项目介绍:
本项目是基于C++实现的一个具有群聊功能的聊天室小程序。客户端和服务器都是基于QT实现的。
运行截图展示:
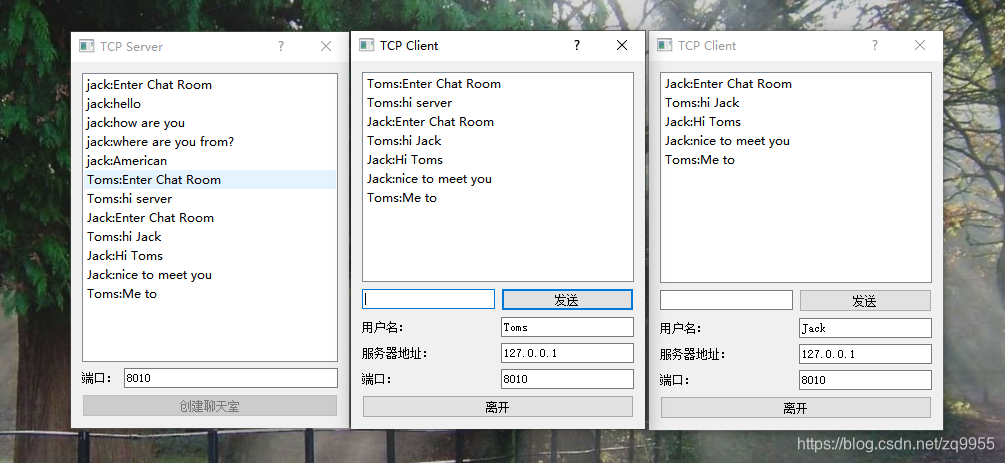
项目目录:
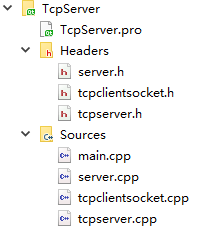
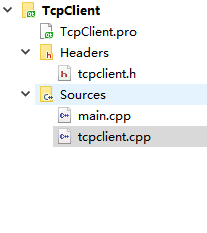
基于TCP的聊天室模型:
tcpserver.h
#ifndef TCPSERVER_H
#define TCPSERVER_H
#include <QDialog>
#include <QListWidget>
#include <QLabel>
#include <QLineEdit>
#include <QPushButton>
#include <QGridLayout>
#include "server.h"
class TcpServer : public QDialog
{
Q_OBJECT
public:
TcpServer(QWidget *parent = 0,Qt::WindowFlags f=0);
~TcpServer();
private:
QListWidget *ContentListWidget;
QLabel *PortLabel;
QLineEdit *PortLineEdit;
QPushButton *CreateBtn;
QGridLayout *mainLayout;
int port;
Server *server;
public slots:
void slotCreateServer();
void updateServer(QString,int);
};
#endif // TCPSERVER_H
tcpserver.cpp
#include "tcpserver.h"
TcpServer::TcpServer(QWidget *parent,Qt::WindowFlags f)
: QDialog(parent,f)
{
setWindowTitle(tr("TCP Server"));
ContentListWidget = new QListWidget;
PortLabel = new QLabel(tr("端口:"));
PortLineEdit = new QLineEdit;
CreateBtn = new QPushButton(tr("创建聊天室"));
mainLayout = new QGridLayout(this);
mainLayout->addWidget(ContentListWidget,0,0,1,2);
mainLayout->addWidget(PortLabel,1,0);
mainLayout->addWidget(PortLineEdit,1,1);
mainLayout->addWidget(CreateBtn,2,0,1,2);
port=8010;
PortLineEdit->setText(QString::number(port));
connect(CreateBtn,SIGNAL(clicked()),this,SLOT(slotCreateServer()));
}
TcpServer::~TcpServer()
{
}
void TcpServer::slotCreateServer()
{
server = new Server(this,port);
connect(server,SIGNAL(updateServer(QString,int)),this,SLOT(updateServer(QString,int)));
CreateBtn->setEnabled(false);
}
void TcpServer::updateServer(QString msg,int length)
{
ContentListWidget->addItem(msg.left(length));
}
tcpclinetsocket.h
#ifndef TCPCLIENTSOCKET_H
#define TCPCLIENTSOCKET_H
#include <QTcpSocket>
#include <QObject>
class TcpClientSocket : public QTcpSocket
{
Q_OBJECT
public:
TcpClientSocket(QObject *parent=0);
signals:
void updateClients(QString,int);
void disconnected(int);
protected slots:
void dataReceived();
void slotDisconnected();
};
#endif // TCPCLIENTSOCKET_H
tcpclientsocket.cpp
#include "tcpclientsocket.h"
TcpClientSocket::TcpClientSocket(QObject *parent)
{
connect(this,SIGNAL(readyRead()),this,SLOT(dataReceived()));
connect(this,SIGNAL(disconnected()),this,SLOT(slotDisconnected()));
}
void TcpClientSocket::dataReceived()
{
while(bytesAvailable()>0)
{
int length = bytesAvailable();
char buf[1024];
read(buf,length);
QString msg=buf;
emit updateClients(msg,length);
}
}
void TcpClientSocket::slotDisconnected()
{
emit disconnected(this->socketDescriptor());
}
server.h
#ifndef SERVER_H
#define SERVER_H
#include <QTcpServer>
#include <QObject>
#include "tcpclientsocket.h"
class Server : public QTcpServer
{
Q_OBJECT
public:
Server(QObject *parent=0,int port=0);
QList<TcpClientSocket*> tcpClientSocketList;
signals:
void updateServer(QString,int);
public slots:
void updateClients(QString,int);
void slotDisconnected(int);
protected:
void incomingConnection(int socketDescriptor);
};
#endif // SERVER_H
server.cpp
#include "server.h"
Server::Server(QObject *parent,int port)
:QTcpServer(parent)
{
listen(QHostAddress::Any,port);
}
void Server::incomingConnection(int socketDescriptor)
{
TcpClientSocket *tcpClientSocket=new TcpClientSocket(this);
connect(tcpClientSocket,SIGNAL(updateClients(QString,int)),this,SLOT(updateClients(QString,int)));
connect(tcpClientSocket,SIGNAL(disconnected(int)),this,SLOT(slotDisconnected(int)));
tcpClientSocket->setSocketDescriptor(socketDescriptor);
tcpClientSocketList.append(tcpClientSocket);
}
void Server::updateClients(QString msg,int length)
{
emit updateServer(msg,length);
for(int i=0;i<tcpClientSocketList.count();i++)
{
QTcpSocket *item = tcpClientSocketList.at(i);
if(item->write(msg.toLatin1(),length)!=length)
{
continue;
}
}
}
void Server::slotDisconnected(int descriptor)
{
for(int i=0;i<tcpClientSocketList.count();i++)
{
QTcpSocket *item = tcpClientSocketList.at(i);
if(item->socketDescriptor()==descriptor)
{
tcpClientSocketList.removeAt(i);
return;
}
}
return;
}
源码连接,可直接打开调试,本来想免费呢,可免费了上传不了资源,有需要的的可以下载下来调试编译,理清思想。
https://download.csdn.net/download/zq9955/14987590