需求:
用freemarker根据doc模板生成word文件并转化为pdf文件
- 准备模板文件
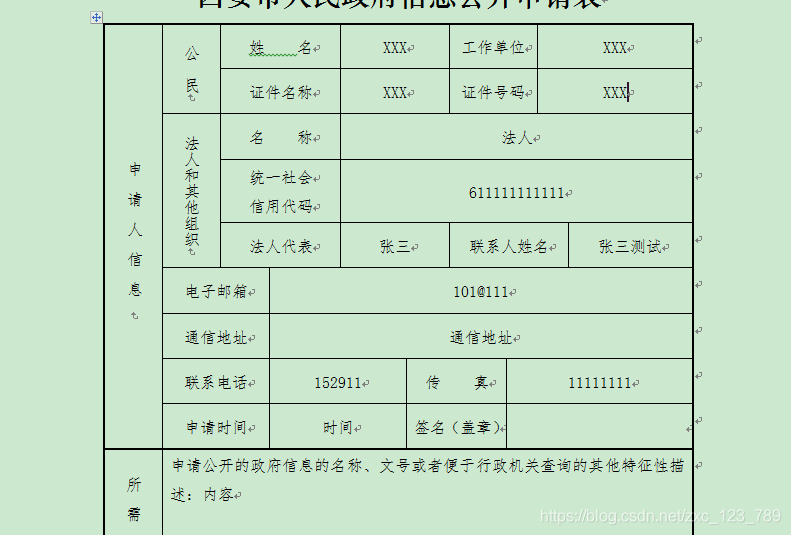
- 将模板文件另存为xml文件
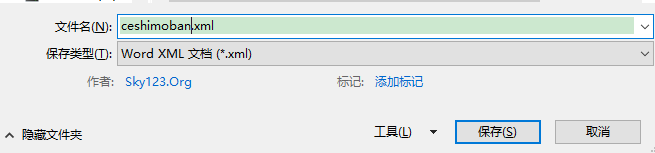
- 将xml内容格式化后用文本编辑器打开
- 用el表达式替换其中要动态填写的数据,并将后缀改为ftl
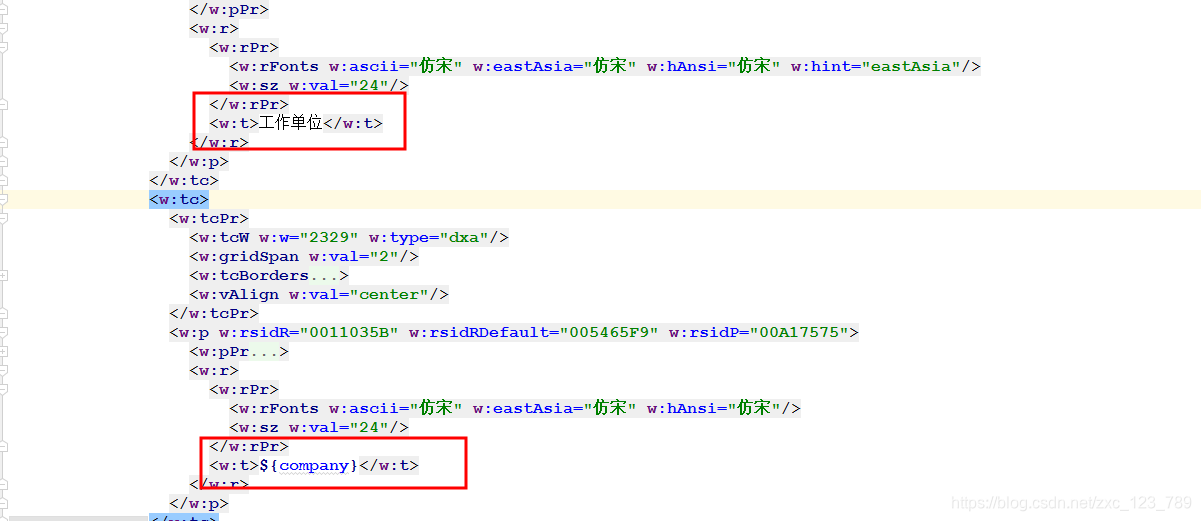
模板已经准备好了 - (spring boot)开始导入maven坐标
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.4.3</version>
</dependency>
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
<version>2.3.23</version>
</dependency>
<dependency>
<groupId>org.docx4j</groupId>
<artifactId>docx4j</artifactId>
<version>6.1.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.docx4j/docx4j-export-fo -->
<dependency>
<groupId>org.docx4j</groupId>
<artifactId>docx4j-export-fo</artifactId>
<version>6.0.1</version>
</dependency>
- 封装好的工具类
package com.cicro.exa.utils;
import com.itextpdf.text.Image;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
import freemarker.template.Configuration;
import freemarker.template.Template;
import freemarker.template.TemplateException;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.io.FileUtils;
import org.docx4j.Docx4J;
import org.docx4j.convert.out.FOSettings;
import org.docx4j.fonts.IdentityPlusMapper;
import org.docx4j.fonts.Mapper;
import org.docx4j.fonts.PhysicalFonts;
import org.docx4j.openpackaging.packages.WordprocessingMLPackage;
import org.springframework.core.io.ClassPathResource;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.util.HashMap;
import java.util.Map;
@Slf4j
public class PdfUtil {
private static String separator = File.separator;
private static Configuration configuration =null;
private static final String DOC_SUFFIX=".doc";
private static final String PDF_SUFFIX=".pdf";
static {
configuration=new Configuration(Configuration.getVersion());
configuration.setDefaultEncoding("UTF-8");
}
public static String convertDocx2Pdf(String wordPath,String pdfPath) {
OutputStream os = null;
InputStream is = null;
try {
is = new FileInputStream(new File(wordPath));
WordprocessingMLPackage mlPackage = WordprocessingMLPackage.load(is);
Mapper fontMapper = new IdentityPlusMapper();
fontMapper.put("隶书", PhysicalFonts.get("LiSu"));
fontMapper.put("宋体", PhysicalFonts.get("SimSun"));
fontMapper.put("微软雅黑", PhysicalFonts.get("Microsoft Yahei"));
fontMapper.put("黑体", PhysicalFonts.get("SimHei"));
fontMapper.put("楷体", PhysicalFonts.get("KaiTi"));
fontMapper.put("新宋体", PhysicalFonts.get("NSimSun"));
fontMapper.put("华文行楷", PhysicalFonts.get("STXingkai"));
fontMapper.put("华文仿宋", PhysicalFonts.get("STFangsong"));
fontMapper.put("宋体扩展", PhysicalFonts.get("simsun-extB"));
fontMapper.put("仿宋", PhysicalFonts.get("FangSong"));
fontMapper.put("仿宋_GB2312", PhysicalFonts.get("FangSong_GB2312"));
fontMapper.put("幼圆", PhysicalFonts.get("YouYuan"));
fontMapper.put("华文宋体", PhysicalFonts.get("STSong"));
fontMapper.put("华文中宋", PhysicalFonts.get("STZhongsong"));
mlPackage.setFontMapper(fontMapper);
String pdfName = wordPath.substring(0, wordPath.lastIndexOf("."));
pdfName = pdfName.substring(pdfName.lastIndexOf("\\") + 1);
String pdfOutPath = pdfPath + pdfName + PDF_SUFFIX;
File file=new File(pdfOutPath);
if (!file.getParentFile().exists()){
