The recent All-Berland Olympiad in Informatics featured n participants with each scoring a certain amount of points.
As the head of the programming committee, you are to determine the set of participants to be awarded with diplomas with respect to the following criteria:
- At least one participant should get a diploma.
- None of those with score equal to zero should get awarded.
- When someone is awarded, all participants with score not less than his score should also be awarded.
Determine the number of ways to choose a subset of participants that will receive the diplomas.
The first line contains a single integer n (1 ≤ n ≤ 100) — the number of participants.
The next line contains a sequence of n integers a1, a2, ..., an (0 ≤ ai ≤ 600) — participants' scores.
It's guaranteed that at least one participant has non-zero score.
Print a single integer — the desired number of ways.
4 1 3 3 2
3
3 1 1 1
1
4 42 0 0 42
1
There are three ways to choose a subset in sample case one.
- Only participants with 3 points will get diplomas.
- Participants with 2 or 3 points will get diplomas.
- Everyone will get a diploma!
The only option in sample case two is to award everyone.
Note that in sample case three participants with zero scores cannot get anything.
题意:给出一列数,统计这串数字中不包括零的不重复的数的数量
题解:排序后暴力一遍
代码
#include<cstdio>
#include<algorithm>
#define Max 110
using namespace std;
int a[Max];
int main()
{
int n;
while(scanf("%d", &n) != EOF)
{
for(int i = 0; i < n; i++)
scanf("%d", &a[i]);
sort(a, a + n);
int count = 0;
int flag = 0;
for(int i = 0; i < n; i++)
{
if(a[i] > flag)
{
count++;
flag = a[i];
}
}
printf("%d\n", count);
}
return 0;
}
The weather is fine today and hence it's high time to climb the nearby pine and enjoy the landscape.
The pine's trunk includes several branches, located one above another and numbered from 2 to y. Some of them (more precise, from 2 to p) are occupied by tiny vile grasshoppers which you're at war with. These grasshoppers are known for their awesome jumping skills: the grasshopper at branch x can jump to branches .
Keeping this in mind, you wisely decided to choose such a branch that none of the grasshoppers could interrupt you. At the same time you wanna settle as high as possible since the view from up there is simply breathtaking.
In other words, your goal is to find the highest branch that cannot be reached by any of the grasshoppers or report that it's impossible.
The only line contains two integers p and y (2 ≤ p ≤ y ≤ 109).
Output the number of the highest suitable branch. If there are none, print -1 instead.
3 6
5
3 4
-1
In the first sample case grasshopper from branch 2 reaches branches 2, 4 and 6 while branch 3 is initially settled by another grasshopper. Therefore the answer is 5.
It immediately follows that there are no valid branches in second sample case.
题解:因为要找最大的位置,所以直接从y暴力到p,然后判断位置是否符合条件,位置的判断为质数的判断
代码
#include<cstdio>
int p, y;
bool judge(int x)
{
for(int i = 2; i <= p && i * i <= x; i++)
{
if(x % i == 0)return false;
}
return true;
}
int main()
{
while(scanf("%d %d", &p, &y) != EOF)
{
bool flag = true;
int i;
for(i = y; i > p; i--)
{
if(judge(i))
{
flag = false;
break;
}
}
if(!flag)printf("%d\n", i);
else printf("-1\n");
}
return 0;
}
Julia is going to cook a chicken in the kitchen of her dormitory. To save energy, the stove in the kitchen automatically turns off after kminutes after turning on.
During cooking, Julia goes to the kitchen every d minutes and turns on the stove if it is turned off. While the cooker is turned off, it stays warm. The stove switches on and off instantly.
It is known that the chicken needs t minutes to be cooked on the stove, if it is turned on, and 2t minutes, if it is turned off. You need to find out, how much time will Julia have to cook the chicken, if it is considered that the chicken is cooked evenly, with constant speed when the stove is turned on and at a constant speed when it is turned off.
The single line contains three integers k, d and t (1 ≤ k, d, t ≤ 1018).
Print a single number, the total time of cooking in minutes. The relative or absolute error must not exceed 10 - 9.
Namely, let's assume that your answer is x and the answer of the jury is y. The checker program will consider your answer correct if .
3 2 6
6.5
4 2 20
20.0
In the first example, the chicken will be cooked for 3 minutes on the turned on stove, after this it will be cooked for . Then the chicken will be cooked for one minute on a turned off stove, it will be cooked for
. Thus, after four minutes the chicken will be cooked for
. Before the fifth minute Julia will turn on the stove and after 2.5 minutes the chicken will be ready
.
In the second example, when the stove is turned off, Julia will immediately turn it on, so the stove will always be turned on and the chicken will be cooked in 20 minutes.
题意:为了节约能源,炉子k分钟自动关闭,Julia每d分钟去一次厨房,若炉子关闭,则开启。炉子开启的时候,将食物煮熟需要t分钟,炉子关闭的时候会处于保温状态,将食物煮熟需要2*t分钟,求将食物煮熟最少需要几分钟
题解:找到一个循环节,然后模拟就行
代码
#include<cstdio>
#include<cmath>
#define ll long long
int main()
{
ll k, d, t;
while(scanf("%lld %lld %lld", &k, &d, &t) != EOF)
{
ll cnt = k / d;
if(k % d != 0)cnt++;
double time = cnt * d;
double k_time = k * 1.0 / t;
double d_time = (time - k) * 1.0 / t / 2.0;
double x = floor(1.0 / (k_time + d_time));
double ans = 1.0 * time * x;
double left = 1.0 - x * (k_time + d_time);
if(left <= k_time)
{
ans += left * t;
}
else
{
ans += k_time * t;
ans += (left - k_time) * t * 2.0;
}
printf("%.10f\n", ans);
}
return 0;
}
Petya and Vasya arranged a game. The game runs by the following rules. Players have a directed graph consisting of n vertices and medges. One of the vertices contains a chip. Initially the chip is located at vertex s. Players take turns moving the chip along some edge of the graph. Petya goes first. Player who can't move the chip loses. If the game lasts for 106 turns the draw is announced.
