于TableViewController,任何action方法必须先在viewController对应的类中事先定义好,当然这部分就是另一篇文章所谈到的事情了