一.使用numpy.array来访问像素,并且使用item(行,列)来访问像素,用itemset(索引值,新值)来修改像素值。

编辑切换为居中
添加图片注释,不超过 140 字(可选)
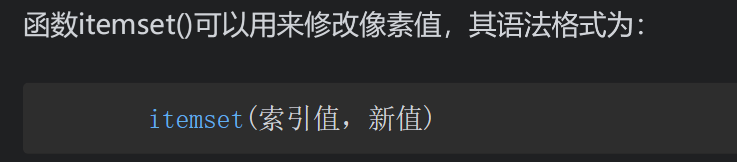
编辑切换为居中
添加图片注释,不超过 140 字(可选)
二.使用Numpy生成一个二维随机数组,用来模拟一幅灰度图像,并对其像素进行访问、修改。
import numpy as np
# random.randint可以生成一个随机数组,对应一个灰度图像
img = np.random.randint(10, 99, size=[5, 5], dtype=np.uint8)
# np.random.randint,low: int
# 生成的数值最低要大于等于low。
# (hign = None时,生成的数值要在[0, low)区间内)
# high: int (可选)
# 如果使用这个值,则生成的数值在[low, high)区间。
# size: int or tuple of ints(可选)
# ,比如size = (m * n* k)则输出同规模即m * n* k个随机数。默认是None的,仅仅返回满足要求的单一随机数。
# dtype: dtype(可选):
# 想要输出的格式。如int64、int等等
print("img=\n", img)
print("读取像素点img.item(3,2)=\n", img.item(3, 2))
img.itemset((3, 2), 255)
print("img的像素值=\n", img)
print("修改后的像素值=\n", img.item(3, 2))
.三.生成一个灰度图像,让其中的像素值均为随机数
import numpy as np
import cv2
# 生成一个随机数组,并且展示为灰度图像
img = np.random.randint(0, 256, size=[256, 256], dtype=np.uint8)
cv2.imshow("1", img)
cv2.waitKey()
cv2.destroyAllWindows()
.四.读取一幅灰度图像,并对其像素值进行访问、修改
import numpy as np
import cv2
img = cv2.imread("20170701031428774.jpg", flags=0) # flags=0,为灰度图像
print("读取像素点(3,2)=\n", img.item(3, 2))
img.itemset((3, 2), 18)
print("修改(3,2)的像素值为255=\n", img.itemset(3, 2))
# 修改一个区域的像素值
cv2.imshow("1", img)
for i in range(10, 100):
for j in range(80, 100):
img.itemset((i, j), 188)
cv2.imshow("2", img)
cv2.waitKey()
cv2.destroyAllWindows()
.五.
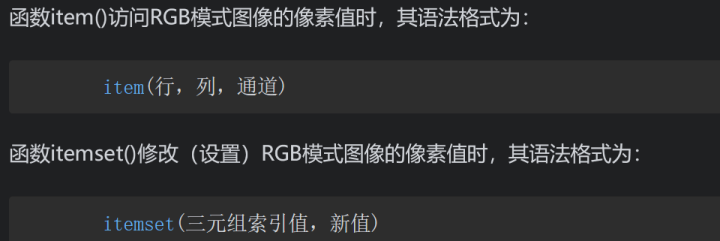
编辑切换为居中
添加图片注释,不超过 140 字(可选)
