文章目录
Java8 BiConsumer<T, U> 函数接口浅析分享(含示例,来戳!)
💗💗💗您的点赞、收藏、评论是博主输出优质文章的的动力!!!💗💗💗
欢迎在评论区与博主沟通交流!!Java8 系列文章持续更新,大佬们关注我!种个草不亏!👇🏻 👇🏻 👇🏻
学起来,开整!Java8 BiConsumer<T, U>函数接口使用分享。
BiConsumer<T, U> 跟我们熟悉的 Consumer< T> 很像,核心思想也是一样的,两者都是表达消费的意思;
源码
BiConsumer<T, U> 提供了两个方法:
- void accept(T t, U u):传入两个泛型参数(当然方法实现想怎么用自己玩,可以看下面示例场景);
- BiConsumer<T, U> andThen(BiConsumer<? super T, ? super U> after):可以理解为在执行 accept 方法前先执行一个前置方法,前置方法执行完成后,会接着执行 accept 方法;
源码
@FunctionalInterface
public interface BiConsumer<T, U> {
void accept(T t, U u);
default BiConsumer<T, U> andThen(BiConsumer<? super T, ? super U> after) {
Objects.requireNonNull(after);
return (l, r) -> {
accept(l, r);
after.accept(l, r);
};
}
}
accept 方法示例
tips: 需要配合文末示例相关代码类食用
示例一
查询所有学生的成绩:
@Test
public void test() {
StudentScoreDto studentScoreDto = new StudentScoreDto();
Student student = new Student();
this.doHandler(student, studentScoreDto, StudentAssemble::queryList, StudentAssemble::calcSort);
System.out.println(JSONObject.toJSONString(studentScoreDto));
}
执行结果:
示例二
查询 “张三” 同学的成绩:
@Test
public void test() {
StudentScoreDto studentScoreDto1 = new StudentScoreDto();
Student student1 = new Student();
student1.setName("张三");
this.doHandler(student1, studentScoreDto1, StudentAssemble::queryList, StudentAssemble::calcSort);
System.out.println(JSONObject.toJSONString(studentScoreDto1));
}
执行结果:
andThen 方法示例
示例一
查询所有学生的成绩,并且输出第一名是哪位同学;
@Test
public void test() {
StudentScoreDto studentScoreDto2 = new StudentScoreDto();
Student student2 = new Student();
this.doHandler(student2, studentScoreDto2, StudentAssemble::queryList, StudentAssemble::calcSort, StudentAssemble::findFirst);
System.out.println(JSONObject.toJSONString(studentScoreDto2));
}
执行结果:
示例二
查询所有学生的成绩,并且输出 “李四” 同学的排名和总分;
@Test
public void test() {
StudentScoreDto studentScoreDto3 = new StudentScoreDto();
studentScoreDto3.setName("李四");
Student student3 = new Student();
this.doHandler(student3, studentScoreDto3, StudentAssemble::queryList, StudentAssemble::calcSort, StudentAssemble::findFirst);
System.out.println(JSONObject.toJSONString(studentScoreDto3));
}
执行结果:
示例相关代码类
tips: 需要配合文末示例相关代码类食用
dohandler 方法
tip:注意这里是方法!!
public void doHandler(Student student,
StudentScoreDto dto,
Function<Student, List<StudentScore>> func1,
BiConsumer<List<StudentScore>, StudentScoreDto> func2) {
List<StudentScore> apply = func1.apply(student);
func2.accept(apply, dto);
}
public void doHandler(Student student,
StudentScoreDto dto,
Function<Student, List<StudentScore>> func1,
BiConsumer<List<StudentScore>, StudentScoreDto> func2,
BiConsumer<List<StudentScore>, StudentScoreDto> func3) {
List<StudentScore> apply = func1.apply(student);
func2.accept(apply, dto);
func3.andThen(func2).accept(apply, dto);
}
student.java
@AllArgsConstructor
@NoArgsConstructor
@Data
public class Student {
/**
* 姓名
*/
private String name;
/**
* 年龄
*/
private Integer age;
/**
* 生日
*/
@JSONField(format="yyyy-MM-dd HH:mm:ss")
private Date birthday;
/**
* 学号
*/
private Integer num;
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", birthday=" + birthday +
", num=" + num +
'}';
}
StudentScore.java
@AllArgsConstructor
@NoArgsConstructor
@Data
public class StudentScore {
/**
* 名称
*/
private String name;
/**
* 科目
*/
private String subject;
/**
* 成绩
*/
private Integer score;
}
StudentScoreDto.java