FileUtils.forceMkdir(file.getParentFile());
}
os = new java.io.FileOutputStream(pdfOutPath);
FOSettings foSettings = Docx4J.createFOSettings();
foSettings.setWmlPackage(mlPackage);
Docx4J.toFO(foSettings, os, Docx4J.FLAG_EXPORT_PREFER_XSL);
is.close();
os.close();
return pdfOutPath;
} catch (Exception e) {
e.printStackTrace();
try {
if(is != null){
is.close();
}
if(os != null){
os.close();
}
}catch (Exception ex){
ex.printStackTrace();
}
}finally {
File file = new File(wordPath);
if(file!=null&&file.isFile()&&file.exists()){
file.delete();
}
}
return "";
}
private static String addTextMark(String inPdfPath) {
PdfStamper stamp = null;
PdfReader reader = null;
try {
String fileName = "pdfMark_" + System.currentTimeMillis() + ".pdf";
String outPdfMarkPath = System.getProperty("java.io.tmpdir").replaceAll(separator + "$", "") + separator + fileName;
reader = new PdfReader(inPdfPath, "PDF".getBytes());
stamp = new PdfStamper(reader, new FileOutputStream(new File(outPdfMarkPath)));
PdfContentByte under;
int pageSize = reader.getNumberOfPages();
ClassPathResource resource = new ClassPathResource("template" + File.separator + "mark.png");
File file = resource.getFile();
Image image = Image.getInstance(file.getPath());
for (int i = 1; i <= pageSize; i++) {
under = stamp.getUnderContent(i);
image.setAbsolutePosition(100, 210);
under.addImage(image);
}
stamp.close();
reader.close();
return outPdfMarkPath;
} catch (Exception e) {
e.printStackTrace();
try {
if (stamp != null) {
stamp.close();
}
if (reader != null) {
reader.close();
}
} catch (Exception ex) {
ex.printStackTrace();
}
} finally {
File file = new File(inPdfPath);
if (file != null && file.exists() && file.isFile()) {
file.delete();
}
}
return "";
}
public static String createDoc(Map<String, String> dataMap, String tempPath, String outFilePath, String tempName) throws IOException {
if (!new File(tempPath).getParentFile().exists()){
FileUtils.forceMkdir(new File(tempPath).getParentFile());
}
configuration.setDirectoryForTemplateLoading(new File(tempPath));
Template template = configuration.getTemplate(tempName);
File outFile = new File(outFilePath+separator+dataMap.get("ysqId")+DOC_SUFFIX);
if (!outFile.getParentFile().exists()){
FileUtils.forceMkdir(outFile.getParentFile());
}
Writer writer=new BufferedWriter(new OutputStreamWriter(new FileOutputStream(outFile),"utf-8"));
try {
template.process(dataMap,writer);
} catch (TemplateException e) {
log.info("word文档{}生成错误",dataMap.get("id"));
e.printStackTrace();
}finally {
writer.close();
}
log.info("word文件路径:{}",outFile.getPath());
return outFile.getPath();
}
public static void main(String[] args) throws IOException {
String tempPath="H:/Java_project/conversion/src/main/resources/";
String outFilePath="D:/downLoad";
String tempName="ysqgk.ftl";
Map dataMap=new HashMap();
dataMap.put("id","123");
dataMap.put("name","测试名称");
dataMap.put("company","测试公司");
dataMap.put("cardName","测试证件");
dataMap.put("cardCode","测试账号");
String doc = createDoc(dataMap, tempPath, outFilePath, tempName);
String pdf = convertDocx2Pdf(doc, "D:/PDF/");
System.out.println(doc);
System.out.println(pdf);
}
}