使用Numpy生成一个由随机数构成的三维数组,用来模拟一幅RGB色彩空间的彩色图像,并使用函数item()和itemset()来访问和修改它。
import numpy as np
# 生成一个随机彩色的RGB图像
img = np.random.randint(low=10, high=99, size=[2, 4, 3], dtype=np.uint8)
print("img=\n", img)
print("读取像素点img[1,2,0]表示第一行第一列B通道的=", img.item(1, 2, 0))
print("读取像素点img[1,2,1]表示第一行第一列G通道的=", img.item(1, 2, 1))
print("读取像素点img[1,2,2]表示第一行第一列R通道的=", img.item(1, 2, 2))
img.itemset((1, 2, 0), 255)
img.itemset((1, 2, 1), 255)
img.itemset((1, 2, 2), 255)
print("修改后的图像像素点的图像是=\n", img)
.六.生成一幅彩色图像,让其中的像素值均为随机数
import numpy as np
import cv2
img = np.random.randint(0, 256, size=[256, 256, 3], dtype=np.uint8)
cv2.imshow("1", img)
cv2.waitKey()
cv2.destroyAllWindows()
.七.读取一幅彩色图像,并对其像素进行访问、修改
import numpy as np
import cv2
img = cv2.imread("20170701031428774.jpg", flags=-1)
for i in range(0, 50):
for j in range(0, 100):
for k in range(0, 3):
img.itemset((i, j, k), 255)
cv2.imshow("1", img)
cv2.waitKey()
cv2.destroyAllWindows()
.八.感兴趣区域(Region of Interest, ROI
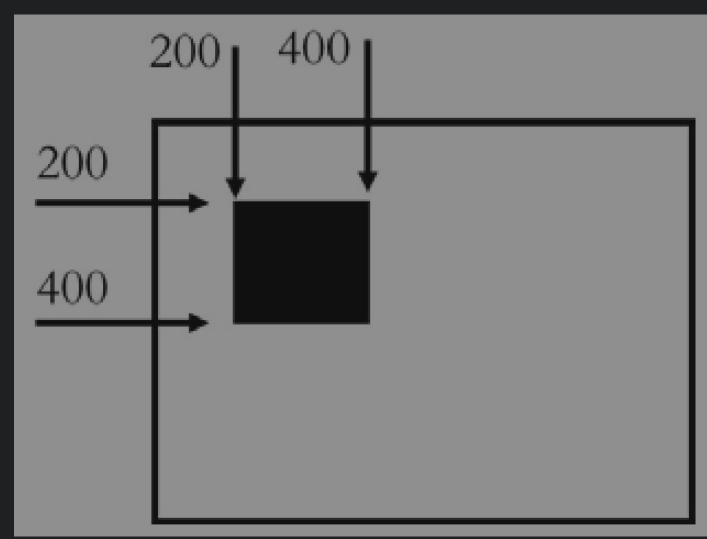
编辑切换为居中
添加图片注释,不超过 140 字(可选)
那么这个图像就可以表示为img[200:400,200:400]
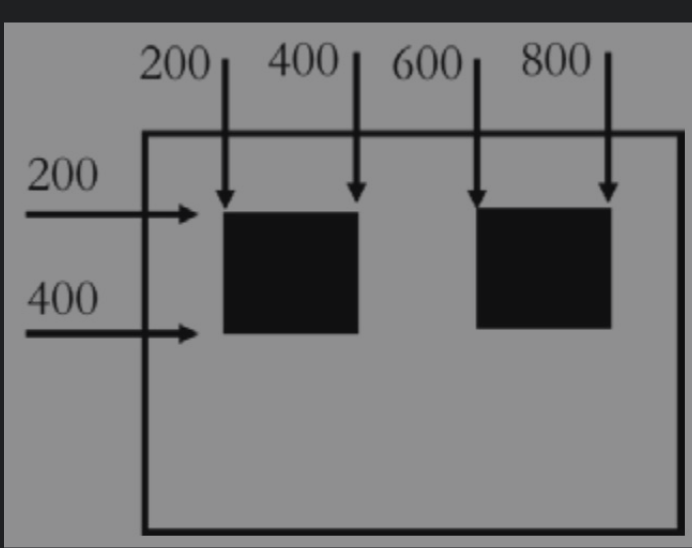
编辑切换为居中
添加图片注释,不超过 140 字(可选)
可以通过:
a=img(200:400,200:400)
img(200:400,600:800)=a
以上语句把黑色部分复制到右边。
a.获取图像lena的脸部信息,并将其显示出来
import cv2
a = cv2.imread("20170701031428774.jpg", -1)
scence = a[220:400, 250:350] # 截取感兴趣部分
cv2.imshow("1", a)
cv2.imshow("2", scence)
cv2.waitKey()
cv2.destroyAllWindows()
.b.对lena图像的脸部进行打码
import numpy as np
import cv2
a = cv2.imread("20170701031428774.jpg", -1)