@AllArgsConstructor
@NoArgsConstructor
@Data
public class StudentScoreDto {
/**
* 名称
*/
private String name;
/**
* 科目
*/
private String subject;
/**
* 成绩
*/
private Integer score;
/**
* 个人排名
*/
private Integer rank;
/**
* 全班排名
*/
private List<StudentScoreDto> rankList;
}
StudentAssemble.java
public class StudentAssemble {
/**
* 查询学生成绩列表
*
* @param student 学生实体对象
* @return 学生成绩集合
*/
public static List<StudentScore> queryList(Student student) {
// 模拟查询学生信息
List<StudentScore> studentScores = Arrays.asList(
new StudentScore("张三", "语文", 81),
new StudentScore("张三", "数学", 88),
new StudentScore("张三", "英语", 90),
new StudentScore("李四", "语文", 72),
new StudentScore("李四", "数学", 97),
new StudentScore("李四", "英语", 77),
new StudentScore("王五", "语文", 95),
new StudentScore("王五", "数学", 62),
new StudentScore("王五", "英语", 92));
if (Objects.isNull(student) || StringUtils.isEmpty(student.getName())) {
return studentScores;
}
String name = student.getName();
return studentScores.stream().filter(e -> name.equals(e.getName())).collect(Collectors.toList());
}
/**
* 计算总分以及排名
*
* @param studentScoreList 学生成绩集合
* @param studentScoreDto 学生成绩DTO
*/
public static void calcSort(List<StudentScore> studentScoreList, StudentScoreDto studentScoreDto) {
if (studentScoreList == null || studentScoreList.size() == 0) {
return;
}
List<StudentScoreDto> tempList = new ArrayList<>();
Map<String, List<StudentScore>> studentScoreMap = studentScoreList.stream().collect(Collectors.groupingBy(StudentScore::getName));
for (List<StudentScore> value : studentScoreMap.values()) {
if (value == null || value.size() == 0) continue;
StudentScore studentScore = value.get(0);
// 汇总学生总成绩,如果成绩不存在,则直接给0
Integer sumScore = value.stream().map(StudentScore::getScore).reduce(Integer::sum).orElse(0);
StudentScoreDto resultDto = new StudentScoreDto();
resultDto.setName(studentScore.getName());
resultDto.setScore(sumScore);
tempList.add(resultDto);
}
AtomicInteger counter = new AtomicInteger(1);
// 处理排名
List<StudentScoreDto> resultList = tempList.stream().sorted(Comparator.comparing(StudentScoreDto::getScore).reversed()).collect(Collectors.toList());
resultList.forEach(e -> e.setRank(counter.getAndIncrement()));
studentScoreDto.setRankList(resultList);
}
/**
* 查询学生成绩
*
* @param studentScoreList 学生成绩集合
* @param studentScoreDto 学生成绩DTO
*/
public static void findFirst(List<StudentScore> studentScoreList, StudentScoreDto studentScoreDto) {
if (studentScoreList == null || studentScoreList.size() == 0) {
return;
}
List<StudentScoreDto> rankList = studentScoreDto.getRankList();
if (StringUtils.isEmpty(studentScoreDto.getName())) {
// 学生名称为空,则输出第一名
Optional<StudentScoreDto> first = rankList.stream().min(Comparator.comparing(StudentScoreDto::getRank));
if (first.isPresent()) {
StudentScoreDto studentScoreDto1 = first.get();
System.out.println("第一名是:" + studentScoreDto1.getName() + ", 总分:" + studentScoreDto1.getScore());
}
return;
}
// 学生名称不为空,则输出学生排名
Optional<StudentScoreDto> first = rankList.stream().filter(e -> studentScoreDto.getName().equals(e.getName())).findFirst();
if (first.isPresent()) {
StudentScoreDto studentScoreDto1 = first.get();
System.out.println(studentScoreDto1.getName() + " 排名:" + studentScoreDto1.getRank() + ", 总分:" + studentScoreDto1.getScore());
}
}
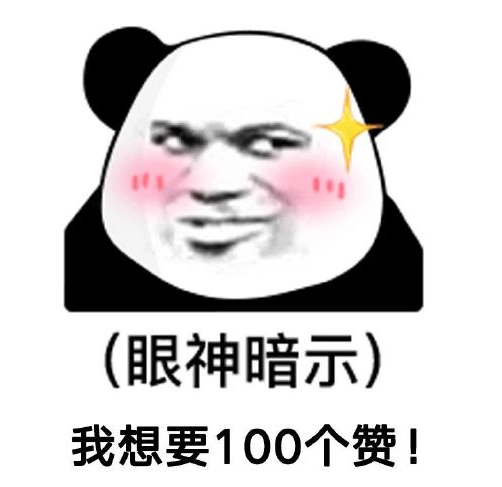