Vasya was performing big laboratory work in "Spelling and parts of speech" at night before the game, so he fell asleep at the very beginning of the game. Petya decided to take the advantage of this situation and make both Petya's and Vasya's moves.
Your task is to help Petya find out if he can win the game or at least draw a tie.
The first line of input contain two integers n and m — the number of vertices and the number of edges in the graph (2 ≤ n ≤ 105, 0 ≤ m ≤ 2·105).
The next n lines contain the information about edges of the graph. i-th line (1 ≤ i ≤ n) contains nonnegative integer ci — number of vertices such that there is an edge from i to these vertices and ci distinct integers ai, j — indices of these vertices (1 ≤ ai, j ≤ n, ai, j ≠ i).
It is guaranteed that the total sum of ci equals to m.
The next line contains index of vertex s — the initial position of the chip (1 ≤ s ≤ n).
If Petya can win print «Win» in the first line. In the next line print numbers v1, v2, ..., vk (1 ≤ k ≤ 106) — the sequence of vertices Petya should visit for the winning. Vertex v1 should coincide with s. For i = 1... k - 1 there should be an edge from vi to vi + 1 in the graph. There must be no possible move from vertex vk. The sequence should be such that Petya wins the game.
If Petya can't win but can draw a tie, print «Draw» in the only line. Otherwise print «Lose».
5 6 2 2 3 2 4 5 1 4 1 5 0 1
Win 1 2 4 5
3 2 1 3 1 1 0 2
Lose
2 2 1 2 1 1 1
Draw
In the first example the graph is the following:
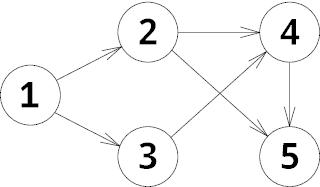
Initially the chip is located at vertex 1. In the first move Petya moves the chip to vertex 2, after that he moves it to vertex 4 for Vasya. After that he moves to vertex 5. Now it is Vasya's turn and there is no possible move, so Petya wins.
In the second example the graph is the following:

Initially the chip is located at vertex 2. The only possible Petya's move is to go to vertex 1. After that he has to go to 3 for Vasya. Now it's Petya's turn but he has no possible move, so Petya loses.
In the third example the graph is the following:
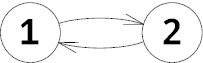
Petya can't win, but he can move along the cycle, so the players will draw a tie.
题意:两个人玩一个游戏,在一个图上,两个人轮流走一步,谁走到的点的出度为0的获胜,给出初始点,问Petya能否获胜,若能则输出Win,若不能,则尽量找到一个环,若能找到,则输出Draw,反之,输出Lose
题解:bfs找是否有环,dfs判断是否存在petya获胜的路径,petya获胜的路径条件为1.无出度 2.路径步数为偶数
代码
#include<cstdio>
#include<vector>
#include<queue>
#include<cstring>
#define Max 200010
using namespace std;
int n, m;
int tot;
bool vis[Max][2];
vector<int> v[Max];
queue<int> q;
int cnt;
bool flag_draw, flag_win, flag_lose;
int path[Max];
int ans;
void init(int a)
{
while(!q.empty())q.pop();
for(int i = 0; i <= n; i++)
v[i].clear();
cnt = 0;
flag_draw = false;
flag_win = false;
flag_lose = false;
memset(vis, false, sizeof(vis));
}
void bfs(int x)
{
q.push(x);
while(!q.empty())
{
int node = q.front();
q.pop();
for(int i = 0; i < v[node].size(); i++)
{
cnt++;
q.push(v[node][i]);
}
if(cnt >= 1000000)
{
flag_draw = true;
break;
}
}
}
void dfs(int x, int cnt)
{
if(flag_win)return;
path[cnt] = x;
vis[x][cnt % 2] = true;
for(int i = 0; i < v[x].size(); i++)
{
int u = v[x][i];
if(!vis[u][(cnt + 1) % 2])dfs(u, cnt + 1);
}
if(cnt % 2 && v[x].size() == 0)
{
ans = cnt;
flag_win = true;
return;
}
if(cnt % 2 == 0 && !flag_win && !flag_draw)
{
flag_lose = true;
return;
}
}
int main()
{
while(scanf("%d %d", &n, &m) != EOF)
{
init(n);
for(int i = 1; i <= n; i++)
{
int num;
scanf("%d", &num);
for(int j = 0; j < num; j++)
{
int u;
scanf("%d", &u);
v[i].push_back(u);
}
}
int s;
scanf("%d", &s);
bfs(s);
dfs(s, 0);
if(flag_win)
{
printf("Win\n");
for(int i = 0; i <= ans; i++)
printf("%d ", path[i]);
printf("\n");
}
else if(flag_lose)
printf("Lose\n");
else printf("Draw\n");
}
return 0;
}