cv2.imshow("1", a)
face = np.random.randint(low=0, high=256, size=(180, 100, 3)) # 生成一个BGR三通道的乱码图像
a[220:400, 250:350] = face # 用这个乱码覆盖
cv2.imshow("2", a)
cv2.waitKey()
cv2.destroyAllWindows()
.效果
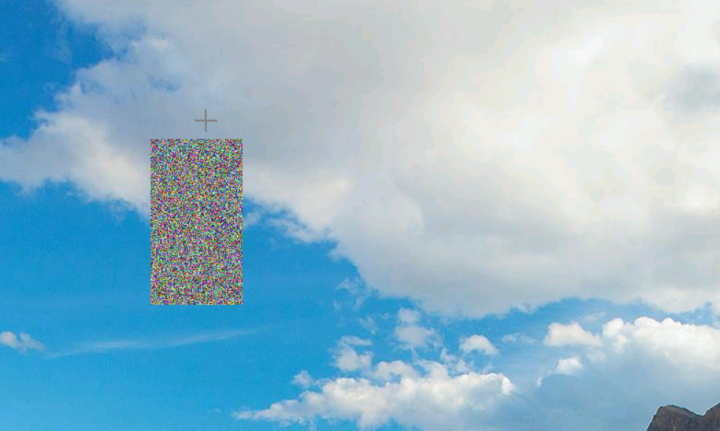
编辑切换为居中
添加图片注释,不超过 140 字(可选)
c.将一幅图像内的ROI复制到另一幅图像内
import cv2
scence = cv2.imread("20170701031428774.jpg", flags=-1)
gray = cv2.imread("22986182_173540093000_2.jpg", flags=-1)
cv2.imshow("1", scence)
cv2.imshow("2", gray)
# 生成scence中的感兴趣的部分
face = scence[220:400, 250:300]
gray[160:340, 200:300] = face # 将scence中的部分覆盖给gray
cv2.imshow("3", gray)
cv2.waitKey()
cv2.destroyAllWindows()
二.
1.获取两个整数a,b,要求交换这两个整数的值
a=int(input())
b=int(input())
a,b=b,a
print("a = {}, b = {}".format(a,b))
.2.给出三个正整数a,b,c,通过比较得出最大值
print(max(a,b,c))
.3.在 IDE 中分别打印出a和b逻辑运算的结果,请按照and,or,not的顺序打印结果,( 使用not时,请分别打印两个变量)
print(a and b)
print(a or b)
print(not a)
print(not b)
4.获取一个自然数n,左移三位后的结果
n=int(input())
print(n<<3)#<<左移运算符,移动多少位
5.商店里新推出了一种商品,普通顾客需要按照原价购买,而会员可以享受七折优惠。如果是在黑名单上的顾客,那么商店将不会出售商品给他。现有一个会员列表,和一个黑名单。此时来了一名顾客,请你打印出这名顾客最终需要付款的价格。如果是在黑名单上的顾客,你需要打印 -1。参数注释:
商品价格:price(int)
会员列表:vip(list)
黑名单:blacklist(list)
顾客:customer(str)
price = int(input())
customer = eval(input())
vip = eval(input())
blacklist = eval(input())
def countPrice(price,customer,vip,blacklist):
if customer in vip:
return '{:.2f}'.format(price*0.7)#{:.2f}保留两位小数
elif customer in blacklist:
return -1
else:
return price
print(countPrice(price,customer,vip,blacklist))